Intro
Master Excel VBA with 5 expert ways to range copy, including dynamic range selection, cell referencing, and more. Learn to automate copying ranges with ease, using relative and absolute references, and discover how to avoid common errors. Improve your VBA skills and boost productivity with these practical range copying techniques.
In the world of Excel VBA, working with ranges is a fundamental skill that can make or break the efficiency of your code. One of the most common tasks you'll encounter is copying data from one range to another. In this article, we'll explore five ways to range copy in Excel VBA, highlighting the benefits and use cases for each method.
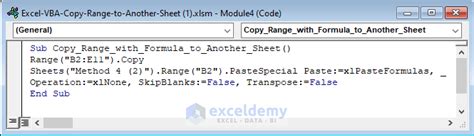
Understanding Range Copying
Before we dive into the methods, it's essential to understand what range copying entails. Range copying involves selecting a range of cells in Excel and duplicating its contents to another range. This can be useful for various tasks, such as data manipulation, report generation, and automation.
Method 1: Using the Range.Copy
Method
The most straightforward way to copy a range in Excel VBA is by using the Range.Copy
method. This method allows you to copy the entire range, including formulas, formatting, and values.
Sub CopyRangeMethod1()
Dim sourceRange As Range
Dim targetRange As Range
Set sourceRange = ThisWorkbook.Worksheets("Sheet1").Range("A1:E10")
Set targetRange = ThisWorkbook.Worksheets("Sheet2").Range("A1:E10")
sourceRange.Copy targetRange
End Sub
This method is ideal for simple copying tasks, but it can be slow for large ranges due to the overhead of copying all data, including formatting.
Method 2: Using the Range.Value
Property
Another way to copy a range is by using the Range.Value
property. This method copies only the values from the source range to the target range.
Sub CopyRangeMethod2()
Dim sourceRange As Range
Dim targetRange As Range
Set sourceRange = ThisWorkbook.Worksheets("Sheet1").Range("A1:E10")
Set targetRange = ThisWorkbook.Worksheets("Sheet2").Range("A1:E10")
targetRange.Value = sourceRange.Value
End Sub
This method is faster than the first method, but it only copies values, not formatting or formulas.
Method 3: Using the Range.Formula
Property
If you need to copy formulas from the source range to the target range, you can use the Range.Formula
property.
Sub CopyRangeMethod3()
Dim sourceRange As Range
Dim targetRange As Range
Set sourceRange = ThisWorkbook.Worksheets("Sheet1").Range("A1:E10")
Set targetRange = ThisWorkbook.Worksheets("Sheet2").Range("A1:E10")
targetRange.Formula = sourceRange.Formula
End Sub
This method copies formulas, but not values or formatting.
Method 4: Using the Range.PasteSpecial
Method
The Range.PasteSpecial
method allows you to copy specific aspects of the source range, such as values, formats, or formulas, to the target range.
Sub CopyRangeMethod4()
Dim sourceRange As Range
Dim targetRange As Range
Set sourceRange = ThisWorkbook.Worksheets("Sheet1").Range("A1:E10")
Set targetRange = ThisWorkbook.Worksheets("Sheet2").Range("A1:E10")
sourceRange.Copy
targetRange.PasteSpecial xlPasteValues
Application.CutCopyMode = False
End Sub
This method provides more flexibility than the previous methods, but it requires using the Application.CutCopyMode
property to clear the clipboard.
Method 5: Using the Range.Resize
Method
The Range.Resize
method allows you to copy a range and resize it to fit the target range.
Sub CopyRangeMethod5()
Dim sourceRange As Range
Dim targetRange As Range
Set sourceRange = ThisWorkbook.Worksheets("Sheet1").Range("A1:E10")
Set targetRange = ThisWorkbook.Worksheets("Sheet2").Range("A1:E20")
sourceRange.Copy
targetRange.Resize(sourceRange.Rows.Count, sourceRange.Columns.Count).PasteSpecial xlPasteValues
Application.CutCopyMode = False
End Sub
This method is useful when you need to copy a range and adjust its size to fit the target range.
Gallery of Excel VBA Copy Range Methods
Excel VBA Copy Range Methods Gallery
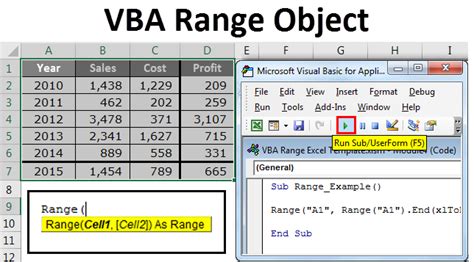
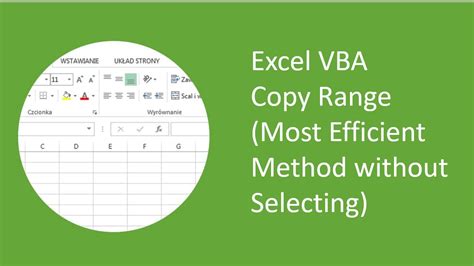
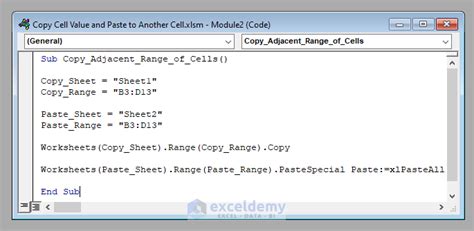
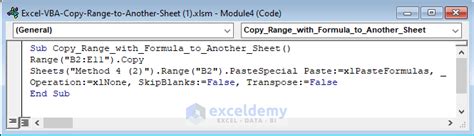
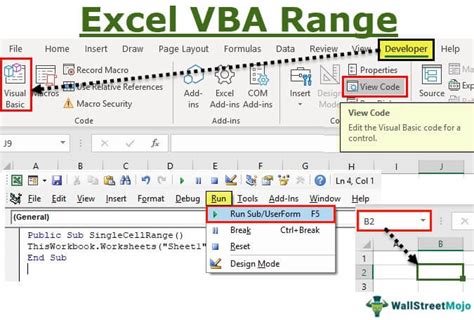
Conclusion: Choose the Right Method
When working with range copying in Excel VBA, it's essential to choose the right method for your specific needs. Each method has its strengths and weaknesses, and understanding these differences can help you write more efficient and effective code. Whether you're copying values, formulas, or formatting, there's a method on this list that's right for you.
Share Your Thoughts
Have you used any of these methods in your Excel VBA projects? Do you have a preferred method for range copying? Share your thoughts and experiences in the comments below!