Next Js Typescript Material Ui Starter Template Summary
Boost your development speed with our Next.js TypeScript Material UI starter template. Kickstart your project with a pre-configured setup, combining the power of Next.js, TypeScript, and Material UI. Learn how to integrate these technologies seamlessly and jumpstart your React application development. Get started with our comprehensive guide and template.
Building a modern web application requires a robust set of tools and technologies. Next.js, TypeScript, and Material-UI are some of the most popular choices among developers. In this article, we will explore how to create a starter template using these technologies.
Why Next.js?
Next.js is a popular React-based framework for building server-rendered, statically generated, and performance-optimized applications. It provides a set of features out of the box, including:
- Server-side rendering (SSR)
- Static site generation (SSG)
- Code splitting
- Internationalization (i18n)
- API routes
Why TypeScript?
TypeScript is a statically typed language that helps developers catch errors early and improve code maintainability. It provides features like:
- Static type checking
- Interoperability with JavaScript
- Optional static typing
Why Material-UI?
Material-UI is a popular React UI framework that provides a set of pre-designed components and a consistent design language. It offers features like:
- Pre-designed components
- Customizable theme
- Accessible components
Setting up the Starter Template
To create a starter template using Next.js, TypeScript, and Material-UI, follow these steps:
- Create a new Next.js project using the following command:
npx create-next-app my-app --ts
This will create a new Next.js project with TypeScript support.
- Install Material-UI using the following command:
npm install @material-ui/core
- Create a new file called
theme.ts
in the root directory and add the following code:
import { createMuiTheme } from '@material-ui/core/styles';
const theme = createMuiTheme({
palette: {
primary: {
main: '#333',
},
secondary: {
main: '#666',
},
},
});
export default theme;
This code creates a basic theme for Material-UI.
- Create a new file called
layout.tsx
in thecomponents
directory and add the following code:
import Header from './header';
import Footer from './footer';
import { ThemeProvider } from '@material-ui/core/styles';
import theme from '../theme';
interface LayoutProps {
children: React.ReactNode;
}
const Layout: React.FC = ({ children }) => {
return (
{children}
);
};
export default Layout;
This code creates a basic layout component that wraps the application with a theme provider.
- Create a new file called
header.tsx
in thecomponents
directory and add the following code:
import { AppBar, Toolbar, Typography } from '@material-ui/core';
const Header: React.FC = () => {
return (
My App
);
};
export default Header;
This code creates a basic header component using Material-UI.
- Create a new file called
footer.tsx
in thecomponents
directory and add the following code:
import { Footer as MUIFooter } from '@material-ui/core';
const Footer: React.FC = () => {
return (
Copyright 2023 My App
);
};
export default Footer;
This code creates a basic footer component using Material-UI.
- Update the
pages/_app.tsx
file to use the layout component:
import type { AppProps } from 'next/app';
import Layout from '../components/layout';
function MyApp({ Component, pageProps }: AppProps) {
return (
);
}
export default MyApp;
This code wraps the application with the layout component.
Gallery Section
Here is an example of a gallery section using Material-UI:
Gallery
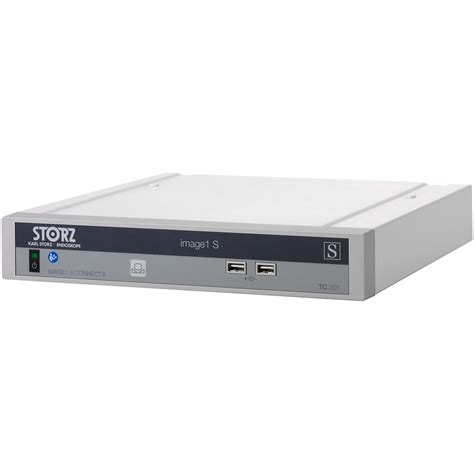
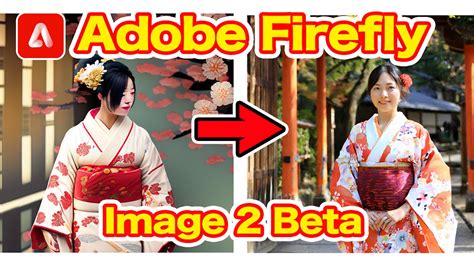
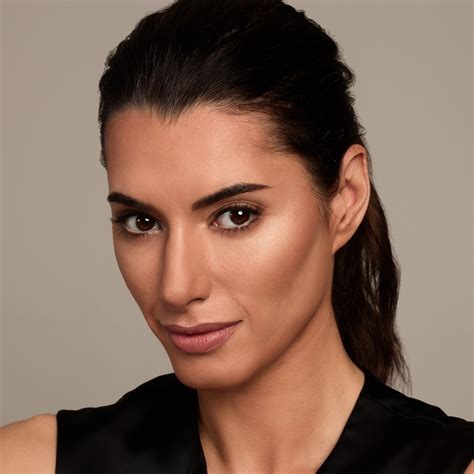
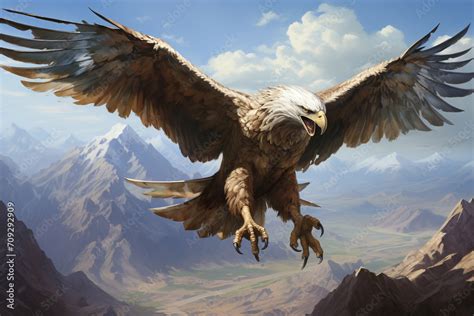
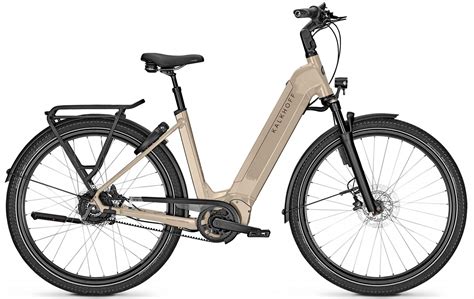
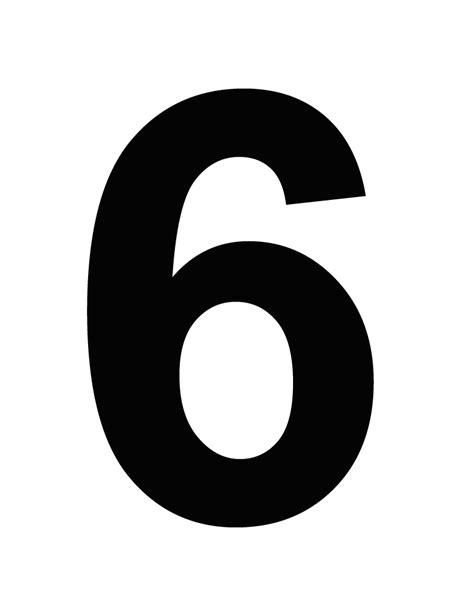
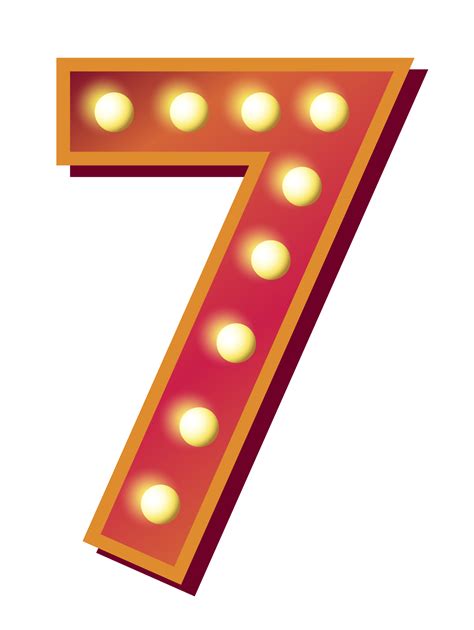
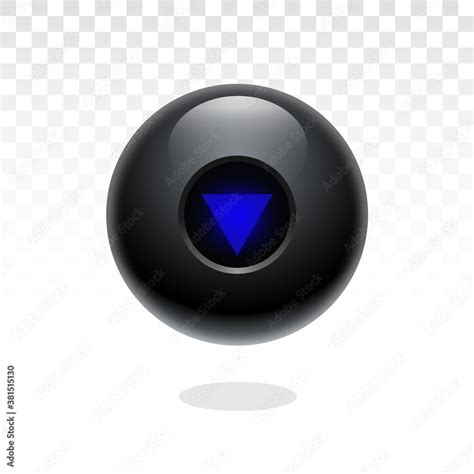
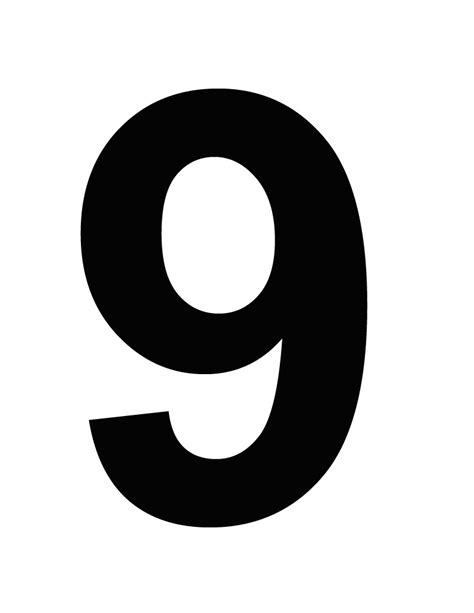
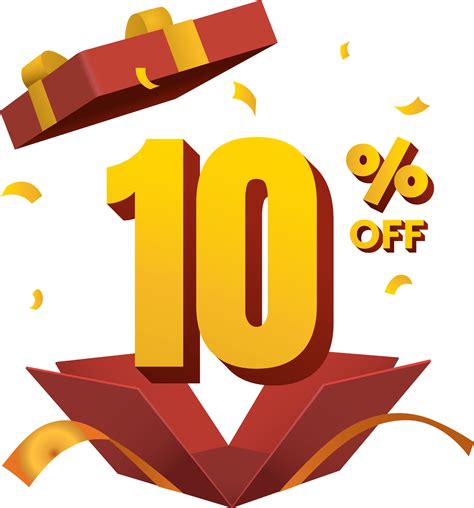
Image 1
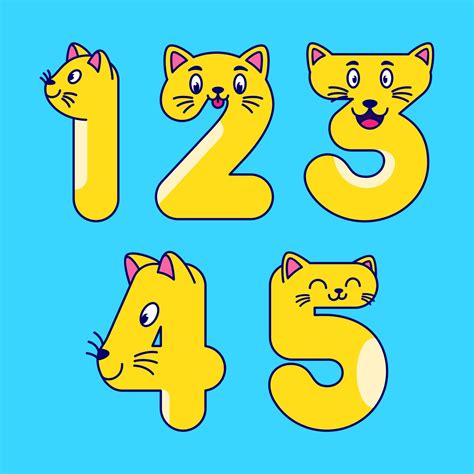
Image 2
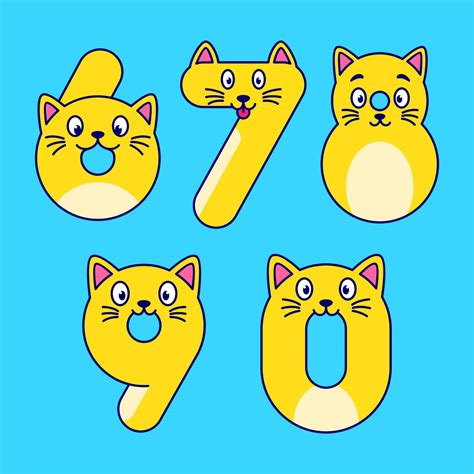
Image 3
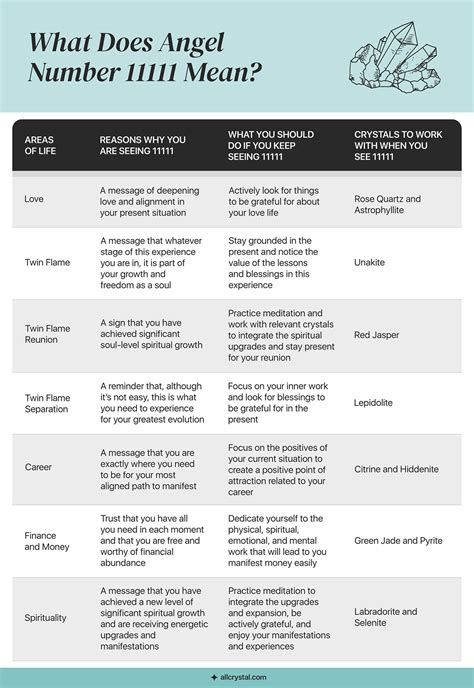
Get Started
To get started with the starter template, clone the repository and run the following command:
npm install
npm run dev
This will start the development server, and you can access the application at http://localhost:3000
.
Conclusion
In this article, we created a starter template using Next.js, TypeScript, and Material-UI. We explored the features of each technology and how they can be used together to build a modern web application. We also created a gallery section using Material-UI and added images to the template.
We hope this article has helped you get started with building your own web application using Next.js, TypeScript, and Material-UI. If you have any questions or need further assistance, please don't hesitate to ask.