Intro
Rescue your VBA code from errors! Learn how to fix invalid forward reference errors, a common issue in Visual Basic for Applications. Discover the causes, symptoms, and step-by-step solutions to resolve this frustrating problem. Master VBA error handling, debugging, and referencing to optimize your codes performance and reliability.
Understanding Invalid Forward Reference Errors in VBA Code
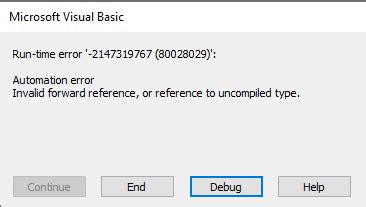
When working with VBA (Visual Basic for Applications) code, you may encounter an error message stating "Invalid Forward Reference" or "Variable not defined". This error occurs when VBA encounters a variable or procedure that has not been declared or defined before it is used in the code.
In VBA, the compiler reads the code from top to bottom, and any variables or procedures must be declared before they are used. If a variable or procedure is used before it is declared, VBA will throw an "Invalid Forward Reference" error.
This article will explain the causes of Invalid Forward Reference errors, provide examples, and offer solutions to fix these errors in your VBA code.
Causes of Invalid Forward Reference Errors
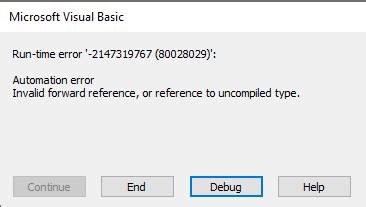
There are several reasons why you may encounter an Invalid Forward Reference error in your VBA code:
- Variables not declared: If a variable is used before it is declared, VBA will throw an error.
- Procedures not defined: If a procedure (such as a subroutine or function) is called before it is defined, VBA will throw an error.
- Late binding: If an object is created using late binding (e.g.,
CreateObject
), VBA may not be able to resolve the object's properties and methods, leading to an error.
Example of an Invalid Forward Reference Error
Sub MySub()
MsgBox myVariable
Dim myVariable As String
End Sub
In this example, the variable myVariable
is used before it is declared, resulting in an Invalid Forward Reference error.
Solutions to Fix Invalid Forward Reference Errors
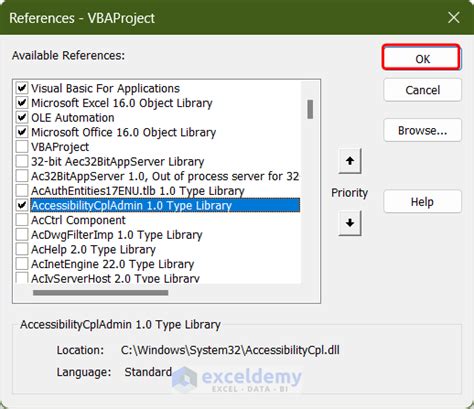
To fix an Invalid Forward Reference error, you can try the following solutions:
- Declare variables before use: Make sure to declare all variables before they are used in your code.
- Define procedures before use: Ensure that all procedures are defined before they are called in your code.
- Use early binding: Instead of using late binding, try using early binding (e.g.,
Dim obj As New MyObject
) to create objects. - Check for typos: Verify that variable and procedure names are spelled correctly and match the declarations.
Example of Fixed Code
Sub MySub()
Dim myVariable As String
myVariable = "Hello World"
MsgBox myVariable
End Sub
In this example, the variable myVariable
is declared before it is used, fixing the Invalid Forward Reference error.
Best Practices to Avoid Invalid Forward Reference Errors
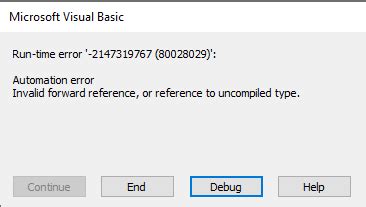
To avoid Invalid Forward Reference errors in your VBA code, follow these best practices:
- Use Option Explicit: This statement forces you to declare all variables, helping you catch errors early.
- Declare variables at the top: Keep all variable declarations at the top of your module or procedure.
- Use meaningful variable names: Use descriptive names for variables to avoid confusion and typos.
- Test your code: Regularly test your code to catch errors and fix them promptly.
By following these best practices and understanding the causes of Invalid Forward Reference errors, you can write more robust and error-free VBA code.
Conclusion
Invalid Forward Reference errors can be frustrating, but by understanding their causes and following best practices, you can avoid them and write more efficient VBA code. Remember to declare variables before use, define procedures before use, and test your code regularly to catch errors early.
Gallery of VBA Error Handling
VBA Error Handling Gallery
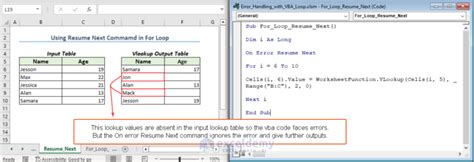
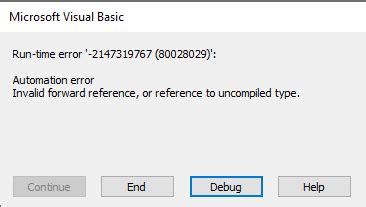
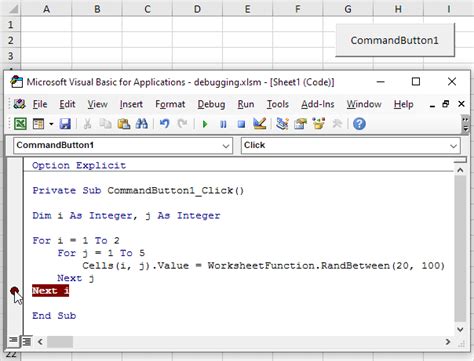
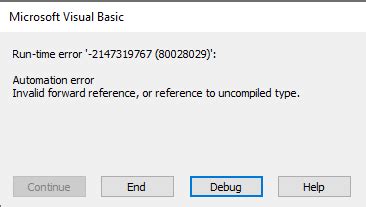
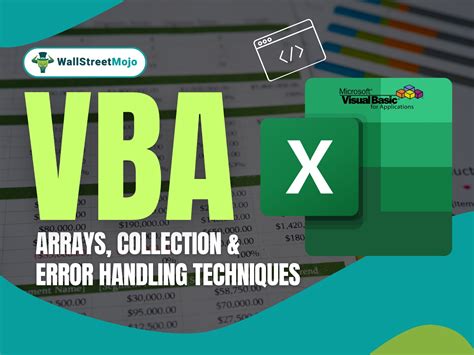
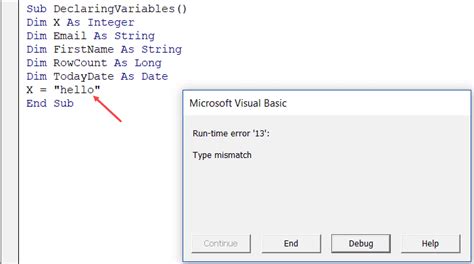
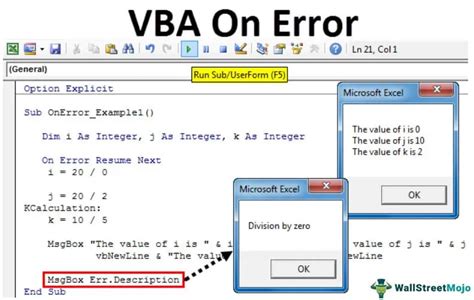
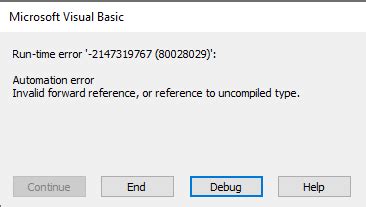
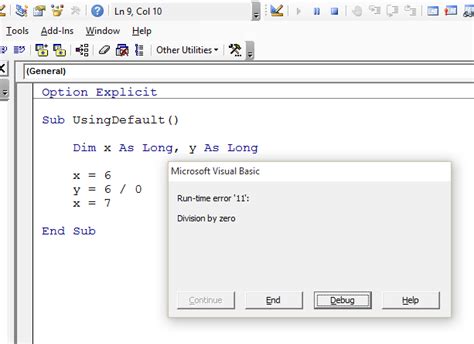
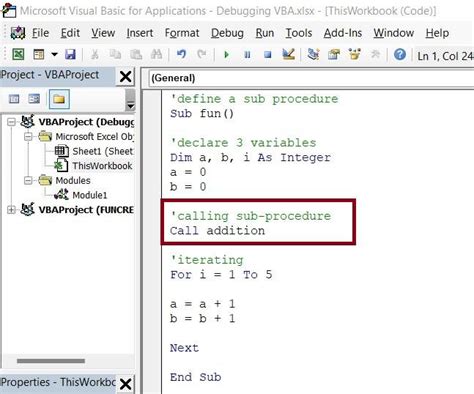
Share your thoughts!
Have you encountered Invalid Forward Reference errors in your VBA code? How did you resolve them? Share your experiences and tips in the comments below!