Intro
Master VBA programming with 5 expert ways to utilize Exit Sub, streamlining your code and improving performance. Learn how to effectively exit subroutines, handle errors, and optimize execution using Exit Sub in VBA, including best practices for sub procedure design and error handling.
Exit Sub is a powerful tool in VBA (Visual Basic for Applications) that allows developers to control the flow of their code. It is a statement that immediately exits a subroutine, transferring control to the statement following the call to the subroutine. In this article, we will explore five ways to use Exit Sub in VBA, including its benefits, syntax, and examples.
What is Exit Sub?
Exit Sub is a statement used in VBA to exit a subroutine prematurely. When a subroutine encounters an Exit Sub statement, it immediately terminates and control is returned to the calling procedure. This can be useful in a variety of situations, such as when an error occurs, when a condition is met, or when a subroutine needs to be terminated early.
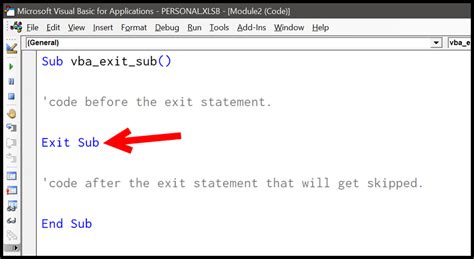
Benefits of Using Exit Sub
Using Exit Sub in VBA has several benefits, including:
- Improved Code Readability: By using Exit Sub, you can make your code more readable by reducing the number of nested If statements and Goto statements.
- Error Handling: Exit Sub can be used to handle errors in a subroutine by exiting the subroutine when an error occurs.
- Performance: Using Exit Sub can improve the performance of your code by reducing the number of unnecessary calculations.
5 Ways to Use Exit Sub in VBA
Here are five ways to use Exit Sub in VBA:
1. Exiting a Subroutine on Error
One common use of Exit Sub is to exit a subroutine when an error occurs. This can be achieved by using the On Error statement to trap errors and then using Exit Sub to exit the subroutine.
Sub Example1()
On Error GoTo ErrorHandler
' Code that may cause an error
Exit Sub
ErrorHandler:
MsgBox "An error occurred"
Exit Sub
End Sub
2. Exiting a Subroutine Based on a Condition
Another way to use Exit Sub is to exit a subroutine based on a condition. This can be achieved by using an If statement to check the condition and then using Exit Sub to exit the subroutine if the condition is met.
Sub Example2()
If Range("A1").Value = "Exit" Then
Exit Sub
End If
' Code that will only be executed if the condition is not met
End Sub
3. Exiting a Subroutine from a Loop
Exit Sub can also be used to exit a subroutine from a loop. This can be achieved by using a Do or For loop and then using Exit Sub to exit the subroutine when a condition is met.
Sub Example3()
Dim i As Integer
For i = 1 To 10
If Range("A" & i).Value = "Exit" Then
Exit Sub
End If
Next i
End Sub
4. Exiting a Subroutine to Transfer Control to Another Subroutine
Exit Sub can also be used to transfer control to another subroutine. This can be achieved by using the Call statement to call another subroutine and then using Exit Sub to exit the current subroutine.
Sub Example4()
Call AnotherSub
Exit Sub
End Sub
Sub AnotherSub()
' Code that will be executed after Example4 exits
End Sub
5. Exiting a Subroutine to Transfer Control to a Specific Line of Code
Finally, Exit Sub can be used to transfer control to a specific line of code. This can be achieved by using a GoSub statement to jump to a specific line of code and then using Exit Sub to exit the subroutine.
Sub Example5()
GoSub MyLabel
Exit Sub
MyLabel:
' Code that will be executed after Example5 exits
End Sub
Exit Sub VBA Gallery
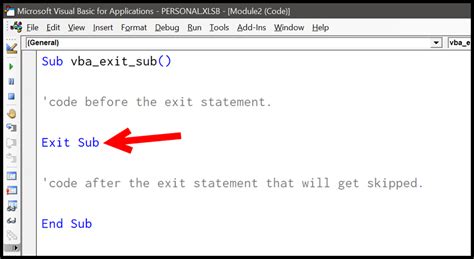
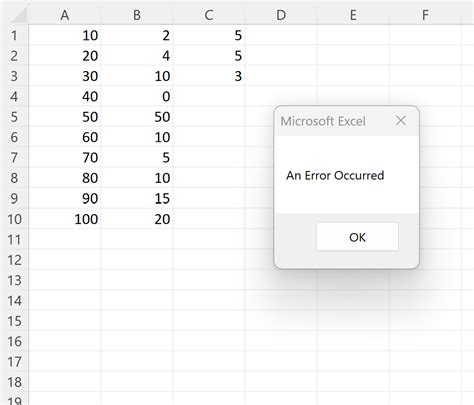
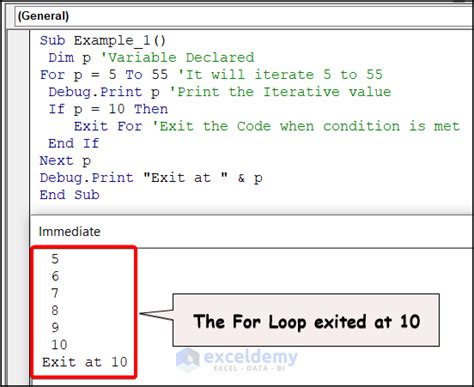
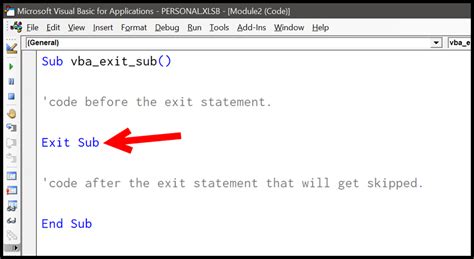
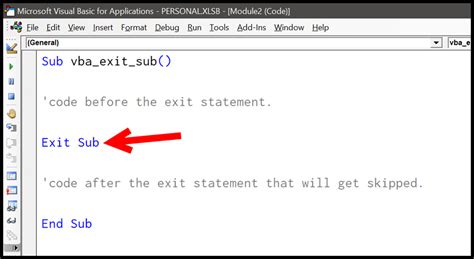
Conclusion
In conclusion, Exit Sub is a powerful tool in VBA that can be used to control the flow of your code. By using Exit Sub, you can improve the readability of your code, handle errors, and transfer control to other subroutines or specific lines of code. Whether you are a beginner or an experienced VBA developer, understanding how to use Exit Sub can help you write more efficient and effective code.
FAQs
Q: What is Exit Sub in VBA? A: Exit Sub is a statement used in VBA to exit a subroutine prematurely.
Q: How do I use Exit Sub in VBA? A: You can use Exit Sub in VBA by inserting the statement into your code where you want to exit the subroutine.
Q: Can I use Exit Sub to transfer control to another subroutine? A: Yes, you can use Exit Sub to transfer control to another subroutine by using the Call statement to call the other subroutine.
Q: Can I use Exit Sub to transfer control to a specific line of code? A: Yes, you can use Exit Sub to transfer control to a specific line of code by using a GoSub statement to jump to the specific line of code.