Working with multiple worksheets in Excel can be a challenge, especially when you're trying to automate tasks using VBA. One common issue that many users face is setting the active worksheet in VBA. In this article, we'll explore five ways to set the active worksheet in VBA, along with examples and explanations to help you master this skill.
Why is it important to set the active worksheet in VBA?
When working with multiple worksheets in Excel, it's essential to specify which worksheet you want to perform actions on. If you don't set the active worksheet, VBA may perform actions on the wrong worksheet, leading to errors or unexpected results. By setting the active worksheet, you can ensure that your VBA code executes correctly and efficiently.
Method 1: Using the ActiveSheet
Property
The ActiveSheet
property is the most common way to set the active worksheet in VBA. This property returns the currently active worksheet, allowing you to perform actions on it.
Sub SetActiveSheet()
Worksheets("Sheet1").Activate
MsgBox "Sheet1 is now the active worksheet"
End Sub
In this example, the Worksheets("Sheet1").Activate
statement sets "Sheet1" as the active worksheet. The MsgBox
statement then displays a message confirming that "Sheet1" is the active worksheet.
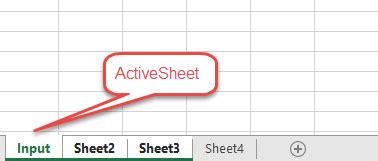
Method 2: Using the Activate
Method
The Activate
method is another way to set the active worksheet in VBA. This method activates a specific worksheet, making it the active worksheet.
Sub ActivateWorksheet()
Worksheets("Sheet2").Activate
MsgBox "Sheet2 is now the active worksheet"
End Sub
In this example, the Worksheets("Sheet2").Activate
statement activates "Sheet2", making it the active worksheet.
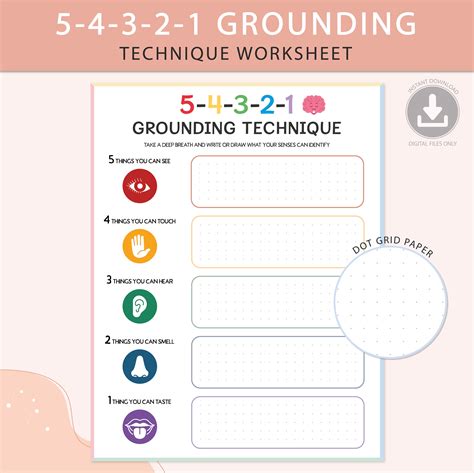
Method 3: Using the Select
Method
The Select
method is another way to set the active worksheet in VBA. This method selects a specific worksheet, making it the active worksheet.
Sub SelectWorksheet()
Worksheets("Sheet3").Select
MsgBox "Sheet3 is now the active worksheet"
End Sub
In this example, the Worksheets("Sheet3").Select
statement selects "Sheet3", making it the active worksheet.
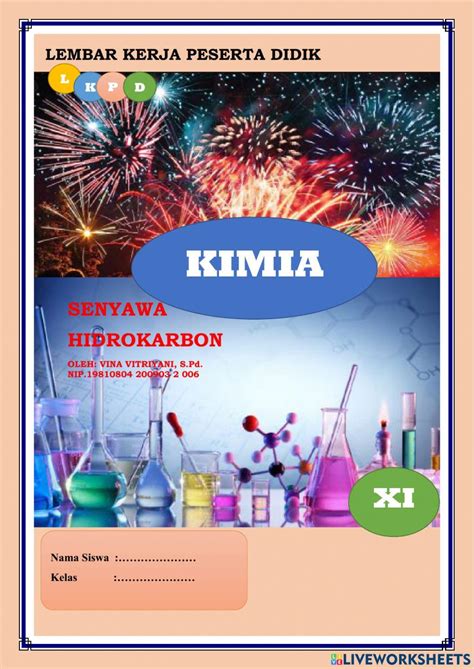
Method 4: Using the Sheets
Collection
The Sheets
collection is a collection of all worksheets in a workbook. You can use this collection to set the active worksheet in VBA.
Sub SetActiveWorksheetUsingSheets()
Sheets("Sheet4").Activate
MsgBox "Sheet4 is now the active worksheet"
End Sub
In this example, the Sheets("Sheet4").Activate
statement sets "Sheet4" as the active worksheet.
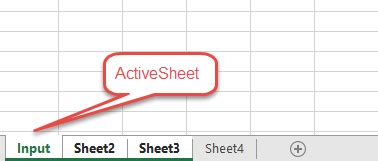
Method 5: Using the Workbook
Object
The Workbook
object represents a workbook in VBA. You can use this object to set the active worksheet in VBA.
Sub SetActiveWorksheetUsingWorkbook()
ThisWorkbook.Worksheets("Sheet5").Activate
MsgBox "Sheet5 is now the active worksheet"
End Sub
In this example, the ThisWorkbook.Worksheets("Sheet5").Activate
statement sets "Sheet5" as the active worksheet.
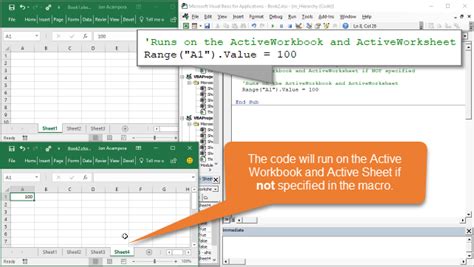
Gallery of VBA Worksheet Examples
VBA Worksheet Examples
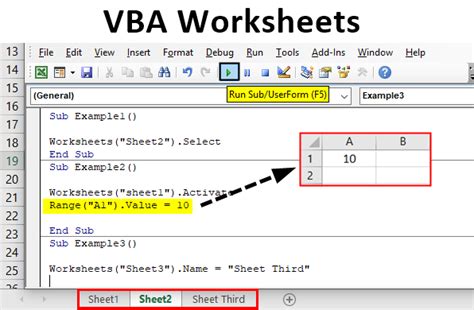
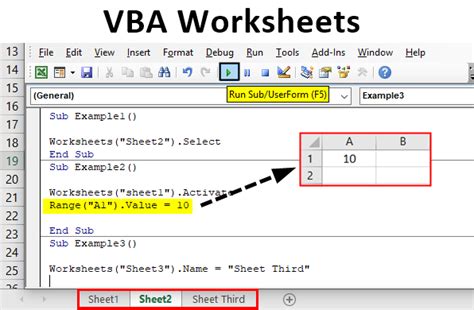
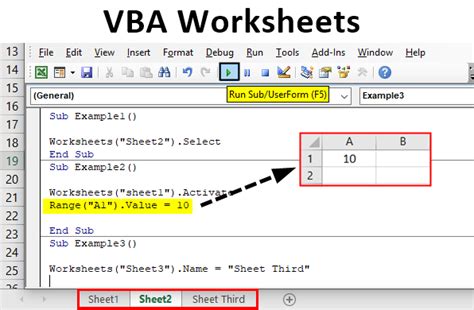
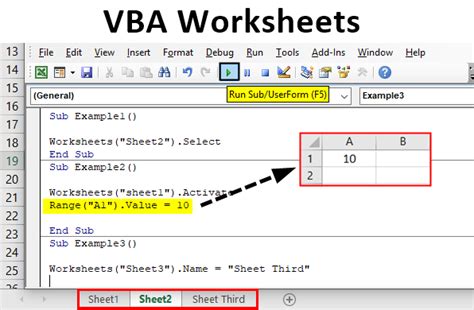
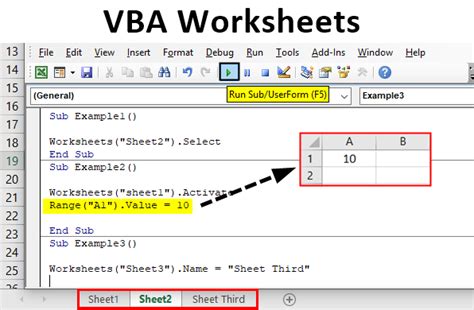
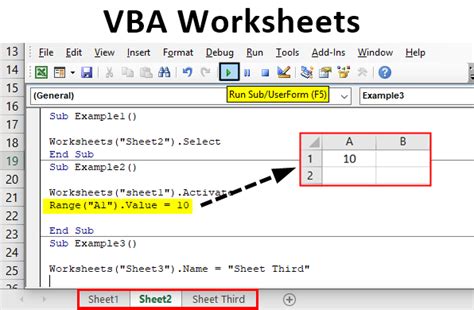
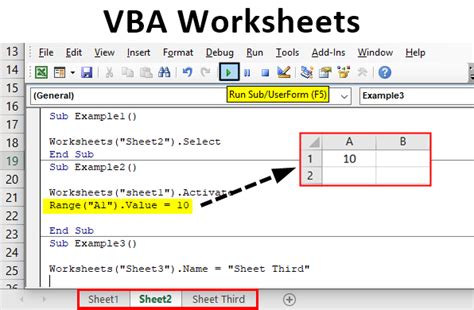
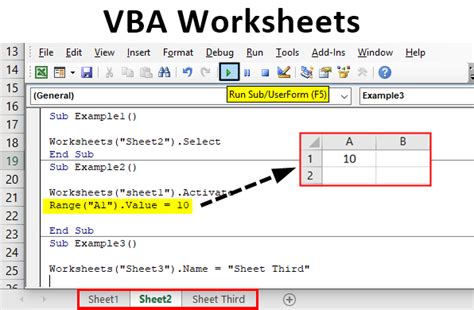
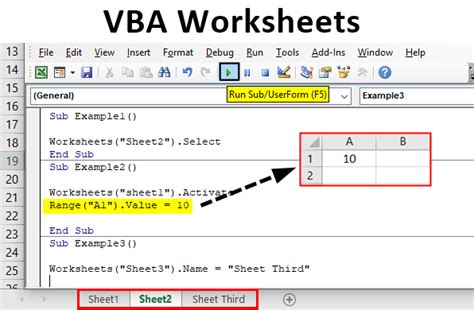
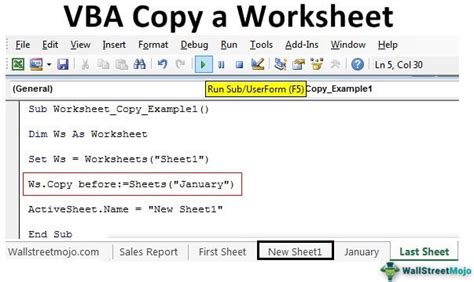
Conclusion
Setting the active worksheet in VBA is a crucial step in automating tasks in Excel. By using one of the five methods outlined in this article, you can ensure that your VBA code executes correctly and efficiently. Remember to always specify the worksheet you want to perform actions on, and use the ActiveSheet
property, Activate
method, Select
method, Sheets
collection, or Workbook
object to set the active worksheet.