Intro
Master Excel VBA with ease! Learn how to select sheets in Excel VBA, a crucial skill for automating tasks. Discover simple and efficient methods to reference, activate, and manipulate worksheets using VBA code. Unlock the full potential of Excel automation with our expert guide, covering worksheet objects, indexing, and looping.
Working with multiple sheets in Excel can be a daunting task, especially when it comes to selecting specific sheets for analysis or reporting. Excel VBA provides a powerful way to automate sheet selection, making it easier to manage and manipulate data across multiple sheets. In this article, we will explore the various ways to select sheets in Excel VBA, including selecting a single sheet, multiple sheets, and even all sheets in a workbook.
Understanding Excel VBA Sheet Selection
Before diving into the code, it's essential to understand the basics of Excel VBA sheet selection. In VBA, sheets are referred to as objects, and each sheet has its own unique properties and methods. The Worksheets
collection contains all the sheets in a workbook, and you can access individual sheets by their index or name.
Selecting a Single Sheet
Selecting a single sheet in Excel VBA is straightforward. You can use the Worksheets
collection to access a specific sheet by its name or index.
Sub SelectSingleSheet()
Worksheets("Sheet1").Select
End Sub
In this example, the code selects the sheet named "Sheet1". You can replace "Sheet1" with the name of the sheet you want to select.
Selecting Multiple Sheets
Selecting multiple sheets in Excel VBA requires a slightly different approach. You can use the Worksheets
collection to select multiple sheets by their names or indices.
Sub SelectMultipleSheets()
Worksheets(Array("Sheet1", "Sheet2", "Sheet3")).Select
End Sub
In this example, the code selects the sheets named "Sheet1", "Sheet2", and "Sheet3".
Selecting All Sheets
Selecting all sheets in a workbook can be useful when you need to perform an action on every sheet. You can use the Worksheets
collection to select all sheets.
Sub SelectAllSheets()
Worksheets.Select
End Sub
In this example, the code selects all sheets in the active workbook.
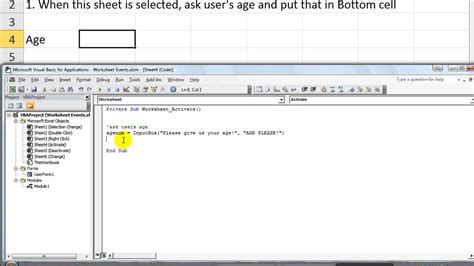
Using Variables to Select Sheets
You can use variables to select sheets in Excel VBA, making your code more dynamic and flexible.
Sub SelectSheetUsingVariable()
Dim sheetName As String
sheetName = "Sheet1"
Worksheets(sheetName).Select
End Sub
In this example, the code uses a variable sheetName
to select the sheet. You can replace the value of sheetName
with the name of the sheet you want to select.
Looping Through Sheets
Looping through sheets in Excel VBA allows you to perform an action on each sheet in a workbook.
Sub LoopThroughSheets()
Dim ws As Worksheet
For Each ws In Worksheets
ws.Select
' Perform an action on the selected sheet
Next ws
End Sub
In this example, the code loops through each sheet in the active workbook, selecting each sheet in turn.
Common Errors When Selecting Sheets
When selecting sheets in Excel VBA, you may encounter errors if the sheet does not exist or if the sheet is not in the active workbook. To avoid these errors, you can use error handling to check if the sheet exists before selecting it.
Sub SelectSheetWithErrorHandling()
On Error Resume Next
Worksheets("Sheet1").Select
If Err.Number <> 0 Then
MsgBox "Sheet not found"
End If
End Sub
In this example, the code uses error handling to check if the sheet exists before selecting it. If the sheet does not exist, the code displays a message box indicating that the sheet was not found.
Conclusion
Selecting sheets in Excel VBA is a fundamental skill that can help you automate tasks and improve productivity. By understanding the basics of sheet selection and using variables, looping, and error handling, you can create powerful VBA code that makes it easy to work with multiple sheets.
Select Sheets in Excel VBA Image Gallery
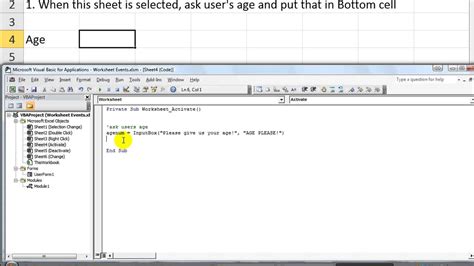
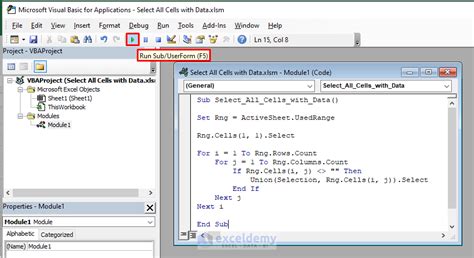
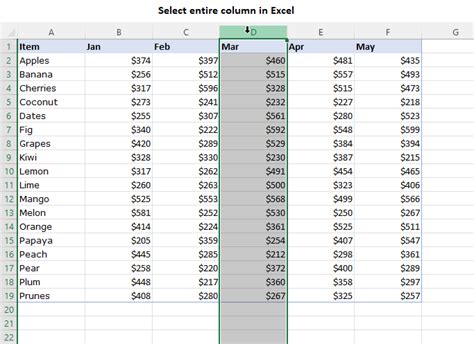
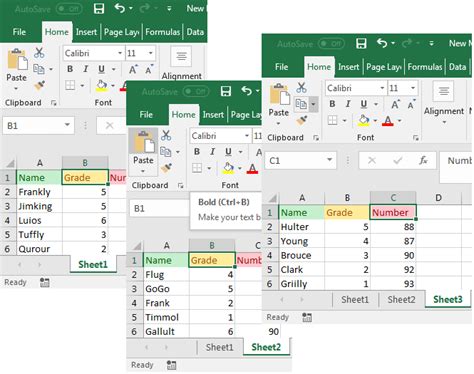
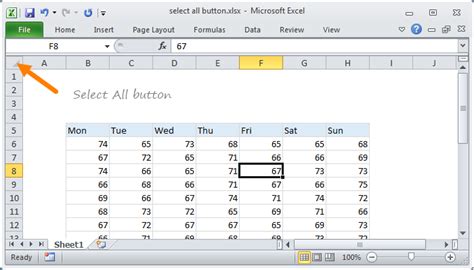
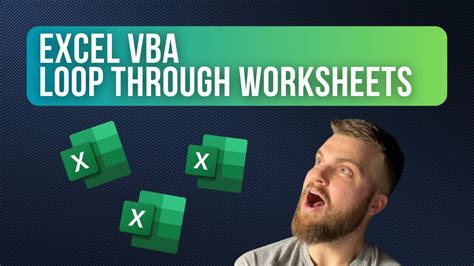
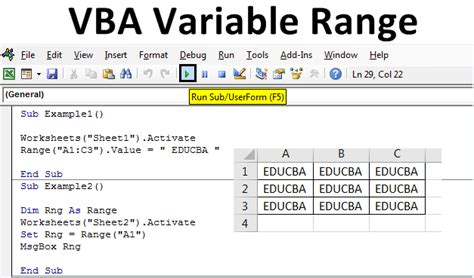
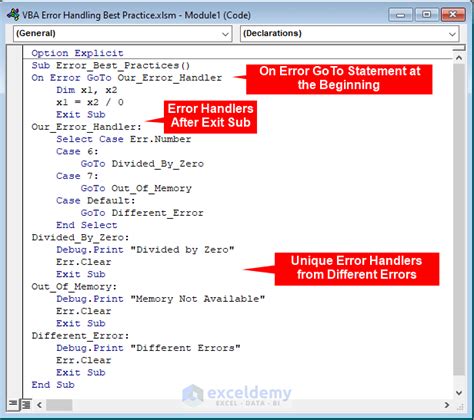
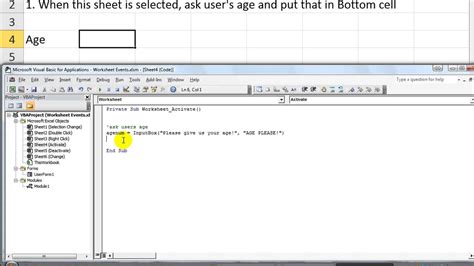
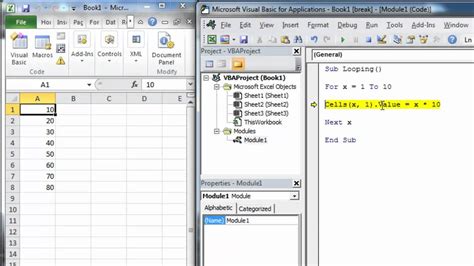
I hope this article has helped you understand how to select sheets in Excel VBA. If you have any questions or need further assistance, please don't hesitate to ask. Share your experiences and tips for working with sheets in Excel VBA in the comments below!