Intro
Resolve Runtime Error 424 Object Required with these 6 expert-approved fixes. Discover the causes of this VBA error, and learn how to debug and repair your code with ease. From object referencing to syntax correction, get step-by-step solutions to overcome this common programming hurdle and ensure seamless code execution.
Runtime Error 424 is a common error encountered by users of Visual Basic for Applications (VBA) and Visual Basic (VB) 6.0. This error occurs when an object is not properly referenced or is missing in the code, leading to the program's inability to identify the object. If you're a programmer or a user who frequently interacts with VBA or VB 6.0 applications, understanding how to fix Runtime Error 424 can be incredibly beneficial.
Understanding the Cause of Runtime Error 424
Before diving into the solutions, it's essential to understand why Runtime Error 424 occurs. This error typically happens when:
- An object variable is not properly initialized.
- An object is not set to a valid object reference.
- The code tries to access an object that doesn't exist.
Solution 1: Ensure Proper Initialization
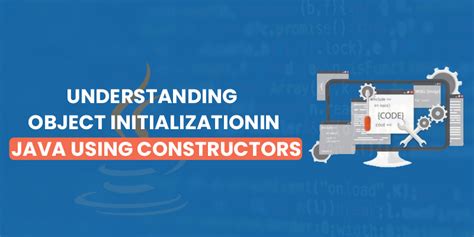
One of the most common reasons for Runtime Error 424 is the failure to properly initialize an object variable before using it. To fix this:
- Declare the object variable using the
Dim
keyword. - Use the
Set
keyword to assign the object variable to a valid object reference.
For example:
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
Solution 2: Verify Object Existence
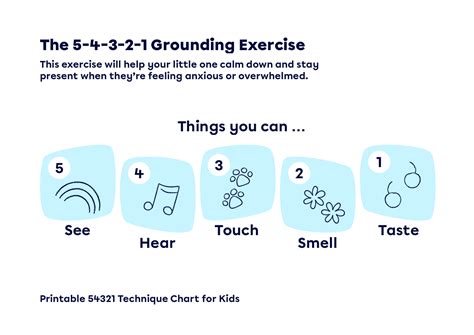
Sometimes, Runtime Error 424 occurs because the code tries to access an object that doesn't exist. To avoid this:
- Before referencing an object, check if it exists using the
If
statement. - Use the
On Error Resume Next
statement to handle situations where the object might not exist.
Example:
On Error Resume Next
If ThisWorkbook.Worksheets("Sheet1") Is Nothing Then
MsgBox "The worksheet does not exist."
Else
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
End If
On Error GoTo 0
Solution 3: Avoid Null Object References
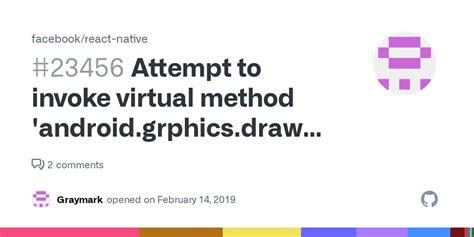
Make sure that the object reference is not null before trying to access its properties or methods. You can do this by checking if the object is not Nothing
:
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
If Not ws Is Nothing Then
' Proceed with the code
End If
Solution 4: Check for Type Mismatch
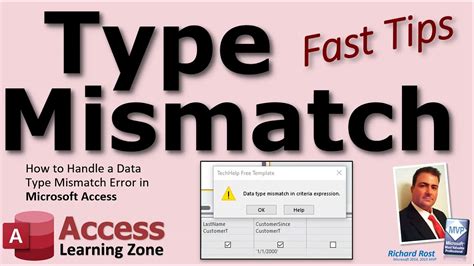
A type mismatch can also lead to Runtime Error 424. Ensure that the object variable is declared with the correct type to match the object it will be referencing:
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1") ' Correct type
Solution 5: Late Binding vs. Early Binding
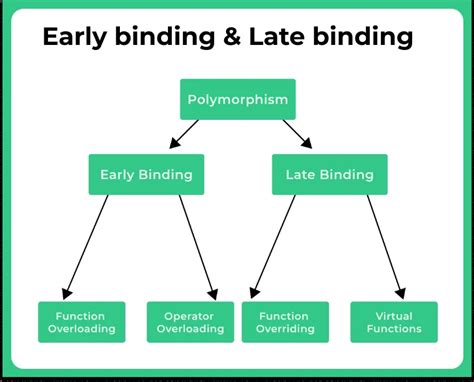
- Late Binding: Declaring an object variable as
Object
instead of a specific type can sometimes lead to Runtime Error 424 if the object doesn't support the method or property being accessed. - Early Binding: Using early binding (specifying the object type) can help catch errors at compile-time rather than runtime.
Dim ws As Object ' Late binding
Set ws = ThisWorkbook.Worksheets("Sheet1")
vs.
Dim ws As Worksheet ' Early binding
Set ws = ThisWorkbook.Worksheets("Sheet1")
Solution 6: Debugging Your Code
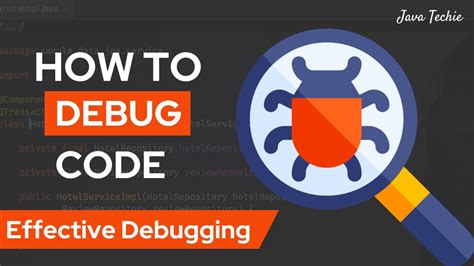
Lastly, good debugging practices can help identify where Runtime Error 424 is coming from:
- Use
MsgBox
statements to check variable values at different points in your code. - Utilize the Immediate Window in the Visual Basic Editor to test expressions and check object properties.
Gallery of Related Images
Images for Troubleshooting Runtime Error 424
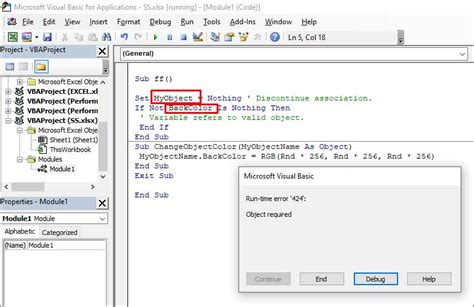
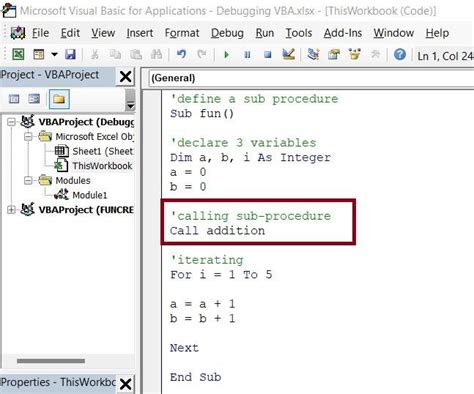
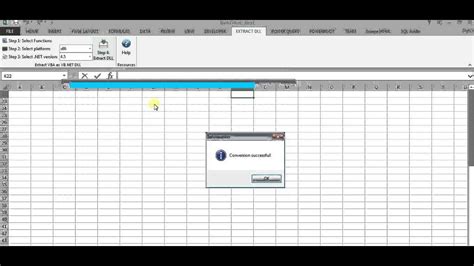
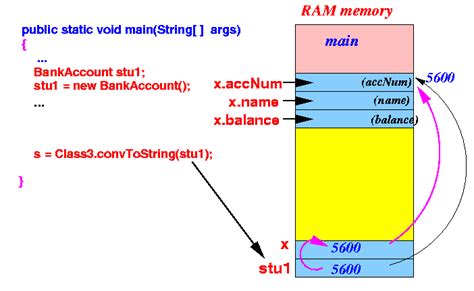
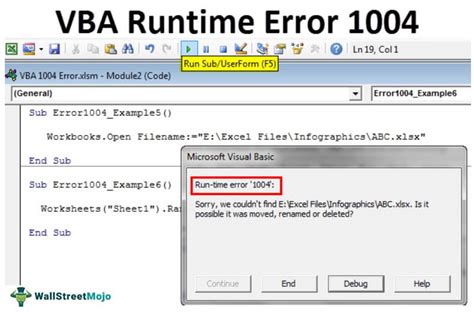
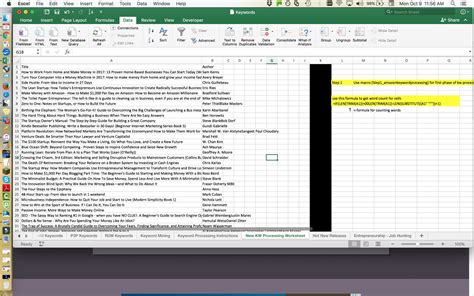
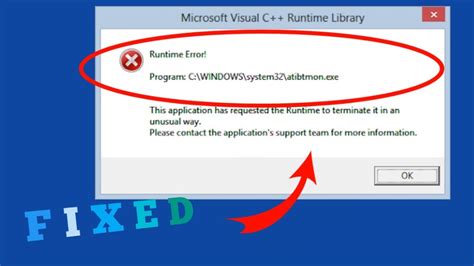
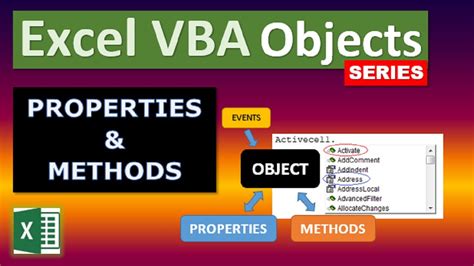
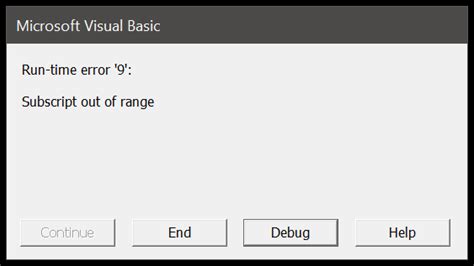
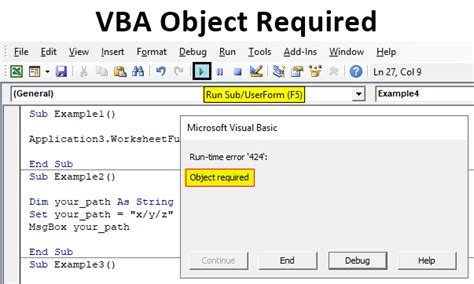
We hope this comprehensive guide has helped you understand and resolve Runtime Error 424 in VBA. Remember to always debug your code thoroughly and check for any type mismatches or null object references. If you have any questions or need further clarification, feel free to ask in the comments below!