Intro
Unlock the power of VBA with reference cells. Discover 5 ways to use reference cells in VBA to simplify your coding, improve accuracy, and boost productivity. Learn how to use absolute references, relative references, named ranges, and more to streamline your workflows and take your VBA skills to the next level.
Using reference cells in VBA can greatly enhance the efficiency and accuracy of your macros. Reference cells are cells in your worksheet that contain values or formulas that you want to use in your VBA code. By referencing these cells, you can create dynamic and flexible macros that can adapt to changing data. Here, we will explore five ways to use reference cells in VBA, along with examples and explanations to help you get started.
The Importance of Reference Cells in VBA
Before we dive into the five ways to use reference cells, it's essential to understand why they are important. Reference cells allow you to decouple your VBA code from specific values or formulas in your worksheet. This means that if the values or formulas change, your macro will automatically adapt to the new values. This makes your macros more robust and easier to maintain.
Way 1: Using Reference Cells to Input Values
One of the most common ways to use reference cells is to input values into your VBA code. For example, suppose you have a cell in your worksheet that contains the name of a file that you want to open. You can use a reference cell to read the value of that cell and use it in your VBA code.
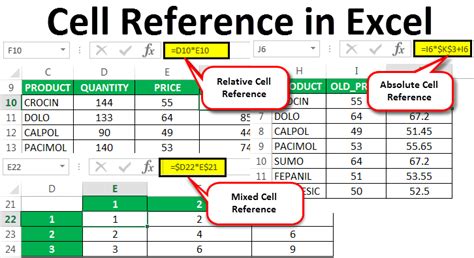
Here is an example of how you can use a reference cell to input a value:
Sub OpenFile()
Dim fileName As String
fileName = Range("A1").Value
Workbooks.Open fileName
End Sub
In this example, the value in cell A1 is used to specify the file name that should be opened.
Way 2: Using Reference Cells to Control Logic
Reference cells can also be used to control the logic of your VBA code. For example, suppose you have a cell that contains a value that determines whether a certain action should be taken. You can use a reference cell to read the value of that cell and use it to control the flow of your code.
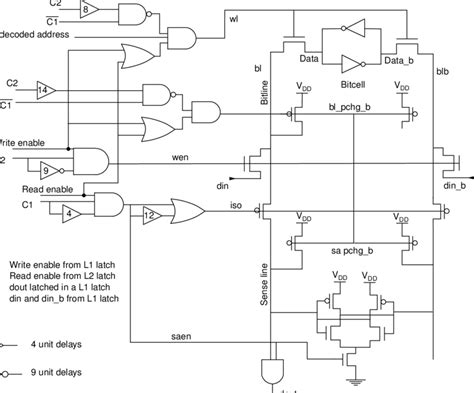
Here is an example of how you can use a reference cell to control logic:
Sub ProcessData()
Dim processFlag As Boolean
processFlag = Range("B1").Value
If processFlag Then
' Process data
Else
' Do not process data
End If
End Sub
In this example, the value in cell B1 is used to determine whether data should be processed.
Way 3: Using Reference Cells to Specify Ranges
Reference cells can also be used to specify ranges in your VBA code. For example, suppose you have a cell that contains the name of a range that you want to use in your code. You can use a reference cell to read the value of that cell and use it to specify the range.
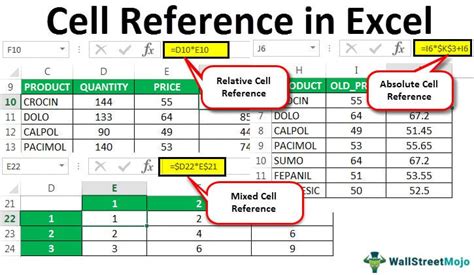
Here is an example of how you can use a reference cell to specify a range:
Sub ProcessRange()
Dim rangeName As String
rangeName = Range("C1").Value
Range(rangeName).Select
End Sub
In this example, the value in cell C1 is used to specify the name of the range that should be selected.
Way 4: Using Reference Cells to Calculate Formulas
Reference cells can also be used to calculate formulas in your VBA code. For example, suppose you have a cell that contains a formula that you want to use in your code. You can use a reference cell to read the value of that cell and use it to calculate the formula.
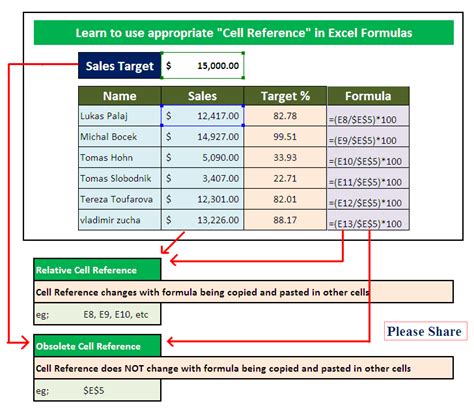
Here is an example of how you can use a reference cell to calculate a formula:
Sub CalculateFormula()
Dim formula As String
formula = Range("D1").Value
result = Evaluate(formula)
End Sub
In this example, the value in cell D1 is used to specify the formula that should be calculated.
Way 5: Using Reference Cells to Store Data
Finally, reference cells can be used to store data in your VBA code. For example, suppose you have a cell that contains a value that you want to store for later use. You can use a reference cell to read the value of that cell and store it in a variable.
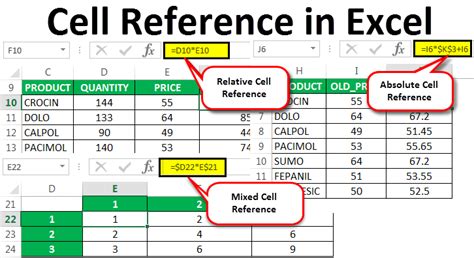
Here is an example of how you can use a reference cell to store data:
Sub StoreData()
Dim data As Variant
data = Range("E1").Value
' Use the stored data later
End Sub
In this example, the value in cell E1 is used to store data that can be used later in the code.
Gallery of Reference Cells in VBA
Reference Cells in VBA Image Gallery
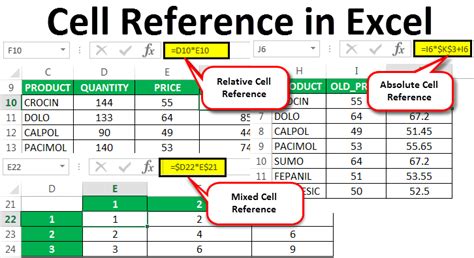
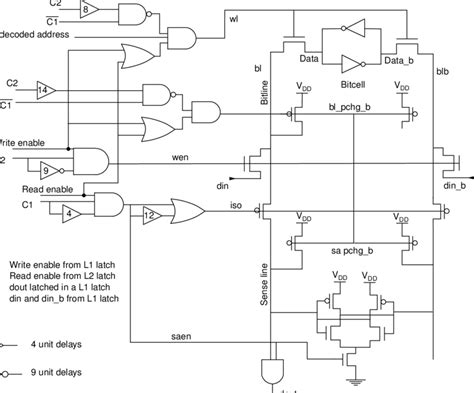
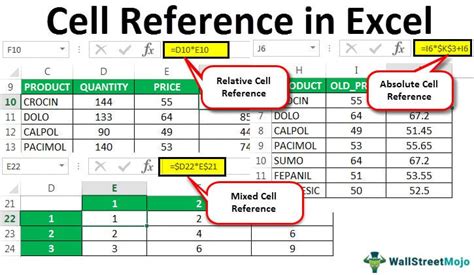
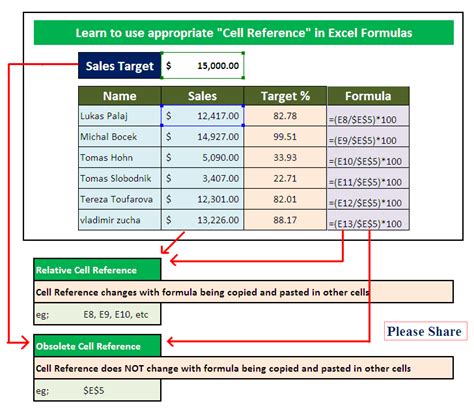
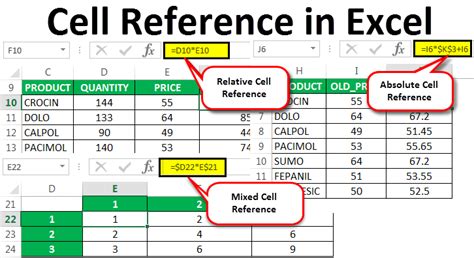
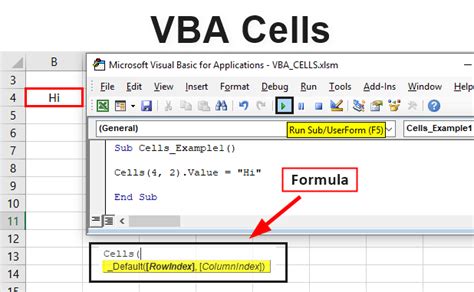
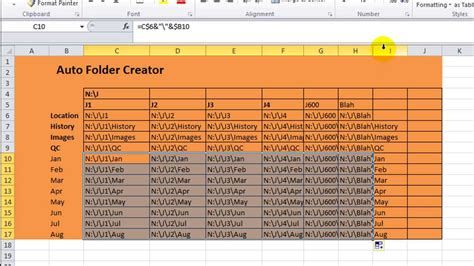
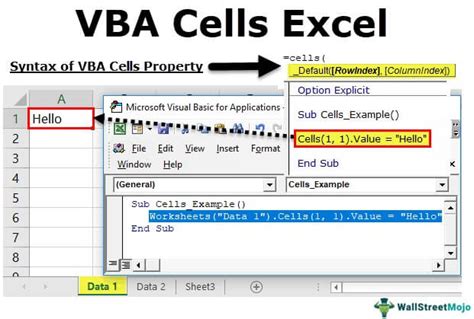
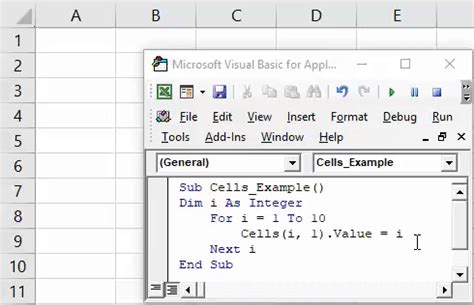
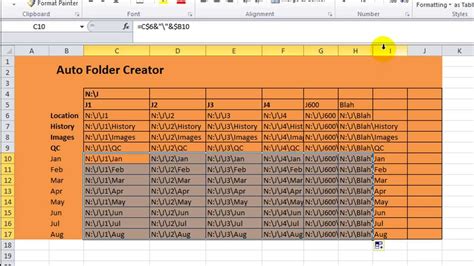
Conclusion
Reference cells are a powerful tool in VBA that can help you create dynamic and flexible macros. By using reference cells, you can decouple your VBA code from specific values or formulas in your worksheet, making it easier to maintain and update your macros. In this article, we have explored five ways to use reference cells in VBA, including using reference cells to input values, control logic, specify ranges, calculate formulas, and store data. We hope that this article has provided you with a comprehensive understanding of how to use reference cells in VBA and has inspired you to start using them in your own projects.
We encourage you to share your own experiences and tips for using reference cells in VBA in the comments section below. If you have any questions or need further clarification on any of the topics covered in this article, please don't hesitate to ask.