Intro
Master VBA looping techniques with our expert guide on 5 ways to loop through worksheets in a workbook. Discover efficient methods using For Each, For Next, and Do While loops, and learn how to dynamically iterate through worksheets using VBA code, improving your Excel automation skills and productivity.
Looping through worksheets in a workbook is a common task in VBA programming, and there are several ways to achieve this. In this article, we will explore five different methods to loop through worksheets in a workbook using VBA.
The Importance of Looping Through Worksheets
In many cases, you may need to perform a specific task on multiple worksheets within a workbook. This could be formatting data, inserting charts, or even deleting unnecessary worksheets. Looping through worksheets allows you to automate these tasks and save time.
Method 1: Using the For Each Loop
One of the simplest ways to loop through worksheets is by using the For Each loop. This method is easy to understand and implement, making it a great starting point for beginners.
Sub LoopThroughWorksheetsForEach()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
'Perform actions on each worksheet
MsgBox ws.Name
Next ws
End Sub
In this example, the For Each loop iterates through each worksheet in the active workbook. The ws
variable represents the current worksheet being processed.
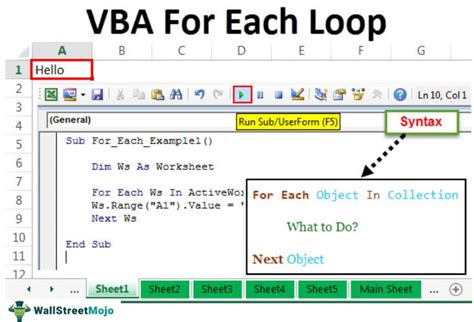
Method 2: Using the For Next Loop
Another way to loop through worksheets is by using the For Next loop. This method is useful when you need to iterate through worksheets in a specific order.
Sub LoopThroughWorksheetsForNext()
Dim i As Integer
For i = 1 To ThisWorkbook.Worksheets.Count
'Perform actions on each worksheet
MsgBox ThisWorkbook.Worksheets(i).Name
Next i
End Sub
In this example, the For Next loop iterates through each worksheet in the active workbook using the worksheet index.
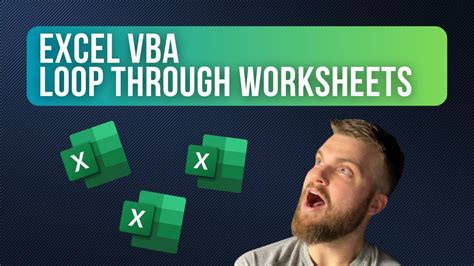
Method 3: Using the While Loop
The While loop is another option for looping through worksheets. This method is useful when you need to iterate through worksheets based on a specific condition.
Sub LoopThroughWorksheetsWhile()
Dim i As Integer
i = 1
While i <= ThisWorkbook.Worksheets.Count
'Perform actions on each worksheet
MsgBox ThisWorkbook.Worksheets(i).Name
i = i + 1
Wend
End Sub
In this example, the While loop iterates through each worksheet in the active workbook using a counter variable.
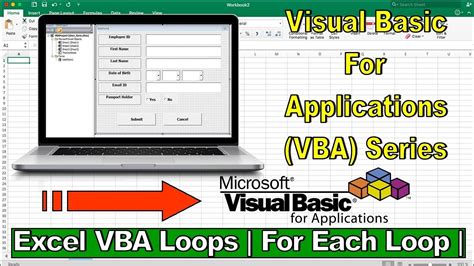
Method 4: Using the Do While Loop
The Do While loop is similar to the While loop, but it checks the condition at the end of the loop.
Sub LoopThroughWorksheetsDoWhile()
Dim i As Integer
i = 1
Do While i <= ThisWorkbook.Worksheets.Count
'Perform actions on each worksheet
MsgBox ThisWorkbook.Worksheets(i).Name
i = i + 1
Loop
End Sub
In this example, the Do While loop iterates through each worksheet in the active workbook using a counter variable.
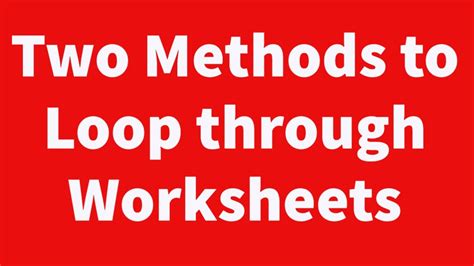
Method 5: Using the For Each Loop with Worksheet Array
Another way to loop through worksheets is by using the For Each loop with a worksheet array.
Sub LoopThroughWorksheetsForEachArray()
Dim wsArray() As Worksheet
Dim ws As Worksheet
ReDim wsArray(1 To ThisWorkbook.Worksheets.Count)
For Each ws In ThisWorkbook.Worksheets
'Add worksheet to array
wsArray(ws.Index) = ws
Next ws
'Loop through worksheet array
For Each ws In wsArray
'Perform actions on each worksheet
MsgBox ws.Name
Next ws
End Sub
In this example, the For Each loop iterates through each worksheet in the active workbook and adds it to a worksheet array. The array is then looped through to perform actions on each worksheet.
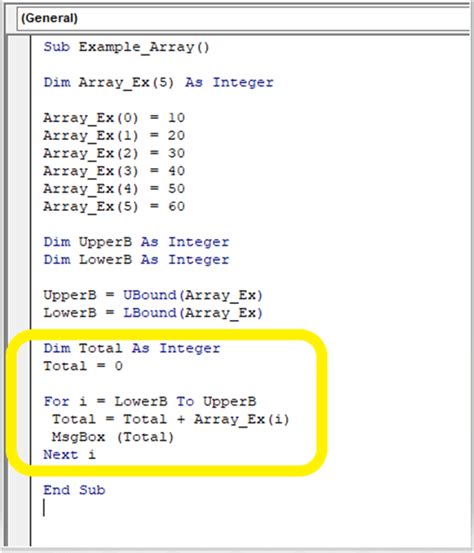
Gallery of Looping Through Worksheets
Looping Through Worksheets
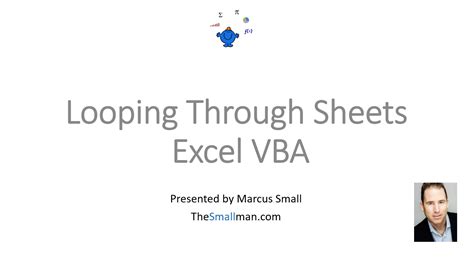
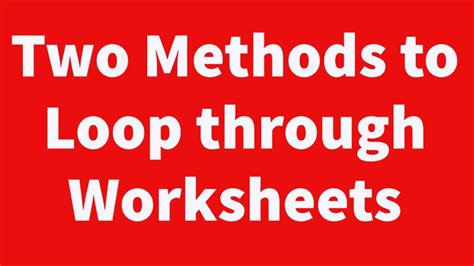
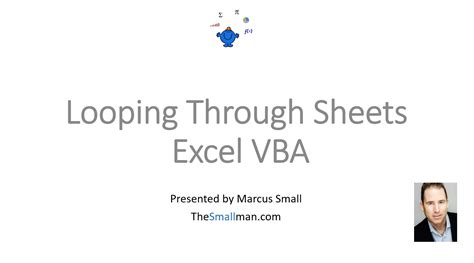
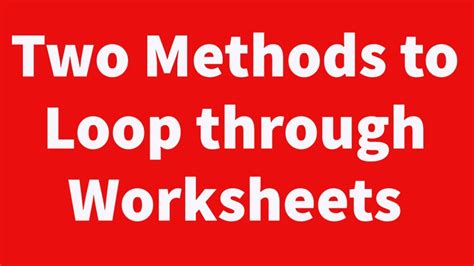
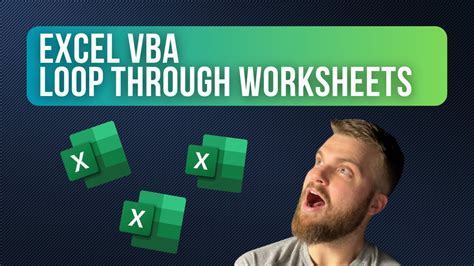
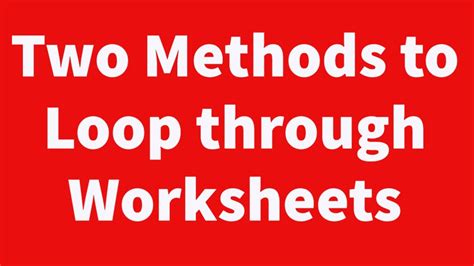
We hope this article has provided you with a comprehensive understanding of the different methods to loop through worksheets in a workbook using VBA. Whether you're a beginner or an experienced programmer, these methods will help you automate tasks and improve your productivity. If you have any questions or need further assistance, please don't hesitate to comment below.