Intro
Working with arrays in VBA can be a powerful tool for managing and manipulating data. One of the most common tasks when working with arrays is looping through them to perform operations on each element. In this article, we'll explore five different ways to loop through an array in VBA, highlighting the benefits and drawbacks of each method.
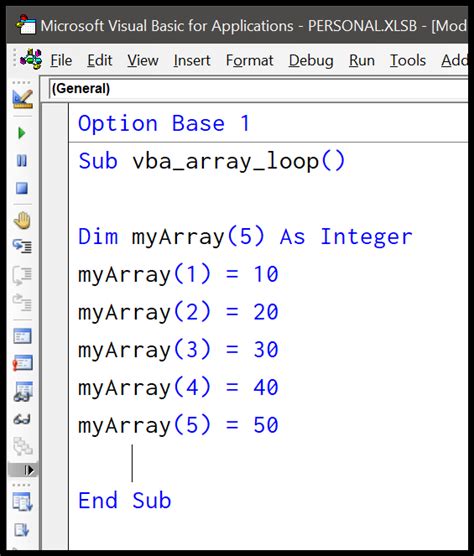
Understanding Arrays in VBA
Before we dive into looping through arrays, it's essential to understand how arrays work in VBA. An array is a collection of values of the same data type stored in a single variable. Arrays can be one-dimensional (single row or column) or multi-dimensional (multiple rows and columns).
Method 1: For Loop
The most common way to loop through an array in VBA is using a For loop. This method is straightforward and easy to understand.
Sub LoopThroughArray()
Dim myArray(1 To 5) As Integer
Dim i As Integer
' Populate the array
For i = 1 To 5
myArray(i) = i
Next i
' Loop through the array
For i = 1 To 5
Debug.Print myArray(i)
Next i
End Sub
In this example, we create an array myArray
with five elements and populate it with values from 1 to 5. We then use a For loop to iterate through the array and print each element to the Immediate window.
Method 2: For Each Loop
Another way to loop through an array is using a For Each loop. This method is more concise and readable than the traditional For loop.
Sub LoopThroughArrayForEach()
Dim myArray(1 To 5) As Integer
Dim element As Variant
' Populate the array
For i = 1 To 5
myArray(i) = i
Next i
' Loop through the array
For Each element In myArray
Debug.Print element
Next element
End Sub
In this example, we use a For Each loop to iterate through the array and print each element to the Immediate window.
Method 3: Do While Loop
A Do While loop can also be used to loop through an array. This method is useful when you need to perform operations on each element until a certain condition is met.
Sub LoopThroughArrayDoWhile()
Dim myArray(1 To 5) As Integer
Dim i As Integer
' Populate the array
For i = 1 To 5
myArray(i) = i
Next i
' Loop through the array
i = 1
Do While i <= 5
Debug.Print myArray(i)
i = i + 1
Loop
End Sub
In this example, we use a Do While loop to iterate through the array and print each element to the Immediate window.
Method 4: LBound and UBound Functions
The LBound and UBound functions can be used to loop through an array by iterating through the indices of the array.
Sub LoopThroughArrayLBoundUBound()
Dim myArray(1 To 5) As Integer
Dim i As Integer
' Populate the array
For i = 1 To 5
myArray(i) = i
Next i
' Loop through the array
For i = LBound(myArray) To UBound(myArray)
Debug.Print myArray(i)
Next i
End Sub
In this example, we use the LBound and UBound functions to get the lower and upper bounds of the array, and then use a For loop to iterate through the indices of the array.
Method 5: Arrays as Collections
In VBA, arrays can be treated as collections, which allows us to loop through them using the For Each loop.
Sub LoopThroughArrayAsCollection()
Dim myArray As Variant
Dim element As Variant
' Populate the array
myArray = Array(1, 2, 3, 4, 5)
' Loop through the array
For Each element In myArray
Debug.Print element
Next element
End Sub
In this example, we create an array myArray
and populate it with values using the Array function. We then use a For Each loop to iterate through the array and print each element to the Immediate window.
Gallery of Array Loop Examples
Gallery of Array Loop Examples
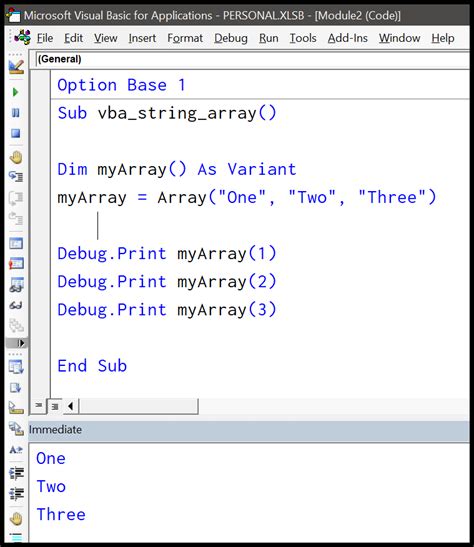
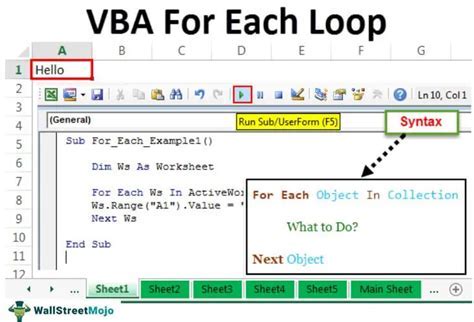
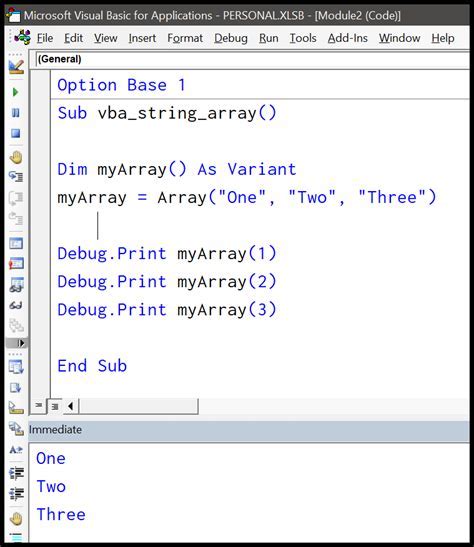
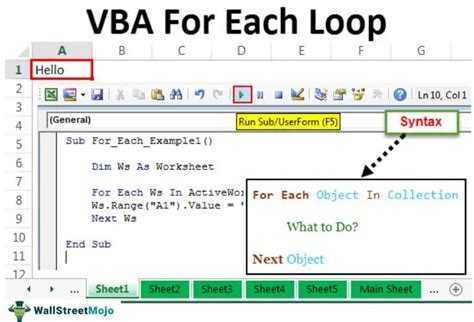
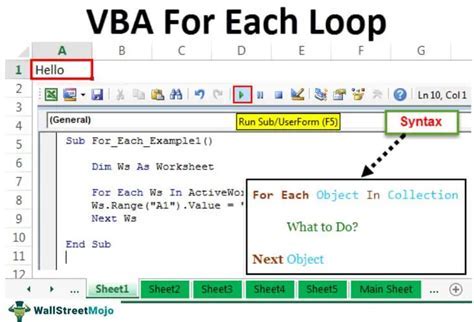
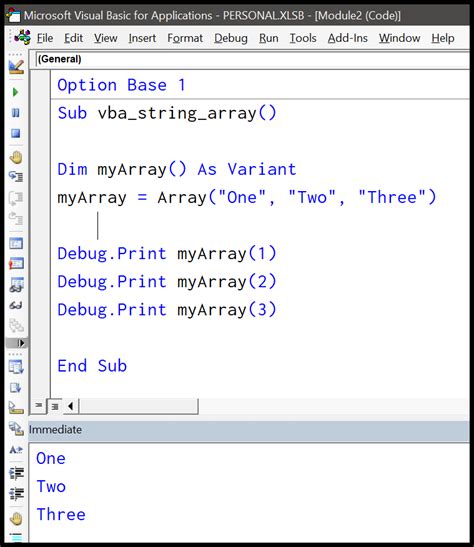
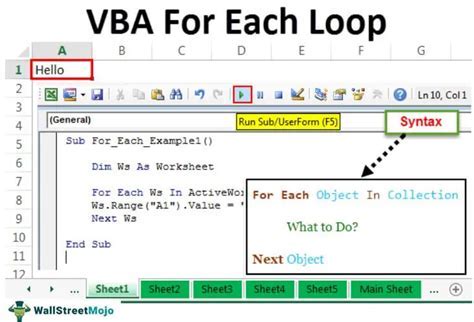
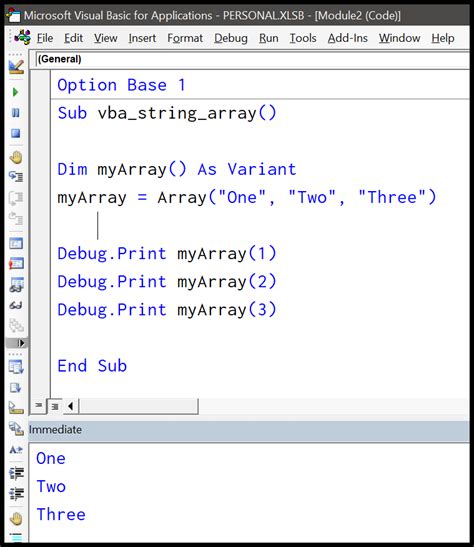
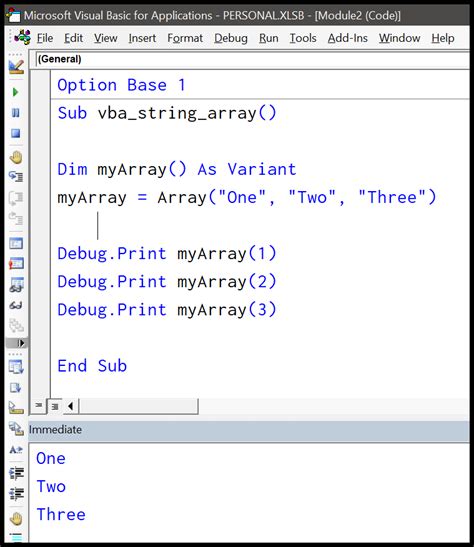
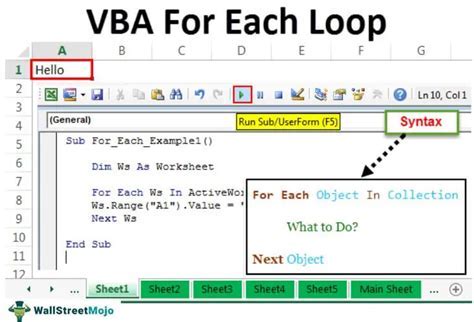
In conclusion, looping through arrays in VBA can be achieved using various methods, each with its own strengths and weaknesses. The choice of method depends on the specific requirements of your project and your personal preference. By understanding the different methods available, you can write more efficient and effective code to manipulate arrays in VBA.
We hope this article has been informative and helpful in your VBA development journey. If you have any questions or need further assistance, please don't hesitate to ask. Share your thoughts and experiences with us in the comments section below.