Intro
Renaming sheets in Excel VBA is a common task that can be achieved in several ways. Whether you're looking to rename a single sheet or multiple sheets, there are various methods to suit your needs. In this article, we'll explore five ways to rename sheets in Excel VBA, along with practical examples and code snippets to get you started.
The Importance of Renaming Sheets in Excel VBA
Renaming sheets in Excel VBA is essential for maintaining a well-organized and readable workbook. By giving your sheets descriptive names, you can easily identify their contents and make it easier for others to understand your workbook's structure. Additionally, renaming sheets can help you avoid confusion when working with multiple sheets that have similar names.
Method 1: Renaming a Single Sheet using VBA
To rename a single sheet using VBA, you can use the Name
property of the Worksheet
object. Here's an example code snippet:
Sub RenameSingleSheet()
' Declare the worksheet object
Dim ws As Worksheet
' Set the worksheet object to the sheet you want to rename
Set ws = ThisWorkbook.Worksheets("OldSheetName")
' Rename the sheet
ws.Name = "NewSheetName"
End Sub
Image:
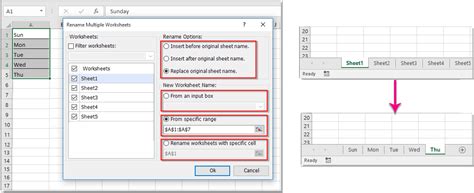
Method 2: Renaming Multiple Sheets using VBA
To rename multiple sheets using VBA, you can use a loop to iterate through the Worksheets
collection and rename each sheet individually. Here's an example code snippet:
Sub RenameMultipleSheets()
' Declare the worksheet object
Dim ws As Worksheet
' Loop through the worksheets collection
For Each ws In ThisWorkbook.Worksheets
' Rename the sheet
ws.Name = "NewSheetName_" & ws.Index
Next ws
End Sub
Image:
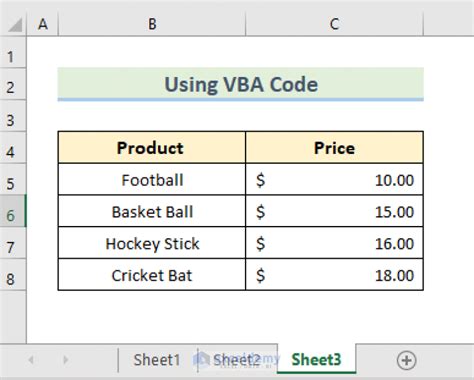
Method 3: Renaming Sheets using a Loop and a Counter
To rename sheets using a loop and a counter, you can use the For
loop to iterate through the Worksheets
collection and rename each sheet using a counter variable. Here's an example code snippet:
Sub RenameSheetsUsingLoopAndCounter()
' Declare the worksheet object and counter variable
Dim ws As Worksheet
Dim i As Integer
' Set the counter variable to 1
i = 1
' Loop through the worksheets collection
For Each ws In ThisWorkbook.Worksheets
' Rename the sheet using the counter variable
ws.Name = "NewSheetName_" & i
' Increment the counter variable
i = i + 1
Next ws
End Sub
Image:

Method 4: Renaming Sheets using an Array
To rename sheets using an array, you can store the new sheet names in an array and then use a loop to iterate through the array and rename each sheet. Here's an example code snippet:
Sub RenameSheetsUsingArray()
' Declare the worksheet object and array
Dim ws As Worksheet
Dim newSheetNames As Variant
' Set the array to the new sheet names
newSheetNames = Array("NewSheetName1", "NewSheetName2", "NewSheetName3")
' Loop through the worksheets collection
For i = 0 To UBound(newSheetNames)
' Set the worksheet object to the current sheet
Set ws = ThisWorkbook.Worksheets(i + 1)
' Rename the sheet using the array
ws.Name = newSheetNames(i)
Next i
End Sub
Image:
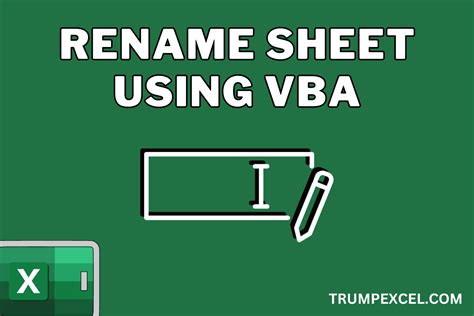
Method 5: Renaming Sheets using a User-Defined Function
To rename sheets using a user-defined function, you can create a function that takes the new sheet name as an argument and renames the sheet accordingly. Here's an example code snippet:
Function RenameSheet(ws As Worksheet, newSheetName As String) As Boolean
' Rename the sheet
ws.Name = newSheetName
' Return True if the sheet was renamed successfully
RenameSheet = True
End Function
Sub RenameSheetUsingFunction()
' Declare the worksheet object
Dim ws As Worksheet
' Set the worksheet object to the sheet you want to rename
Set ws = ThisWorkbook.Worksheets("OldSheetName")
' Rename the sheet using the user-defined function
If RenameSheet(ws, "NewSheetName") Then
MsgBox "Sheet renamed successfully"
Else
MsgBox "Error renaming sheet"
End If
End Sub
Image:
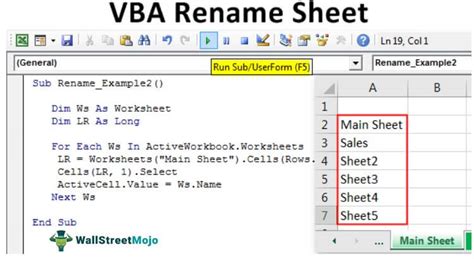
Gallery of Renaming Sheets in Excel VBA:
Renaming Sheets in Excel VBA Image Gallery
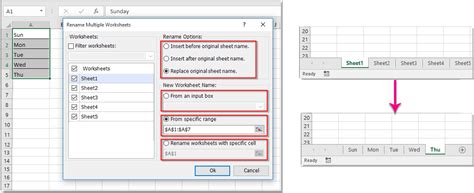
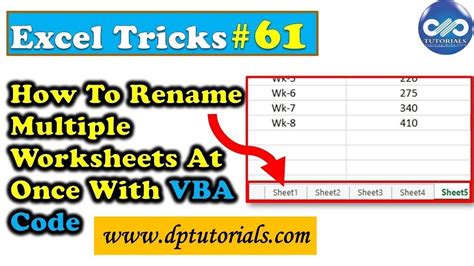
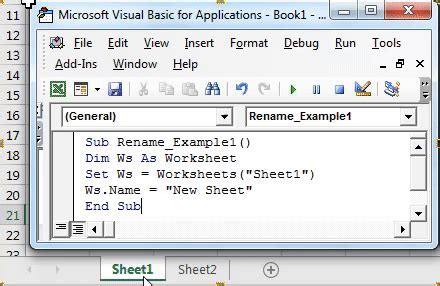
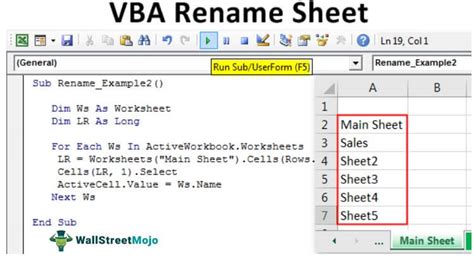
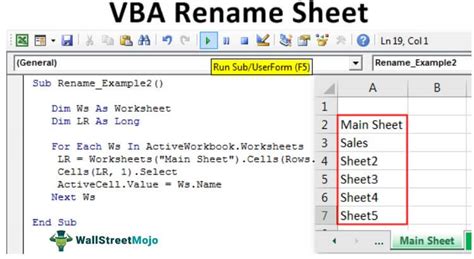
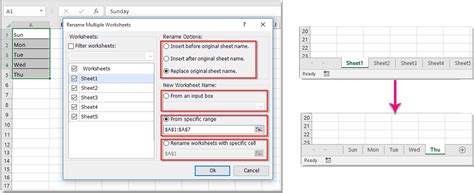
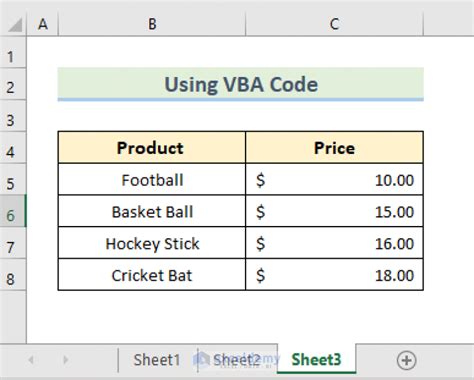
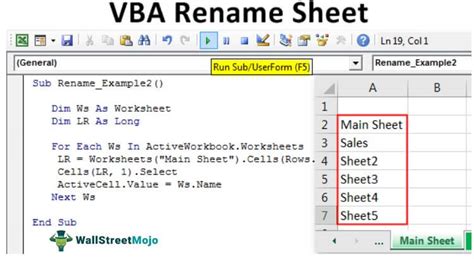
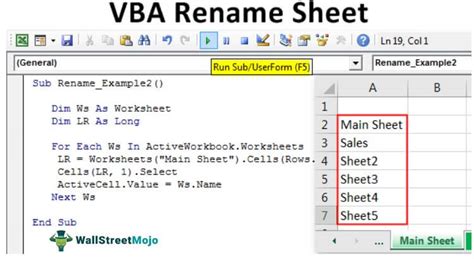
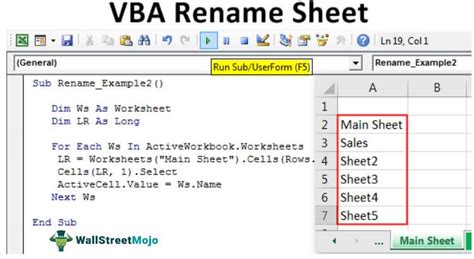
Conclusion
Renaming sheets in Excel VBA is a straightforward process that can be achieved using various methods. Whether you're looking to rename a single sheet or multiple sheets, there's a method that suits your needs. By using the techniques outlined in this article, you can rename sheets quickly and efficiently, making it easier to manage your worksheets and keep your data organized.
What's Next?
We hope you found this article helpful in renaming sheets in Excel VBA. If you have any questions or need further assistance, please don't hesitate to ask. In our next article, we'll explore more advanced topics in Excel VBA, such as working with charts and pivot tables. Stay tuned!