Intro
Discover how to calculate the sum of a range in VBA with ease. Learn the simple formula and techniques to sum a range of cells in Excel using VBA. Master the art of range calculation and improve your spreadsheet skills. Get the most out of your data with this comprehensive VBA sum range guide.
Mastering the art of calculating the sum of a range in VBA is an essential skill for any Excel power user. Whether you're dealing with simple arithmetic or complex financial models, being able to efficiently sum a range of values can save you time and effort. In this comprehensive guide, we'll explore the different methods for calculating the sum of a range in VBA, including examples, tips, and best practices.
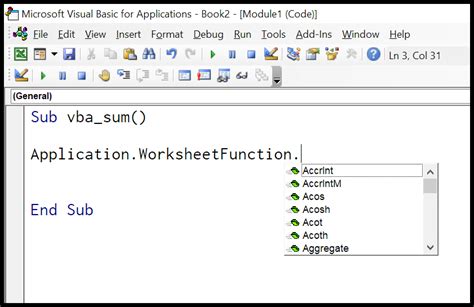
Understanding the Basics of VBA Ranges
Before diving into the calculation methods, it's essential to understand the basics of VBA ranges. A range in VBA refers to a group of cells that can be selected and manipulated together. Ranges can be defined in various ways, including:
- A single cell (e.g.,
Range("A1")
) - A group of adjacent cells (e.g.,
Range("A1:A10")
) - A group of non-adjacent cells (e.g.,
Range("A1,A3,A5")
) - A entire row or column (e.g.,
Range("A:A")
orRange("1:1")
)
Method 1: Using the Application.Sum
Method
The Application.Sum
method is the most straightforward way to calculate the sum of a range in VBA. This method takes a range as an argument and returns the sum of its values.
Sub SumRangeExample()
Dim total As Double
total = Application.Sum(Range("A1:A10"))
MsgBox "The sum of the range is: " & total
End Sub
Method 2: Using the Range.Sum
Method
Alternatively, you can use the Range.Sum
method to calculate the sum of a range. This method is similar to the Application.Sum
method but is applied directly to the range object.
Sub SumRangeExample()
Dim total As Double
total = Range("A1:A10").Sum
MsgBox "The sum of the range is: " & total
End Sub
Method 3: Using a Loop
If you need more control over the calculation or want to perform additional operations on the range values, you can use a loop to iterate through the cells and calculate the sum.
Sub SumRangeExample()
Dim total As Double
Dim cell As Range
total = 0
For Each cell In Range("A1:A10")
total = total + cell.Value
Next cell
MsgBox "The sum of the range is: " & total
End Sub
Tips and Best Practices
- When working with large ranges, it's more efficient to use the
Application.Sum
orRange.Sum
methods, as they are optimized for performance. - If you need to perform additional operations on the range values, consider using a loop to iterate through the cells.
- Always declare variables and use explicit data typing to avoid errors and improve code readability.
- Use meaningful variable names and comments to make your code easier to understand and maintain.
Common Errors and Troubleshooting
- Error: "Object required" - This error occurs when you try to use the
Application.Sum
orRange.Sum
methods on a range that is not properly defined. - Solution: Verify that the range is correctly defined and that the method is applied to the correct object.
- Error: "Type mismatch" - This error occurs when you try to assign the result of the sum calculation to a variable of the wrong data type.
- Solution: Verify that the variable is declared with the correct data type (e.g.,
Double
) and that the assignment is correct.
Sum of Range in VBA Image Gallery
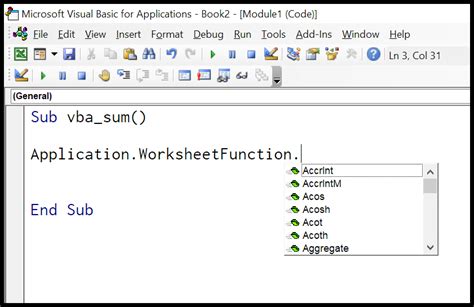
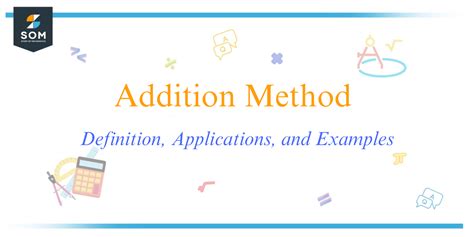
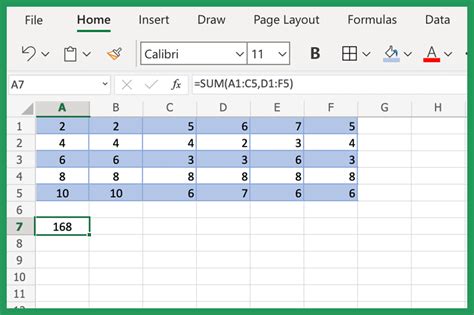
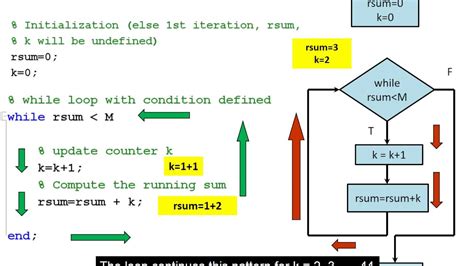
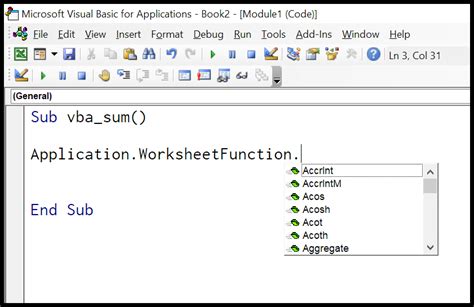
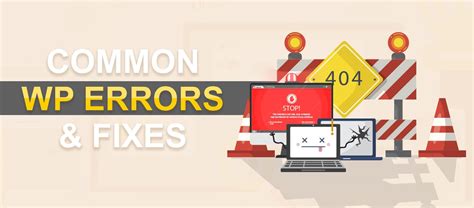
We hope this comprehensive guide has helped you master the art of calculating the sum of a range in VBA. Whether you're a beginner or an experienced developer, these methods and tips will help you streamline your code and improve your productivity. Do you have any questions or need further assistance? Please leave a comment below, and we'll be happy to help.