In today's fast-paced business world, managing emails efficiently is crucial for productivity and effective communication. For those using Microsoft Access, incorporating VBA (Visual Basic for Applications) can significantly enhance email management capabilities. Here are five ways to read emails with Access VBA, covering various scenarios and providing solutions for streamlined email processing.
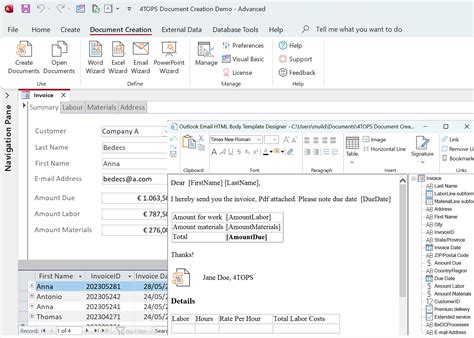
Method 1: Using the Outlook Object Library
One of the most straightforward methods to read emails in Access VBA is by utilizing the Outlook Object Library. This approach allows you to directly interact with Outlook, enabling you to read, write, and manage emails programmatically.
Steps to Integrate Outlook with Access VBA:
- Open Access and Press Alt + F11 to open the VBA Editor.
- Go to Tools > References, and check if "Microsoft Outlook Object Library" is listed. If not, browse and add it.
- Use Early Binding: Declare Outlook objects explicitly, like
Dim olApp As New Outlook.Application
. - Write Code to Read Emails: Loop through the inbox, read the subject and body of each email, and perform actions as needed.
Example Code:
Sub ReadEmailsFromOutlook()
Dim olApp As New Outlook.Application
Dim olNamespace As Namespace
Dim olInbox As MAPIFolder
Dim olItem As Object
Set olNamespace = olApp.GetNamespace("MAPI")
Set olInbox = olNamespace.GetDefaultFolder(olFolderInbox)
For Each olItem In olInbox.Items
If olItem.Class = olMail Then
Debug.Print olItem.Subject, olItem.Body
End If
Next olItem
Set olItem = Nothing
Set olInbox = Nothing
Set olNamespace = Nothing
Set olApp = Nothing
End Sub
Method 2: Using CDO (Collaboration Data Objects)
For situations where Outlook is not available or preferred, CDO offers an alternative way to interact with email servers, allowing you to read emails directly from a POP3 or IMAP account.
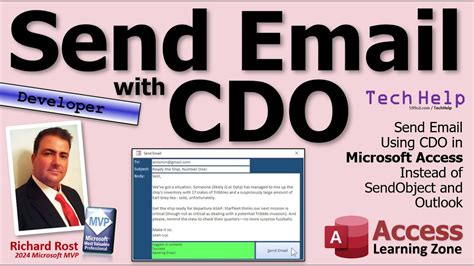
Steps to Integrate CDO with Access VBA:
- Install and Register CDO: Ensure CDO is installed and registered on your system.
- Add Reference to CDO: In the VBA Editor, go to Tools > References, and add "Microsoft CDO for Windows 2000 Library".
- Write Code to Connect to Email Server: Use CDO to establish a connection to the email server and read emails.
Example Code:
Sub ReadEmailsUsingCDO()
Dim cdoSession As New CDO.Session
Dim cdoInbox As New CDO.GetItems
Dim cdoItem As CDO.IExchangeItem
' Configure CDO session
With cdoSession
.Config("http://schemas.microsoft.com/cdo/configuration/url") = "your_email_server"
.Config("http://schemas.microsoft.com/cdo/configuration/username") = "your_username"
.Config("http://schemas.microsoft.com/cdo/configuration/password") = "your_password"
End With
' Retrieve emails
Set cdoInbox = cdoSession.GetItems()
For Each cdoItem In cdoInbox
Debug.Print cdoItem.Subject, cdoItem.TextBody
Next cdoItem
Set cdoItem = Nothing
Set cdoInbox = Nothing
Set cdoSession = Nothing
End Sub
Method 3: Using the Winsock Control
This method involves using the Winsock control in Access to establish a direct connection to the email server via POP3 or IMAP protocols, allowing for email retrieval.
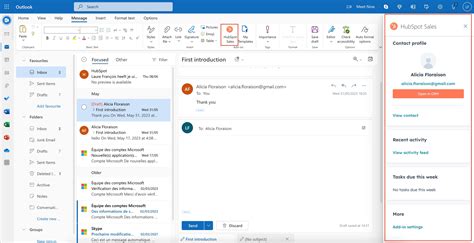
Steps to Use the Winsock Control:
- Insert the Winsock Control: On an Access form, insert the Winsock control from the toolbox.
- Configure Winsock Properties: Set the protocol (POP3 or IMAP) and other properties as needed.
- Write Code to Connect and Read Emails: Use the Winsock control's events and methods to connect to the email server and read emails.
Example Code:
Private Sub cmdReadEmails_Click()
' Connect to email server
Winsock1.Close
Winsock1.LocalPort = 0
Winsock1.Protocol = sckTCPProtocol
Winsock1.RemoteHost = "your_email_server"
Winsock1.RemotePort = 110 ' POP3 port
Winsock1.Connect
' Send POP3 commands to read emails
Winsock1.SendData "USER your_username" & vbCrLf
Winsock1.SendData "PASS your_password" & vbCrLf
Winsock1.SendData "LIST" & vbCrLf
Winsock1.SendData "RETR 1" & vbCrLf ' Retrieve first email
' Process email data received
Dim strEmail As String
strEmail = Winsock1.GetData
Debug.Print strEmail
End Sub
Method 4: Using the Redemption Library
Redemption is a COM library that provides a convenient way to work with emails, including reading emails from Outlook or other email clients.
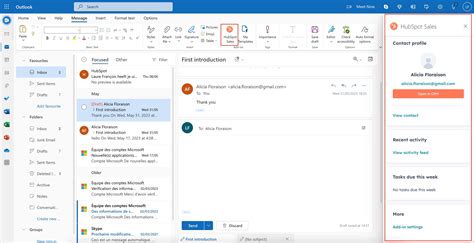
Steps to Use Redemption:
- Install and Register Redemption: Ensure Redemption is installed and registered on your system.
- Add Reference to Redemption: In the VBA Editor, go to Tools > References, and add "Redemption".
- Write Code to Read Emails: Use Redemption to connect to Outlook or another email client and read emails.
Example Code:
Sub ReadEmailsUsingRedemption()
Dim rdoSession As New Redemption.RDO.RDOSession
Dim rdoInbox As New Redemption.RDO.RDOFolder
' Create a new Redemption session
rdoSession.MAPIOBJECT = CreateObject("Outlook.Application").GetNamespace("MAPI")
' Retrieve emails from the inbox
Set rdoInbox = rdoSession.GetDefaultFolder(olFolderInbox)
For Each rdoItem In rdoInbox.Items
If rdoItem.Class = olMail Then
Debug.Print rdoItem.Subject, rdoItem.Body
End If
Next rdoItem
Set rdoItem = Nothing
Set rdoInbox = Nothing
Set rdoSession = Nothing
End Sub
Method 5: Using IMAP and CDO (Alternative Approach)
This method uses CDO to connect to an IMAP email server, providing an alternative approach to reading emails.
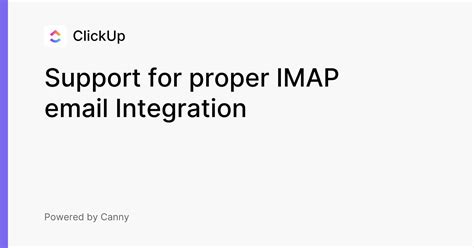
Steps to Use IMAP and CDO:
- Install and Register CDO: Ensure CDO is installed and registered on your system.
- Add Reference to CDO: In the VBA Editor, go to Tools > References, and add "Microsoft CDO for Windows 2000 Library".
- Write Code to Connect to IMAP Server: Use CDO to establish an IMAP connection and read emails.
Example Code:
Sub ReadEmailsUsingIMAPAndCDO()
Dim cdoSession As New CDO.Session
Dim cdoInbox As New CDO.GetItems
Dim cdoItem As CDO.IExchangeItem
' Configure CDO session for IMAP
With cdoSession
.Config("http://schemas.microsoft.com/cdo/configuration/url") = "your_imap_server"
.Config("http://schemas.microsoft.com/cdo/configuration/username") = "your_username"
.Config("http://schemas.microsoft.com/cdo/configuration/password") = "your_password"
.Config("http://schemas.microsoft.com/cdo/configuration/sendusing") = 2 ' cdoSendUsingPort
.Config("http://schemas.microsoft.com/cdo/configuration/sendusername") = "your_username"
.Config("http://schemas.microsoft.com/cdo/configuration/sendpassword") = "your_password"
End With
' Retrieve emails from the inbox
Set cdoInbox = cdoSession.GetItems()
For Each cdoItem In cdoInbox
Debug.Print cdoItem.Subject, cdoItem.TextBody
Next cdoItem
Set cdoItem = Nothing
Set cdoInbox = Nothing
Set cdoSession = Nothing
End Sub
Gallery of Access VBA Email Integration
Access VBA Email Integration Image Gallery
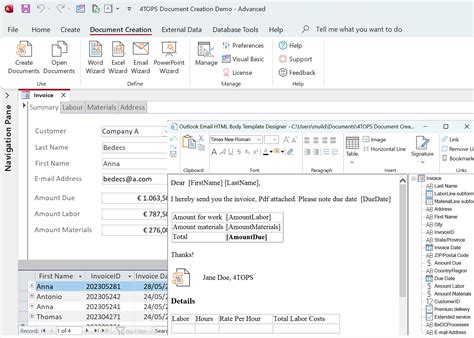
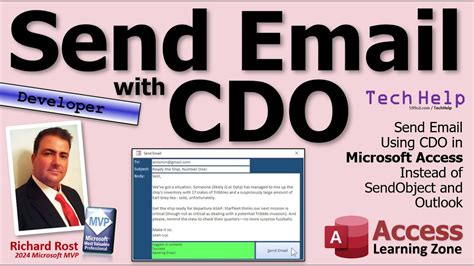
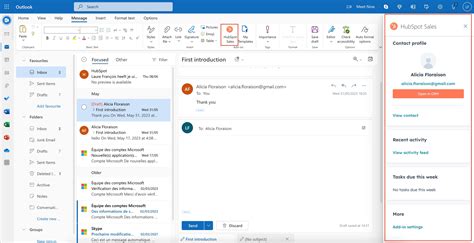
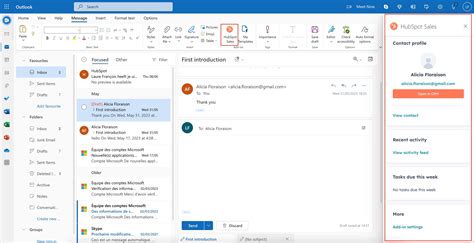
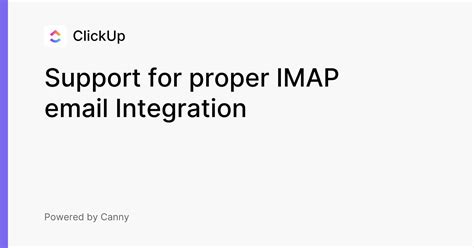
We hope you found this article helpful in understanding the different ways to read emails with Access VBA. Whether you're using Outlook, CDO, Winsock, Redemption, or IMAP, integrating email capabilities into your Access application can greatly enhance its functionality. Don't hesitate to experiment with these methods and adapt them to suit your specific needs. If you have any questions or need further clarification, please feel free to ask in the comments below.