Intro
Unlock the power of data analysis by exporting PowerShell data to Excel with ease. Learn how to seamlessly integrate PowerShell with Excel, leveraging cmdlets, and modules to streamline data export. Discover the simplest methods to format and manipulate data, making it Excel-ready, and elevate your reporting capabilities.
Working with data in PowerShell can be a powerful experience, but sometimes you need to share that data with others or analyze it further in a more user-friendly format. This is where exporting PowerShell data to Excel comes in. Excel is a popular choice for data analysis, and with the right techniques, you can easily export your PowerShell data to Excel for further manipulation.
PowerShell is an incredible tool for automating tasks and working with data, but it can be overwhelming to manage large datasets directly in the console. Excel, on the other hand, provides a visual interface that makes it easier to analyze and understand complex data. By exporting your PowerShell data to Excel, you can take advantage of Excel's features, such as filtering, sorting, and charting, to gain deeper insights into your data.
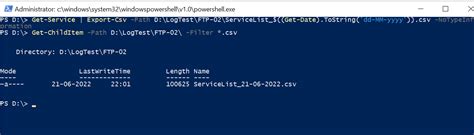
In this article, we will explore the various methods for exporting PowerShell data to Excel, including using the Export-CSV cmdlet, working with Excel COM objects, and leveraging third-party modules.
Why Export PowerShell Data to Excel?
There are several reasons why you might want to export your PowerShell data to Excel:
- Data analysis: Excel provides a wide range of tools and features for analyzing data, including filtering, sorting, and charting.
- Data visualization: Excel's visualization tools, such as charts and graphs, can help you understand complex data and identify trends.
- Data sharing: Excel is a widely used format, making it easy to share your data with others who may not have PowerShell installed.
- Data manipulation: Excel provides a range of tools for manipulating data, including formulas, pivot tables, and macros.
Method 1: Using the Export-CSV Cmdlet
The Export-CSV cmdlet is a built-in cmdlet in PowerShell that allows you to export data to a CSV file, which can then be easily imported into Excel.
# Create a sample array
$data = @(
[PSCustomObject]@{Name='John';Age=25;City='New York'},
[PSCustomObject]@{Name='Jane';Age=30;City='Chicago'},
[PSCustomObject]@{Name='Bob';Age=35;City='Los Angeles'}
)
# Export the data to a CSV file
$data | Export-CSV -Path 'C:\data.csv' -NoTypeInformation
This code creates a sample array of custom objects and exports it to a CSV file using the Export-CSV cmdlet. The -NoTypeInformation
parameter is used to exclude the type information from the CSV file.
Method 2: Working with Excel COM Objects
Another method for exporting PowerShell data to Excel is by using Excel COM objects. This method provides more control over the export process and allows you to manipulate the Excel file programmatically.
# Create a sample array
$data = @(
[PSCustomObject]@{Name='John';Age=25;City='New York'},
[PSCustomObject]@{Name='Jane';Age=30;City='Chicago'},
[PSCustomObject]@{Name='Bob';Age=35;City='Los Angeles'}
)
# Create a new Excel application
$excel = New-Object -ComObject Excel.Application
# Create a new workbook
$workbook = $excel.Workbooks.Add()
# Create a new worksheet
$worksheet = $workbook.Sheets.Item(1)
# Set the data in the worksheet
for ($i = 0; $i -lt $data.Count; $i++) {
$worksheet.Cells.Item(1, $i + 1) = $data[$i].Name
$worksheet.Cells.Item(2, $i + 1) = $data[$i].Age
$worksheet.Cells.Item(3, $i + 1) = $data[$i].City
}
# Save the workbook
$workbook.SaveAs('C:\data.xlsx')
# Close the workbook and application
$workbook.Close()
$excel.Quit()
This code creates a sample array of custom objects and exports it to an Excel file using Excel COM objects. The data is set in the worksheet, and the workbook is saved to a file.
Method 3: Leveraging Third-Party Modules
There are also third-party modules available that can simplify the process of exporting PowerShell data to Excel. One popular module is the ImportExcel
module.
# Install the ImportExcel module
Install-Module -Name ImportExcel
# Create a sample array
$data = @(
[PSCustomObject]@{Name='John';Age=25;City='New York'},
[PSCustomObject]@{Name='Jane';Age=30;City='Chicago'},
[PSCustomObject]@{Name='Bob';Age=35;City='Los Angeles'}
)
# Export the data to an Excel file
$data | Export-Excel -Path 'C:\data.xlsx'
This code creates a sample array of custom objects and exports it to an Excel file using the Export-Excel
cmdlet from the ImportExcel
module.
Tips and Variations
Here are some additional tips and variations to keep in mind when exporting PowerShell data to Excel:
- Use the
-NoTypeInformation
parameter: When using the Export-CSV cmdlet, use the-NoTypeInformation
parameter to exclude type information from the CSV file. - Use Excel COM objects for more control: If you need more control over the export process, consider using Excel COM objects.
- Use third-party modules for simplicity: Third-party modules like
ImportExcel
can simplify the process of exporting PowerShell data to Excel. - Use Excel templates: If you have a standard Excel template, you can use it to format your data before exporting it.
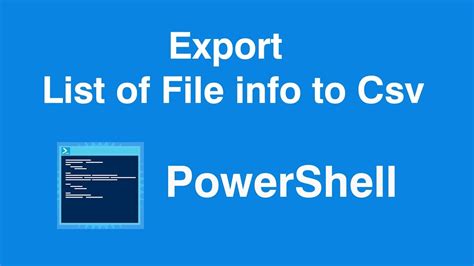
Gallery of Exporting PowerShell Data
Exporting PowerShell Data to Excel Gallery
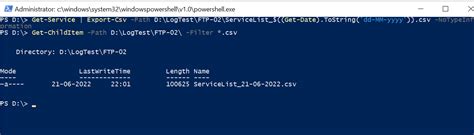
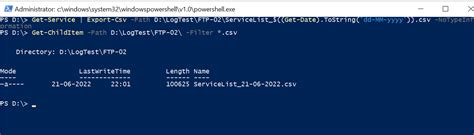
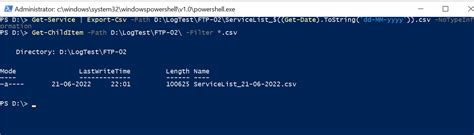
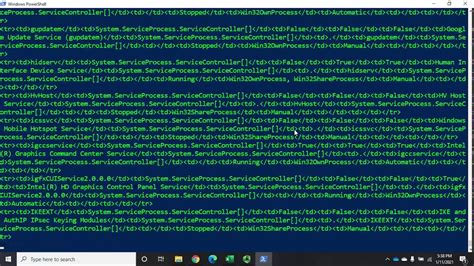
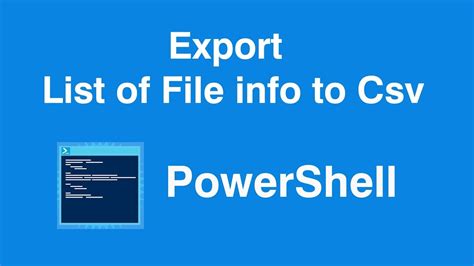
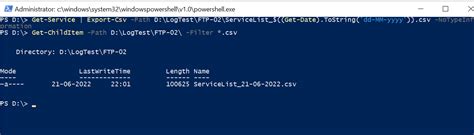
We hope this article has provided you with a comprehensive guide on how to export PowerShell data to Excel. Whether you're using the Export-CSV cmdlet, working with Excel COM objects, or leveraging third-party modules, there are many ways to get your data into Excel for further analysis.