Intro
Mastering nested if statements in VBA is a crucial skill for any Excel user looking to take their spreadsheet game to the next level. Nested if statements allow you to create complex decision-making processes that can save you time and effort in the long run. However, they can also be confusing and difficult to manage if not used correctly. In this article, we will explore five ways to master nested if statements in VBA and take your Excel skills to new heights.
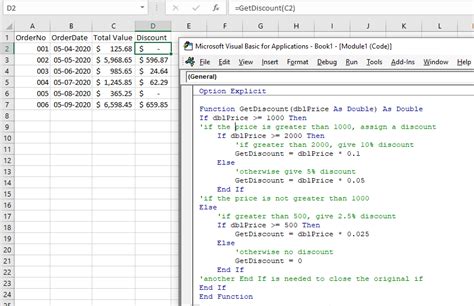
Understanding the Basics of Nested If Statements
Before we dive into the five ways to master nested if statements, let's quickly review the basics. A nested if statement is a type of if statement that is contained within another if statement. This allows you to create multiple layers of decision-making, making your code more efficient and effective.
How to Write a Nested If Statement
Writing a nested if statement is relatively straightforward. Here is an example:
If Condition1 Then
If Condition2 Then
'Code to execute if Condition1 and Condition2 are true
Else
'Code to execute if Condition1 is true but Condition2 is false
End If
Else
'Code to execute if Condition1 is false
End If
Way #1: Keep it Simple
The first way to master nested if statements is to keep it simple. This means avoiding unnecessary complexity and focusing on the specific problem you are trying to solve. Here are a few tips for keeping your nested if statements simple:
- Use clear and concise language when writing your conditions.
- Avoid using too many layers of nesting.
- Use indentation to make your code more readable.
By following these tips, you can ensure that your nested if statements are easy to understand and maintain.

Way #2: Use Select Case Statements
The second way to master nested if statements is to use select case statements. Select case statements are a type of decision-making statement that allows you to evaluate a single expression against multiple values. Here is an example:
Select Case Expression
Case Value1
'Code to execute if Expression = Value1
Case Value2
'Code to execute if Expression = Value2
Case Else
'Code to execute if Expression does not match any of the values
End Select
Select case statements can often be used in place of nested if statements, making your code more efficient and easier to read.
Way #3: Use VBA's Built-in Functions
The third way to master nested if statements is to use VBA's built-in functions. VBA has a number of built-in functions that can help simplify your code and reduce the need for nested if statements. Here are a few examples:
- The
IIF
function, which allows you to evaluate a condition and return one of two values. - The
Switch
function, which allows you to evaluate a list of expressions and return a value. - The
Choose
function, which allows you to select a value from a list based on a position.
By using these built-in functions, you can simplify your code and make it more efficient.
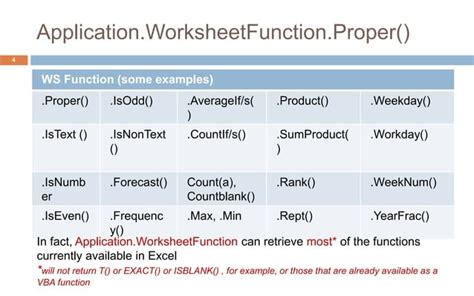
Way #4: Use Boolean Variables
The fourth way to master nested if statements is to use Boolean variables. Boolean variables are variables that can have one of two values: True
or False
. By using Boolean variables, you can simplify your code and reduce the need for nested if statements. Here is an example:
Dim IsCondition1 As Boolean
IsCondition1 = (Condition1)
If IsCondition1 Then
'Code to execute if Condition1 is true
Else
'Code to execute if Condition1 is false
End If
By using Boolean variables, you can make your code more readable and easier to maintain.
Way #5: Practice, Practice, Practice
The fifth and final way to master nested if statements is to practice, practice, practice. The more you practice using nested if statements, the more comfortable you will become with them. Here are a few tips for practicing:
- Start with simple examples and gradually move on to more complex ones.
- Use online resources, such as tutorials and coding challenges, to practice your skills.
- Work on real-world projects that require the use of nested if statements.
By practicing regularly, you can improve your skills and become a master of nested if statements.
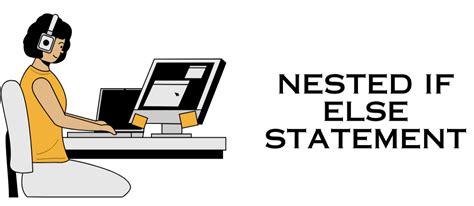
Conclusion
Mastering nested if statements in VBA is a crucial skill for any Excel user looking to take their spreadsheet game to the next level. By following the five ways outlined in this article, you can improve your skills and become a master of nested if statements. Remember to keep it simple, use select case statements, use VBA's built-in functions, use Boolean variables, and practice, practice, practice.
Nested If Statements Image Gallery
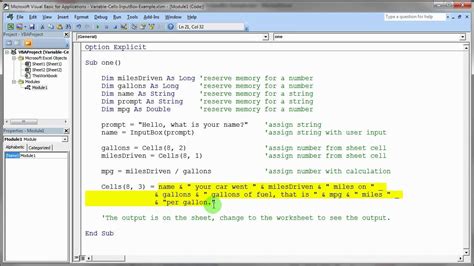
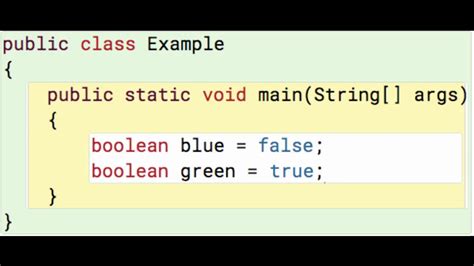
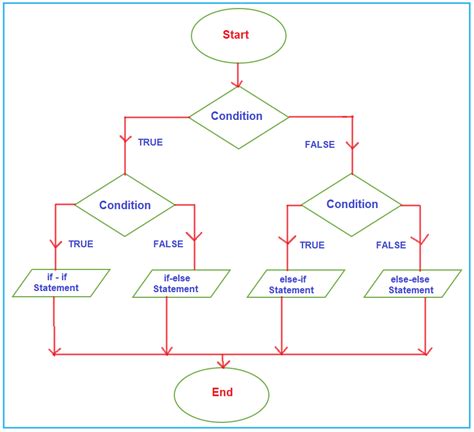
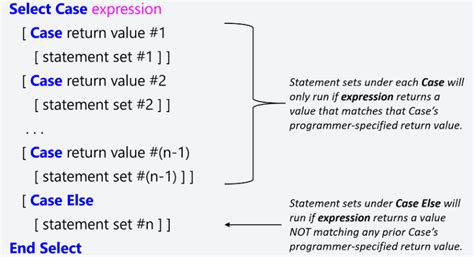

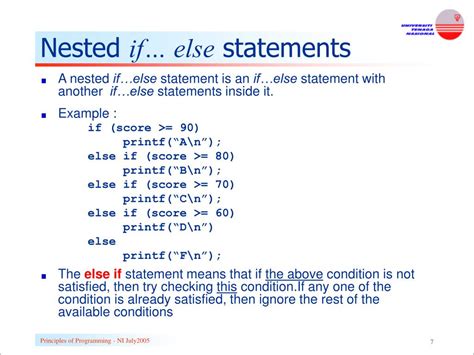
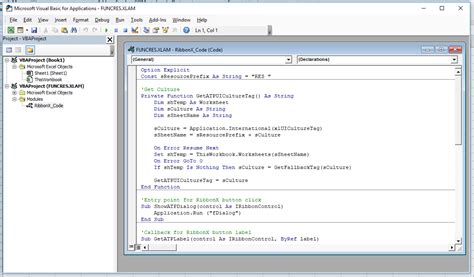
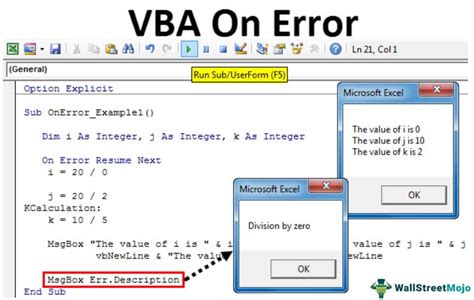
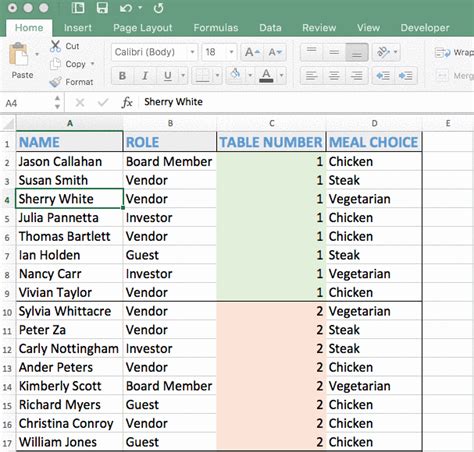
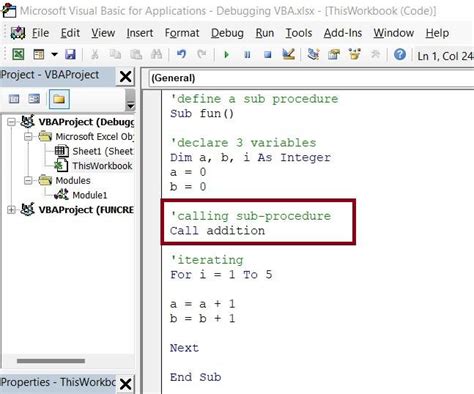
What's your experience with nested if statements in VBA? Do you have any tips or tricks to share? Let us know in the comments below!