Mastering VBA is an essential skill for anyone working with Microsoft Excel, and one of the most versatile functions in VBA is the Mid function. The Mid function allows you to extract a specific part of a string, making it a powerful tool for data manipulation and analysis. In this article, we will explore five essential ways to use the Mid function in VBA, along with practical examples and tips to help you master this function.
What is the Mid Function?
The Mid function is a built-in VBA function that returns a specified number of characters from a string, starting from a specified position. The syntax for the Mid function is:
Mid(string, start, length)
Where:
string
is the original string from which you want to extract characters.start
is the position in the string where you want to start extracting characters.length
is the number of characters you want to extract.
Essential Way 1: Extracting a Substring
One of the most common uses of the Mid function is to extract a substring from a larger string. For example, suppose you have a string that contains a person's full name, and you want to extract just the first name.
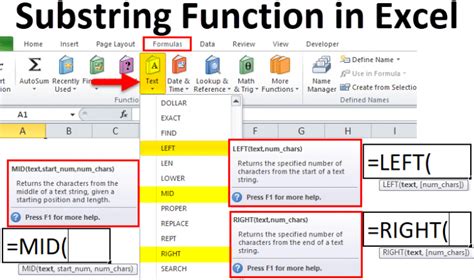
In this example, the full name is "John Smith", and we want to extract the first name "John". We can use the Mid function like this:
firstName = Mid(fullName, 1, 4)
This code extracts the first 4 characters from the full name, starting from position 1 (the first character).
Essential Way 2: Parsing a Date String
Another common use of the Mid function is to parse a date string into its individual components (day, month, year). For example, suppose you have a date string in the format "dd/mm/yyyy", and you want to extract the day, month, and year separately.
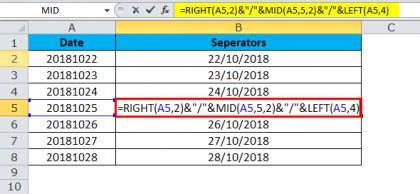
In this example, the date string is "12/03/2023", and we want to extract the day, month, and year. We can use the Mid function like this:
day = Mid(dateString, 1, 2)
month = Mid(dateString, 4, 2)
year = Mid(dateString, 7, 4)
This code extracts the day, month, and year from the date string, using the Mid function to extract the relevant characters.
Essential Way 3: Removing Unwanted Characters
The Mid function can also be used to remove unwanted characters from a string. For example, suppose you have a string that contains a phone number with dashes or parentheses, and you want to remove these characters.
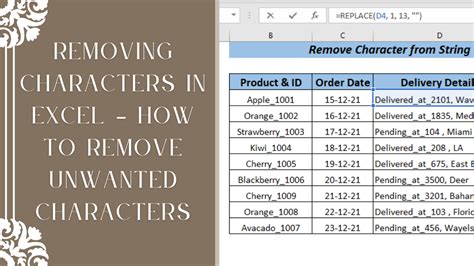
In this example, the phone number is "(123) 456-7890", and we want to remove the parentheses and dashes. We can use the Mid function like this:
phoneNumber = Mid(phoneNumber, 2, Len(phoneNumber) - 2)
This code removes the first and last characters from the phone number string (the parentheses), and then uses the Len function to get the length of the resulting string.
Essential Way 4: Extracting a File Extension
The Mid function can also be used to extract a file extension from a file path. For example, suppose you have a file path that includes the file name and extension, and you want to extract just the extension.
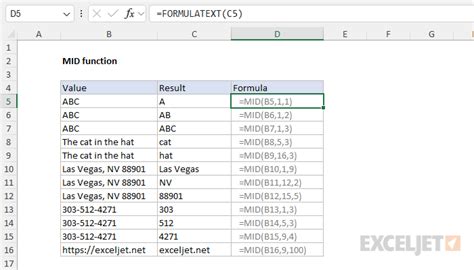
In this example, the file path is "C:\Users\Documents\example.txt", and we want to extract the file extension ".txt". We can use the Mid function like this:
fileExtension = Mid(filePath, InStrRev(filePath, ".") + 1)
This code uses the InStrRev function to find the last occurrence of the dot (.) in the file path, and then uses the Mid function to extract the file extension starting from that position.
Essential Way 5: Creating a Random String
Finally, the Mid function can be used to create a random string by extracting random characters from a larger string. For example, suppose you want to generate a random password that consists of a combination of letters and numbers.
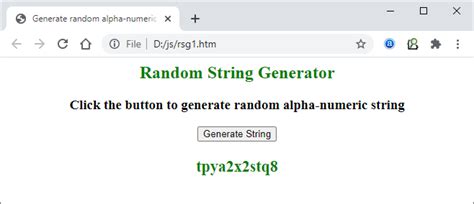
In this example, we can use the Mid function like this:
randomString = Mid("abcdefghijklmnopqrstuvwxyz0123456789", Int((Len("abcdefghijklmnopqrstuvwxyz0123456789") * Rnd)), 1)
This code extracts a random character from the string of letters and numbers, using the Rnd function to generate a random number between 1 and the length of the string.
Gallery of Mid Function Examples
Mid Function Image Gallery
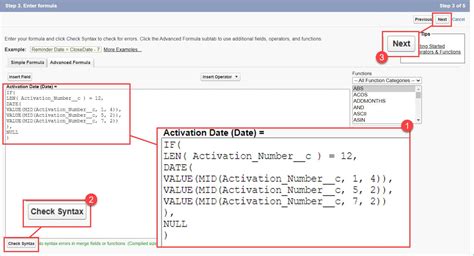
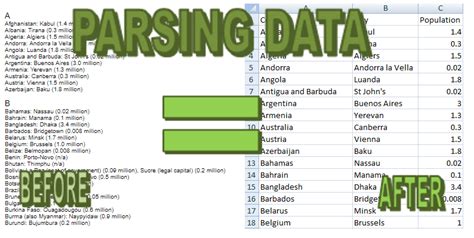
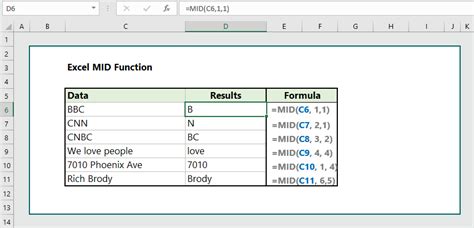
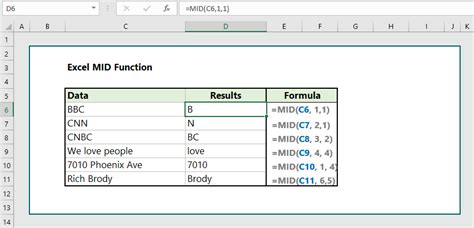
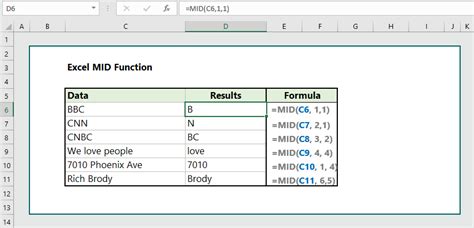
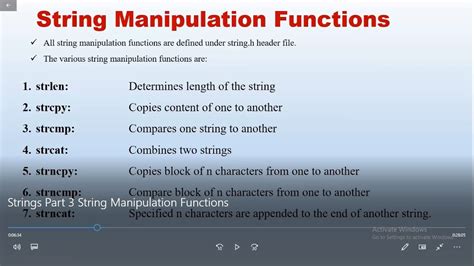
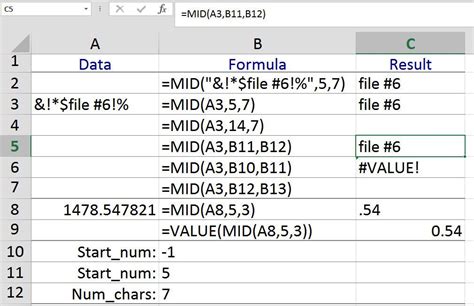
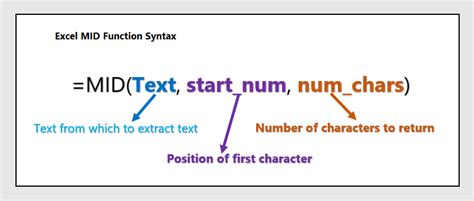
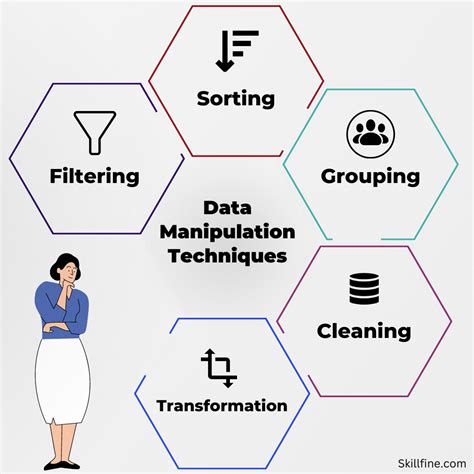
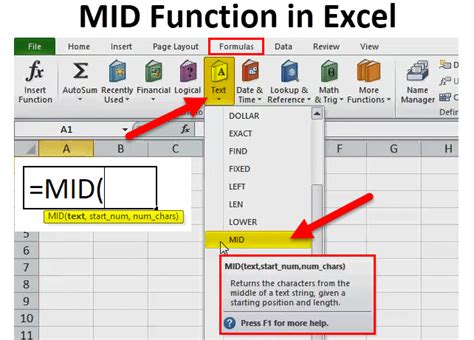
We hope this article has provided you with a comprehensive understanding of the Mid function in VBA, along with practical examples and tips to help you master this function. Whether you're a beginner or an advanced user, the Mid function is an essential tool to have in your VBA toolkit. We encourage you to try out these examples and experiment with different uses of the Mid function to improve your VBA skills.
Don't forget to share your thoughts and experiences with the Mid function in the comments section below. How do you use the Mid function in your VBA projects? Do you have any tips or tricks to share with our readers?