The art of manipulating strings in Excel VBA! Replacing characters in a string is a common task, and Excel VBA provides several ways to achieve this. In this article, we'll explore the different methods to replace characters in a string using Excel VBA.
Why Replace Characters in a String?
Before we dive into the how-to, let's quickly discuss why you might need to replace characters in a string. Here are a few scenarios:
- Data cleaning: You might need to replace special characters or unwanted text in a dataset.
- Text processing: You might want to replace certain characters with others to make text more readable or compatible with other systems.
- String manipulation: You might need to replace characters to create a new string that meets specific requirements.
Method 1: Using the Replace Function
The Replace function is a straightforward way to replace characters in a string. The syntax is as follows:
Replace(expression, find, replacewith[, start[, count]])
expression
is the string you want to modify.find
is the character(s) you want to replace.replacewith
is the character(s) you want to replace with.start
is the position where you want to start the replacement (optional).count
is the number of replacements you want to make (optional).
Here's an example:
Sub ReplaceCharacters()
Dim str As String
str = "Hello, World!"
str = Replace(str, ",", ".")
MsgBox str ' Outputs: "Hello. World!"
End Sub
Method 2: Using the VBA RegExp Object
The VBA RegExp object provides a more powerful way to replace characters in a string using regular expressions. Here's an example:
Sub ReplaceCharactersRegExp()
Dim str As String
str = "Hello, World!"
With New RegExp
.Pattern = ","
.Replacement = "."
str =.Replace(str)
End With
MsgBox str ' Outputs: "Hello. World!"
End Sub
Method 3: Using the Split and Join Functions
Another way to replace characters in a string is to use the Split and Join functions. Here's an example:
Sub ReplaceCharactersSplitJoin()
Dim str As String
str = "Hello, World!"
str = Join(Split(str, ","), ".")
MsgBox str ' Outputs: "Hello. World!"
End Sub
Method 4: Using a Loop
If you need more control over the replacement process, you can use a loop to iterate through the string and replace characters manually. Here's an example:
Sub ReplaceCharactersLoop()
Dim str As String
str = "Hello, World!"
For i = 1 To Len(str)
If Mid(str, i, 1) = "," Then
Mid(str, i, 1) = "."
End If
Next i
MsgBox str ' Outputs: "Hello. World!"
End Sub
Conclusion
In this article, we've explored four different methods to replace characters in a string using Excel VBA. Each method has its strengths and weaknesses, and the choice of method depends on the specific requirements of your project. Whether you're a beginner or an experienced VBA developer, we hope this article has provided you with the knowledge and inspiration to tackle your string manipulation tasks with confidence.
Gallery of String Manipulation
String Manipulation Image Gallery
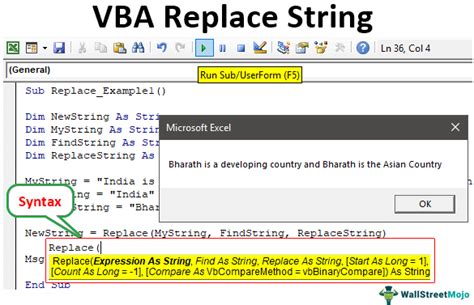
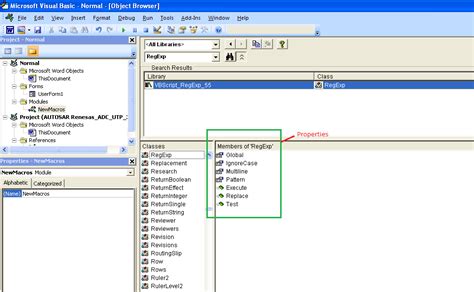
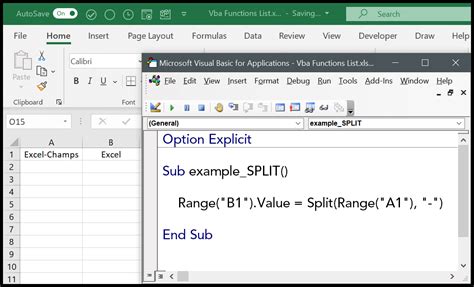
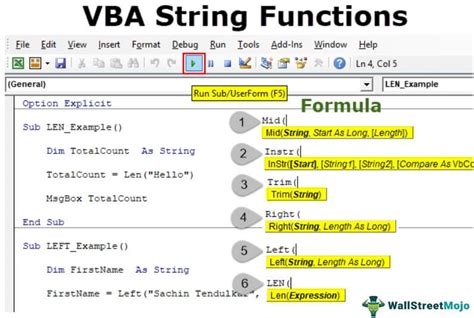
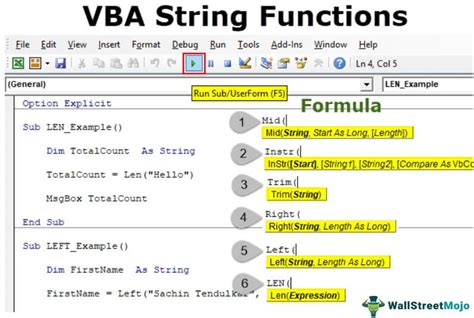
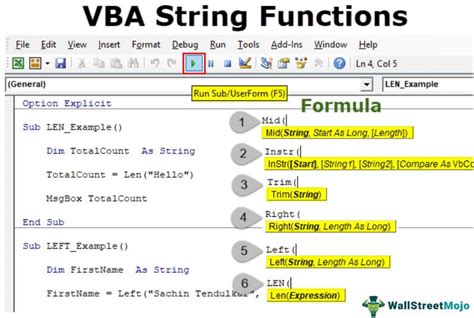
FAQs
- Q: What is the difference between the Replace function and the VBA RegExp object? A: The Replace function is a built-in VBA function that replaces characters in a string, while the VBA RegExp object provides more advanced regular expression-based string manipulation capabilities.
- Q: Can I use the Split and Join functions to replace characters in a string? A: Yes, you can use the Split and Join functions to replace characters in a string by splitting the string into an array, replacing the characters, and then joining the array back into a string.
- Q: How can I replace multiple characters in a string using VBA? A: You can use the Replace function or the VBA RegExp object to replace multiple characters in a string by specifying multiple replacement patterns or using regular expressions.