Intro
Refresh Pivot Table Excel VBA Made Easy: Discover how to automate pivot table updates with Excel VBA. Learn to refresh pivot tables with a single click, eliminate manual updates, and boost productivity. Master VBA coding for pivot table refresh, including pivot cache, data source, and worksheet interactions, and simplify your Excel workflow.
Pivot tables are a powerful tool in Excel that allows users to summarize, analyze, and visualize large datasets. However, working with pivot tables can be intimidating, especially for those who are new to Excel or VBA programming. In this article, we will explore how to refresh pivot tables in Excel using VBA, making it easy for you to automate and streamline your workflow.
Why Refresh Pivot Tables?
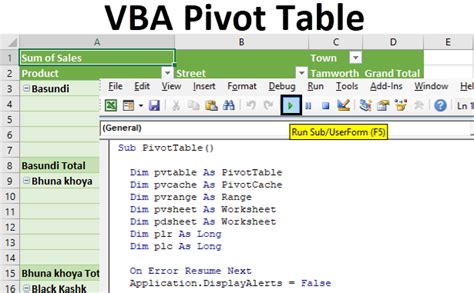
Before we dive into the world of VBA, let's quickly discuss why refreshing pivot tables is important. Pivot tables rely on data to generate summaries and insights. When the underlying data changes, the pivot table needs to be refreshed to reflect the new information. This is where VBA comes in – by automating the refresh process, you can save time and ensure that your pivot tables are always up-to-date.
Understanding VBA Basics
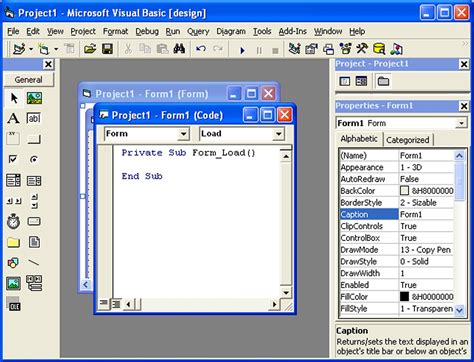
Before we start writing code, let's cover some basic VBA concepts. VBA stands for Visual Basic for Applications, which is a programming language used to create and automate tasks in Microsoft Office applications, including Excel. To access the VBA editor in Excel, press Alt + F11
or navigate to Developer
> Visual Basic
in the ribbon.
VBA Syntax and Structure
VBA code consists of modules, procedures, and variables. A module is a container that holds VBA code, while a procedure is a block of code that performs a specific task. Variables are used to store and manipulate data.
Refreshing Pivot Tables with VBA
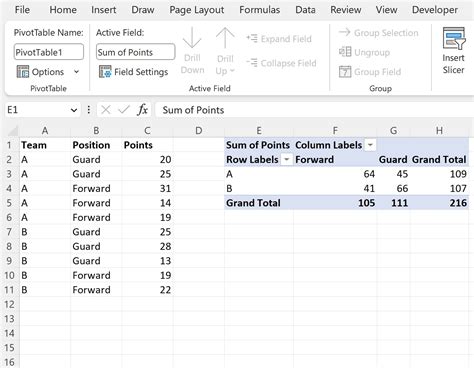
Now that we have a basic understanding of VBA, let's move on to refreshing pivot tables. The following code snippet demonstrates how to refresh a pivot table using VBA:
Sub RefreshPivotTable()
Dim pt As PivotTable
Set pt = ThisWorkbook.Sheets("YourSheetName").PivotTables("YourPivotTableName")
pt.RefreshTable
End Sub
In this example, we define a subroutine called RefreshPivotTable
that takes no arguments. We then declare a variable pt
as a PivotTable
object and set it to the pivot table we want to refresh. Finally, we call the RefreshTable
method to update the pivot table.
Refreshing Multiple Pivot Tables
If you have multiple pivot tables in your workbook, you can refresh them all at once using a loop:
Sub RefreshAllPivotTables()
Dim ws As Worksheet
Dim pt As PivotTable
For Each ws In ThisWorkbook.Worksheets
For Each pt In ws.PivotTables
pt.RefreshTable
Next pt
Next ws
End Sub
This code loops through all worksheets in the workbook and refreshes each pivot table it finds.
Automating Pivot Table Refresh
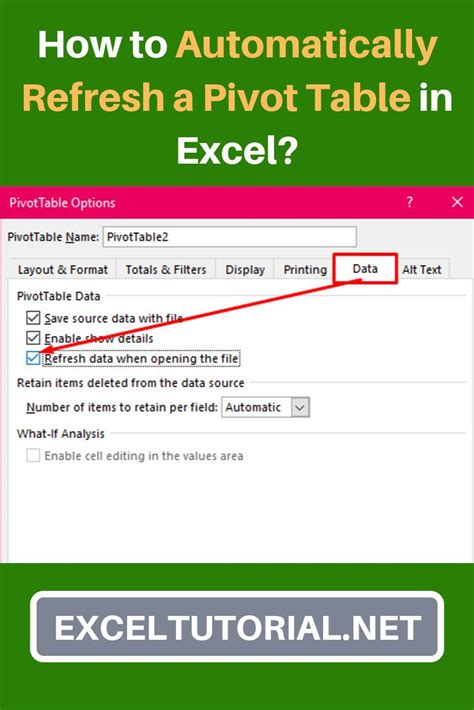
While manually running a VBA script can be convenient, you can also automate the pivot table refresh process using Excel's built-in features.
Using the Workbook_Open Event
You can use the Workbook_Open
event to refresh pivot tables automatically when the workbook is opened:
Private Sub Workbook_Open()
RefreshAllPivotTables
End Sub
This code calls the RefreshAllPivotTables
subroutine we defined earlier when the workbook is opened.
Using a Scheduled Task
Alternatively, you can use Excel's built-in scheduling feature to refresh pivot tables at regular intervals:
Sub RefreshPivotTablesOnSchedule()
Application.OnTime TimeValue("14:00:00"), "RefreshAllPivotTables"
End Sub
This code schedules the RefreshAllPivotTables
subroutine to run at 2:00 PM every day.
Gallery of Pivot Table Refresh
Pivot Table Refresh Images
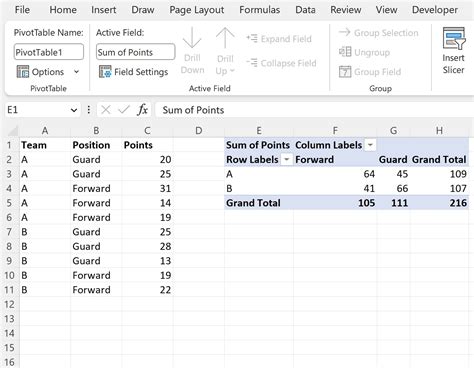
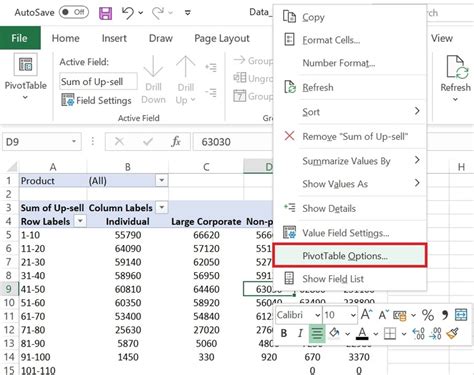
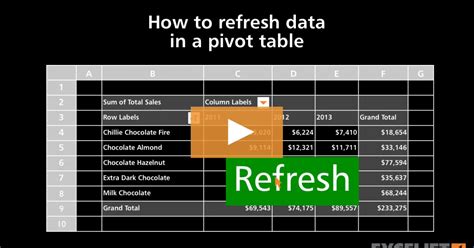
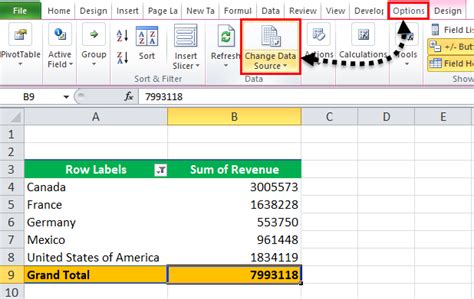
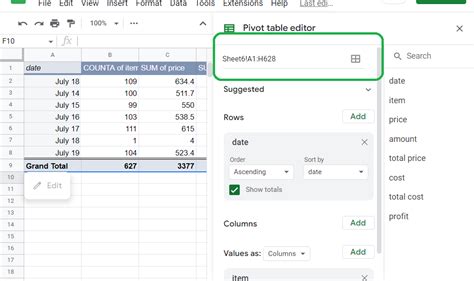
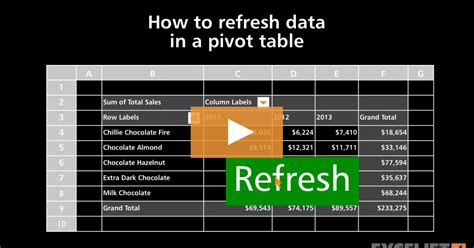
We hope this article has helped you understand how to refresh pivot tables in Excel using VBA. By automating this process, you can save time and ensure that your pivot tables are always up-to-date. Share your experiences and tips in the comments below!
FAQs
Q: How do I access the VBA editor in Excel?
A: Press Alt + F11
or navigate to Developer
> Visual Basic
in the ribbon.
Q: What is the syntax for refreshing a pivot table in VBA?
A: pt.RefreshTable
Q: How can I automate the pivot table refresh process?
A: Use the Workbook_Open
event or schedule a task using Excel's built-in features.
Q: Can I refresh multiple pivot tables at once? A: Yes, use a loop to iterate through all pivot tables in the workbook.
We hope this article has been helpful. If you have any further questions or need more assistance, feel free to ask!