Intro
Unlock the power of data analysis in Excel VBA! Learn 5 effective ways to split data in VBA Excel, including techniques for splitting text strings, arrays, and databases. Discover how to use VBA functions like Split, Text-to-Columns, and array formulas to efficiently manipulate and organize your data for better insights and decision-making.
VBA Excel is a powerful tool for manipulating and analyzing data in Microsoft Excel. One of the most common tasks in data analysis is splitting data into separate categories or groups. In this article, we will explore five ways to split data in VBA Excel.
The Importance of Data Splitting
Data splitting is an essential step in data analysis, as it allows you to categorize and organize your data in a meaningful way. By splitting your data, you can perform separate analyses on each group, identify trends and patterns, and make more informed decisions. In VBA Excel, data splitting can be achieved using various techniques, each with its own strengths and weaknesses.
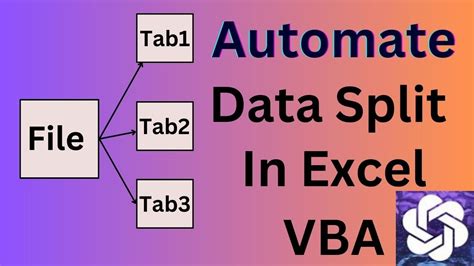
Method 1: Using the Split Function
The Split function is a built-in VBA function that allows you to split a string into an array of substrings based on a specified delimiter. This function can be used to split data into separate categories by defining a delimiter that separates the categories.
Sub SplitDataUsingSplitFunction()
Dim rawData As String
Dim splitData As Variant
Dim delimiter As String
' Define the delimiter
delimiter = ","
' Define the raw data
rawData = "Category1,Value1,Category2,Value2,Category3,Value3"
' Split the data using the Split function
splitData = Split(rawData, delimiter)
' Print the split data
For i = 0 To UBound(splitData)
Debug.Print splitData(i)
Next i
End Sub
Method 2: Using the Text to Columns Feature
The Text to Columns feature in Excel allows you to split data into separate columns based on a specified delimiter. This feature can be automated using VBA by using the Range.TextToColumns
method.
Sub SplitDataUsingTextToColumns()
Dim rawDataRange As Range
Dim delimiter As String
' Define the delimiter
delimiter = ","
' Define the raw data range
Set rawDataRange = Range("A1")
' Split the data using the Text to Columns feature
rawDataRange.TextToColumns Destination:=Range("A1"), DataType:=xlDelimitedText, TextQualifier:=xlDoubleQuote, ConsecutiveDelimiter:=False, Tab:=False, Semicolon:=False, Comma:=True, Space:=False, Other:=False, OtherChar:=" "
' Print the split data
For i = 1 To Range("A1").CurrentRegion.Columns.Count
Debug.Print Range("A1").Offset(0, i - 1).Value
Next i
End Sub
Method 3: Using the Power Query Editor
The Power Query Editor is a powerful tool in Excel that allows you to manipulate and transform data. One of the features of the Power Query Editor is the ability to split data into separate columns based on a specified delimiter.
Sub SplitDataUsingPowerQueryEditor()
Dim rawDataRange As Range
Dim delimiter As String
' Define the delimiter
delimiter = ","
' Define the raw data range
Set rawDataRange = Range("A1")
' Split the data using the Power Query Editor
With ActiveSheet.QueryTables.Add(Connection:="TEXT;" & ActiveWorkbook.Path & "\" & ActiveSheet.Name & ".txt", Destination:=Range("A1"))
.Name = "SplitData"
.FieldNames = True
.RowNumbers = False
.FillAdjacentFormulas = False
.PreserveFormatting = True
.RefreshOnFileOpen = False
.RefreshStyle = xlInsertDeleteCells
.SavePassword = False
.SaveData = True
.AdjustColumnWidth = True
.RefreshPeriod = 0
.TextFilePlatform = 437
.TextFileStartRow = 1
.TextFileParseType = xlDelimitedText
.TextFileTextQualifier = xlTextQualifierDoubleQuote
.TextFileConsecutiveDelimiter = False
.TextFileTabDelimiter = False
.TextFileSemicolonDelimiter = False
.TextFileCommaDelimiter = True
.TextFileSpaceDelimiter = False
.TextFileOtherDelimiter = " "
.TextFileColumnDataTypes = Array(1)
.TextFileTrailingMinusNumbers = True
.Refresh BackgroundQuery:=False
End With
' Print the split data
For i = 1 To Range("A1").CurrentRegion.Columns.Count
Debug.Print Range("A1").Offset(0, i - 1).Value
Next i
End Sub
Method 4: Using the VBA Scripting Dictionary
The VBA Scripting Dictionary is a powerful tool that allows you to store and manipulate data in a dictionary-like structure. One of the features of the VBA Scripting Dictionary is the ability to split data into separate categories by defining a key-value pair.
Sub SplitDataUsingVbaScriptingDictionary()
Dim rawData As String
Dim dictionary As Object
Dim key As Variant
Dim value As Variant
' Define the raw data
rawData = "Category1,Value1,Category2,Value2,Category3,Value3"
' Create a new dictionary
Set dictionary = CreateObject("Scripting.Dictionary")
' Split the data into key-value pairs
For i = 0 To UBound(Split(rawData, ",")) - 1 Step 2
key = Split(rawData, ",")(i)
value = Split(rawData, ",")(i + 1)
dictionary.Add key, value
Next i
' Print the split data
For Each key In dictionary.Keys
Debug.Print key & ": " & dictionary(key)
Next key
End Sub
Method 5: Using the VBA ArrayList
The VBA ArrayList is a powerful tool that allows you to store and manipulate data in a list-like structure. One of the features of the VBA ArrayList is the ability to split data into separate categories by defining a list of values.
Sub SplitDataUsingVbaArrayList()
Dim rawData As String
Dim arrayList As Object
Dim value As Variant
' Define the raw data
rawData = "Category1,Value1,Category2,Value2,Category3,Value3"
' Create a new array list
Set arrayList = CreateObject("System.Collections.ArrayList")
' Split the data into a list of values
For Each value In Split(rawData, ",")
arrayList.Add value
Next value
' Print the split data
For i = 0 To arrayList.Count - 1 Step 2
Debug.Print arrayList(i) & ": " & arrayList(i + 1)
Next i
End Sub
Conclusion
In this article, we have explored five ways to split data in VBA Excel. Each method has its own strengths and weaknesses, and the choice of method depends on the specific requirements of the project. Whether you are using the Split function, the Text to Columns feature, the Power Query Editor, the VBA Scripting Dictionary, or the VBA ArrayList, you can achieve powerful data splitting capabilities in VBA Excel.
Data Splitting in VBA Excel Image Gallery
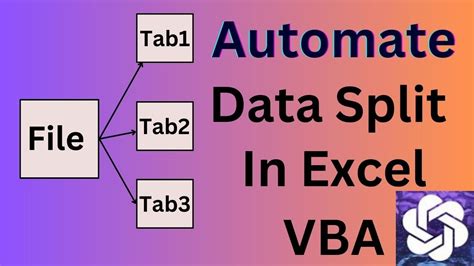
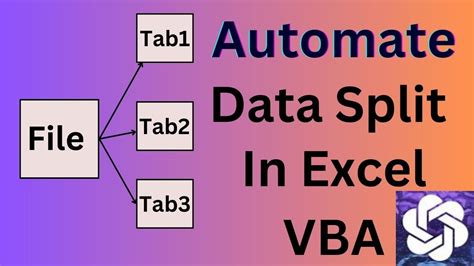
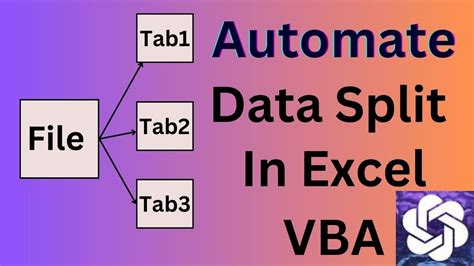
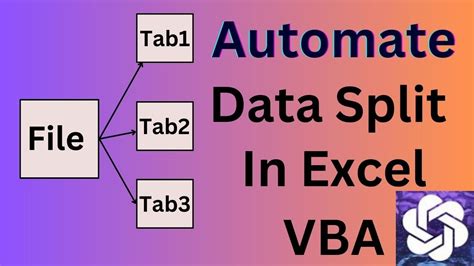
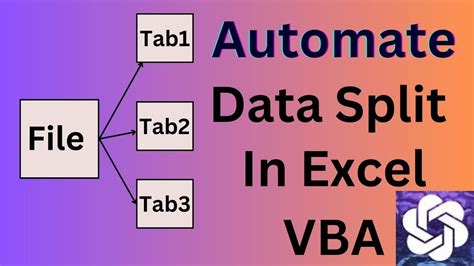
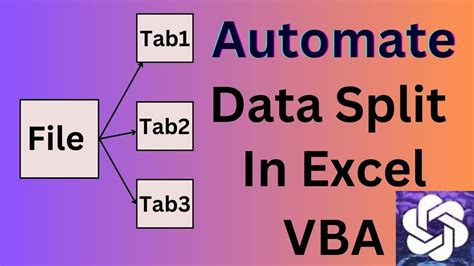
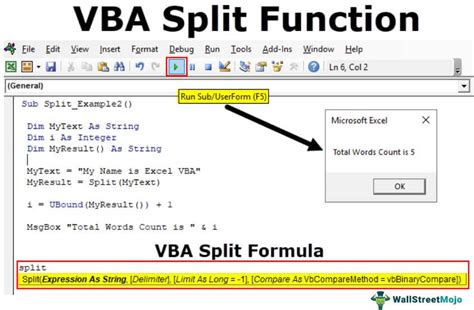
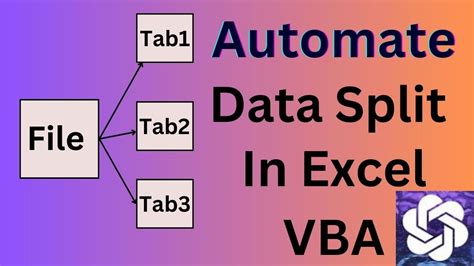
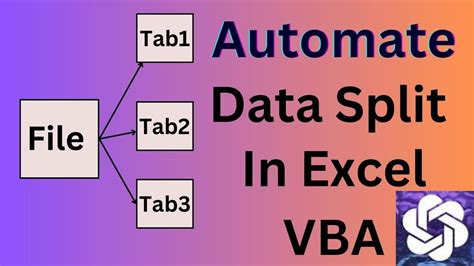
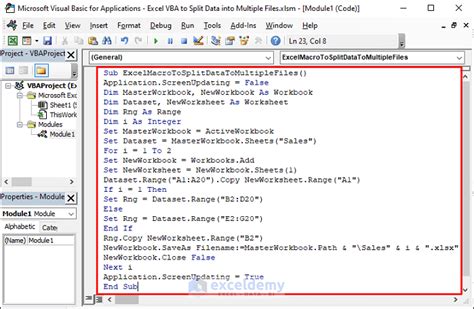