Intro
Master VBA debugging with this step-by-step guide. Learn to identify and fix errors, use debug tools like breakpoints and watches, and optimize your code for performance. Improve your VBA skills and reduce debugging time with expert tips and techniques. Perfect for Excel VBA developers and power users.
As a Visual Basic for Applications (VBA) developer, you're likely no stranger to the frustration of dealing with errors and bugs in your code. Debugging can be a time-consuming and daunting task, especially for complex projects. However, with the right strategies and techniques, you can master the art of debugging and become a more efficient and effective VBA developer.
In this article, we'll take a step-by-step approach to debugging VBA code, covering the basics of debugging, common error types, and advanced debugging techniques. Whether you're a beginner or an experienced VBA developer, this guide will help you improve your debugging skills and take your coding to the next level.
Understanding VBA Errors
Before we dive into debugging, it's essential to understand the types of errors you may encounter in VBA. There are three primary types of errors:
- Syntax errors: These occur when there's a mistake in the code's syntax, such as a missing or mismatched bracket, a typo, or incorrect use of a keyword.
- Runtime errors: These occur when the code is executed, and an error occurs due to incorrect logic, invalid data, or an unexpected event.
- Logic errors: These occur when the code is executed, but the results are incorrect due to flawed logic or incorrect assumptions.
Basic Debugging Techniques
Now that we've covered the types of errors, let's explore some basic debugging techniques:
- Use the Visual Basic Editor: The Visual Basic Editor (VBE) is an essential tool for debugging VBA code. To access the VBE, press Alt + F11 or navigate to Developer > Visual Basic in the ribbon.
- Set breakpoints: Breakpoints allow you to pause the code at a specific point, enabling you to inspect variables, expressions, and the call stack. To set a breakpoint, click in the margin next to the line of code or press F9.
- Use the Debugging toolbar: The Debugging toolbar provides quick access to common debugging commands, such as stepping through code, inspecting variables, and resetting the code.
- Use the Immediate window: The Immediate window allows you to execute code, inspect variables, and debug expressions in real-time.
Advanced Debugging Techniques
Now that we've covered the basics, let's explore some advanced debugging techniques:
- Use the Watch window: The Watch window allows you to monitor variables and expressions in real-time, enabling you to track changes and identify issues.
- Use the Call Stack: The Call Stack displays the sequence of procedure calls, enabling you to identify the source of errors and understand the code's flow.
- Use the Locals window: The Locals window displays the values of local variables, enabling you to inspect and debug variables in real-time.
- Use the Object Browser: The Object Browser provides a hierarchical view of objects, enabling you to explore and debug complex object models.
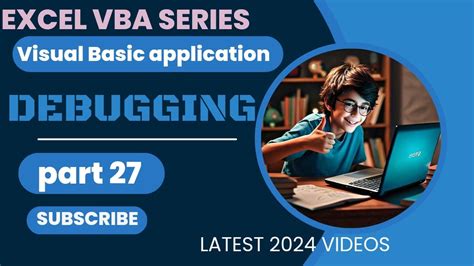
Common VBA Errors and How to Fix Them
Here are some common VBA errors and their solutions:
- Error 424: Object required: This error occurs when VBA can't find the object you're trying to reference. Solution: Check the object's name, and ensure it's properly declared and initialized.
- Error 91: Object variable or With block variable not set: This error occurs when VBA can't find the object variable or With block variable. Solution: Check the object variable or With block variable, and ensure it's properly declared and initialized.
- Error 1004: Method 'Range' of object '_Worksheet' failed: This error occurs when VBA can't find the Range object. Solution: Check the Range object's name, and ensure it's properly declared and initialized.
Best Practices for Debugging VBA Code
Here are some best practices for debugging VBA code:
- Use meaningful variable names: Use descriptive variable names to improve code readability and reduce errors.
- Use comments: Use comments to explain complex code, provide context, and improve code readability.
- Test incrementally: Test code incrementally to identify and fix errors early on.
- Use debugging tools: Use VBA's built-in debugging tools, such as the Watch window, Call Stack, and Locals window, to inspect and debug code.
Conclusion
Mastering VBA debugging requires practice, patience, and persistence. By understanding the types of errors, using basic and advanced debugging techniques, and following best practices, you can improve your debugging skills and become a more efficient and effective VBA developer.
We hope this guide has provided you with the knowledge and skills to tackle even the most complex VBA debugging challenges. Happy coding!
VBA Debugging Image Gallery
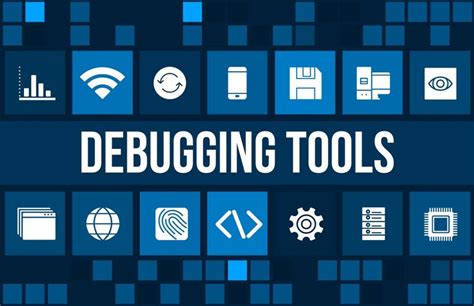
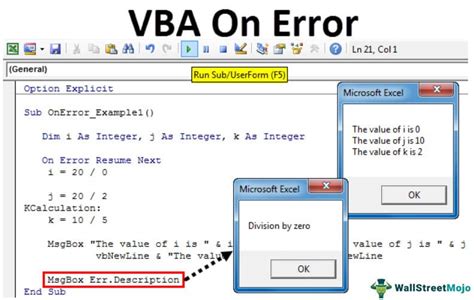
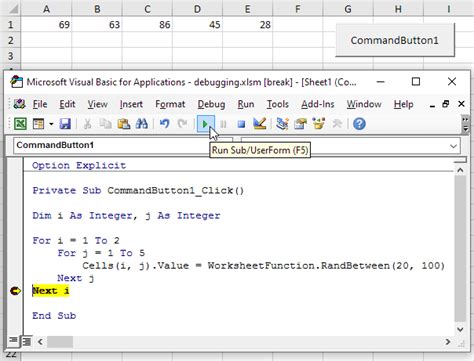
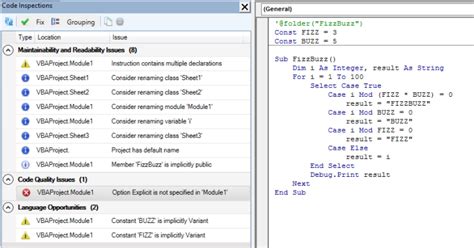
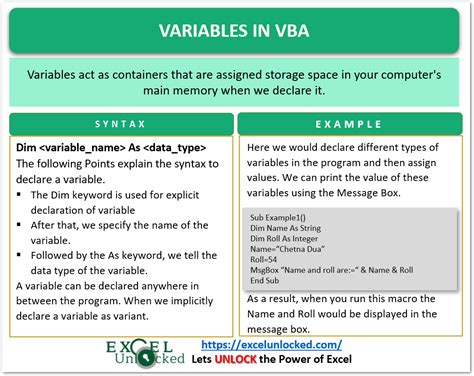
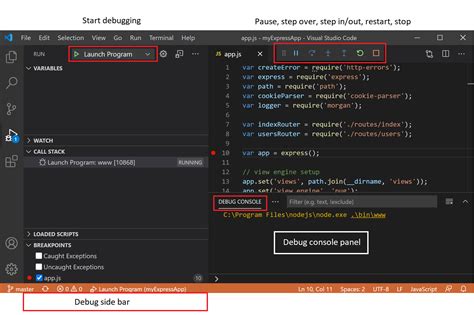
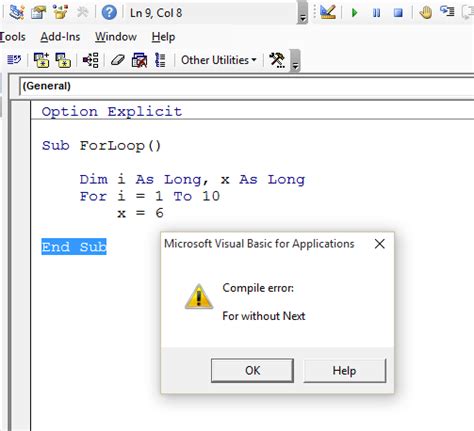
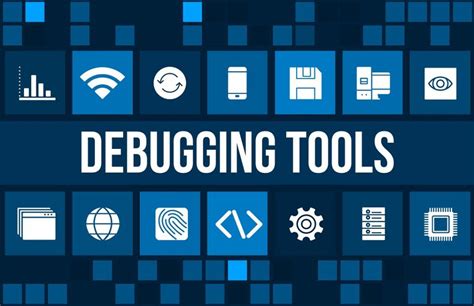
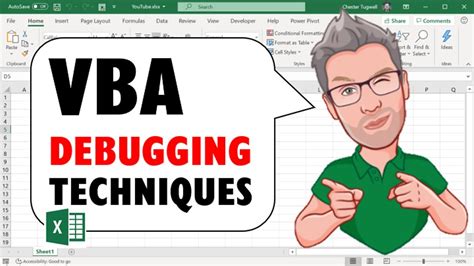
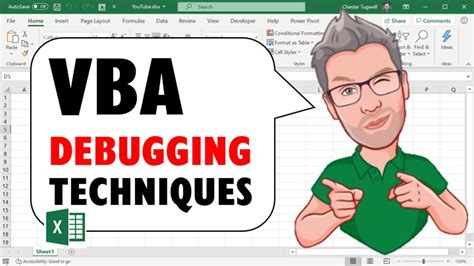
FAQ
Q: What are the most common VBA errors? A: The most common VBA errors include syntax errors, runtime errors, and logic errors.
Q: How do I debug VBA code? A: To debug VBA code, use the Visual Basic Editor, set breakpoints, use the Debugging toolbar, and use the Immediate window.
Q: What are some advanced VBA debugging techniques? A: Advanced VBA debugging techniques include using the Watch window, Call Stack, and Locals window.
Q: How do I fix common VBA errors? A: To fix common VBA errors, check the object's name, ensure it's properly declared and initialized, and use debugging tools to inspect and debug code.
Q: What are some best practices for debugging VBA code? A: Best practices for debugging VBA code include using meaningful variable names, using comments, testing incrementally, and using debugging tools.