Intro
Unlock the power of global variables in Excel VBA. Learn how to declare, assign, and use public and private variables across multiple modules and workbooks. Mastering global variables streamlines code, enhances collaboration, and optimizes performance. Discover best practices, common pitfalls, and expert tips for efficient VBA development.
Mastering Global Variables in Excel VBA is a crucial skill for any Excel developer. Global variables allow you to store and access data across multiple modules, forms, and procedures in your VBA project. In this article, we will explore the importance of global variables, how to declare and use them, and best practices for managing global variables in your Excel VBA projects.
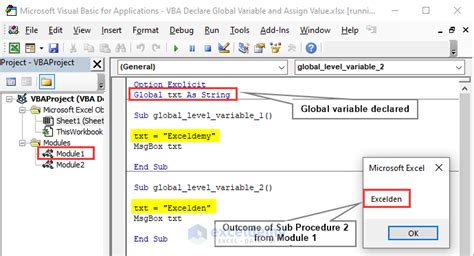
Understanding Global Variables
Global variables are variables that are accessible from any module, form, or procedure in your VBA project. They are declared at the top of a module, outside of any procedure, and are available to all procedures in the project. Global variables are useful when you need to store data that needs to be accessed across multiple procedures or modules.
Declaring Global Variables
To declare a global variable, you use the Public
keyword followed by the variable name and data type. For example:
Public MyGlobalVariable As String
In this example, MyGlobalVariable
is a global variable that can hold a string value. You can declare global variables at the top of any module, but it's common to declare them in a separate module dedicated to global variables.
Using Global Variables
Once you've declared a global variable, you can access it from any procedure or module in your project. For example:
Sub MyProcedure()
MyGlobalVariable = "Hello, World!"
MsgBox MyGlobalVariable
End Sub
In this example, the MyProcedure
subroutine assigns a value to the MyGlobalVariable
global variable and then displays the value in a message box.
Best Practices for Managing Global Variables
While global variables can be useful, they can also make your code harder to understand and debug. Here are some best practices for managing global variables in your Excel VBA projects:
- Use meaningful variable names. Choose variable names that clearly indicate what the variable represents.
- Avoid using global variables for temporary storage. Instead, use local variables within procedures to store temporary data.
- Keep global variables to a minimum. Only use global variables when necessary, and avoid declaring too many global variables.
- Use a consistent naming convention. Use a consistent naming convention for your global variables to make them easy to identify.
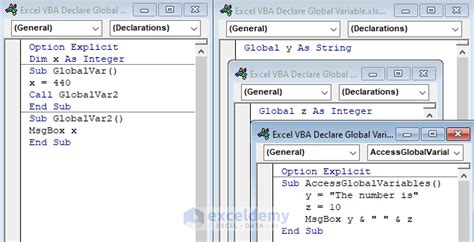
Advantages of Global Variables
Global variables have several advantages that make them useful in Excel VBA programming:
- Accessibility: Global variables are accessible from any module, form, or procedure in your project.
- Convenience: Global variables can be used to store data that needs to be accessed across multiple procedures or modules.
- Flexibility: Global variables can be used to store different types of data, including strings, numbers, and dates.
Disadvantages of Global Variables
While global variables have several advantages, they also have some disadvantages:
- Scope: Global variables have a global scope, which means they can be accessed from anywhere in your project. This can make it harder to understand and debug your code.
- Namespace pollution: Global variables can pollute the namespace, making it harder to find and use variables.
- Security: Global variables can be a security risk, as they can be accessed and modified by any procedure or module in your project.
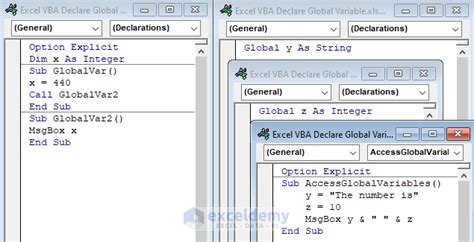
Alternatives to Global Variables
While global variables can be useful, there are alternatives that you can use in certain situations:
- Local variables: Local variables are declared within a procedure and are only accessible within that procedure.
- Module-level variables: Module-level variables are declared at the top of a module and are only accessible within that module.
- Class modules: Class modules are used to create objects that can store data and provide methods for manipulating that data.
Conclusion
Mastering global variables in Excel VBA is a crucial skill for any Excel developer. Global variables allow you to store and access data across multiple modules, forms, and procedures in your VBA project. By following best practices for declaring and using global variables, you can make your code more efficient and easier to understand.
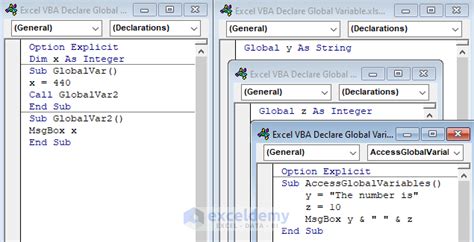
Gallery of Mastering Global Variables In Excel Vba
Mastering Global Variables In Excel Vba Image Gallery
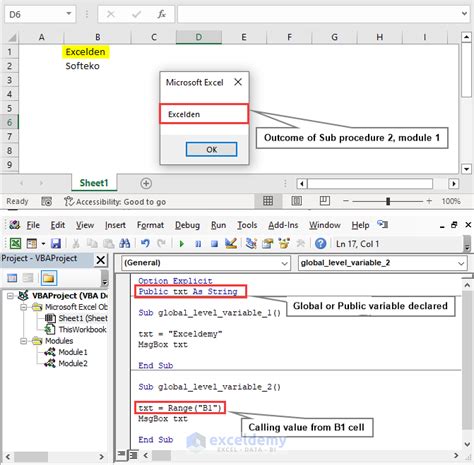
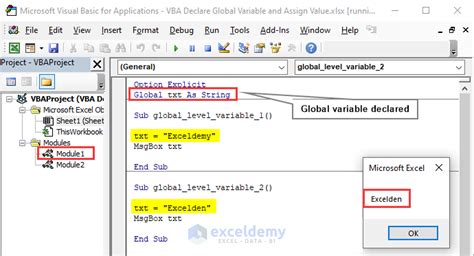
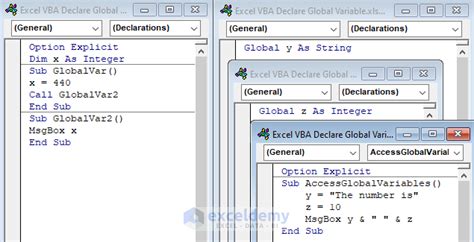
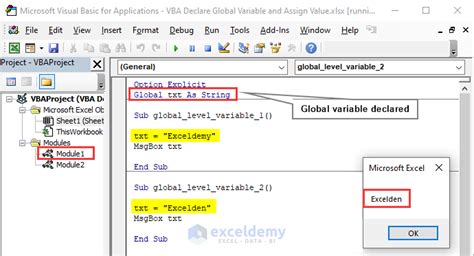
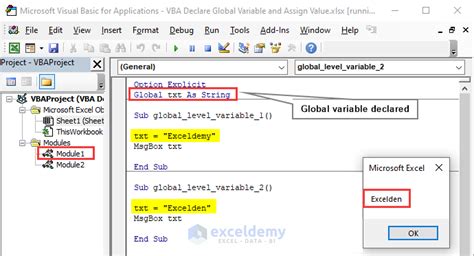
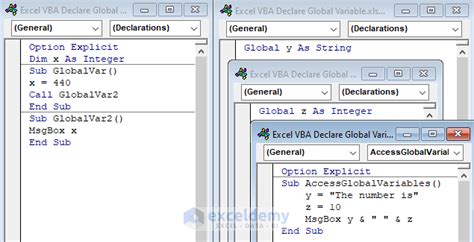
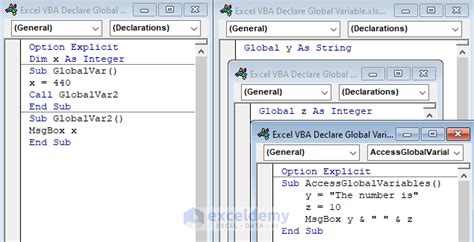
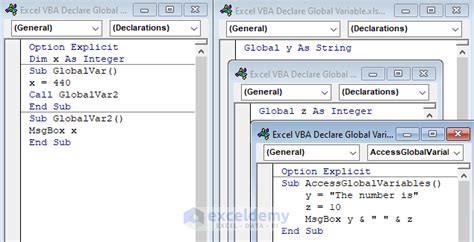
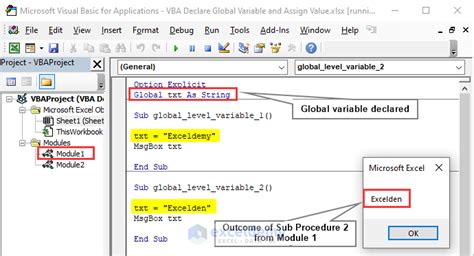
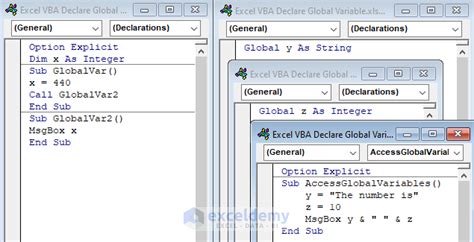