Intro
Master VBA cell referencing with our expert guide. Discover 5 ways to refer to a cell in VBA, including Range, Cells, Offset, and more. Learn how to use absolute and relative references, and optimize your code with best practices. Improve your Excel automation skills and take your VBA coding to the next level.
When working with Excel VBA, one of the most fundamental concepts is referencing cells. There are multiple ways to refer to a cell in VBA, and the method you choose can greatly impact the efficiency and readability of your code. In this article, we'll explore five common ways to refer to a cell in VBA, along with examples and best practices.
Why Referencing Cells is Important
Before we dive into the different methods, it's essential to understand why referencing cells is crucial in VBA. When you write VBA code, you need to specify which cells or ranges you want to manipulate. The way you refer to cells can affect the performance, accuracy, and maintainability of your code. By choosing the right method, you can make your code more efficient, readable, and less prone to errors.
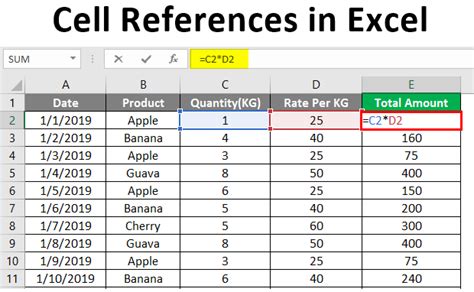
Method 1: Using the Range Object
The most common way to refer to a cell in VBA is by using the Range object. This method allows you to specify a cell or range by its address.
Sub ReferToCellRange()
Dim cell As Range
Set cell = Range("A1")
cell.Value = "Hello World"
End Sub
In this example, we set the value of cell A1 to "Hello World" using the Range object.
Method 2: Using the Cells Property
Another way to refer to a cell is by using the Cells property. This method allows you to specify a cell by its row and column numbers.
Sub ReferToCellCells()
Cells(1, 1).Value = "Hello World"
End Sub
In this example, we set the value of cell A1 to "Hello World" using the Cells property.
Method 3: Using the Offset Property
The Offset property allows you to refer to a cell by specifying an offset from a base cell.
Sub ReferToCellOffset()
Dim baseCell As Range
Set baseCell = Range("A1")
baseCell.Offset(1, 1).Value = "Hello World"
End Sub
In this example, we set the value of cell B2 to "Hello World" by offsetting one row and one column from cell A1.
Method 4: Using the Find Method
The Find method allows you to search for a cell that meets specific criteria, such as a value or format.
Sub ReferToCellFind()
Dim searchRange As Range
Set searchRange = Range("A1:A10")
Dim foundCell As Range
Set foundCell = searchRange.Find("Hello World")
If Not foundCell Is Nothing Then
foundCell.Value = "Found it!"
End If
End Sub
In this example, we search for a cell in range A1:A10 that contains the value "Hello World". If we find it, we set its value to "Found it!".
Method 5: Using the Evaluate Method
The Evaluate method allows you to evaluate a string as if it were a formula.
Sub ReferToCellEvaluate()
Dim formula As String
formula = "=A1*2"
Range("B1").Value = Evaluate(formula)
End Sub
In this example, we evaluate the formula "=A1*2" and set the value of cell B1 to the result.
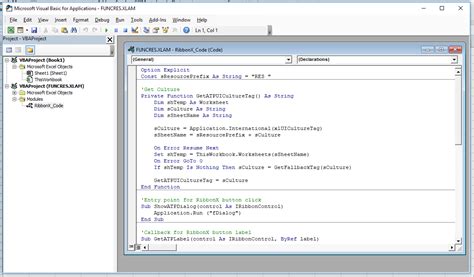
Best Practices
When referring to cells in VBA, keep the following best practices in mind:
- Use the Range object instead of the Cells property when possible.
- Avoid using the Select method, which can slow down your code.
- Use the Find method instead of looping through cells to find a specific value.
- Use the Evaluate method sparingly, as it can be slow and may not work as expected in all cases.
By following these best practices and choosing the right method for your needs, you can write more efficient, readable, and maintainable VBA code.
Gallery of Refer to Cell in VBA
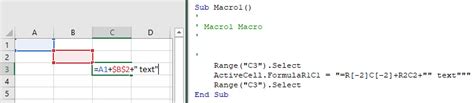
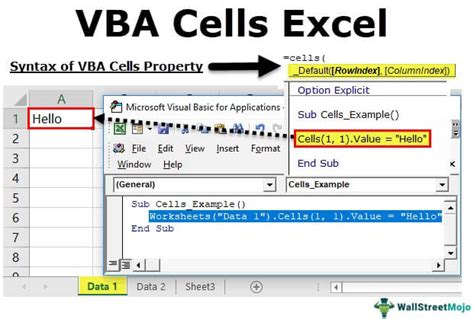
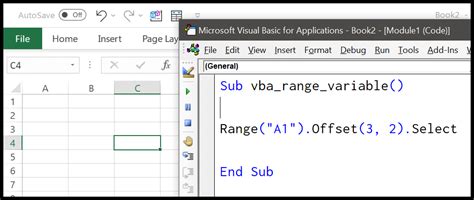
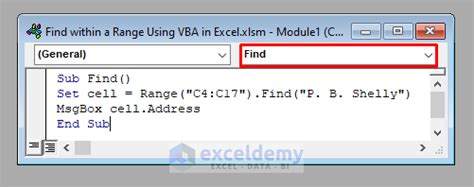
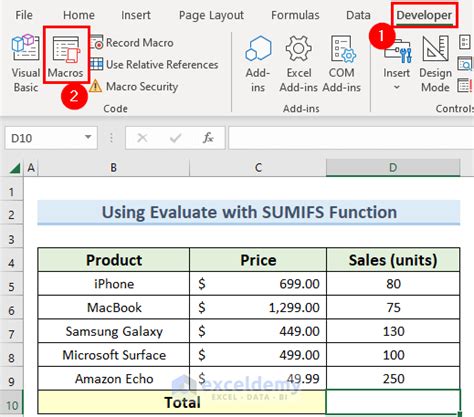
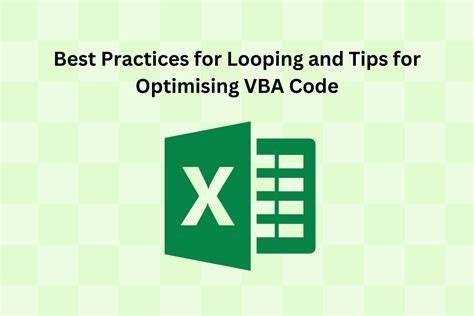
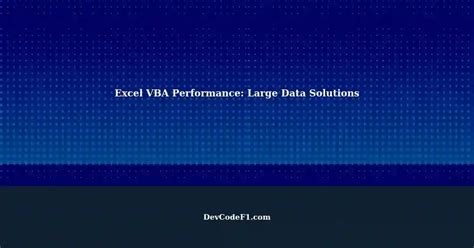
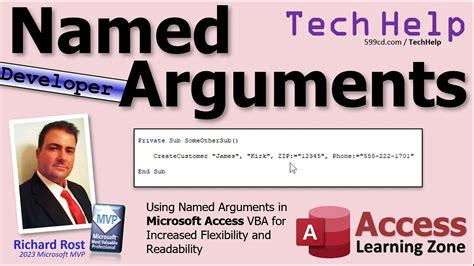
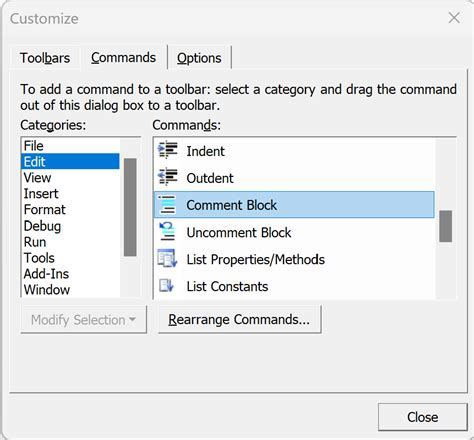
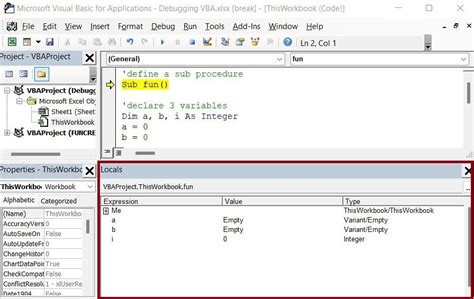
Conclusion
Referencing cells is a fundamental aspect of VBA programming. By understanding the different methods available, you can write more efficient, readable, and maintainable code. Remember to follow best practices, such as using the Range object instead of the Cells property, and avoiding the Select method. With practice and experience, you'll become proficient in referencing cells and writing effective VBA code.
We hope this article has been helpful in your VBA journey. Do you have any questions or need further clarification on any of the methods? Share your thoughts and experiences in the comments below!