Intro
Discover how to set column width in Excel VBA with ease. Master the VBA code to automatically adjust column widths, making your spreadsheets more readable. Learn about AutoFit, column resizing, and VBA worksheet manipulation to enhance your Excel skills and streamline your workflow. Boost productivity and format your data with precision.
Adjusting column widths in Excel can be a tedious task, especially when working with large datasets. Fortunately, Excel VBA provides a simple way to automate this process. In this article, we will explore how to set column widths in Excel VBA easily.
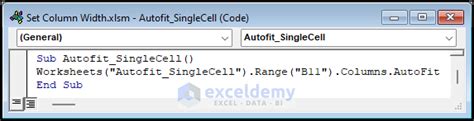
Why Use VBA to Set Column Widths?
Before we dive into the code, let's discuss why you might want to use VBA to set column widths in the first place. Here are a few reasons:
- Efficiency: Manually adjusting column widths can be time-consuming, especially when working with large datasets. VBA allows you to automate this process, saving you time and effort.
- Consistency: By using VBA to set column widths, you can ensure that your columns are consistently sized throughout your worksheet.
- Customization: VBA allows you to customize the column width settings to suit your specific needs.
Basic VBA Code to Set Column Widths
Here is some basic VBA code that sets the column width for a specific column:
Sub SetColumnWidth()
Columns("A").ColumnWidth = 20
End Sub
In this code, we're using the Columns
object to reference column A, and then setting the ColumnWidth
property to 20.
Setting Multiple Column Widths
To set multiple column widths, you can simply repeat the code for each column:
Sub SetColumnWidths()
Columns("A").ColumnWidth = 20
Columns("B").ColumnWidth = 15
Columns("C").ColumnWidth = 25
End Sub
Using a Loop to Set Column Widths
If you need to set column widths for a large number of columns, using a loop can be a more efficient approach:
Sub SetColumnWidthsLoop()
Dim i As Integer
For i = 1 To 10
Columns(i).ColumnWidth = 20
Next i
End Sub
In this code, we're using a For
loop to iterate through columns 1 to 10, and setting the column width to 20 for each column.
AutoFit Column Widths
If you want to automatically adjust the column widths to fit the contents of the cells, you can use the AutoFit
method:
Sub AutoFitColumnWidths()
Columns("A").AutoFit
End Sub
This code will automatically adjust the width of column A to fit the contents of the cells.
Best Practices for Setting Column Widths in VBA
Here are some best practices to keep in mind when setting column widths in VBA:
- Use meaningful variable names: Instead of using generic variable names like
i
, use more descriptive names likecolumnNumber
. - Use constants: Instead of hardcoding values like
20
, define constants to make your code more readable and maintainable. - Test your code: Before deploying your code, test it thoroughly to ensure that it works as expected.
Gallery of Setting Column Widths in Excel VBA
Setting Column Widths in Excel VBA Image Gallery
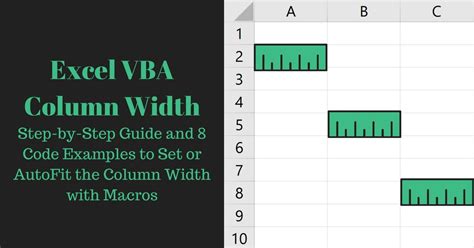
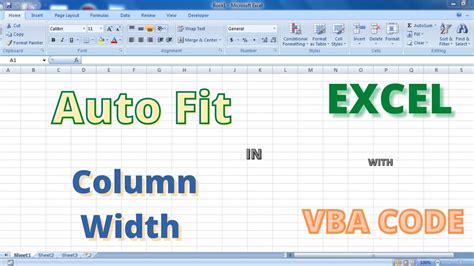
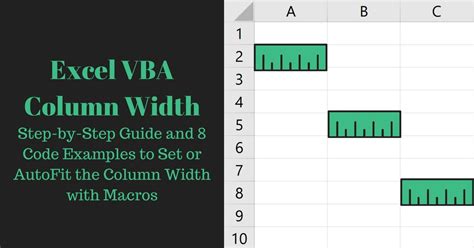
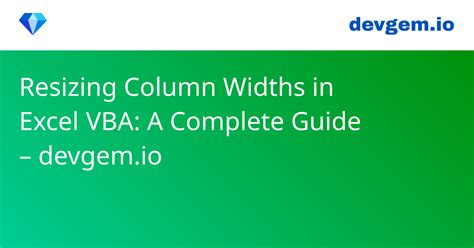
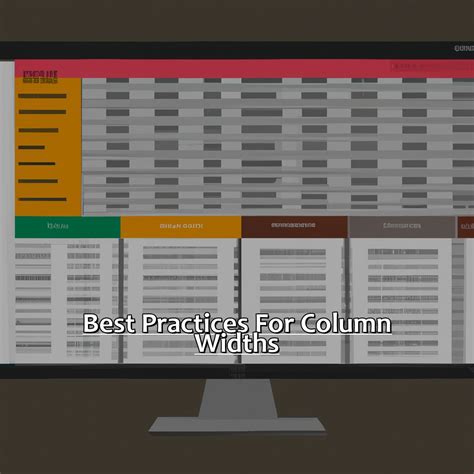
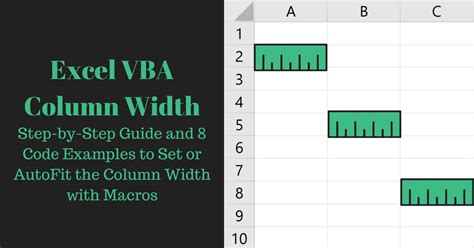
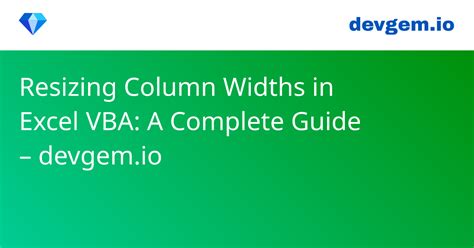
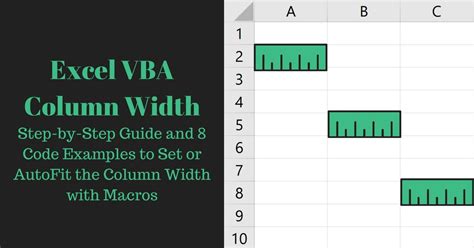
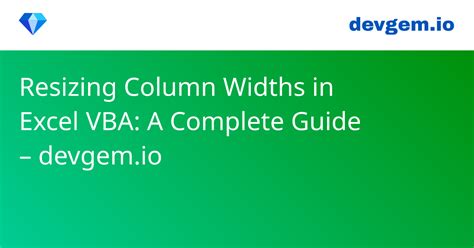
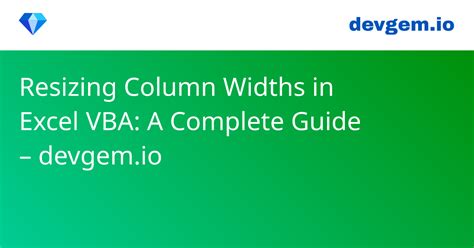
We hope this article has helped you learn how to set column widths in Excel VBA easily. With these tips and best practices, you'll be able to automate the process of adjusting column widths in your Excel worksheets. Do you have any questions or need further assistance? Leave a comment below!