Intro
Formatting dates in VBA can be a daunting task, especially for those who are new to programming. However, with the right guidance, it can be made easy. In this article, we will explore the different ways to format dates in VBA, including using the Format function, the DateSerial function, and the DateAdd function.
Understanding Dates in VBA
Before we dive into formatting dates, it's essential to understand how VBA handles dates. In VBA, dates are stored as serial numbers, with January 1, 1900, being the first serial number (1). Each subsequent day is represented by a unique serial number. This makes it easy to perform calculations and comparisons with dates.
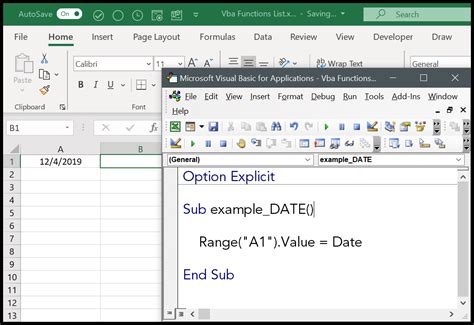
Using the Format Function
The Format function is one of the most commonly used functions in VBA for formatting dates. This function takes a date value and returns a string representation of that date in the desired format.
Sub FormatDate()
Dim dt As Date
dt = #1/1/2022#
MsgBox Format(dt, "yyyy-mm-dd") ' Output: 2022-01-01
End Sub
In the above example, the Format function is used to format the date January 1, 2022, into the string "2022-01-01".
Common Date Formats
Here are some common date formats used in VBA:
- "yyyy-mm-dd" - Year-Month-Day (e.g., 2022-01-01)
- "dd-mmm-yyyy" - Day-Month-Year (e.g., 01-Jan-2022)
- "mm/dd/yyyy" - Month/Day/Year (e.g., 01/01/2022)
- "dddd, mmmm dd, yyyy" - Full Date (e.g., Friday, January 01, 2022)
Using the DateSerial Function
The DateSerial function is used to create a date value from individual year, month, and day components.
Sub CreateDate()
Dim dt As Date
dt = DateSerial(2022, 1, 1)
MsgBox dt ' Output: 1/1/2022
End Sub
In the above example, the DateSerial function is used to create the date January 1, 2022.
Using the DateAdd Function
The DateAdd function is used to add or subtract a specified interval from a date value.
Sub AddDate()
Dim dt As Date
dt = #1/1/2022#
MsgBox DateAdd("d", 7, dt) ' Output: 1/8/2022
End Sub
In the above example, the DateAdd function is used to add 7 days to the date January 1, 2022.
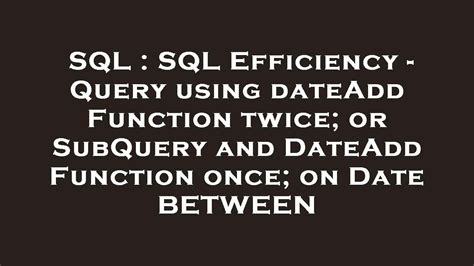
Practical Examples
Here are some practical examples of formatting dates in VBA:
- Create a date range:
Format(dtStart, "yyyy-mm-dd") & " to " & Format(dtEnd, "yyyy-mm-dd")
- Calculate the difference between two dates:
DateDiff("d", dtStart, dtEnd)
- Add a specified interval to a date:
DateAdd("m", 3, dt)
Gallery of VBA Date Formatting Examples
VBA Date Formatting Examples
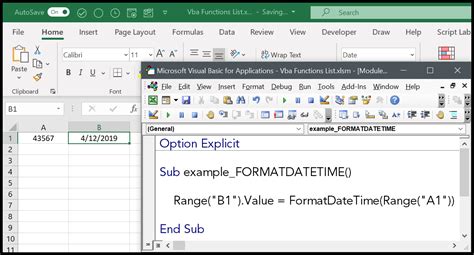
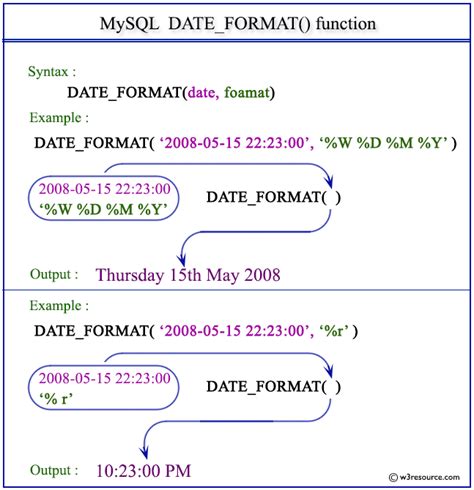
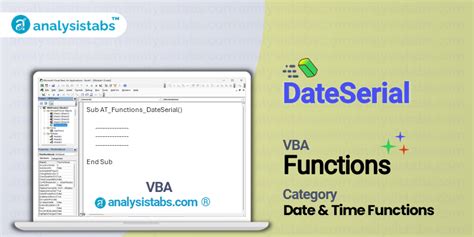

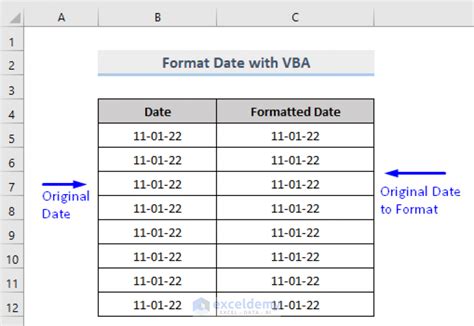

We hope this article has made formatting dates in VBA easy for you. Remember to practice and experiment with different date formats and functions to become proficient in VBA date formatting.
Share your thoughts and experiences with VBA date formatting in the comments section below.