Intro
Discover how to integrate Flask with Bootstrap 5 templates to create robust and visually appealing web applications. Learn five practical ways to leverage Flasks flexibility with Bootstrap 5s responsive design, including template inheritance, CSS customization, and UI component integration. Boost your web development skills and create stunning web apps with ease.
Building web applications with Flask is a popular choice among developers, given its lightweight and flexible nature. However, creating a visually appealing user interface can be a challenge. That's where Bootstrap 5 comes in - a powerful front-end framework that helps create responsive and mobile-first UI components. In this article, we'll explore five ways to use Flask with a Bootstrap 5 template to create stunning web applications.
Why Use Bootstrap 5 with Flask?
Before diving into the ways to use Flask with Bootstrap 5, let's quickly discuss why this combination is a great choice. Bootstrap 5 provides a set of pre-designed UI components, layouts, and utilities that make it easy to create responsive and consistent interfaces. By combining Bootstrap 5 with Flask, you can leverage the strengths of both frameworks to build robust and visually appealing web applications.
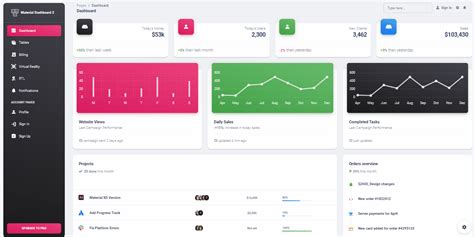
1. Using a Pre-Existing Bootstrap 5 Template
One of the easiest ways to use Flask with Bootstrap 5 is to use a pre-existing template. There are many free and paid Bootstrap 5 templates available online that you can download and use with your Flask application. Simply create a new Flask project, download a Bootstrap 5 template, and copy the template files into your project directory.
Steps to Use a Pre-Existing Template:
- Download a Bootstrap 5 template from a reputable source.
- Create a new Flask project using
flask new myapp
. - Copy the template files into your project directory.
- Update the template files to reference your Flask application's routes and views.
Example:
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def index():
return render_template("index.html")
if __name__ == "__main__":
app.run(debug=True)
2. Creating a Custom Bootstrap 5 Template
If you prefer to create a custom Bootstrap 5 template from scratch, you can do so using the Bootstrap 5 CSS and JavaScript files. This approach requires more effort, but provides complete control over the design and layout of your application.
Steps to Create a Custom Template:
- Create a new Flask project using
flask new myapp
. - Download the Bootstrap 5 CSS and JavaScript files from the official Bootstrap website.
- Create a new directory for your template files and add the Bootstrap 5 files.
- Create a new HTML file for your application's layout and add the necessary Bootstrap 5 classes and components.
Example:
My App
Welcome to My App!
3. Using a Flask-Bootstrap Extension
Flask-Bootstrap is a popular extension that provides a set of helpers and templates for using Bootstrap 5 with Flask. This extension simplifies the process of using Bootstrap 5 with Flask and provides a set of pre-defined templates and layouts.
Steps to Use Flask-Bootstrap:
- Install Flask-Bootstrap using
pip install flask-bootstrap
. - Create a new Flask project using
flask new myapp
. - Import Flask-Bootstrap in your application and initialize it.
- Use the Flask-Bootstrap templates and helpers to create your application's UI.
Example:
from flask import Flask, render_template
from flask_bootstrap import Bootstrap
app = Flask(__name__)
bootstrap = Bootstrap(app)
@app.route("/")
def index():
return render_template("index.html")
if __name__ == "__main__":
app.run(debug=True)
4. Using a Template Engine
Flask provides a built-in template engine called Jinja2 that allows you to render templates with dynamic data. You can use Jinja2 to render Bootstrap 5 templates with dynamic data from your Flask application.
Steps to Use Jinja2:
- Create a new Flask project using
flask new myapp
. - Create a new directory for your template files and add a new Jinja2 template file.
- Use Jinja2 syntax to render dynamic data in your template file.
- Use the
render_template
function to render the template file with dynamic data.
Example:
My App
Welcome to My App!
5. Using a Third-Party Library
There are many third-party libraries available that provide Bootstrap 5 integration with Flask. One popular library is Flask-Admin, which provides a set of tools for building administrative interfaces with Flask.
Steps to Use Flask-Admin:
- Install Flask-Admin using
pip install flask-admin
. - Create a new Flask project using
flask new myapp
. - Import Flask-Admin in your application and initialize it.
- Use the Flask-Admin templates and helpers to create your application's administrative interface.
Example:
from flask import Flask, render_template
from flask_admin import Admin
app = Flask(__name__)
admin = Admin(app, name="My App", template_mode="bootstrap5")
@app.route("/")
def index():
return render_template("index.html")
if __name__ == "__main__":
app.run(debug=True)
In conclusion, there are many ways to use Flask with Bootstrap 5 templates to create stunning web applications. Whether you choose to use a pre-existing template, create a custom template, or use a third-party library, the combination of Flask and Bootstrap 5 provides a powerful and flexible way to build robust and visually appealing web applications.
Gallery of Bootstrap 5 Templates
Bootstrap 5 Templates










We hope this article has provided you with a comprehensive guide to using Flask with Bootstrap 5 templates. Whether you're a seasoned developer or just starting out, the combination of Flask and Bootstrap 5 provides a powerful and flexible way to build robust and visually appealing web applications.