Intro
When it comes to working with arrays in VBA, one of the most common challenges developers face is returning arrays from functions. Unlike many other programming languages, VBA doesn't directly support the return of arrays from functions in the classical sense. However, with a few clever workarounds and best practices, you can achieve similar functionality and make your VBA coding experience easier and more efficient.
Understanding VBA Arrays
Before diving into how to return arrays from functions, it's crucial to understand the basics of VBA arrays. Arrays in VBA are variables that can hold multiple values. They are defined using the Dim
statement, and their size can be fixed or dynamic. Arrays are powerful tools for storing and manipulating data, but their use within functions requires careful consideration.
The Problem with Returning Arrays
The main issue with returning arrays from VBA functions is that the function must know the size of the array it's going to return. This presents a problem when the size of the array is determined within the function or is dynamic. Furthermore, if the function doesn't execute correctly or reaches an error, handling the returned array can become complicated.
Workaround: Using Variant Data Type
One common workaround for returning arrays from VBA functions is to use the Variant data type. A Variant can hold any type of data, including arrays, making it a flexible solution for returning arrays of varying sizes.
Function ReturnArray() As Variant
Dim myArray() As Variant
ReDim myArray(1 To 10) 'Dynamic sizing
'Populate the array
For i = 1 To 10
myArray(i) = i
Next i
'Return the array
ReturnArray = myArray
End Function
Workaround: Using a Collection or Dictionary
Another approach is to use a Collection or Dictionary object, which can be returned from a function more easily than an array. Collections and Dictionaries are useful for storing data that doesn't need to be in a specific order or when the number of items is dynamic.
Function ReturnCollection() As Collection
Dim myCollection As New Collection
'Populate the collection
For i = 1 To 10
myCollection.Add i
Next i
'Return the collection
Set ReturnCollection = myCollection
End Function
Best Practices for Returning Arrays
- Use Variants Wisely: While Variants offer flexibility, they can make your code harder to understand and debug. Use them when necessary but prefer strongly typed variables when possible.
- Error Handling: Always implement robust error handling within your functions, especially when dealing with arrays or collections to avoid runtime errors.
- Document Your Code: Clearly document the functions that return arrays or collections, including what the function does, what it returns, and how to handle the returned data.
Gallery of VBA Array Functions
VBA Array Functions Gallery
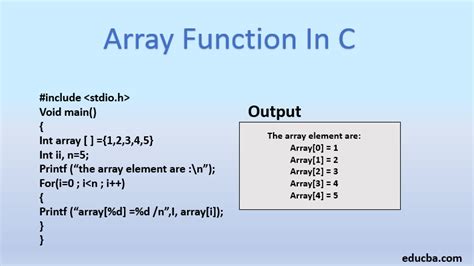
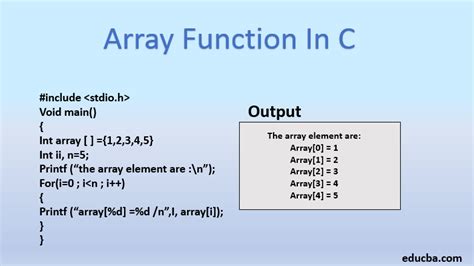
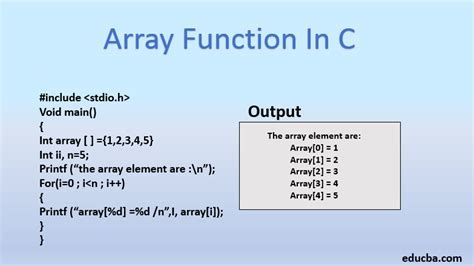

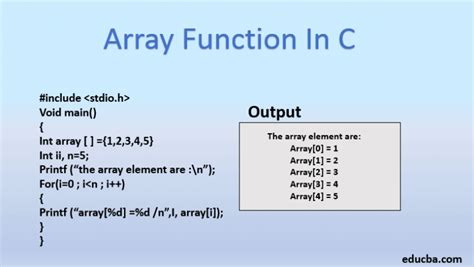
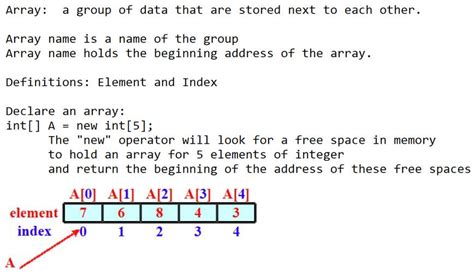
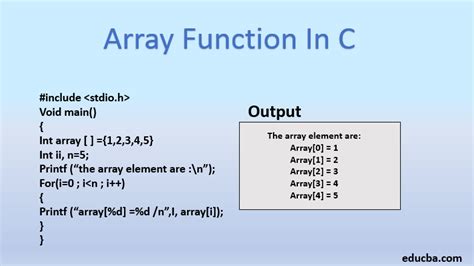
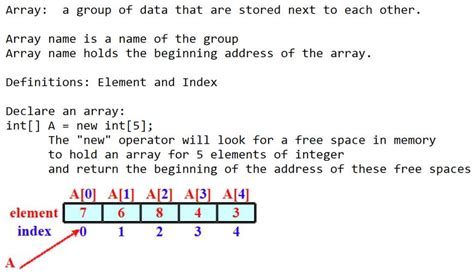
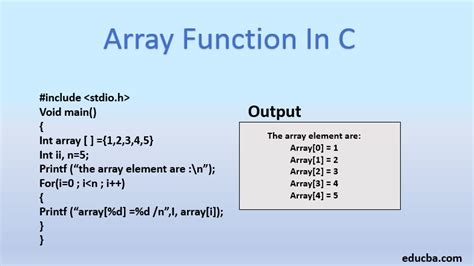
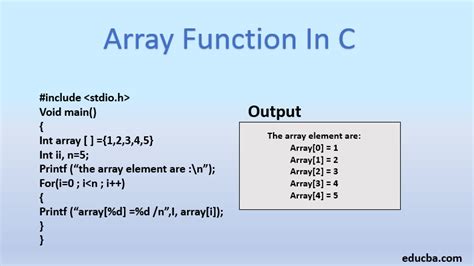
Conclusion: Mastering Array Functions in VBA
Returning arrays from VBA functions requires a bit of creativity due to the limitations in VBA. By understanding how to use Variants, Collections, and Dictionaries effectively, you can write robust and flexible code that meets your needs. Remember to follow best practices, such as error handling and clear documentation, to ensure your array functions are both powerful and maintainable. With practice and patience, you'll become proficient in using array functions to simplify your VBA projects and improve their efficiency.