Intro
Excel users often find themselves in situations where they need to perform lookups and return data from other cells. Two of the most powerful tools in VBA for achieving this are the INDEX and MATCH functions. While many users are familiar with VLOOKUP, INDEX and MATCH offer more flexibility and power. In this article, we will explore five ways to use INDEX and MATCH in VBA to take your Excel skills to the next level.
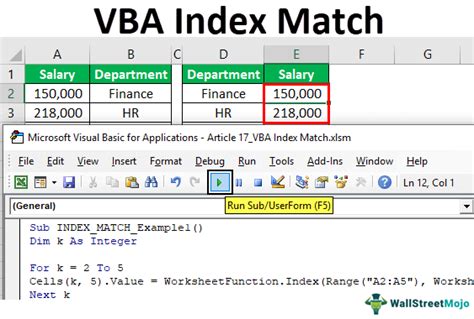
What are INDEX and MATCH?
Before diving into the examples, let's briefly explain what INDEX and MATCH do:
- INDEX returns a value at a specified position in a range or array.
- MATCH returns the relative position of a value within a range or array.
When used together, INDEX and MATCH allow you to perform lookups and return data from other cells, similar to VLOOKUP. However, INDEX and MATCH offer more flexibility, as you can use them to return values from multiple columns or rows.
1. Basic Lookup with INDEX and MATCH
One of the most common uses of INDEX and MATCH is to perform a basic lookup. For example, suppose you have a table with employee names in column A and their corresponding salaries in column B. You can use INDEX and MATCH to return the salary for a specific employee.
Sub BasicLookup()
Dim rng As Range
Set rng = Range("A2:B10") ' assume data is in range A2:B10
Dim employee As String
employee = "John Doe"
Dim salary As Variant
salary = Application.Index(rng.Columns(2), Application.Match(employee, rng.Columns(1), 0))
MsgBox "Salary for " & employee & ": " & salary
End Sub
In this example, we use MATCH to find the relative position of the employee name in column A, and then use INDEX to return the corresponding salary from column B.
2. Multiple Column Lookup
Another advantage of using INDEX and MATCH is that you can perform lookups across multiple columns. For example, suppose you have a table with employee names in column A, departments in column B, and job titles in column C. You can use INDEX and MATCH to return the job title for a specific employee in a specific department.
Sub MultipleColumnLookup()
Dim rng As Range
Set rng = Range("A2:C10") ' assume data is in range A2:C10
Dim employee As String
employee = "John Doe"
Dim department As String
department = "Sales"
Dim jobTitle As Variant
jobTitle = Application.Index(rng.Columns(3), Application.Match(1, (Application.Match(employee, rng.Columns(1), 0) = rng.Columns(1)) * (Application.Match(department, rng.Columns(2), 0) = rng.Columns(2)), 0))
MsgBox "Job title for " & employee & " in " & department & ": " & jobTitle
End Sub
In this example, we use MATCH to find the relative position of the employee name in column A and the department in column B, and then use INDEX to return the corresponding job title from column C.
3. Returning Multiple Values
INDEX and MATCH can also be used to return multiple values. For example, suppose you have a table with employee names in column A and their corresponding salaries in column B. You can use INDEX and MATCH to return the salaries for all employees in a specific department.
Sub ReturnMultipleValues()
Dim rng As Range
Set rng = Range("A2:B10") ' assume data is in range A2:B10
Dim department As String
department = "Sales"
Dim salaries As Variant
salaries = Application.Index(rng.Columns(2), Application.Match(department, rng.Columns(1), 0))
MsgBox "Salaries for employees in " & department & ": " & Join(salaries, ", ")
End Sub
In this example, we use MATCH to find the relative position of the department in column A, and then use INDEX to return the corresponding salaries from column B. The Join function is used to concatenate the salaries into a single string.
4. Using INDEX and MATCH with Arrays
INDEX and MATCH can also be used with arrays. For example, suppose you have an array of employee names and corresponding salaries. You can use INDEX and MATCH to return the salary for a specific employee.
Sub UsingArrays()
Dim employees() As Variant
employees = Array("John Doe", "Jane Doe", "Bob Smith")
Dim salaries() As Variant
salaries = Array(50000, 60000, 70000)
Dim employee As String
employee = "John Doe"
Dim salary As Variant
salary = Application.Index(salaries, Application.Match(employee, employees, 0))
MsgBox "Salary for " & employee & ": " & salary
End Sub
In this example, we use MATCH to find the relative position of the employee name in the employees array, and then use INDEX to return the corresponding salary from the salaries array.
5. Using INDEX and MATCH with Dynamic Ranges
Finally, INDEX and MATCH can be used with dynamic ranges. For example, suppose you have a table with employee names in column A and their corresponding salaries in column B. You can use INDEX and MATCH to return the salary for a specific employee, even if the range of data changes.
Sub DynamicRange()
Dim rng As Range
Set rng = Range("A2:B" & Cells(Rows.Count, "A").End(xlUp).Row) ' assume data is in range A2:Bdynamic
Dim employee As String
employee = "John Doe"
Dim salary As Variant
salary = Application.Index(rng.Columns(2), Application.Match(employee, rng.Columns(1), 0))
MsgBox "Salary for " & employee & ": " & salary
End Sub
In this example, we use the Cells and End functions to dynamically determine the range of data, and then use INDEX and MATCH to return the salary for a specific employee.
INDEX and MATCH Image Gallery
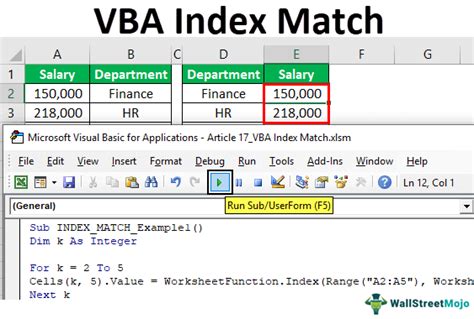
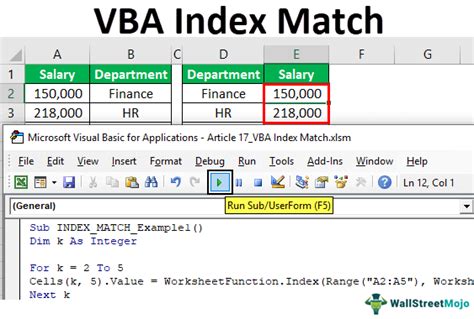
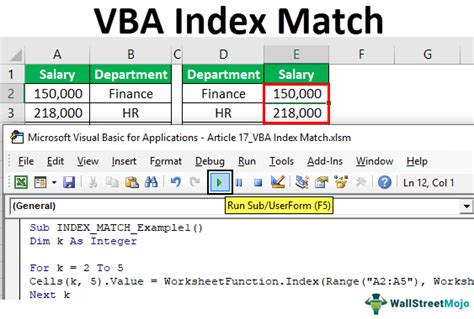
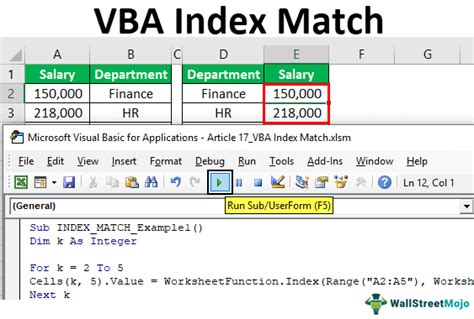
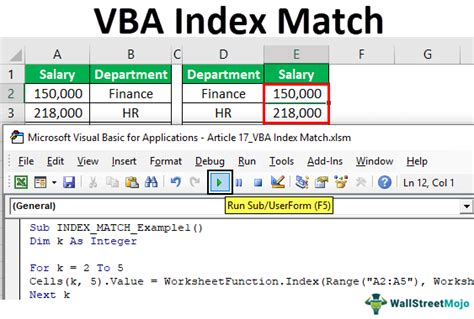
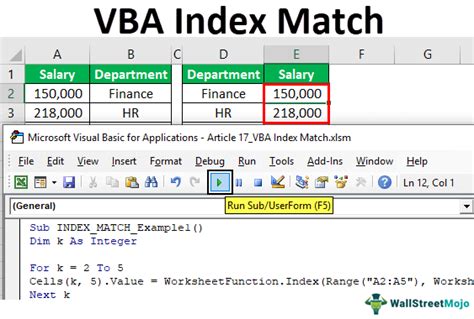
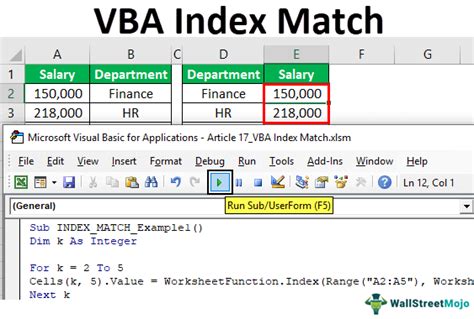
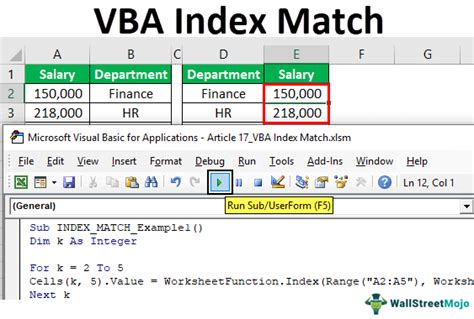
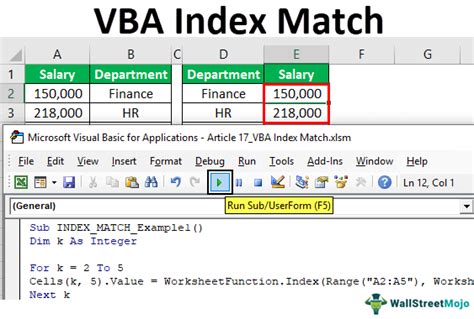
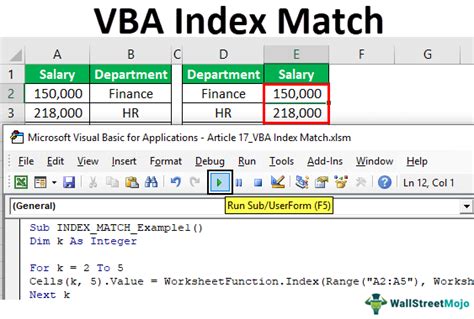
Conclusion
In this article, we explored five ways to use INDEX and MATCH in VBA to perform lookups and return data from other cells. We demonstrated how to use INDEX and MATCH to perform basic lookups, multiple column lookups, return multiple values, use arrays, and dynamic ranges. By mastering INDEX and MATCH, you can take your Excel skills to the next level and perform complex data analysis tasks with ease.
We hope this article has been informative and helpful. If you have any questions or need further clarification on any of the examples, please don't hesitate to ask. Happy coding!