Intro
Discover 5 efficient ways to get row count in VBA, improving your Excel automation skills. Learn how to use VBA properties, functions, and methods to accurately count rows in your worksheets. Boost productivity with these expert-approved techniques, covering Range.Find, Worksheet.Rows.Count, and more.
Getting the row count in VBA is a crucial task, especially when working with large datasets. Knowing the number of rows in a worksheet or range can help you make informed decisions, such as looping through data, creating charts, or performing data analysis. In this article, we'll explore five ways to get the row count in VBA.
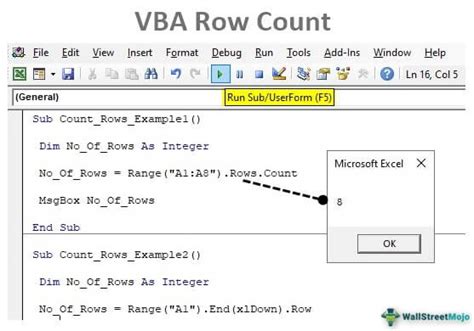
Why Get Row Count in VBA?
Before we dive into the methods, let's discuss why getting the row count is important. Here are a few reasons:
- Looping through data: When working with large datasets, you may need to loop through each row to perform tasks such as data validation, formatting, or calculations.
- Creating charts: Knowing the number of rows can help you create charts with the correct data range.
- Data analysis: Row count can help you perform statistical analysis, such as calculating averages, sums, or standard deviations.
Method 1: Using the Rows.Count
Property
The simplest way to get the row count is by using the Rows.Count
property. This method returns the total number of rows in a worksheet or range.
Sub GetRowCountUsingRowsCount()
Dim rowCount As Long
rowCount = ActiveSheet.Rows.Count
MsgBox "Row Count: " & rowCount
End Sub
Method 2: Using the Range.Rows.Count
Property
Another way to get the row count is by using the Range.Rows.Count
property. This method returns the number of rows in a specific range.
Sub GetRowCountUsingRangeRowsCount()
Dim rowCount As Long
rowCount = Range("A1:A100").Rows.Count
MsgBox "Row Count: " & rowCount
End Sub
Method 3: Using the Worksheet.UsedRange.Rows.Count
Property
This method returns the number of rows in the used range of a worksheet. The used range is the range of cells that contains data or formatting.
Sub GetRowCountUsingUsedRangeRowsCount()
Dim rowCount As Long
rowCount = ActiveSheet.UsedRange.Rows.Count
MsgBox "Row Count: " & rowCount
End Sub
Method 4: Using the Application.CountA
Function
This method uses the Application.CountA
function to count the number of non-blank cells in a column. Since Excel stores data in rows, this method can be used to get the row count.
Sub GetRowCountUsingCountA()
Dim rowCount As Long
rowCount = Application.CountA(ActiveSheet.Range("A:A"))
MsgBox "Row Count: " & rowCount
End Sub
Method 5: Using the Application.WorksheetFunction.CountA
Function
This method is similar to Method 4, but it uses the Application.WorksheetFunction.CountA
function instead.
Sub GetRowCountUsingWorksheetFunctionCountA()
Dim rowCount As Long
rowCount = Application.WorksheetFunction.CountA(ActiveSheet.Range("A:A"))
MsgBox "Row Count: " & rowCount
End Sub
Gallery of VBA Row Count Methods
VBA Row Count Methods Gallery
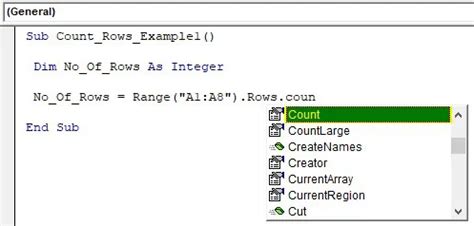
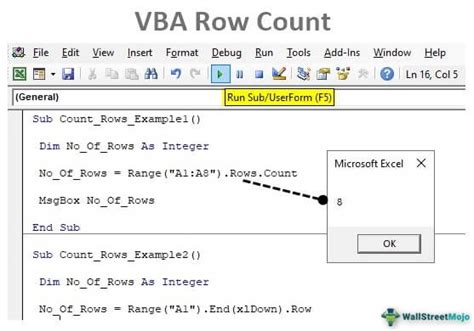
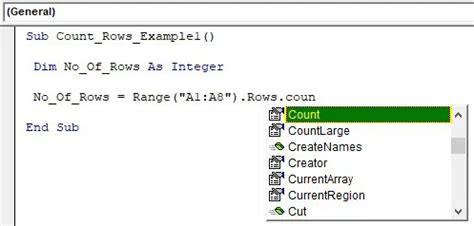
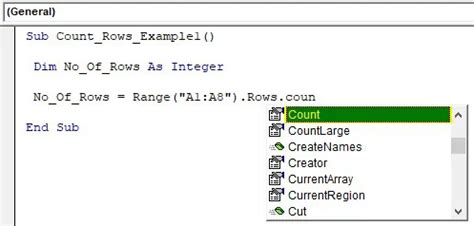
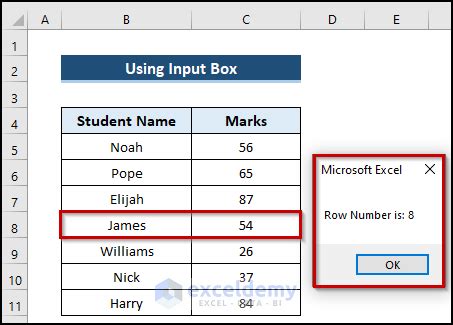
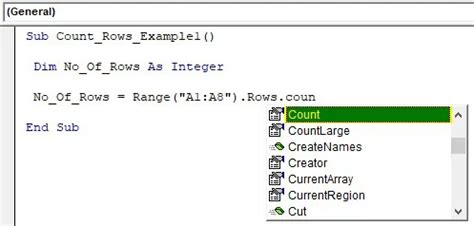
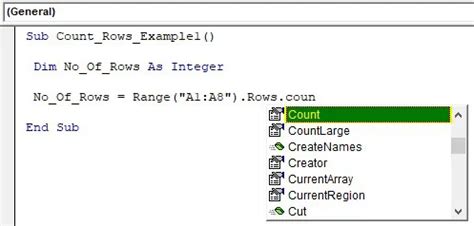
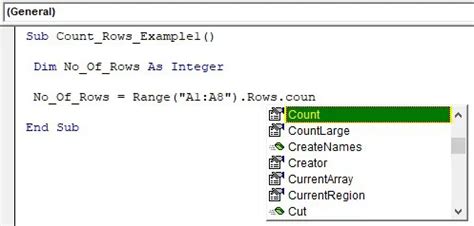
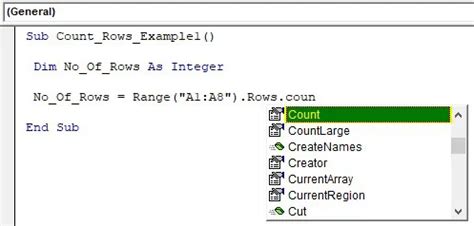
Conclusion
In this article, we've explored five ways to get the row count in VBA. Each method has its own advantages and disadvantages, and the choice of method depends on the specific use case. By using the correct method, you can efficiently get the row count and perform tasks such as looping through data, creating charts, or performing data analysis.
Share Your Thoughts
Have you used any of these methods to get the row count in VBA? Do you have any other methods to share? Please leave a comment below and let's discuss!