Intro
Master the art of creating a linked list in C++ with our easy-to-follow template. Learn how to implement a singly linked list, doubly linked list, and circular linked list with our step-by-step guide. Discover how to insert, delete, and traverse nodes with ease. Boost your C++ skills with our linked list template tutorial.
Linked lists are a fundamental data structure in computer science, and understanding how to implement them in C++ is crucial for any aspiring programmer. In this article, we'll delve into the world of linked lists, exploring their benefits, implementation, and applications.
What is a Linked List?
A linked list is a dynamic collection of nodes, each of which contains a value and a reference (i.e., a "link") to the next node in the list. This structure allows for efficient insertion and deletion of nodes at any point in the list, making it a popular choice for applications where data is constantly being added or removed.
Why Use Linked Lists?
Linked lists offer several advantages over other data structures, including:
- Dynamic size: Linked lists can grow or shrink as needed, making them ideal for applications where the amount of data is uncertain.
- Efficient insertion and deletion: Linked lists allow for fast insertion and deletion of nodes at any point in the list, making them suitable for applications where data is constantly being added or removed.
- Good cache performance: Linked lists exhibit good cache performance, as the nodes are stored in contiguous blocks of memory.
Implementing a Linked List in C++
Here's a basic template for a singly linked list in C++:
// linked_list.h
#ifndef LINKED_LIST_H
#define LINKED_LIST_H
class Node {
public:
int data;
Node* next;
Node(int value) : data(value), next(nullptr) {}
};
class LinkedList {
private:
Node* head;
public:
LinkedList() : head(nullptr) {}
void insert(int value);
void remove(int value);
void printList();
};
#endif // LINKED_LIST_H
// linked_list.cpp
#include "linked_list.h"
#include
void LinkedList::insert(int value) {
Node* newNode = new Node(value);
if (head == nullptr) {
head = newNode;
} else {
Node* current = head;
while (current->next!= nullptr) {
current = current->next;
}
current->next = newNode;
}
}
void LinkedList::remove(int value) {
if (head == nullptr) return;
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next!= nullptr) {
if (current->next->data == value) {
Node* temp = current->next;
current->next = current->next->next;
delete temp;
return;
}
current = current->next;
}
}
void LinkedList::printList() {
Node* current = head;
while (current!= nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
This implementation provides basic functionality for inserting and removing nodes from the list, as well as printing the list.
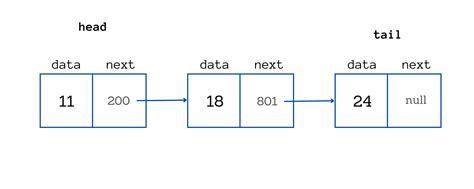
Using the Linked List Template
To use the linked list template, simply create an instance of the LinkedList
class and call the insert
, remove
, and printList
methods as needed:
int main() {
LinkedList list;
list.insert(1);
list.insert(2);
list.insert(3);
list.printList(); // Output: 1 2 3
list.remove(2);
list.printList(); // Output: 1 3
return 0;
}
This example demonstrates how to create a linked list, insert nodes, remove nodes, and print the list.
Applications of Linked Lists
Linked lists have numerous applications in computer science, including:
- Database query results: Linked lists can be used to store the results of a database query, allowing for efficient insertion and deletion of rows.
- Browser history: Linked lists can be used to implement browser history, allowing users to navigate forward and backward through their browsing history.
- Dynamic memory allocation: Linked lists can be used to implement dynamic memory allocation, allowing for efficient allocation and deallocation of memory blocks.
Linked List Image Gallery
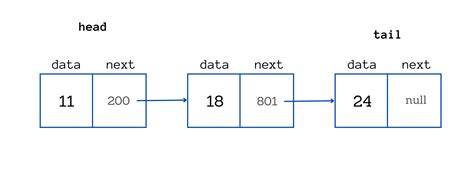
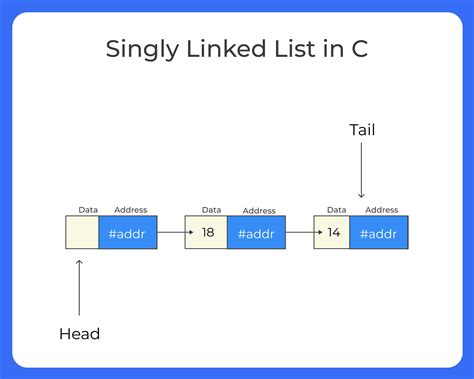

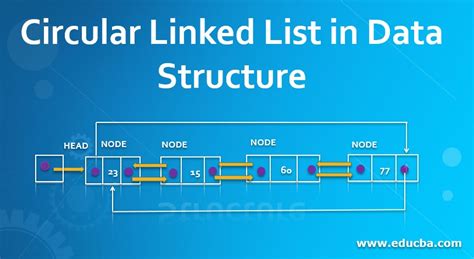
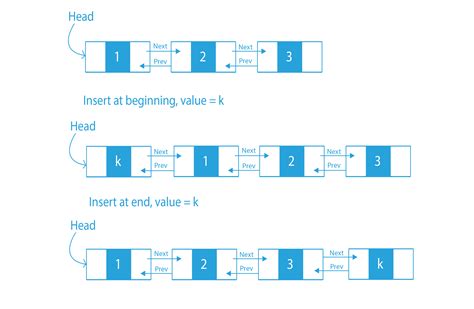
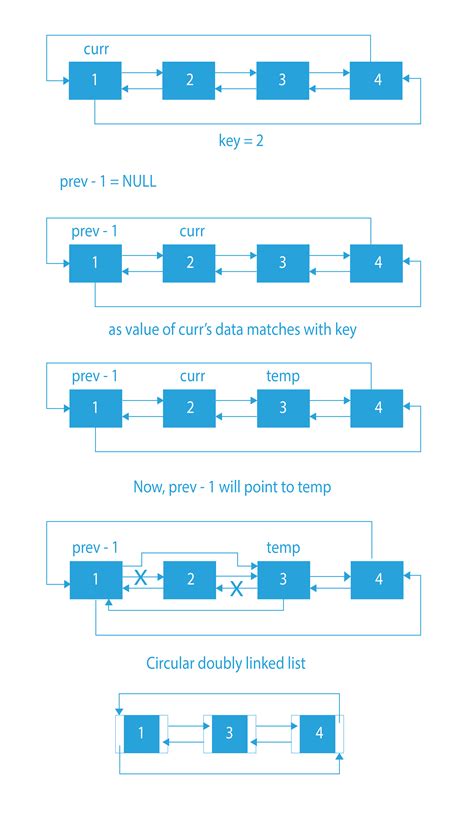
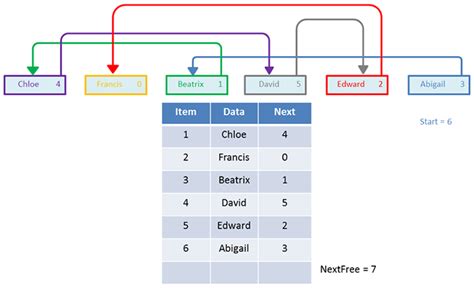
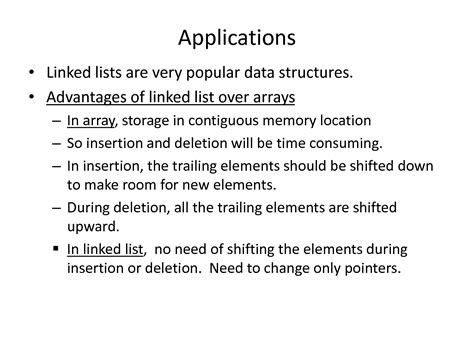
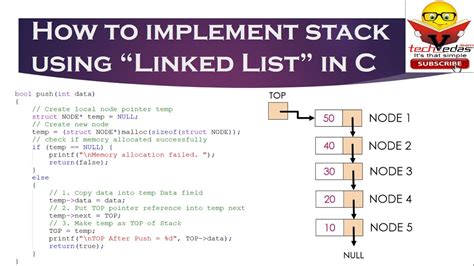
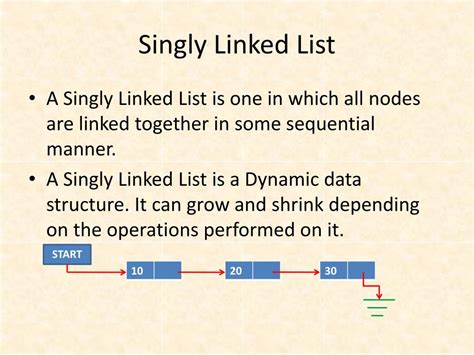
Conclusion
In this article, we've explored the world of linked lists, including their benefits, implementation, and applications. We've also provided a basic template for a singly linked list in C++, which can be used as a starting point for more complex implementations. Whether you're a seasoned programmer or just starting out, linked lists are an essential data structure to understand and master.
FAQ
Q: What is a linked list? A: A linked list is a dynamic collection of nodes, each of which contains a value and a reference to the next node in the list.
Q: What are the advantages of linked lists? A: Linked lists offer several advantages, including dynamic size, efficient insertion and deletion, and good cache performance.
Q: How do I implement a linked list in C++? A: You can implement a linked list in C++ using the template provided in this article.
Q: What are some applications of linked lists? A: Linked lists have numerous applications in computer science, including database query results, browser history, and dynamic memory allocation.
We hope this article has been informative and helpful. Do you have any questions or comments about linked lists? Share them with us in the comments below!