Intro
Master the art of string concatenation in VBA with this comprehensive guide. Learn how to efficiently combine strings, numbers, and variables using operators like & and +, and discover best practices for debugging and optimizing your code. Improve your VBA skills and simplify your string manipulation tasks with expert tips and examples.
String concatenation is a fundamental concept in programming, and it's no exception in VBA (Visual Basic for Applications). When working with strings in VBA, concatenation allows you to combine two or more strings into a single string. This can be useful for tasks such as building messages, creating file paths, or formatting data. In this article, we'll delve into the world of string concatenation in VBA, exploring the different methods, best practices, and common pitfalls to avoid.
The Importance of String Concatenation in VBA
String concatenation is a crucial technique in VBA, especially when working with text data. By combining strings, you can create dynamic text that can be used in various contexts, such as:
- Building error messages or notifications
- Creating file paths or URLs
- Formatting data for output or reporting
- Constructing SQL queries or database commands
Without string concatenation, you'd be limited to working with fixed, static strings, which can become inflexible and cumbersome.
Methods of String Concatenation in VBA
VBA provides several methods for concatenating strings, each with its strengths and weaknesses.
1. Using the &
Operator
The &
operator is the most common method for concatenating strings in VBA. It's simple, intuitive, and easy to read.
Dim str1 As String
Dim str2 As String
Dim result As String
str1 = "Hello"
str2 = "World"
result = str1 & " " & str2
2. Using the +
Operator
The +
operator can also be used for string concatenation, but it's generally less preferred than the &
operator. This is because the +
operator can be ambiguous when working with numeric values.
result = str1 + " " + str2
3. Using the Join
Function
The Join
function is a more advanced method for concatenating strings. It allows you to concatenate an array of strings into a single string, using a specified delimiter.
Dim arr() As String
arr = Array("Hello", "World")
result = Join(arr, " ")
Best Practices for String Concatenation in VBA
To ensure efficient and readable string concatenation, follow these best practices:
- Use the
&
operator for most cases, as it's the most readable and maintainable method. - Avoid using the
+
operator for string concatenation, as it can lead to confusion with numeric operations. - Use the
Join
function when working with arrays of strings or when you need to concatenate multiple strings with a specific delimiter. - Use meaningful variable names to improve code readability.
- Keep your concatenated strings concise and focused on a single task.
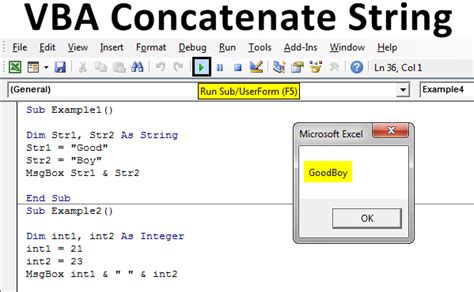
Common Pitfalls to Avoid
When working with string concatenation in VBA, be aware of the following common pitfalls:
- Null values: Be cautious when concatenating strings that may contain null values, as this can lead to unexpected results.
- Type mismatches: Ensure that you're concatenating strings with the correct data type, as type mismatches can cause errors.
- Performance: Avoid excessive string concatenation, as it can impact performance, especially when working with large strings or loops.
Conclusion
Mastering string concatenation in VBA is essential for working with text data in a flexible and efficient manner. By following best practices and avoiding common pitfalls, you can write more readable, maintainable, and efficient code. Remember to use the &
operator for most cases, and explore the Join
function for more advanced concatenation tasks.
Gallery of String Concatenation Examples
String Concatenation Examples
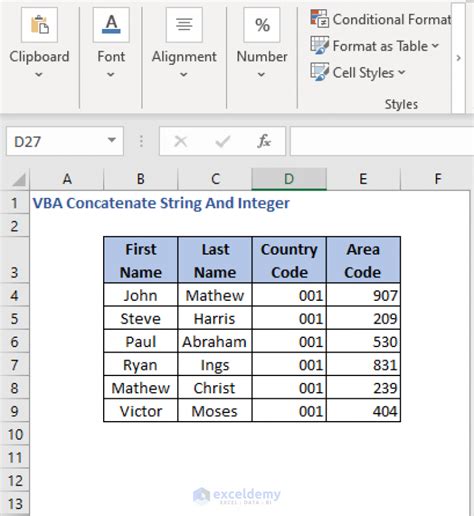
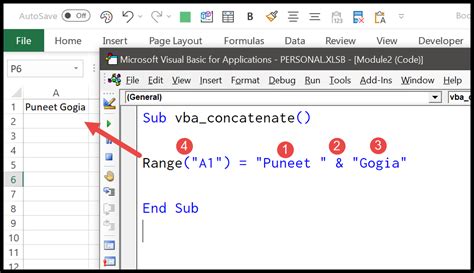
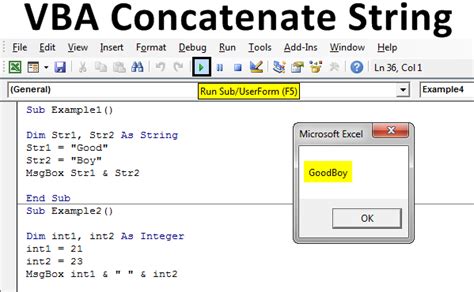
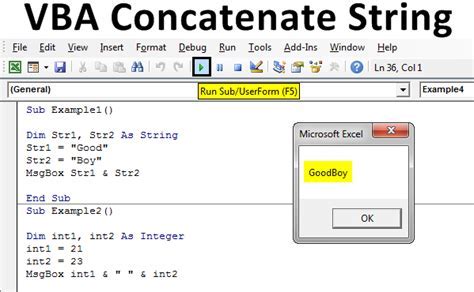
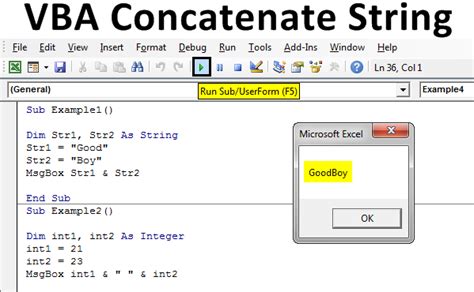
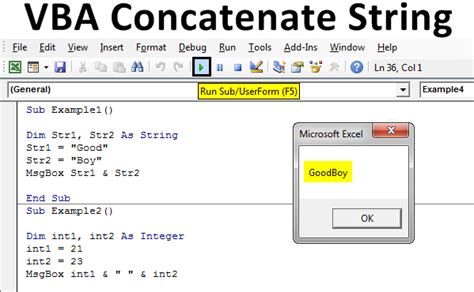
We hope this article has helped you master the art of string concatenation in VBA. Share your thoughts and experiences with string concatenation in the comments below!