Intro
Boost your VBA coding skills with efficient progress bars. Learn how to master VBA progress bars to enhance user experience, track task status, and speed up development. Discover expert techniques, best practices, and code examples to create customizable, interactive, and responsive progress bars, optimizing your VBA workflow with accurate, reliable, and visually appealing results.
Mastering VBA progress bars is an essential skill for any developer looking to create efficient and user-friendly code. In this article, we will delve into the world of VBA progress bars, exploring their importance, benefits, and step-by-step instructions on how to create and customize them.
The Importance of VBA Progress Bars
VBA progress bars are a crucial component of any well-designed application. They provide users with visual feedback on the progress of a task, helping to manage expectations and reduce anxiety. By incorporating progress bars into your code, you can significantly enhance the user experience, making your application more engaging and interactive.
Benefits of VBA Progress Bars
- Improved User Experience: Progress bars keep users informed about the progress of a task, reducing frustration and anxiety.
- Enhanced Code Efficiency: By providing visual feedback, progress bars enable developers to identify performance bottlenecks and optimize code for better performance.
- Increased Transparency: Progress bars offer a clear indication of the time required to complete a task, allowing users to plan and manage their time more effectively.
Creating a Basic VBA Progress Bar
To create a basic VBA progress bar, follow these steps:
- Open the Visual Basic Editor by pressing
Alt + F11
or navigating toDeveloper
>Visual Basic
in the ribbon. - Insert a new module by clicking
Insert
>Module
or pressingAlt + F11
again. - Paste the following code into the module:
Sub ProgressBarExample()
Dim i As Long
Dim maxIterations As Long
maxIterations = 100
' Initialize progress bar
UserForm1.Show
' Simulate a time-consuming task
For i = 1 To maxIterations
' Update progress bar
UserForm1.ProgressBar1.Value = i
' Simulate a delay
Application.Wait Now + #12:00:01 AM#
Next i
' Hide progress bar
UserForm1.Hide
End Sub
- Create a new user form by clicking
Insert
>User Form
or pressingAlt + F11
again. - Add a progress bar control to the user form by dragging and dropping the
ProgressBar
control from theToolbox
. - Set the
Value
property of the progress bar to0
and theMax
property to100
.
Customizing VBA Progress Bars
To customize your VBA progress bar, you can modify its appearance, behavior, and interaction with the user. Here are some examples:
Changing the Progress Bar's Appearance
- Set the
ForeColor
property to change the color of the progress bar. - Set the
BackColor
property to change the background color of the progress bar. - Set the
BorderStyle
property to change the border style of the progress bar.
Changing the Progress Bar's Behavior
- Set the
Enabled
property toFalse
to disable the progress bar. - Set the
Visible
property toFalse
to hide the progress bar. - Use the
ProgressBar1.Value
property to update the progress bar's value.
Interacting with the User
- Use the
MsgBox
function to display a message box with the progress bar's value. - Use the
InputBox
function to prompt the user to enter a value.
Example Code: Customizing the Progress Bar
Sub CustomProgressBarExample()
Dim i As Long
Dim maxIterations As Long
maxIterations = 100
' Initialize progress bar
UserForm1.Show
' Change progress bar's appearance
UserForm1.ProgressBar1.ForeColor = RGB(255, 0, 0) ' Red
UserForm1.ProgressBar1.BackColor = RGB(0, 0, 255) ' Blue
' Simulate a time-consuming task
For i = 1 To maxIterations
' Update progress bar
UserForm1.ProgressBar1.Value = i
' Simulate a delay
Application.Wait Now + #12:00:01 AM#
' Display a message box with the progress bar's value
MsgBox "Progress: " & UserForm1.ProgressBar1.Value & "%"
Next i
' Hide progress bar
UserForm1.Hide
End Sub
Best Practices for VBA Progress Bars
- Use a consistent design: Use a consistent design throughout your application to create a cohesive user experience.
- Provide clear feedback: Provide clear feedback to the user about the progress of the task.
- Test and optimize: Test your progress bar and optimize its performance for better efficiency.
- Use VBA's built-in controls: Use VBA's built-in controls, such as the
ProgressBar
control, to create a progress bar.
Gallery of VBA Progress Bars
VBA Progress Bar Image Gallery
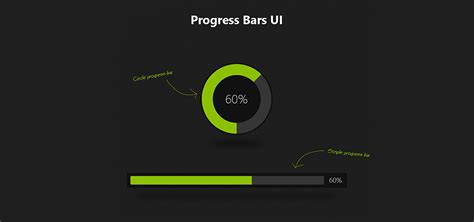
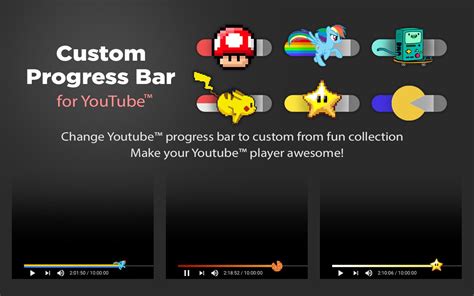
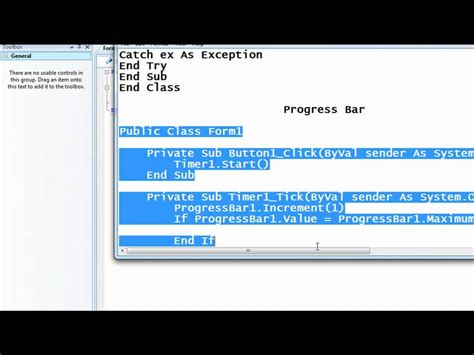
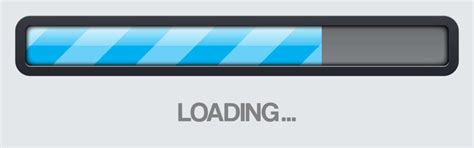
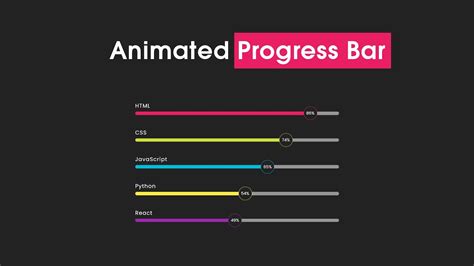
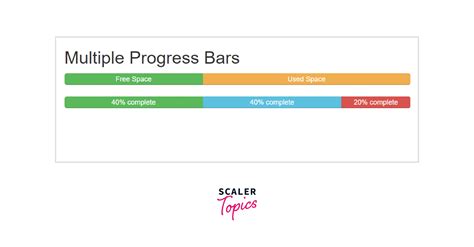
Conclusion
Mastering VBA progress bars is an essential skill for any developer looking to create efficient and user-friendly code. By following the steps outlined in this article, you can create and customize your own VBA progress bars, providing users with a better experience and improving the overall performance of your application. Remember to test and optimize your progress bar, and don't hesitate to reach out if you have any questions or need further assistance. Happy coding!