Intro
Discover how to rename worksheets in Excel VBA with ease. Learn 5 efficient methods to change worksheet names programmatically, including using the Worksheet.Name property, Range objects, and loops. Improve your Excel automation skills and optimize your spreadsheet management with these expert-approved VBA techniques for renaming worksheets.
Rename Worksheet in Excel VBA: 5 Effective Methods
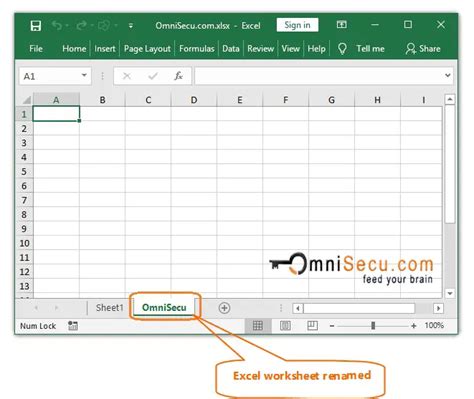
Renaming worksheets in Excel VBA can be a crucial task, especially when working with multiple worksheets or sheets. Having a clear and descriptive name for each worksheet can significantly improve the organization and readability of your Excel files. In this article, we will explore five effective methods to rename a worksheet in Excel VBA.
Method 1: Rename Worksheet using the Activate Method
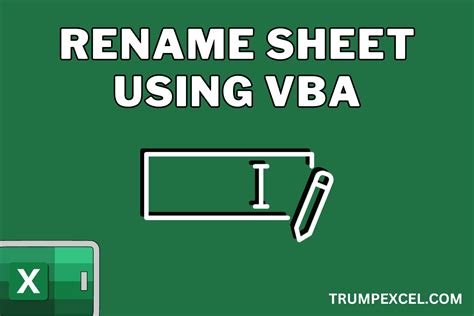
The first method involves using the Activate method to select the worksheet you want to rename. This method is straightforward and easy to implement. Here's an example code snippet that demonstrates how to rename a worksheet using the Activate method:
Sub RenameWorksheetActivate()
Worksheets("Sheet1").Activate
ActiveSheet.Name = "NewSheetName"
End Sub
How it works:
- The
Worksheets("Sheet1").Activate
line selects the worksheet named "Sheet1" and activates it. - The
ActiveSheet.Name = "NewSheetName"
line renames the active worksheet to "NewSheetName".
Method 2: Rename Worksheet using the Sheets Collection
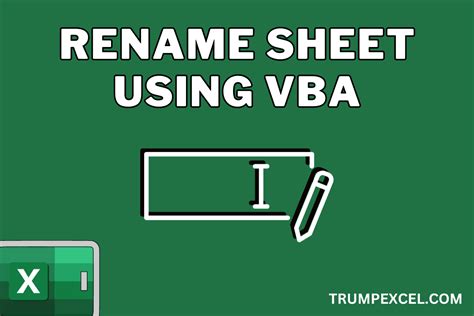
The second method involves using the Sheets collection to access the worksheet you want to rename. This method is useful when you need to rename multiple worksheets in a single code block. Here's an example code snippet that demonstrates how to rename a worksheet using the Sheets collection:
Sub RenameWorksheetSheetsCollection()
Sheets("Sheet1").Name = "NewSheetName"
End Sub
How it works:
- The
Sheets("Sheet1")
line accesses the worksheet named "Sheet1" using the Sheets collection. - The
.Name = "NewSheetName"
line renames the worksheet to "NewSheetName".
Method 3: Rename Worksheet using the Worksheets Collection
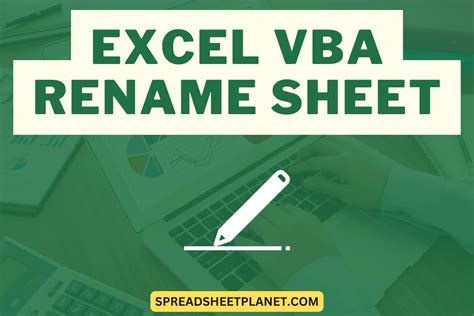
The third method involves using the Worksheets collection to access the worksheet you want to rename. This method is similar to the second method, but it uses the Worksheets collection instead of the Sheets collection. Here's an example code snippet that demonstrates how to rename a worksheet using the Worksheets collection:
Sub RenameWorksheetWorksheetsCollection()
Worksheets("Sheet1").Name = "NewSheetName"
End Sub
How it works:
- The
Worksheets("Sheet1")
line accesses the worksheet named "Sheet1" using the Worksheets collection. - The
.Name = "NewSheetName"
line renames the worksheet to "NewSheetName".
Method 4: Rename Worksheet using a Variable
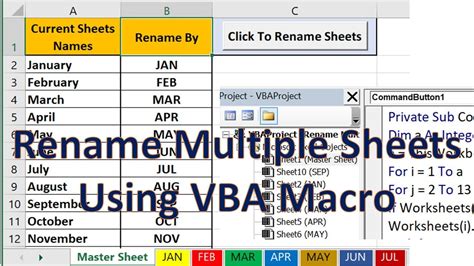
The fourth method involves using a variable to store the worksheet name and then renaming the worksheet using the variable. This method is useful when you need to rename multiple worksheets in a single code block. Here's an example code snippet that demonstrates how to rename a worksheet using a variable:
Sub RenameWorksheetVariable()
Dim ws As Worksheet
Set ws = Worksheets("Sheet1")
ws.Name = "NewSheetName"
End Sub
How it works:
- The
Dim ws As Worksheet
line declares a variablews
of type Worksheet. - The
Set ws = Worksheets("Sheet1")
line sets thews
variable to the worksheet named "Sheet1". - The
ws.Name = "NewSheetName"
line renames the worksheet to "NewSheetName".
Method 5: Rename Worksheet using a Loop
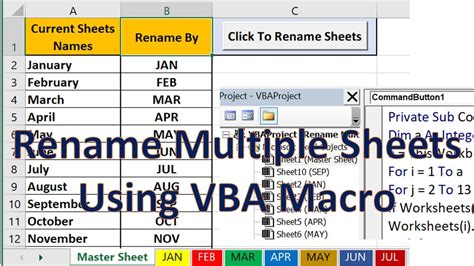
The fifth method involves using a loop to rename multiple worksheets in a single code block. This method is useful when you need to rename a large number of worksheets. Here's an example code snippet that demonstrates how to rename a worksheet using a loop:
Sub RenameWorksheetLoop()
Dim i As Integer
For i = 1 To 10
Worksheets(i).Name = "NewSheetName" & i
Next i
End Sub
How it works:
- The
Dim i As Integer
line declares a variablei
of type Integer. - The
For i = 1 To 10
line starts a loop that iterates from 1 to 10. - The
Worksheets(i).Name = "NewSheetName" & i
line renames each worksheet to "NewSheetName" followed by the loop counteri
.
Excel VBA Rename Worksheet Image Gallery
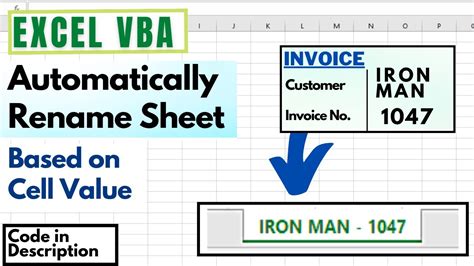
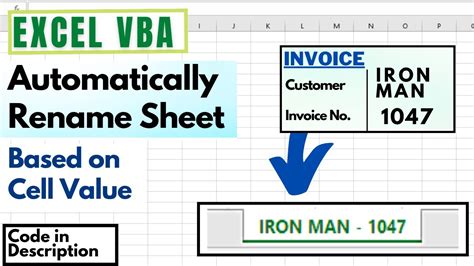
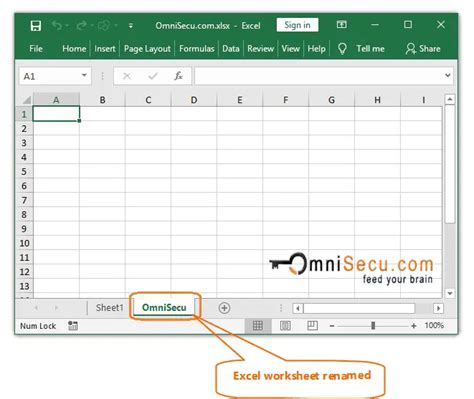
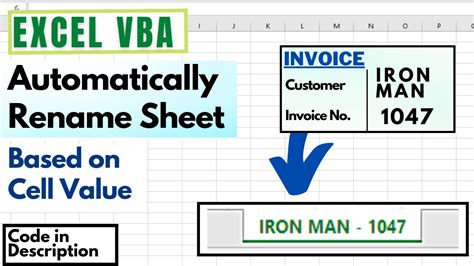
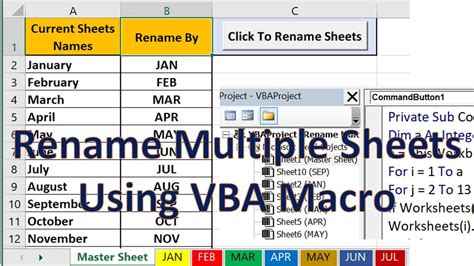
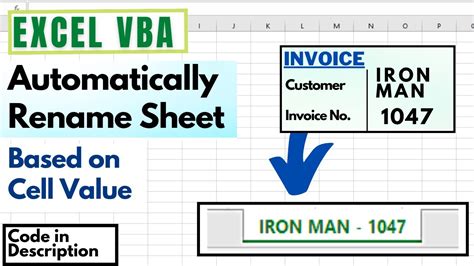
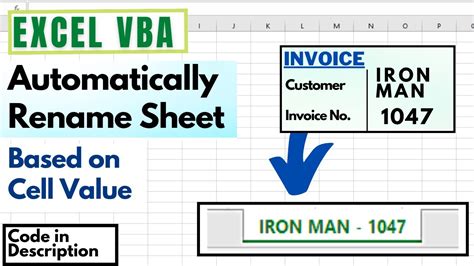
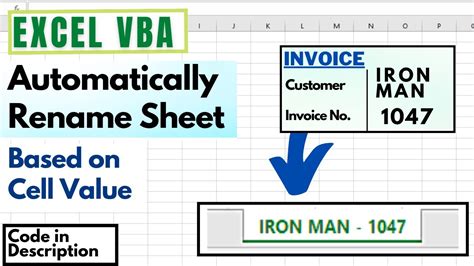
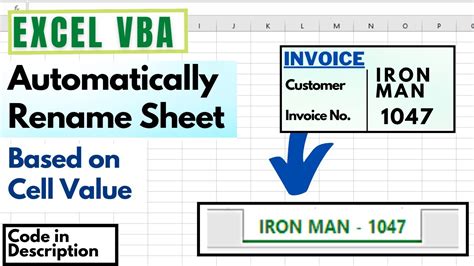
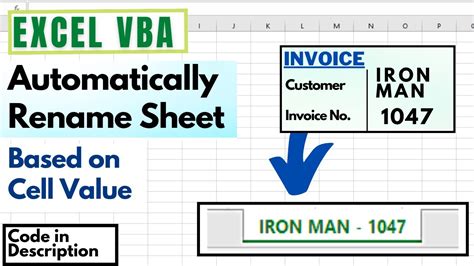
We hope this article has provided you with a comprehensive understanding of how to rename a worksheet in Excel VBA using different methods. Whether you're a beginner or an experienced VBA developer, these methods can help you rename worksheets efficiently and effectively. If you have any questions or need further assistance, please don't hesitate to ask. Share your thoughts and experiences in the comments section below.