Intro
Checking if a value falls within a specified range is a common requirement in various programming tasks. Whether you're validating user input, filtering data, or performing calculations, knowing how to check if a value is between two numbers is essential. In this article, we'll explore five different ways to achieve this in various programming languages.
Method 1: Simple Comparison
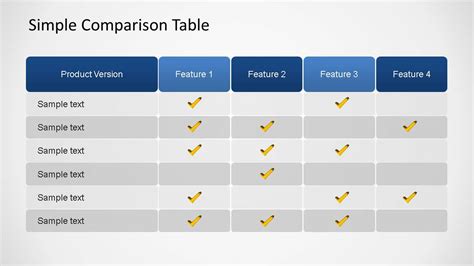
One of the most straightforward methods to check if a value is between two numbers is by using simple comparison operators. This approach involves checking if the value is greater than or equal to the lower bound and less than or equal to the upper bound.
Here's an example in Python:
def is_between(x, lower, upper):
return lower <= x <= upper
# Example usage:
print(is_between(5, 1, 10)) # Output: True
print(is_between(15, 1, 10)) # Output: False
This method is easy to implement and works well for most use cases. However, it may not be the most efficient approach when dealing with large datasets or complex calculations.
Method 2: Using the `in` Operator with a Range Object
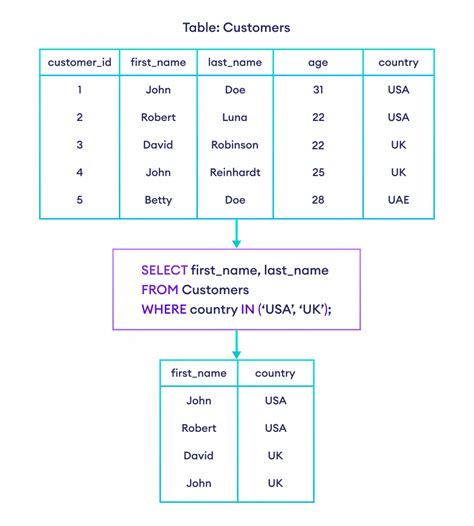
In some programming languages, such as Python and JavaScript, you can use the in
operator to check if a value is within a range object.
Here's an example in Python:
def is_between(x, lower, upper):
return x in range(lower, upper + 1)
# Example usage:
print(is_between(5, 1, 10)) # Output: True
print(is_between(15, 1, 10)) # Output: False
This method is concise and readable, but it may not be suitable for all use cases, especially when dealing with floating-point numbers or non-integer ranges.
Method 3: Using a Ternary Operator
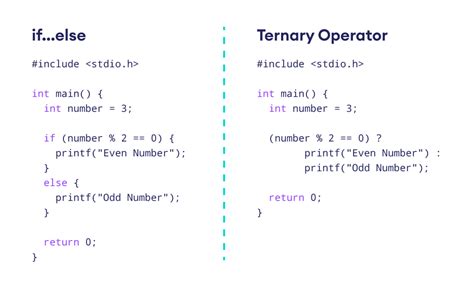
The ternary operator is a concise way to check if a value is between two numbers. This approach uses a single line of code to evaluate the condition and return a boolean value.
Here's an example in Java:
public boolean isBetween(int x, int lower, int upper) {
return (x >= lower && x <= upper)? true : false;
}
// Example usage:
System.out.println(isBetween(5, 1, 10)); // Output: true
System.out.println(isBetween(15, 1, 10)); // Output: false
This method is compact and efficient, but it may not be as readable as other approaches, especially for complex conditions.
Method 4: Using a Conditional Statement
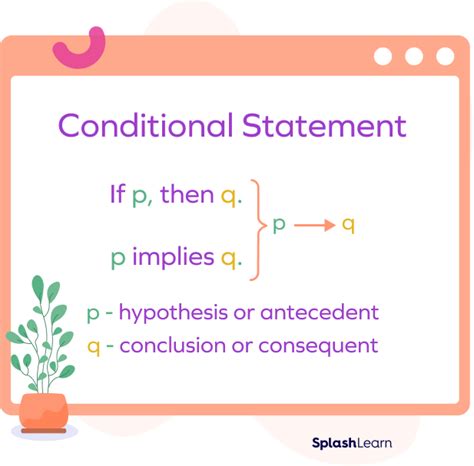
Conditional statements, such as if-else
statements, can be used to check if a value is between two numbers. This approach involves evaluating the condition and executing a block of code based on the result.
Here's an example in C++:
bool isBetween(int x, int lower, int upper) {
if (x >= lower && x <= upper) {
return true;
} else {
return false;
}
}
// Example usage:
std::cout << std::boolalpha << isBetween(5, 1, 10) << std::endl; // Output: true
std::cout << std::boolalpha << isBetween(15, 1, 10) << std::endl; // Output: false
This method is easy to understand and implement, but it may not be as concise as other approaches.
Method 5: Using a Mathematical Function
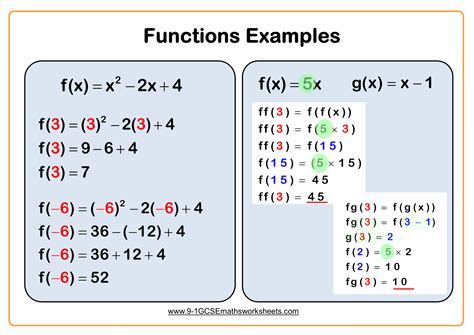
Mathematical functions, such as the clamp
function, can be used to check if a value is between two numbers. This approach involves applying a mathematical operation to the value and checking if the result falls within the desired range.
Here's an example in JavaScript:
function clamp(x, lower, upper) {
return Math.min(Math.max(x, lower), upper);
}
function isBetween(x, lower, upper) {
return clamp(x, lower, upper) === x;
}
// Example usage:
console.log(isBetween(5, 1, 10)); // Output: true
console.log(isBetween(15, 1, 10)); // Output: false
This method is efficient and concise, but it may not be as readable as other approaches, especially for non-mathematical backgrounds.
Gallery of Check if Value is Between Two Numbers
Check if Value is Between Two Numbers Image Gallery
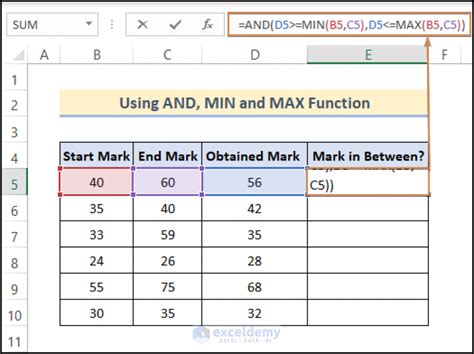
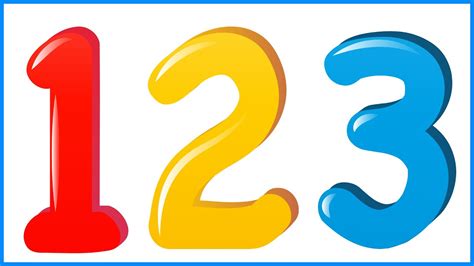
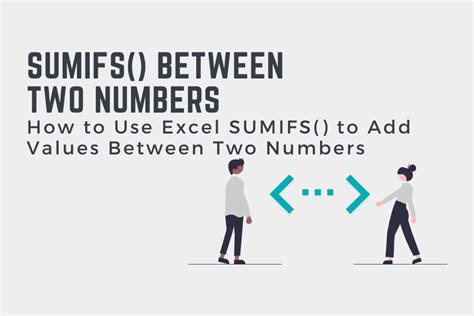
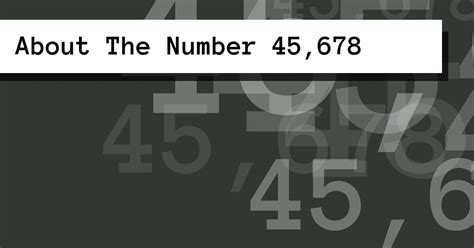
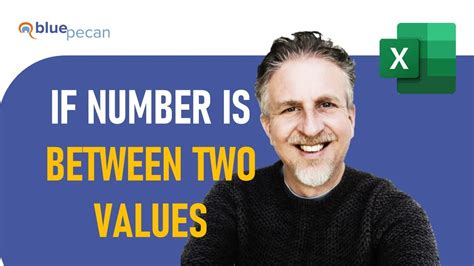
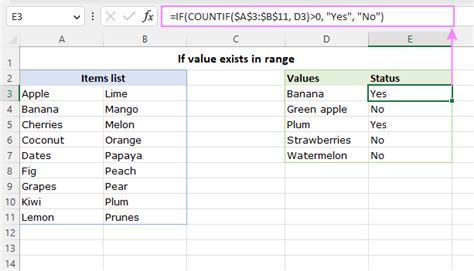
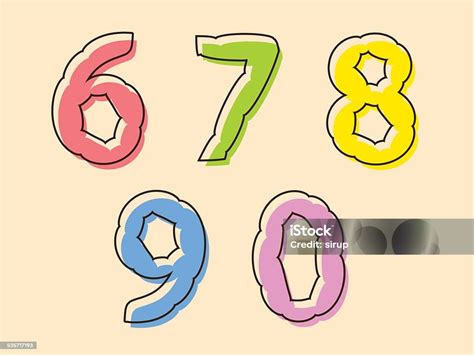
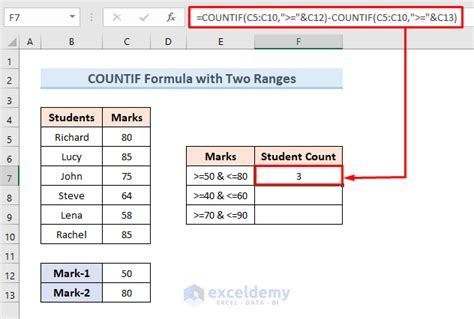
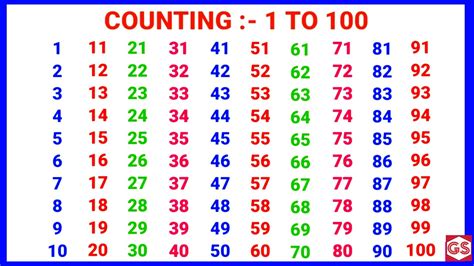
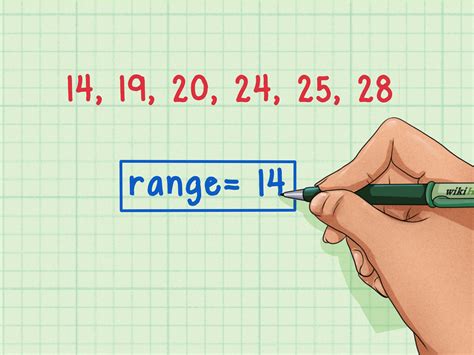
In conclusion, checking if a value is between two numbers is a common task in programming, and there are various methods to achieve this. The choice of method depends on the specific use case, programming language, and personal preference. We hope this article has provided you with a comprehensive understanding of the different approaches and has helped you choose the best method for your needs.