Intro
Resolve the Object Expected error in VBA with ease. Learn how to troubleshoot and fix this common error in Visual Basic for Applications. Discover the causes, symptoms, and step-by-step solutions to get your VBA code running smoothly. Master error handling and debugging techniques to optimize your VBA projects.
What is the "Object Expected" Error in VBA?
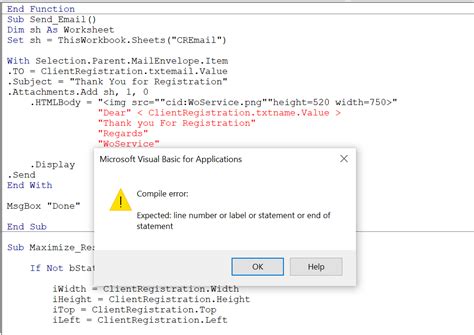
The "Object Expected" error is a common error in Visual Basic for Applications (VBA) that occurs when the code is expecting an object reference, but instead receives a value or a variable that is not an object. This error can be frustrating to troubleshoot, especially for beginners. In this article, we will explore the causes of this error and provide step-by-step solutions to fix it.
Causes of the "Object Expected" Error
The "Object Expected" error can occur due to various reasons, including:
- Using a variable that is not declared as an object
- Using a value or a variable that is not an object reference
- Misspelling object names or property names
- Using an object that has not been initialized or set
- Using an object that is not supported in the current application or version
Fixing the "Object Expected" Error
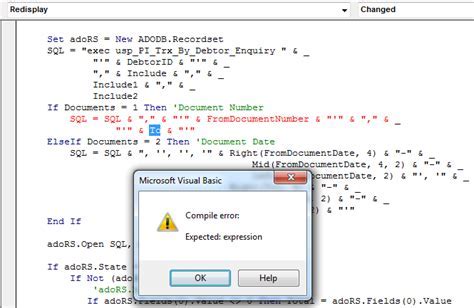
To fix the "Object Expected" error, follow these steps:
Step 1: Check Variable Declarations
Ensure that the variable is declared as an object using the As Object
keyword.
Dim obj As Object
Step 2: Check Object References
Verify that the object reference is correct and the object has been initialized or set.
Set obj = CreateObject("Excel.Application")
Step 3: Check Spelling and Case
Ensure that object names, property names, and method names are spelled correctly and in the correct case.
obj.Range("A1").Value = "Hello World"
Step 4: Check Object Support
Verify that the object is supported in the current application or version.
If obj Is Nothing Then
MsgBox "Object is not supported"
End If
Step 5: Use Early Binding
Using early binding can help to catch errors at compile-time rather than runtime.
Dim obj As Excel.Application
Common "Object Expected" Error Scenarios
Here are some common scenarios where the "Object Expected" error may occur:
- Using the
Range
object in Excel VBA without declaring it as an object
Dim rng As Range
Set rng = Range("A1")
- Using the
Workbook
object in Excel VBA without declaring it as an object
Dim wb As Workbook
Set wb = Workbooks.Add
- Using the
ADO
object in Access VBA without declaring it as an object
Dim ado As ADODB.Connection
Set ado = New ADODB.Connection
Gallery of VBA Error Handling
VBA Error Handling Gallery
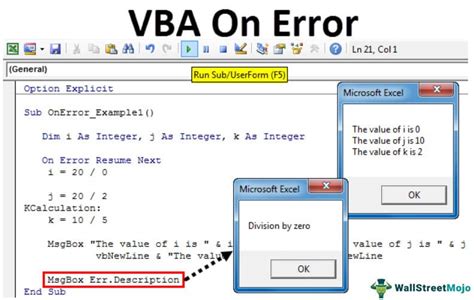
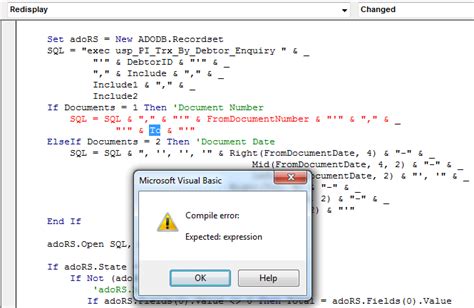
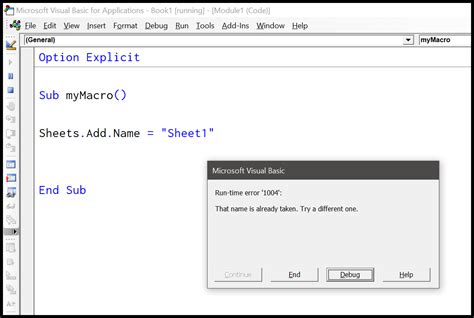
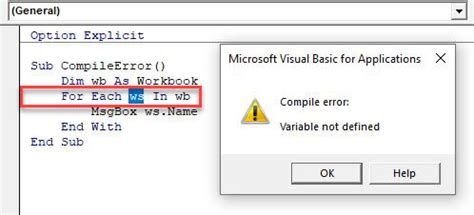
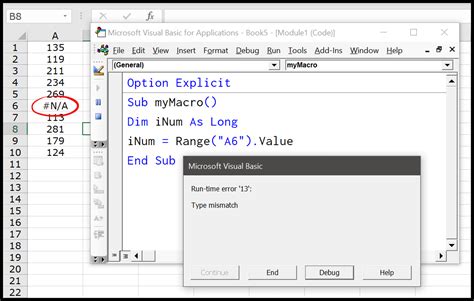
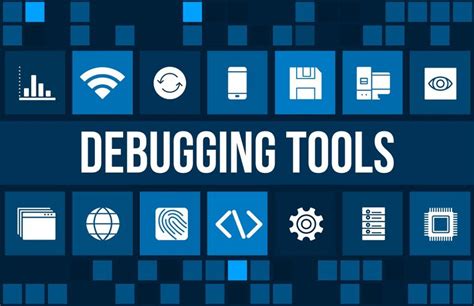
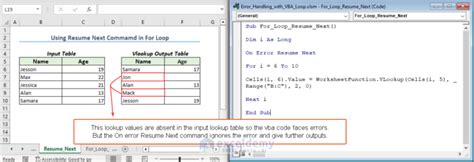
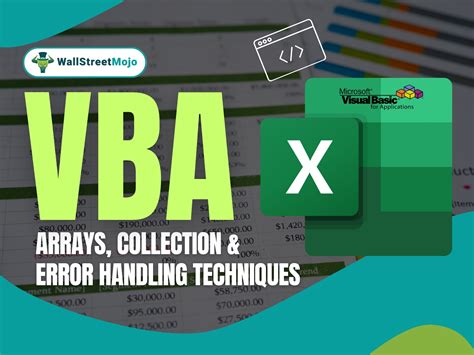
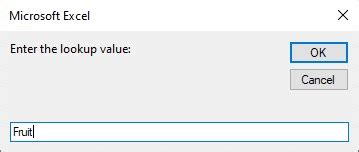
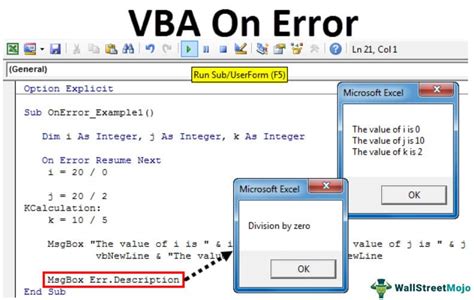
Conclusion
The "Object Expected" error is a common error in VBA that can be fixed by following the steps outlined in this article. By checking variable declarations, object references, spelling and case, object support, and using early binding, developers can troubleshoot and fix this error. Additionally, understanding common error scenarios and using error handling techniques can help to improve the overall quality and reliability of VBA code.
We hope this article has been helpful in fixing the "Object Expected" error in VBA. If you have any further questions or need additional assistance, please don't hesitate to comment below.