Intro
Master string manipulation in VBA with our expert guide. Discover 5 efficient ways to split strings in VBA, including using the Split function, InStr, Mid, and more. Learn how to handle delimiters, substrings, and array manipulation. Optimize your code with these VBA string splitting techniques and improve your Excel skills.
The art of string manipulation in VBA! Splitting a string is a common task in programming, and VBA is no exception. Whether you're working with text data, parsing user input, or generating reports, knowing how to split a string in VBA is an essential skill. In this article, we'll explore five ways to split a string in VBA, each with its own strengths and weaknesses.
The Importance of String Splitting in VBA
String splitting is a fundamental operation in programming, and VBA is no exception. When working with text data, you often need to break down a string into its constituent parts, such as words, phrases, or substrings. This can be useful for a variety of tasks, including data processing, validation, and reporting. In VBA, string splitting is commonly used in applications such as:
- Text processing and parsing
- Data validation and cleaning
- Report generation and formatting
- User input processing and validation
Method 1: Using the Split
Function
The Split
function is a built-in VBA function that splits a string into an array of substrings based on a specified delimiter. The syntax is simple:
Split(string, delimiter)
For example, to split a string into individual words, you can use the following code:
Dim myString As String
myString = "Hello World, this is a test string"
Dim words As Variant
words = Split(myString, " ")
Debug.Print words(0) ' prints "Hello"
Debug.Print words(1) ' prints "World,"
Method 2: Using the Text to Columns
Method
The Text to Columns
method is a powerful tool in VBA that allows you to split a string into individual columns based on a specified delimiter. This method is particularly useful when working with large datasets or complex text data.
To use the Text to Columns
method, follow these steps:
- Open the Visual Basic Editor and insert a new module.
- Create a new subroutine and add the following code:
Sub SplitString()
Dim myString As String
myString = "Hello World, this is a test string"
Dim words As Variant
words = Split(myString, " ")
' Create a range to hold the split values
Dim rng As Range
Set rng = Range("A1")
' Use the Text to Columns method to split the string
rng.TextToColumns Destination:=rng, DataType:=xlDelimitedText, _
TextQualifier:=xlDoubleQuote, ConsecutiveDelimiter:=False, _
Tab:=False, Semicolon:=False, Comma:=False, Space:=True, _
Other:=False, FieldInfo:=Array(1, 1), TrailingMinusNumbers:=True
End Sub
Method 3: Using Regular Expressions
Regular expressions (regex) are a powerful tool for pattern matching and text manipulation. In VBA, you can use the RegExp
object to split a string based on a specified pattern.
To use regex in VBA, follow these steps:
- Open the Visual Basic Editor and insert a new module.
- Create a new subroutine and add the following code:
Sub SplitStringRegex()
Dim myString As String
myString = "Hello World, this is a test string"
Dim regex As Object
Set regex = CreateObject("VBScript.RegExp")
regex.Pattern = "\s+" ' split on one or more spaces
regex.Global = True
Dim words As Variant
words = regex.Split(myString)
Debug.Print words(0) ' prints "Hello"
Debug.Print words(1) ' prints "World,"
End Sub
Method 4: Using the InStr
and Mid
Functions
The InStr
and Mid
functions are two powerful string manipulation functions in VBA. By combining these functions, you can split a string into individual substrings based on a specified delimiter.
To use the InStr
and Mid
functions, follow these steps:
- Open the Visual Basic Editor and insert a new module.
- Create a new subroutine and add the following code:
Sub SplitStringInStrMid()
Dim myString As String
myString = "Hello World, this is a test string"
Dim delimiter As String
delimiter = " "
Dim words As Variant
words = SplitStringInStrMidHelper(myString, delimiter)
Debug.Print words(0) ' prints "Hello"
Debug.Print words(1) ' prints "World,"
End Sub
Function SplitStringInStrMidHelper(string As String, delimiter As String) As Variant
Dim i As Long
Dim startIndex As Long
Dim endIndex As Long
Dim result As Variant
i = 1
startIndex = 1
Do While i <= Len(string)
endIndex = InStr(startIndex, string, delimiter)
If endIndex = 0 Then
endIndex = Len(string) + 1
End If
result = Mid(string, startIndex, endIndex - startIndex)
startIndex = endIndex + 1
i = i + 1
Loop
SplitStringInStrMidHelper = result
End Function
Method 5: Using the Scripting.FileSystemObject
The Scripting.FileSystemObject
is a powerful object in VBA that allows you to interact with the file system. By using this object, you can split a string into individual files based on a specified delimiter.
To use the Scripting.FileSystemObject
, follow these steps:
- Open the Visual Basic Editor and insert a new module.
- Create a new subroutine and add the following code:
Sub SplitStringFileSystemObject()
Dim myString As String
myString = "Hello World, this is a test string"
Dim fso As Object
Set fso = CreateObject("Scripting.FileSystemObject")
Dim words As Variant
words = SplitStringFileSystemObjectHelper(myString, " ")
Debug.Print words(0) ' prints "Hello"
Debug.Print words(1) ' prints "World,"
End Sub
Function SplitStringFileSystemObjectHelper(string As String, delimiter As String) As Variant
Dim i As Long
Dim startIndex As Long
Dim endIndex As Long
Dim result As Variant
i = 1
startIndex = 1
Do While i <= Len(string)
endIndex = InStr(startIndex, string, delimiter)
If endIndex = 0 Then
endIndex = Len(string) + 1
End If
result = Mid(string, startIndex, endIndex - startIndex)
startIndex = endIndex + 1
i = i + 1
Loop
SplitStringFileSystemObjectHelper = result
End Function
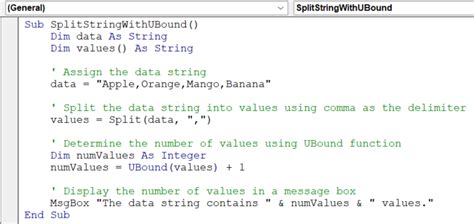
Gallery of VBA String Splitting Methods
VBA String Splitting Methods
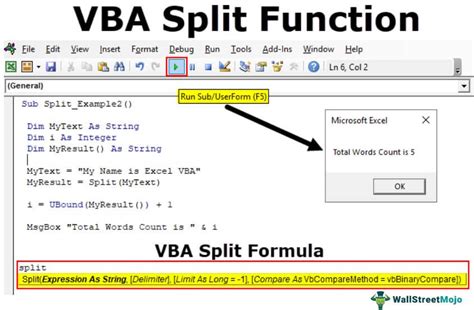
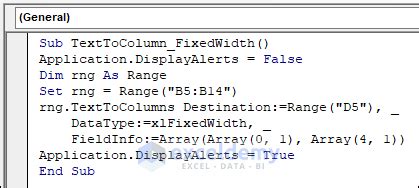
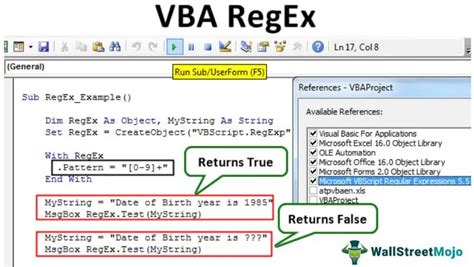
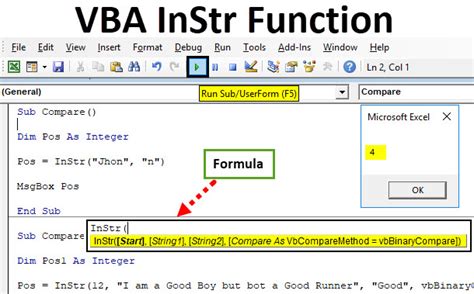
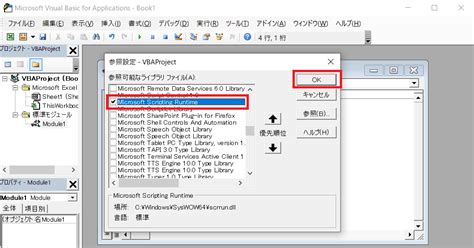
We hope this article has provided you with a comprehensive overview of the different methods for splitting a string in VBA. Whether you're a beginner or an experienced programmer, mastering string splitting techniques is an essential skill for any VBA developer. Remember to experiment with different methods and find the one that works best for your specific use case.
What's your favorite method for splitting strings in VBA? Share your thoughts and experiences in the comments below!