Intro
Troubleshoot Method Range of Object Worksheet Failed errors with our 5 expert fixes. Learn how to resolve VBA compile errors, worksheet object issues, and range errors in Excel. Master error handling and debugging techniques to fix common mistakes and improve your coding skills.
The "Method Range of Object Worksheet Failed" error is a common issue that can arise when working with Excel VBA, particularly when trying to automate tasks or manipulate data in a worksheet. This error can be frustrating, especially for those new to VBA programming. In this article, we will explore the causes of this error and provide five fixes to help you overcome it.
Understanding the Error
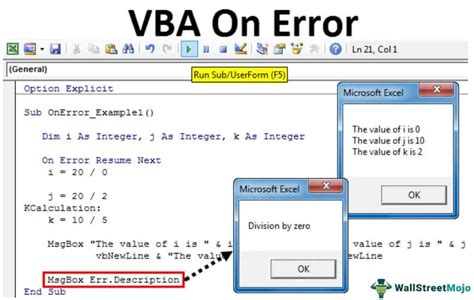
The "Method Range of Object Worksheet Failed" error typically occurs when VBA is unable to identify the worksheet or range you are trying to reference. This could be due to a variety of reasons, including incorrect syntax, misspelled sheet names, or attempting to access a worksheet that does not exist.
Cause 1: Incorrect Syntax
One of the most common causes of this error is incorrect syntax. VBA is very particular about the way you reference worksheets and ranges. Make sure that you are using the correct syntax for referencing worksheets and ranges.Fix 1: Verify Worksheet and Range References
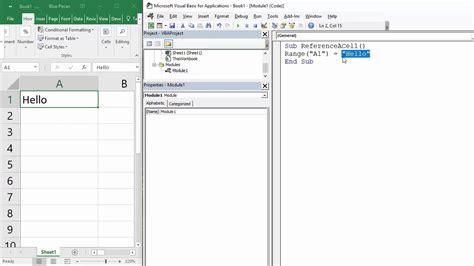
Before you start debugging your code, make sure that you have verified the worksheet and range references. Check that the worksheet name is spelled correctly and that the range reference is accurate. You can use the following code to verify the worksheet and range references:
Sub VerifyWorksheetAndRange()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("YourWorksheetName")
Dim rng As Range
Set rng = ws.Range("A1:B2")
Debug.Print rng.Address
End Sub
Replace "YourWorksheetName" with the actual name of your worksheet and "A1:B2" with the actual range you are trying to reference.
Cause 2: Misspelled Sheet Names
Another common cause of this error is misspelled sheet names. Make sure that the sheet name is spelled correctly and that there are no leading or trailing spaces.Fix 2: Check for Misspelled Sheet Names
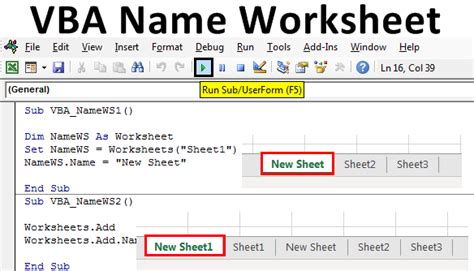
To check for misspelled sheet names, go to the Project Explorer in the Visual Basic Editor and expand the "Microsoft Excel Objects" folder. Check that the sheet name is spelled correctly and that there are no leading or trailing spaces. You can also use the following code to check for misspelled sheet names:
Sub CheckForMisspelledSheetNames()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.Name = "YourWorksheetName" Then
Debug.Print "Sheet name found: " & ws.Name
Else
Debug.Print "Sheet name not found: " & ws.Name
End If
Next ws
End Sub
Replace "YourWorksheetName" with the actual name of your worksheet.
Cause 3: Attempting to Access a Worksheet That Does Not Exist
Another cause of this error is attempting to access a worksheet that does not exist. Make sure that the worksheet you are trying to access exists in the workbook.Fix 3: Verify Worksheet Existence
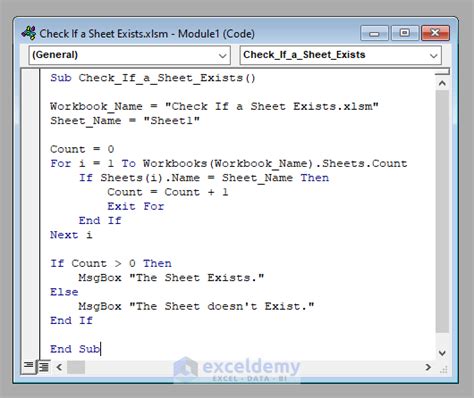
To verify worksheet existence, you can use the following code:
Sub VerifyWorksheetExistence()
Dim ws As Worksheet
On Error Resume Next
Set ws = ThisWorkbook.Worksheets("YourWorksheetName")
If ws Is Nothing Then
Debug.Print "Worksheet not found: " & "YourWorksheetName"
Else
Debug.Print "Worksheet found: " & ws.Name
End If
On Error GoTo 0
End Sub
Replace "YourWorksheetName" with the actual name of your worksheet.
Cause 4: Worksheet Not Active
Another cause of this error is that the worksheet is not active. Make sure that the worksheet you are trying to access is active.Fix 4: Activate Worksheet
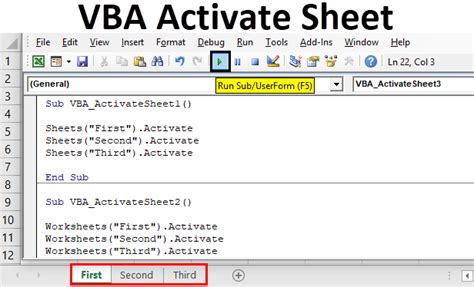
To activate a worksheet, you can use the following code:
Sub ActivateWorksheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("YourWorksheetName")
ws.Activate
End Sub
Replace "YourWorksheetName" with the actual name of your worksheet.
Cause 5: Worksheet Protected
Another cause of this error is that the worksheet is protected. Make sure that the worksheet you are trying to access is not protected.Fix 5: Unprotect Worksheet
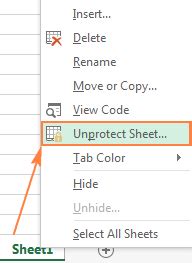
To unprotect a worksheet, you can use the following code:
Sub UnprotectWorksheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("YourWorksheetName")
ws.Unprotect
End Sub
Replace "YourWorksheetName" with the actual name of your worksheet.
Gallery of Excel VBA Error Fixes:
Excel VBA Error Fixes Image Gallery
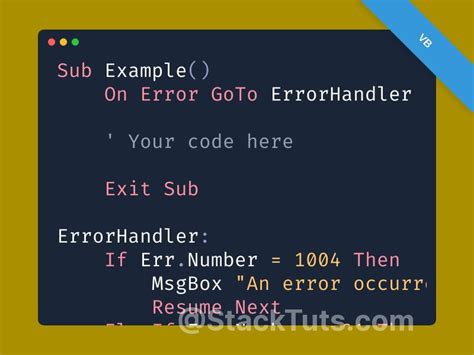
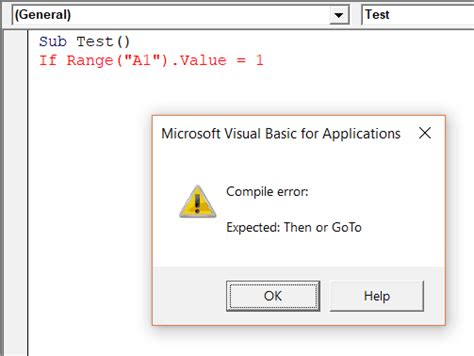
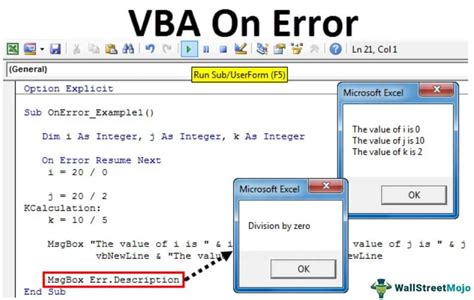
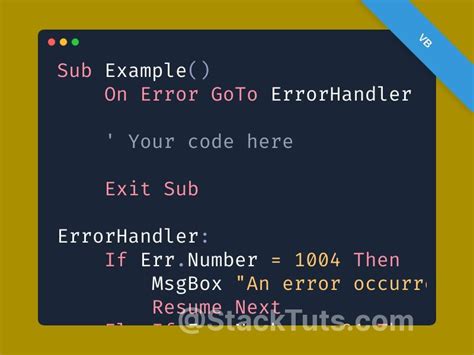
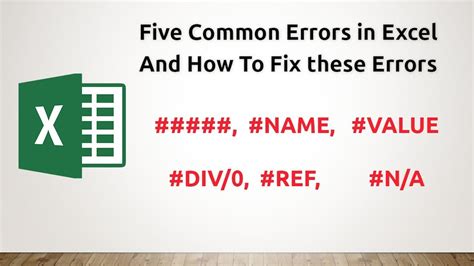
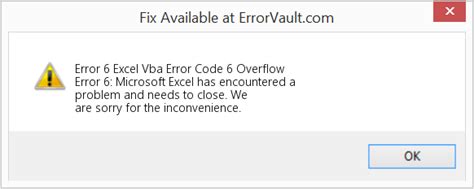
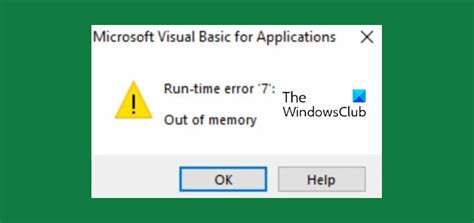
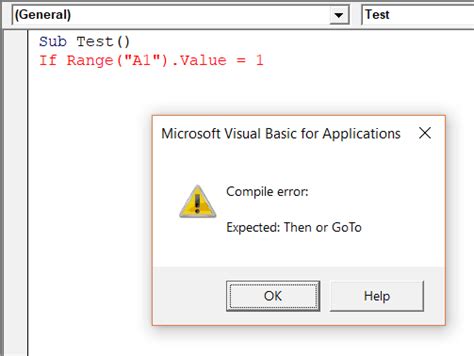
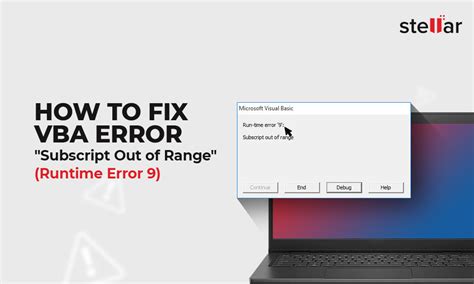
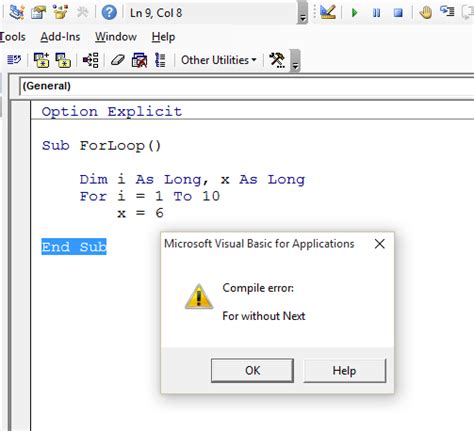
We hope this article has helped you to identify and fix the "Method Range of Object Worksheet Failed" error in Excel VBA. Remember to verify worksheet and range references, check for misspelled sheet names, verify worksheet existence, activate worksheets, and unprotect worksheets. If you have any further questions or need additional assistance, please don't hesitate to ask.