Intro
Discover how to streamline email management with VBA. Learn 5 efficient ways to save attachments from multiple emails, including automating file saving, using loops, and leveraging Excel VBA. Improve productivity and reduce manual effort with these expert tips and code examples, perfect for Outlook VBA and email automation enthusiasts.
In today's digital age, email has become an essential tool for communication in both personal and professional settings. With the rise of email, managing attachments has become a crucial aspect of our daily lives. However, manually saving attachments from multiple emails can be a time-consuming and tedious task. Fortunately, with the help of VBA (Visual Basic for Applications), you can automate this process and save a significant amount of time. In this article, we will explore five ways to save attachments from multiple emails using VBA.
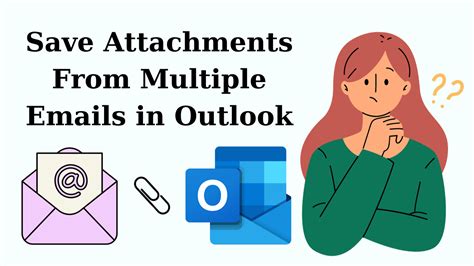
Method 1: Using a Simple VBA Script
One of the simplest ways to save attachments from multiple emails using VBA is by creating a script that loops through all emails in a specific folder and saves their attachments to a designated folder. Here's an example script:
Sub SaveAttachments()
Dim olApp As New Outlook.Application
Dim olNamespace As Namespace
Dim olFolder As MAPIFolder
Dim olItem As Object
Dim olAttachment As Attachment
Dim i As Long
Set olNamespace = olApp.GetNamespace("MAPI")
Set olFolder = olNamespace.GetDefaultFolder(olFolderInbox)
For Each olItem In olFolder.Items
If olItem.Class = olMail Then
For Each olAttachment In olItem.Attachments
olAttachment.SaveAsFile "C:\Attachments\" & olAttachment.FileName
Next olAttachment
End If
Next olItem
End Sub
This script uses the Outlook object library to access the inbox folder and loop through each email. It then checks if the email has any attachments and saves them to a folder named "Attachments" on the C drive.
Step-by-Step Instructions
- Open the Visual Basic Editor in Outlook by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, click Insert > Module to insert a new module.
- Paste the script into the module.
- Update the folder path in the script to the desired location.
- Run the script by clicking Run > Run Sub/UserForm or by pressing F5.
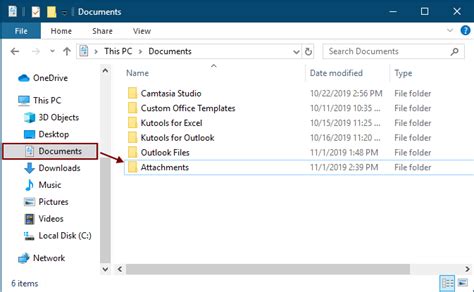
Method 2: Using a VBA Macro with UserForm
Another way to save attachments from multiple emails using VBA is by creating a macro that uses a UserForm to select the folder and email account. Here's an example script:
Sub SaveAttachmentsWithUserForm()
Dim olApp As New Outlook.Application
Dim olNamespace As Namespace
Dim olFolder As MAPIFolder
Dim olItem As Object
Dim olAttachment As Attachment
Dim i As Long
Dim folderPath As String
Dim emailAccount As String
folderPath = InputBox("Enter the folder path to save attachments:", "Folder Path")
emailAccount = InputBox("Enter the email account to save attachments from:", "Email Account")
Set olNamespace = olApp.GetNamespace("MAPI")
Set olFolder = olNamespace.GetDefaultFolder(olFolderInbox)
For Each olItem In olFolder.Items
If olItem.Class = olMail Then
For Each olAttachment In olItem.Attachments
olAttachment.SaveAsFile folderPath & olAttachment.FileName
Next olAttachment
End If
Next olItem
End Sub
This script uses the Outlook object library to access the inbox folder and loop through each email. It then prompts the user to enter the folder path and email account using an InputBox. Finally, it saves the attachments to the specified folder.
Step-by-Step Instructions
- Open the Visual Basic Editor in Outlook by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, click Insert > Module to insert a new module.
- Paste the script into the module.
- Update the folder path and email account variables to the desired values.
- Run the script by clicking Run > Run Sub/UserForm or by pressing F5.
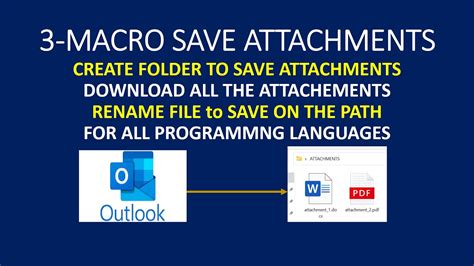
Method 3: Using a VBA Script with Outlook Rules
Another way to save attachments from multiple emails using VBA is by creating a script that uses Outlook rules to automatically save attachments to a designated folder. Here's an example script:
Sub SaveAttachmentsWithRules()
Dim olApp As New Outlook.Application
Dim olNamespace As Namespace
Dim olRule As Outlook.Rule
Dim olCondition As Outlook.Condition
Dim olAction As Outlook.Action
Set olNamespace = olApp.GetNamespace("MAPI")
For Each olRule In olNamespace.Rules
If olRule.Name = "Save Attachments" Then
olRule.Delete
End If
Next olRule
Set olRule = olNamespace.CreateRule
olRule.Name = "Save Attachments"
olRule.RuleType = olRuleReceive
Set olCondition = olRule.Conditions.Item(olConditionSubject)
olCondition.Enabled = True
olCondition.Text = "*"
Set olAction = olRule.Actions.Item(olActionSave)
olAction.Enabled = True
olAction.FolderPath = "C:\Attachments"
olRule.Save
End Sub
This script uses the Outlook object library to access the rules folder and create a new rule named "Save Attachments". The rule is set to save attachments to a folder named "Attachments" on the C drive.
Step-by-Step Instructions
- Open the Visual Basic Editor in Outlook by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, click Insert > Module to insert a new module.
- Paste the script into the module.
- Update the folder path variable to the desired value.
- Run the script by clicking Run > Run Sub/UserForm or by pressing F5.
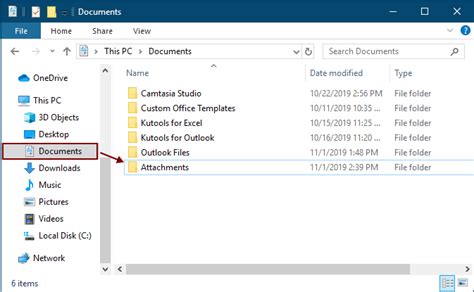
Method 4: Using a VBA Macro with Excel
Another way to save attachments from multiple emails using VBA is by creating a macro that uses Excel to extract the attachments from the emails. Here's an example script:
Sub SaveAttachmentsWithExcel()
Dim olApp As New Outlook.Application
Dim olNamespace As Namespace
Dim olFolder As MAPIFolder
Dim olItem As Object
Dim olAttachment As Attachment
Dim i As Long
Dim xlApp As Excel.Application
Dim xlWorkbook As Excel.Workbook
Dim xlWorksheet As Excel.Worksheet
Set olNamespace = olApp.GetNamespace("MAPI")
Set olFolder = olNamespace.GetDefaultFolder(olFolderInbox)
Set xlApp = New Excel.Application
Set xlWorkbook = xlApp.Workbooks.Add
Set xlWorksheet = xlWorkbook.Sheets(1)
For Each olItem In olFolder.Items
If olItem.Class = olMail Then
For Each olAttachment In olItem.Attachments
olAttachment.SaveAsFile "C:\Attachments\" & olAttachment.FileName
xlWorksheet.Cells(i, 1).Value = olAttachment.FileName
i = i + 1
Next olAttachment
End If
Next olItem
xlWorkbook.SaveAs "C:\Attachments\Attachments.xlsx"
xlApp.Quit
End Sub
This script uses the Outlook object library to access the inbox folder and loop through each email. It then uses Excel to extract the attachments from the emails and save them to a folder named "Attachments" on the C drive.
Step-by-Step Instructions
- Open the Visual Basic Editor in Outlook by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, click Insert > Module to insert a new module.
- Paste the script into the module.
- Update the folder path variable to the desired value.
- Run the script by clicking Run > Run Sub/UserForm or by pressing F5.
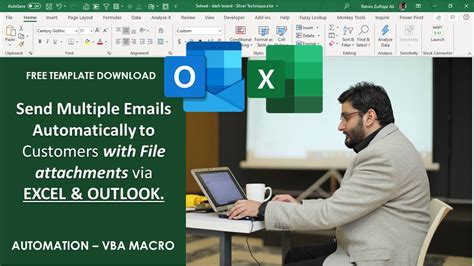
Method 5: Using a VBA Script with Outlook VBA Editor
Another way to save attachments from multiple emails using VBA is by creating a script that uses the Outlook VBA Editor to access the inbox folder and loop through each email. Here's an example script:
Sub SaveAttachmentsWithVBAEditor()
Dim olApp As New Outlook.Application
Dim olNamespace As Namespace
Dim olFolder As MAPIFolder
Dim olItem As Object
Dim olAttachment As Attachment
Dim i As Long
Set olNamespace = olApp.GetNamespace("MAPI")
Set olFolder = olNamespace.GetDefaultFolder(olFolderInbox)
For Each olItem In olFolder.Items
If olItem.Class = olMail Then
For Each olAttachment In olItem.Attachments
olAttachment.SaveAsFile "C:\Attachments\" & olAttachment.FileName
Next olAttachment
End If
Next olItem
End Sub
This script uses the Outlook object library to access the inbox folder and loop through each email. It then saves the attachments to a folder named "Attachments" on the C drive.
Step-by-Step Instructions
- Open the Visual Basic Editor in Outlook by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, click Insert > Module to insert a new module.
- Paste the script into the module.
- Update the folder path variable to the desired value.
- Run the script by clicking Run > Run Sub/UserForm or by pressing F5.
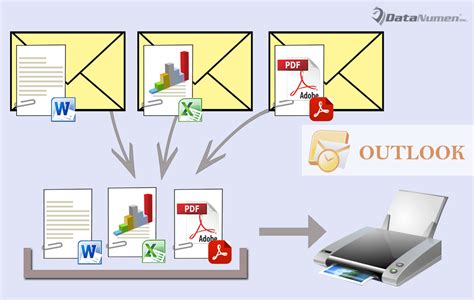
Conclusion
In conclusion, saving attachments from multiple emails using VBA can be achieved through various methods. Each method has its own advantages and disadvantages, and the choice of method depends on the specific requirements of the task. By following the step-by-step instructions and using the provided scripts, you can automate the process of saving attachments from multiple emails and save time.
Gallery of VBA Scripts to Save Attachments from Multiple Emails
VBA Scripts to Save Attachments from Multiple Emails
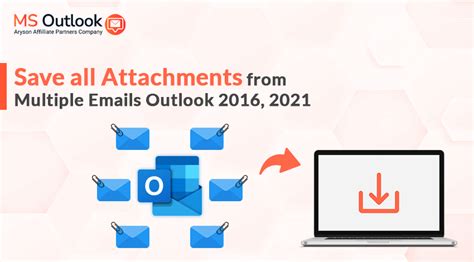
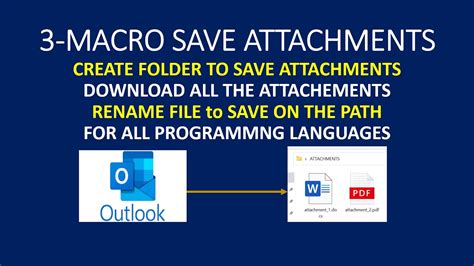
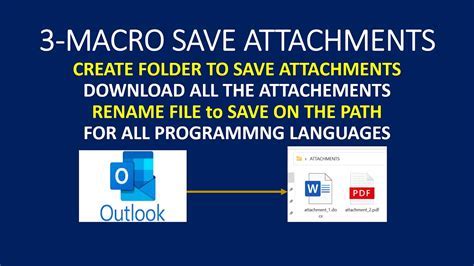
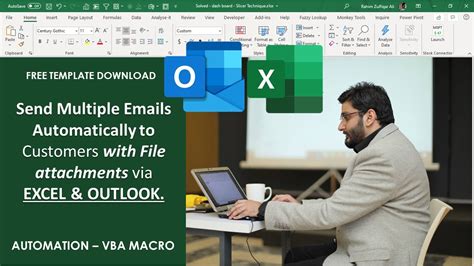
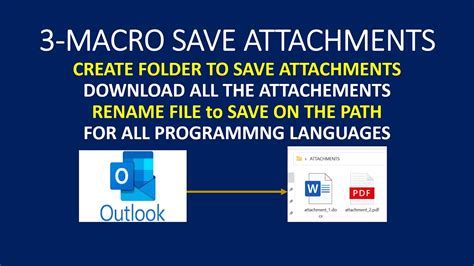
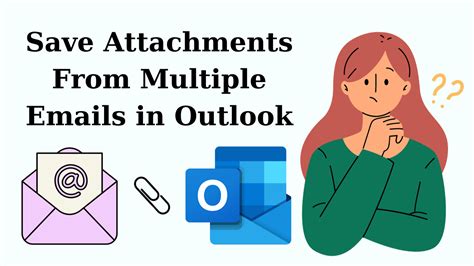
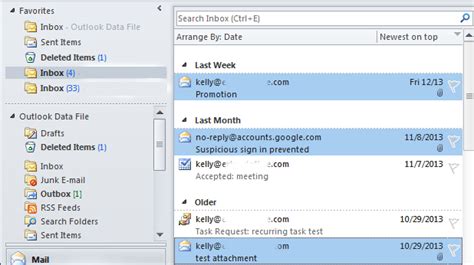
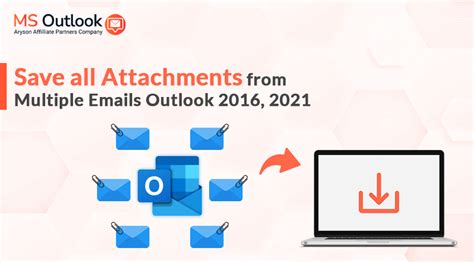
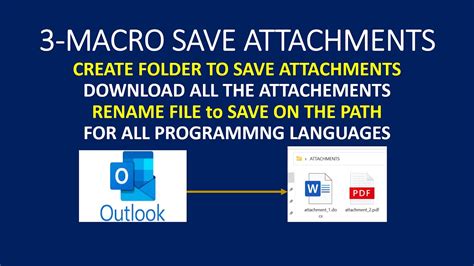
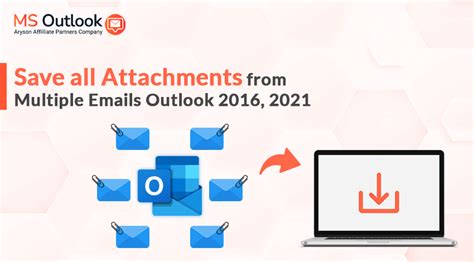
We hope this article has been helpful in providing you with the necessary information to save attachments from multiple emails using VBA. If you have any questions or need further assistance, please don't hesitate to ask.