Intro
Pythons Invalid Non-Printable Character U+00a0 is a common issue that many programmers face when working with Python. This error occurs when Python encounters a non-printable character, specifically U+00a0, which is a non-breaking space character. In this article, we will explore the reasons behind this error and provide 5 ways to fix it.
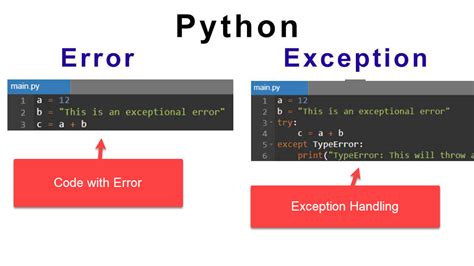
Understanding the Error
Before we dive into the solutions, it's essential to understand the error message. The error message "Invalid non-printable character U+00a0" indicates that Python has encountered a non-printable character that it cannot interpret. This character is often invisible, making it difficult to identify.
5 Ways to Fix Pythons Invalid Non-Printable Character U+00a0
Here are five ways to fix the Invalid Non-Printable Character U+00a0 error in Python:
Method 1: Remove the Non-Breaking Space Character
The most straightforward way to fix the error is to remove the non-breaking space character from your code. You can do this by copying and pasting your code into a text editor and then re-pasting it into your Python environment.
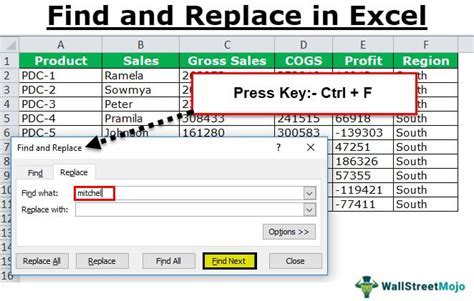
Step-by-Step Instructions
- Copy your code into a text editor, such as Notepad or TextEdit.
- Use the "Find and Replace" function to search for the non-breaking space character (U+00a0).
- Replace the character with a regular space (U+0020).
- Copy and paste the modified code into your Python environment.
Method 2: Use the `.replace()` Method
If you're working with a string that contains the non-breaking space character, you can use the .replace()
method to replace it with a regular space.
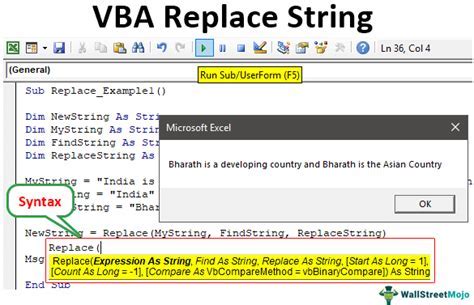
Example Code
my_string = "Hello World"
my_string = my_string.replace(u'\xa0', u' ')
print(my_string)
Method 3: Use the ` encode()` and `decode()` Methods
Another way to fix the error is to use the encode()
and decode()
methods to convert the string to a bytes object and then back to a string.
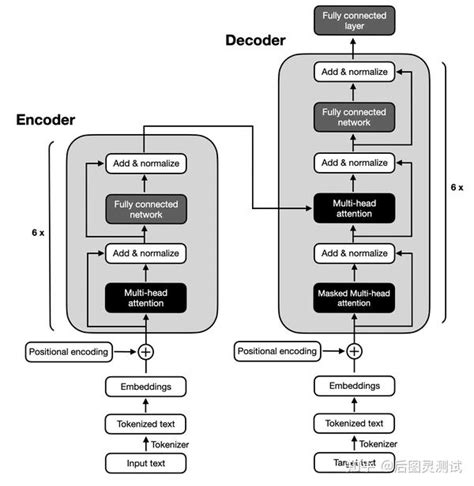
Example Code
my_string = "Hello World"
my_bytes = my_string.encode('utf-8')
my_string = my_bytes.decode('utf-8')
print(my_string)
Method 4: Use a Regular Expression
You can use a regular expression to replace the non-breaking space character with a regular space.
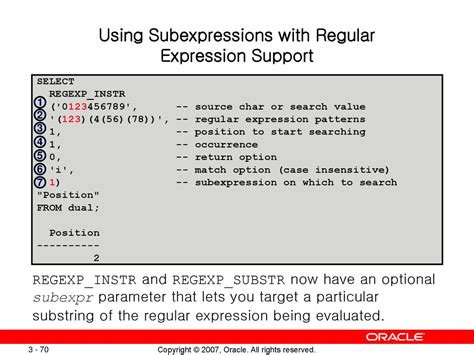
Example Code
import re
my_string = "Hello World"
my_string = re.sub(u'\xa0', u' ', my_string)
print(my_string)
Method 5: Use a Unicode-Aware Text Editor
Finally, you can use a Unicode-aware text editor to edit your code. These editors can display non-printable characters, making it easier to identify and remove them.
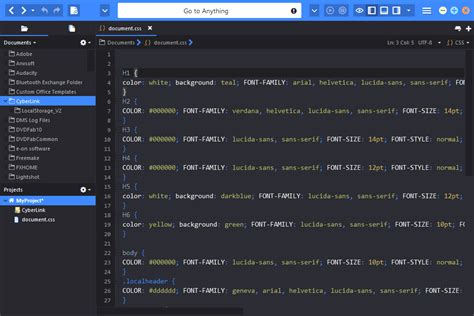
Recommended Text Editors
- Sublime Text
- Atom
- Visual Studio Code
Gallery of Python Error U+00a0
Python Error U+00a0 Gallery
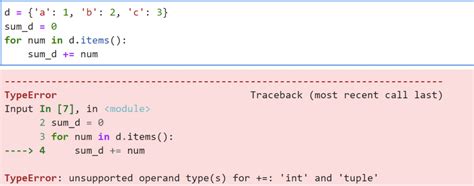
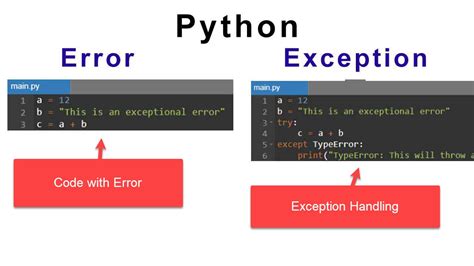
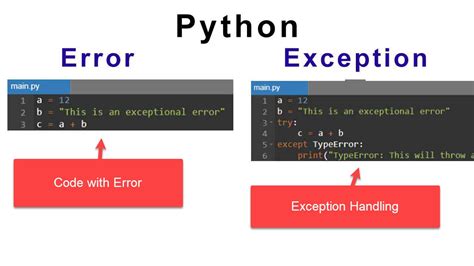
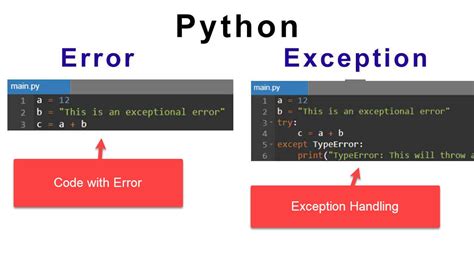
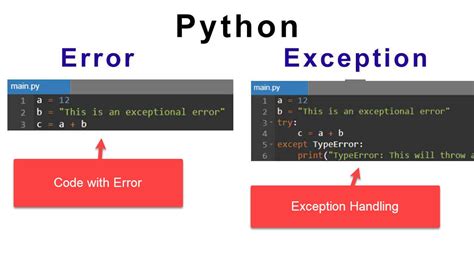
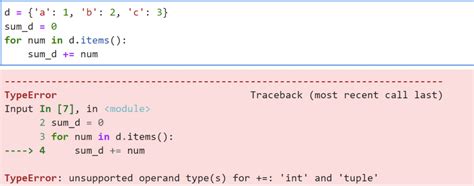
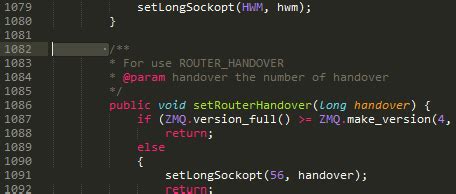


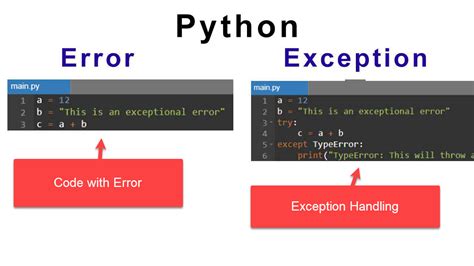
We hope this article has helped you fix the Pythons Invalid Non-Printable Character U+00a0 error. Remember to remove non-breaking space characters, use the .replace()
method, encode and decode strings, use regular expressions, and use Unicode-aware text editors to avoid this error in the future. If you have any questions or need further assistance, please leave a comment below.