Intro
Learn how to efficiently remove items from collections in VBA with these 3 expert-approved methods. Discover the best practices for deleting items, handling errors, and optimizing performance. Master the art of VBA collection management and improve your coding skills with these actionable tips and tricks.
In the world of Visual Basic for Applications (VBA), managing collections is an essential skill for any developer. Collections in VBA are a type of data structure that allows you to store and manipulate a series of items. Removing an item from a collection can be done in several ways, each with its own specific use case and considerations. Here's a detailed guide on how to remove an item from a collection in VBA, focusing on three primary methods.
Understanding Collections in VBA
Before diving into how to remove items, it's crucial to understand what collections are and how they work. A collection in VBA is similar to an array but offers more flexibility and is dynamically sized. You can think of a collection as a container that can hold a series of objects or values. Unlike arrays, you don't need to specify the size of a collection beforehand, and you can add or remove items dynamically.
Method 1: Using the Remove Method
The most straightforward way to remove an item from a collection is by using the Remove
method. This method requires you to specify the index or key of the item you wish to remove.
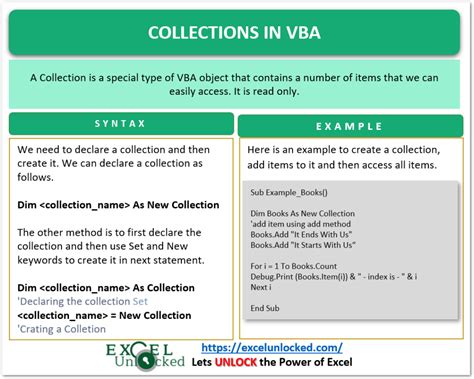
Here's an example of how you might use the Remove
method:
Sub RemoveItemFromCollection()
Dim myCollection As New Collection
myCollection.Add "Item 1", "Key1"
myCollection.Add "Item 2", "Key2"
myCollection.Add "Item 3", "Key3"
' Remove an item by its index
myCollection.Remove 2
' Or remove an item by its key
' myCollection.Remove "Key2"
' Iterate through the collection to see the result
For Each item In myCollection
Debug.Print item
Next item
End Sub
Method 2: Using a Loop to Find and Remove an Item
Sometimes, you might not know the index or key of the item you want to remove, but you know a unique property or value that identifies it. In such cases, you can loop through the collection to find the item and then remove it.
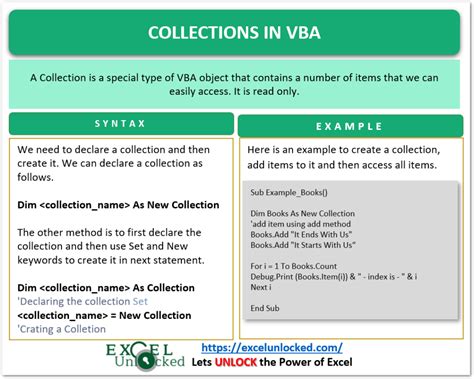
Here's an example:
Sub RemoveItemUsingLoop()
Dim myCollection As New Collection
myCollection.Add "Item 1"
myCollection.Add "Item 2"
myCollection.Add "Item 3"
Dim i As Long
For i = myCollection.Count To 1 Step -1
If myCollection(i) = "Item 2" Then
myCollection.Remove i
Exit For
End If
Next i
' Iterate through the collection to see the result
For Each item In myCollection
Debug.Print item
Next item
End Sub
Method 3: Creating a New Collection Without the Item
Another approach to removing an item from a collection is to create a new collection that excludes the item you want to remove. This method involves looping through the original collection and adding all items except the one you want to remove to a new collection.
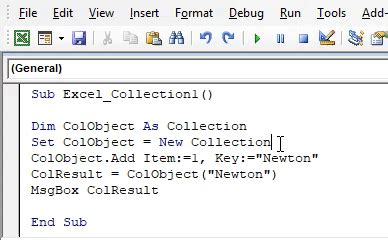
Here's an example of how you can do this:
Sub CreateNewCollectionWithoutItem()
Dim myOriginalCollection As New Collection
myOriginalCollection.Add "Item 1"
myOriginalCollection.Add "Item 2"
myOriginalCollection.Add "Item 3"
Dim myNewCollection As New Collection
Dim item As Variant
For Each item In myOriginalCollection
If item <> "Item 2" Then
myNewCollection.Add item
End If
Next item
' Now myNewCollection contains all items except "Item 2"
' Iterate through the new collection to see the result
For Each item In myNewCollection
Debug.Print item
Next item
End Sub
Gallery of Collection Operations in VBA
VBA Collection Operations Gallery
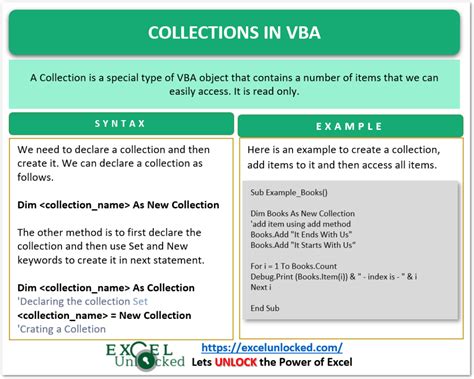
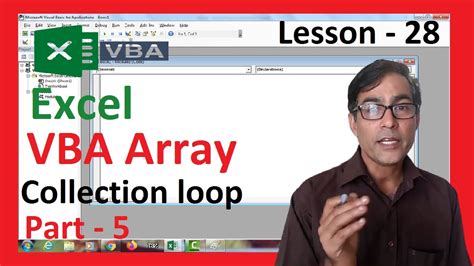
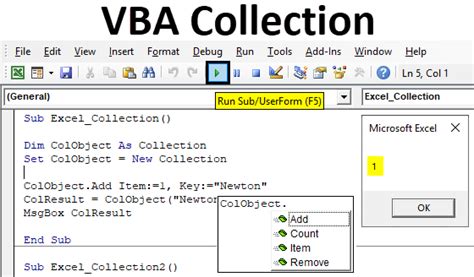
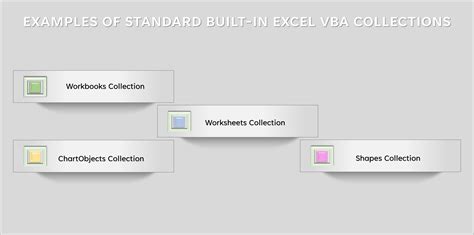
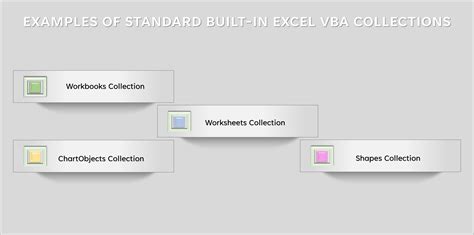
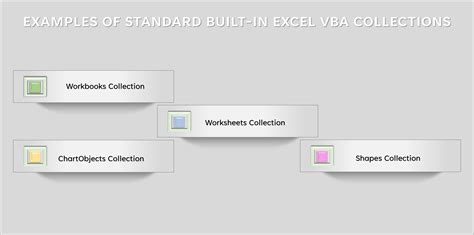
Conclusion
Removing items from a collection in VBA is a fundamental skill that can be achieved through various methods, each with its own strengths and use cases. Whether you're using the Remove
method directly, looping through the collection to find and remove an item, or creating a new collection without the item, understanding these methods will enhance your VBA programming capabilities. By mastering these techniques, you'll be better equipped to manage and manipulate collections in your VBA projects, making your code more efficient and effective.