Intro
Unlock the power of VBA with the Right function! Discover 5 practical ways to use this versatile function in your Excel macros, including extracting substrings, formatting data, and more. Learn expert tips and tricks to boost your VBA skills and streamline your workflow with this essential guide to using Right in VBA programming.
The Right function in VBA is a powerful tool that allows users to extract a specified number of characters from the right side of a string. This function is often used in data manipulation and analysis tasks, such as extracting dates, times, or specific codes from text strings. In this article, we will explore five ways to use the Right function in VBA, along with examples and practical applications.
Understanding the Right Function
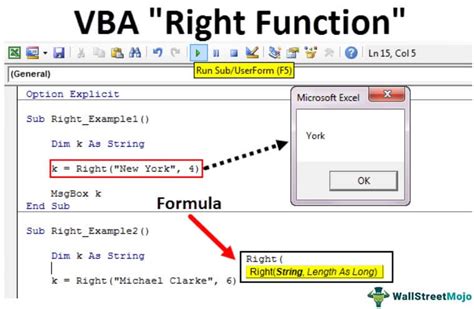
The Right function in VBA takes two arguments: the string from which to extract characters, and the number of characters to extract. The syntax for the Right function is as follows:
Right(string, length)
Where:
- string is the text string from which to extract characters.
- length is the number of characters to extract from the right side of the string.
Example 1: Extracting a Fixed Number of Characters
Suppose we have a text string that contains a date in the format "mm/dd/yyyy". We can use the Right function to extract the last four characters of the string, which represent the year.
Dim dateString As String
dateString = "02/12/2022"
Dim year As String
year = Right(dateString, 4)
Debug.Print year ' Output: 2022
In this example, the Right function extracts the last four characters of the date string, which represent the year.
Extracting Variable-Length Strings
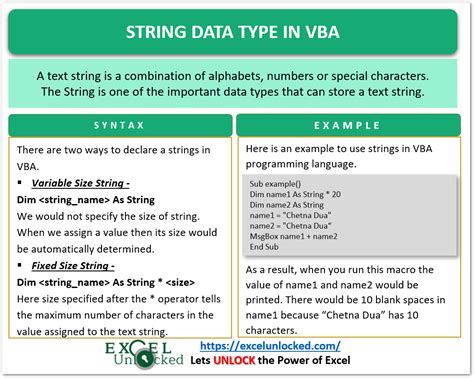
Sometimes, we may need to extract a variable number of characters from the right side of a string. We can use the Len function to determine the length of the string and then pass that value to the Right function.
Dim variableString As String
variableString = "Hello World"
Dim length As Integer
length = Len(variableString)
Dim extractedString As String
extractedString = Right(variableString, length - 5)
Debug.Print extractedString ' Output: World
In this example, the Len function determines the length of the variable string, and then the Right function extracts all characters except the first five.
Example 3: Extracting a Substring from a Delimited String
Suppose we have a string that contains multiple substrings separated by a delimiter, such as a comma or semicolon. We can use the Right function to extract the last substring.
Dim delimitedString As String
delimitedString = "apple,banana,cherry"
Dim delimiter As String
delimiter = ","
Dim lastSubstring As String
lastSubstring = Right(delimitedString, Len(delimitedString) - InStrRev(delimitedString, delimiter))
Debug.Print lastSubstring ' Output: cherry
In this example, the InStrRev function finds the position of the last delimiter, and then the Right function extracts the substring to the right of that delimiter.
Using the Right Function with Dates and Times
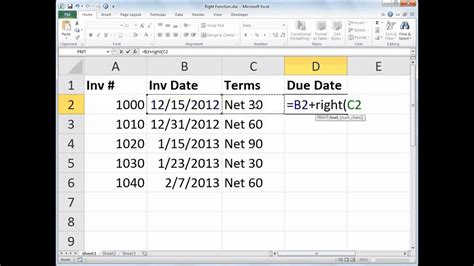
The Right function can be useful when working with dates and times. For example, we can use it to extract the time component from a date-time string.
Dim dateTimeString As String
dateTimeString = "2022-02-12 14:30:00"
Dim timeComponent As String
timeComponent = Right(dateTimeString, 8)
Debug.Print timeComponent ' Output: 14:30:00
In this example, the Right function extracts the last eight characters of the date-time string, which represent the time component.
Example 5: Extracting a Code from a Text String
Suppose we have a text string that contains a code in the format "XXXX-XXXX-XXXX-XXXX". We can use the Right function to extract the last four characters of the code.
Dim codeString As String
codeString = "1234-5678-9012-3456"
Dim lastFourDigits As String
lastFourDigits = Right(codeString, 4)
Debug.Print lastFourDigits ' Output: 3456
In this example, the Right function extracts the last four characters of the code string.
Gallery of Right Function Examples
Right Function Examples
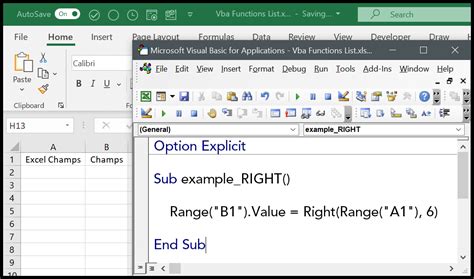
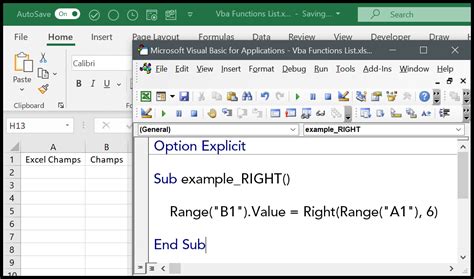
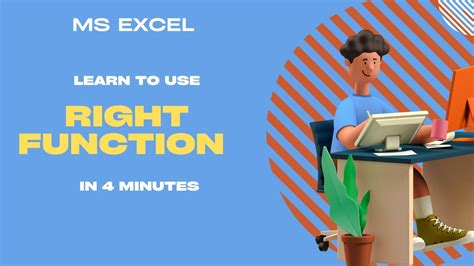
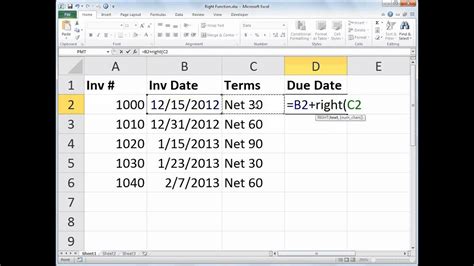
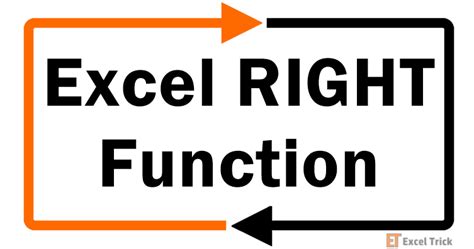
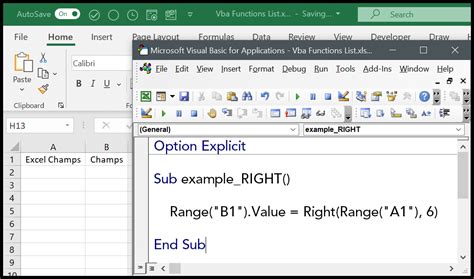
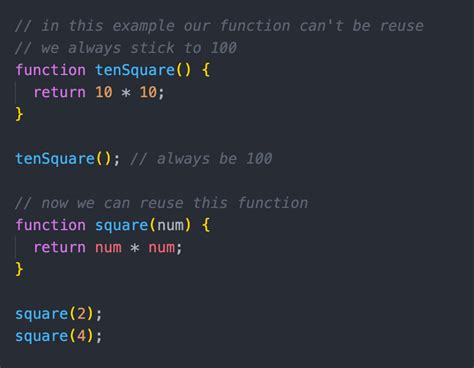
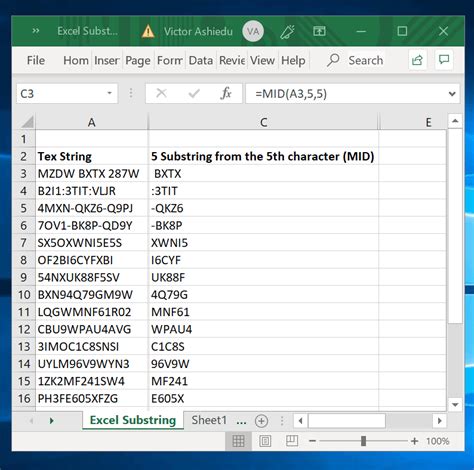
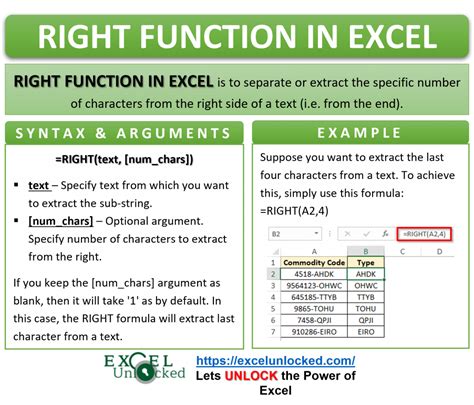
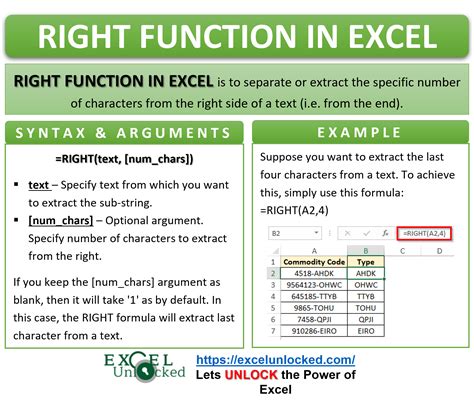
We hope this article has provided you with a comprehensive understanding of the Right function in VBA and its various applications. Whether you're working with dates, times, codes, or text strings, the Right function can be a powerful tool in your VBA toolkit. We encourage you to try out the examples and experiment with different scenarios to get the most out of this versatile function.
If you have any questions or would like to share your own experiences with the Right function, please leave a comment below. We'd love to hear from you!