Intro
Random number generators are a crucial tool in various fields, including statistics, engineering, and computer science. In VBA (Visual Basic for Applications), creating a random number generator can be useful for simulations, modeling, and testing. Here are five ways to create a random number generator in VBA:
Random numbers are essential in many applications, including statistical analysis, simulations, and modeling. In VBA, you can generate random numbers using various methods, each with its strengths and weaknesses. In this article, we will explore five ways to create a random number generator in VBA, providing you with a comprehensive understanding of the different approaches.
Why Do You Need a Random Number Generator in VBA?
Before we dive into the methods, let's quickly discuss why you might need a random number generator in VBA. Random numbers can be useful in various scenarios, such as:
- Simulating real-world events, like stock prices or weather patterns
- Modeling complex systems, like population growth or chemical reactions
- Testing hypotheses, like the effectiveness of a new marketing strategy
- Creating games, like lotteries or card games
Method 1: Using the Rnd
Function
The Rnd
function is a built-in VBA function that generates a random number between 0 and 1. You can use this function to generate random numbers within a specific range by multiplying the result by the desired range.
Sub GenerateRandomNumber()
Dim randomNumber As Double
randomNumber = Rnd * 100 ' generates a random number between 0 and 100
Debug.Print randomNumber
End Sub
Image:
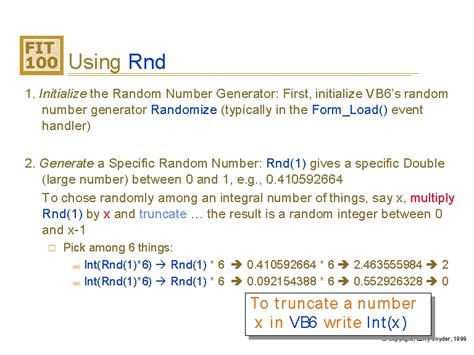
Method 2: Using the Randomize
Statement
The Randomize
statement is used to initialize the random number generator with a seed value. This ensures that the random numbers generated are truly random and not reproducible.
Sub GenerateRandomNumber()
Dim randomNumber As Double
Randomize ' initializes the random number generator
randomNumber = Int((100 * Rnd) + 1) ' generates a random number between 1 and 100
Debug.Print randomNumber
End Sub
Image:
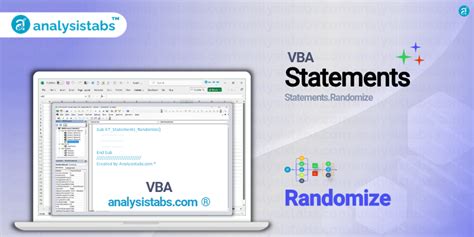
Method 3: Using a Loop to Generate Random Numbers
This method uses a loop to generate multiple random numbers. You can specify the number of random numbers you want to generate and the range of values.
Sub GenerateRandomNumbers()
Dim i As Integer
Dim randomNumber As Double
For i = 1 To 10 ' generates 10 random numbers
randomNumber = Rnd * 100 ' generates a random number between 0 and 100
Debug.Print randomNumber
Next i
End Sub
Image:
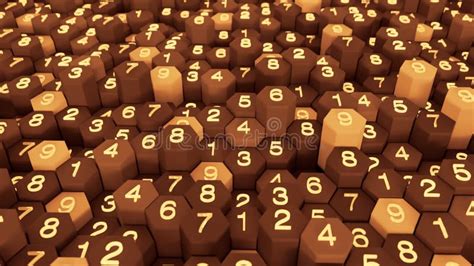
Method 4: Using an Array to Store Random Numbers
This method generates an array of random numbers. You can specify the size of the array and the range of values.
Sub GenerateRandomNumberArray()
Dim randomNumberArray() As Double
Dim i As Integer
ReDim randomNumberArray(1 To 10) ' generates an array of 10 random numbers
For i = 1 To 10
randomNumberArray(i) = Rnd * 100 ' generates a random number between 0 and 100
Next i
Debug.Print randomNumberArray
End Sub
Image:
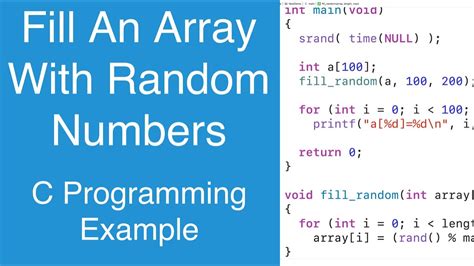
Method 5: Using a Custom Function to Generate Random Numbers
This method creates a custom function that generates a random number within a specified range.
Function GenerateRandomNumber(minValue As Double, maxValue As Double) As Double
GenerateRandomNumber = Rnd * (maxValue - minValue) + minValue
End Function
Sub TestGenerateRandomNumber()
Dim randomNumber As Double
randomNumber = GenerateRandomNumber(1, 100) ' generates a random number between 1 and 100
Debug.Print randomNumber
End Sub
Image:
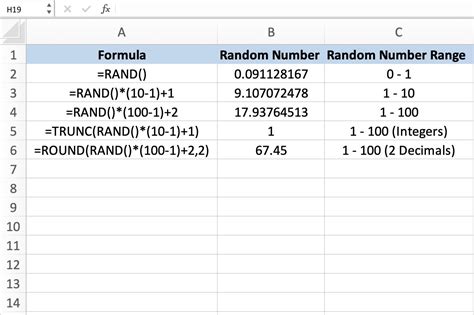
Gallery of Random Number Generators in VBA
Random Number Generators in VBA
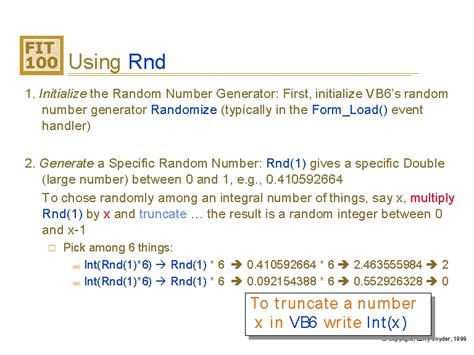
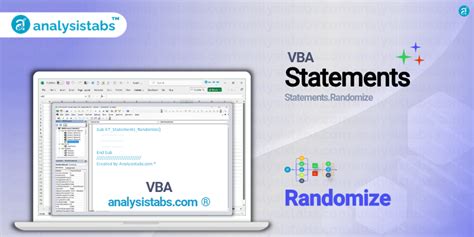
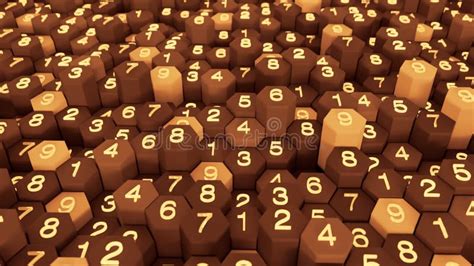
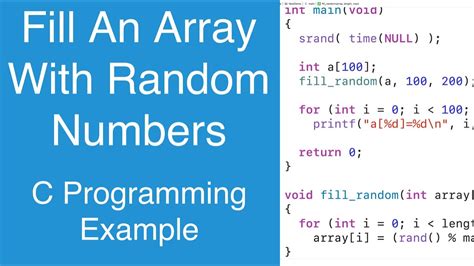
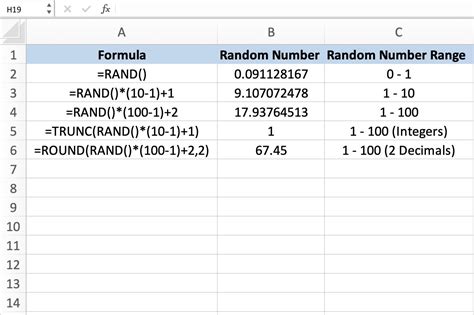
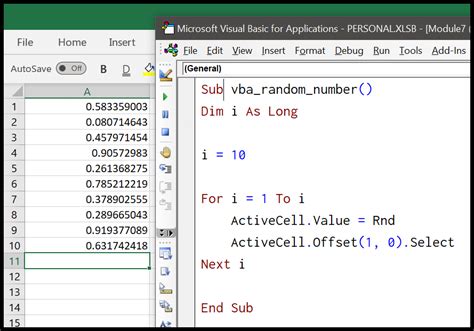
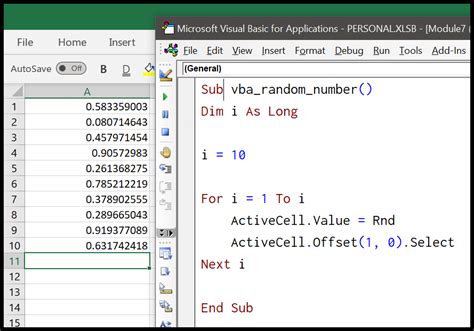
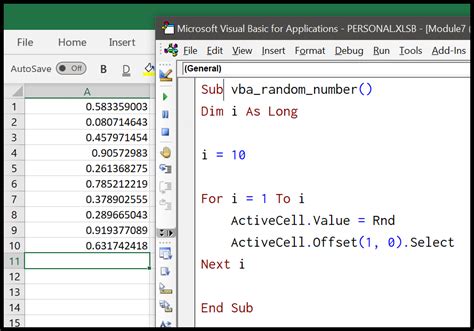
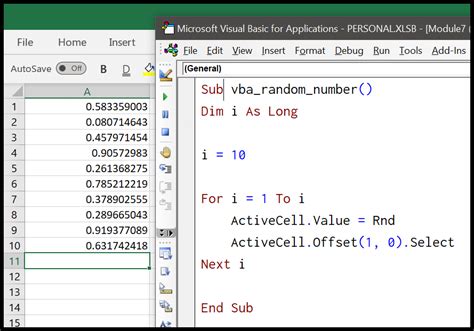
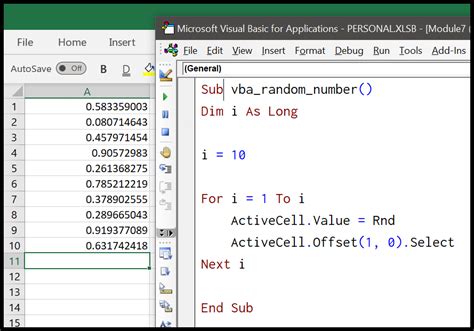
In conclusion, there are several ways to create a random number generator in VBA, each with its strengths and weaknesses. By understanding the different methods, you can choose the best approach for your specific needs. Whether you need to simulate real-world events, model complex systems, or test hypotheses, a random number generator can be a valuable tool in your VBA arsenal.
We hope this article has been informative and helpful in your VBA journey. If you have any questions or need further clarification, please don't hesitate to ask. Share your thoughts and experiences with random number generators in VBA in the comments section below.