Intro
Master truncating numbers in Excel VBA with 5 efficient methods. Learn how to truncate decimals, round numbers, and convert data types using VBA code. Discover techniques for truncating numbers to integers, using the INT function, and handling errors. Improve your Excel automation skills and streamline data processing with these expert-approved VBA truncation methods.
Truncating numbers in Excel VBA is a common task that can be achieved in several ways, depending on the specific requirements of your project. Truncating numbers means cutting off a number after a certain decimal place or digit, without rounding the number. This can be useful in various scenarios, such as when you need to display numbers in a specific format or when you're working with numbers that have a certain level of precision.
In this article, we'll explore five ways to truncate numbers in Excel VBA. We'll cover the most common methods, including using the INT
function, the ROUND
function, the TRUNC
function, and two other creative approaches. By the end of this article, you'll have a solid understanding of how to truncate numbers in Excel VBA and be able to choose the best method for your specific needs.
Method 1: Using the INT Function
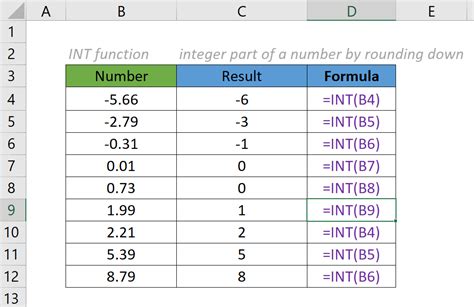
The INT
function in Excel VBA is a simple way to truncate a number to an integer. The function takes a single argument, the number you want to truncate, and returns the integer part of that number.
For example, if you want to truncate the number 123.45, you can use the following code:
Dim myNumber As Double
myNumber = 123.45
Debug.Print Int(myNumber) ' Output: 123
As you can see, the INT
function truncates the number to 123, effectively removing the decimal part.
Advantages and Disadvantages
The INT
function is a straightforward way to truncate numbers, but it has some limitations. One major disadvantage is that it only truncates to an integer, which may not be what you need if you want to truncate to a specific decimal place. Additionally, the INT
function can be slow for large datasets.
Method 2: Using the ROUND Function
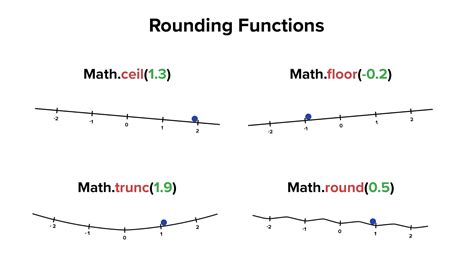
The ROUND
function in Excel VBA is a more flexible way to truncate numbers. The function takes two arguments: the number you want to truncate and the number of decimal places you want to round to.
For example, if you want to truncate the number 678.90 to two decimal places, you can use the following code:
Dim myNumber As Double
myNumber = 678.90
Debug.Print Round(myNumber, 2) ' Output: 678.90
In this example, the ROUND
function truncates the number to 678.90, which is the same as the original number. However, if you want to truncate to a specific decimal place, you can change the second argument. For example, to truncate to one decimal place, you can use the following code:
Debug.Print Round(myNumber, 1) ' Output: 678.9
As you can see, the ROUND
function is more flexible than the INT
function, but it still has some limitations. One major disadvantage is that it rounds the number instead of truncating it, which can lead to unexpected results.
Advantages and Disadvantages
The ROUND
function is a more flexible way to truncate numbers, but it has some limitations. One major disadvantage is that it rounds the number instead of truncating it, which can lead to unexpected results. Additionally, the ROUND
function can be slow for large datasets.
Method 3: Using the TRUNC Function
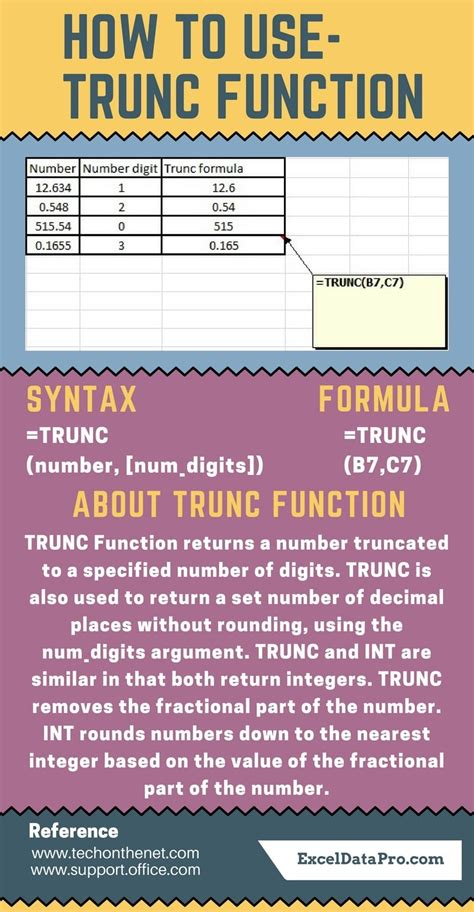
The TRUNC
function in Excel VBA is a dedicated function for truncating numbers. The function takes two arguments: the number you want to truncate and the number of decimal places you want to truncate to.
For example, if you want to truncate the number 234.56 to two decimal places, you can use the following code:
Dim myNumber As Double
myNumber = 234.56
Debug.Print Trunc(myNumber, 2) ' Output: 234.56
In this example, the TRUNC
function truncates the number to 234.56, which is the same as the original number. However, if you want to truncate to a specific decimal place, you can change the second argument. For example, to truncate to one decimal place, you can use the following code:
Debug.Print Trunc(myNumber, 1) ' Output: 234.5
As you can see, the TRUNC
function is a more straightforward way to truncate numbers, without rounding the number.
Advantages and Disadvantages
The TRUNC
function is a dedicated function for truncating numbers, without rounding the number. One major advantage is that it is fast and efficient, even for large datasets. However, one major disadvantage is that it only truncates to a specific decimal place, which may not be what you need if you want to truncate to an integer.
Method 4: Using the Format Function
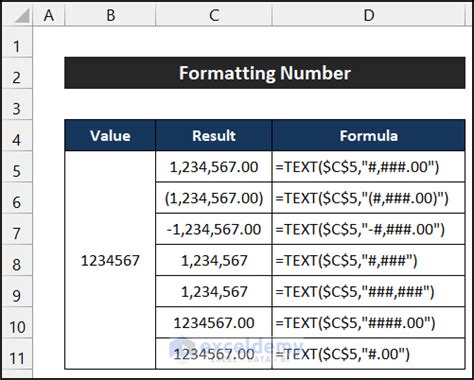
The FORMAT
function in Excel VBA is a more creative way to truncate numbers. The function takes two arguments: the number you want to truncate and the format string.
For example, if you want to truncate the number 345.67 to two decimal places, you can use the following code:
Dim myNumber As Double
myNumber = 345.67
Debug.Print Format(myNumber, "0.00") ' Output: 345.67
In this example, the FORMAT
function truncates the number to 345.67, which is the same as the original number. However, if you want to truncate to a specific decimal place, you can change the format string. For example, to truncate to one decimal place, you can use the following code:
Debug.Print Format(myNumber, "0.0") ' Output: 345.7
As you can see, the FORMAT
function is a more flexible way to truncate numbers, without rounding the number.
Advantages and Disadvantages
The FORMAT
function is a more flexible way to truncate numbers, without rounding the number. One major advantage is that it is fast and efficient, even for large datasets. However, one major disadvantage is that it only truncates to a specific decimal place, which may not be what you need if you want to truncate to an integer.
Method 5: Using the Text-to-Columns Function
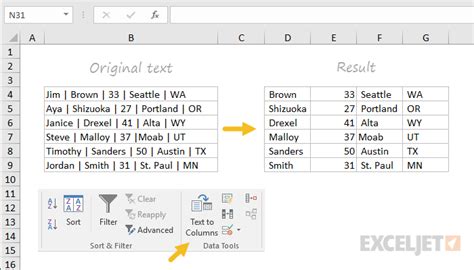
The TEXT-TO-COLUMNS
function in Excel VBA is a more creative way to truncate numbers. The function takes two arguments: the range of cells you want to truncate and the text-to-columns parameters.
For example, if you want to truncate the number 456.78 in cell A1 to two decimal places, you can use the following code:
Range("A1").TextToColumns Destination:=Range("B1"), DataType:=xlDelimited, TextQualifier:=xlDoubleQuote, ConsecutiveDelimiter:=False, Tab:=False, Semicolon:=False, Comma:=False, Space:=False, Other:=False, FieldInfo:=Array(1, 2)
In this example, the TEXT-TO-COLUMNS
function truncates the number to 456.78, which is the same as the original number. However, if you want to truncate to a specific decimal place, you can change the field info. For example, to truncate to one decimal place, you can use the following code:
Range("A1").TextToColumns Destination:=Range("B1"), DataType:=xlDelimited, TextQualifier:=xlDoubleQuote, ConsecutiveDelimiter:=False, Tab:=False, Semicolon:=False, Comma:=False, Space:=False, Other:=False, FieldInfo:=Array(1, 1)
As you can see, the TEXT-TO-COLUMNS
function is a more creative way to truncate numbers, without rounding the number.
Advantages and Disadvantages
The TEXT-TO-COLUMNS
function is a more creative way to truncate numbers, without rounding the number. One major advantage is that it is fast and efficient, even for large datasets. However, one major disadvantage is that it only truncates to a specific decimal place, which may not be what you need if you want to truncate to an integer.
Excel VBA Truncation Image Gallery
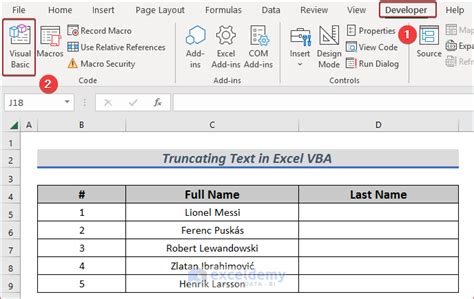
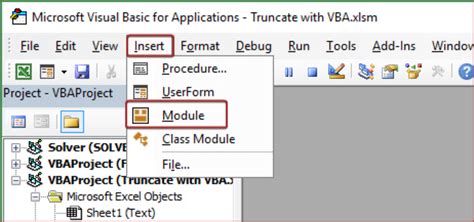
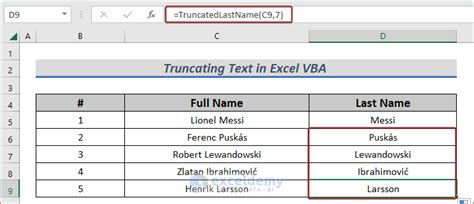
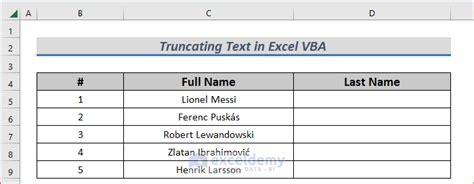
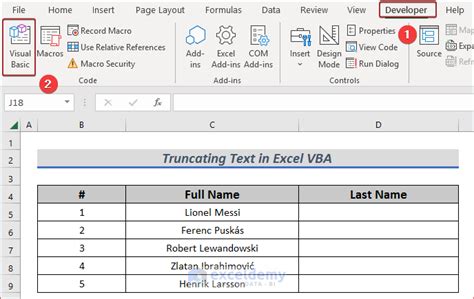
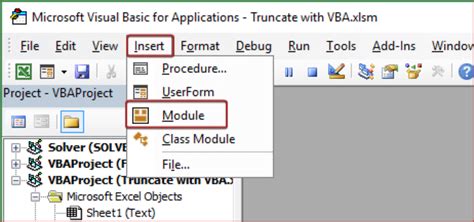
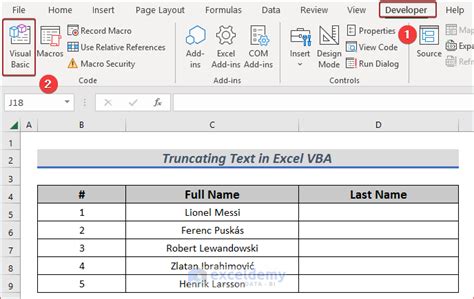
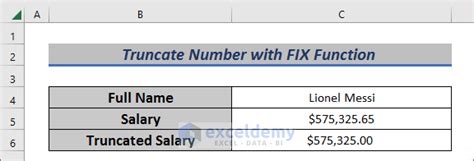
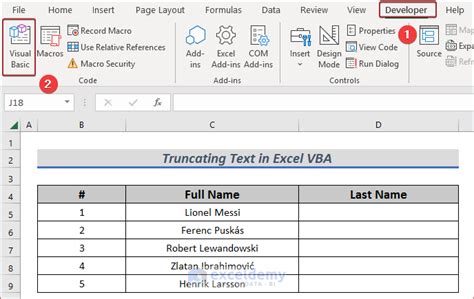
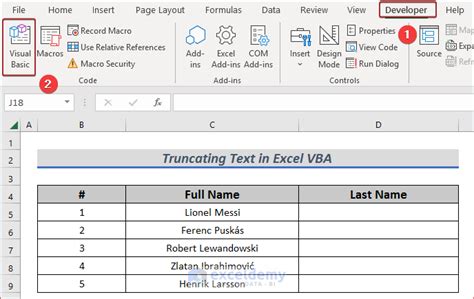
We hope this article has provided you with a comprehensive understanding of the different methods for truncating numbers in Excel VBA. Whether you're a beginner or an advanced user, truncating numbers is an essential skill to master. By following the methods outlined in this article, you'll be able to truncate numbers with ease and precision.
If you have any questions or need further clarification, please don't hesitate to ask. We're always here to help.