Intro
Resolve frustrating Python errors with our expert guide on 5 Ways To Fix SyntaxError: Cannot Assign To Function Call. Discover how to identify and fix function call assignment mistakes, understand Python syntax rules, and troubleshoot common issues like invalid syntax, undefined variables, and misplaced operators.
Are you tired of encountering the frustrating "SyntaxError: cannot assign to function call" error in your Python code? This error occurs when you try to assign a value to a function call, which is not allowed in Python. In this article, we will explore five ways to fix this error and get your code running smoothly.
Understanding the Error
Before we dive into the solutions, let's understand what causes this error. In Python, a function call is an expression that evaluates to a value. You cannot assign a value to an expression, as it is not a valid target for assignment. For example, consider the following code:
def add(a, b):
return a + b
add(2, 3) = 5 # SyntaxError: cannot assign to function call
In this example, we are trying to assign the value 5 to the result of the add
function call, which is not allowed.
Solution 1: Use a Variable to Store the Result
One way to fix this error is to store the result of the function call in a variable and then assign the value to the variable. Here's an example:
def add(a, b):
return a + b
result = add(2, 3)
result = 5 # This is allowed
By storing the result of the add
function call in the result
variable, we can then assign a new value to the variable.
Solution 2: Use a Different Assignment Operator
Another way to fix this error is to use a different assignment operator, such as the +=
operator, which is allowed on function calls. Here's an example:
def add(a, b):
return a + b
result = add(2, 3)
result += 5 # This is allowed
In this example, we are using the +=
operator to add 5 to the result of the add
function call.
Solution 3: Use a Function with a Side Effect
If the function you are calling has a side effect, such as modifying an external variable or printing to the console, you can use the function call as an expression. Here's an example:
x = 0
def increment():
global x
x += 1
increment() # This is allowed
print(x) # prints 1
In this example, the increment
function has a side effect of modifying the external variable x
.
Solution 4: Use a Lambda Function
Another way to fix this error is to use a lambda function, which is a small anonymous function that can be defined inline. Here's an example:
add = lambda a, b: a + b
result = add(2, 3)
result = 5 # This is allowed
In this example, we define a lambda function add
that takes two arguments and returns their sum. We can then assign a new value to the result
variable.
Solution 5: Reconsider Your Code Design
Finally, if none of the above solutions work for you, it may be worth reconsidering your code design. Ask yourself if you really need to assign a value to a function call. Perhaps there is a better way to achieve your goal without using a function call as an assignment target.
In conclusion, the "SyntaxError: cannot assign to function call" error is a common error in Python that can be fixed in several ways. By using a variable to store the result, using a different assignment operator, using a function with a side effect, using a lambda function, or reconsidering your code design, you can avoid this error and write more effective Python code.
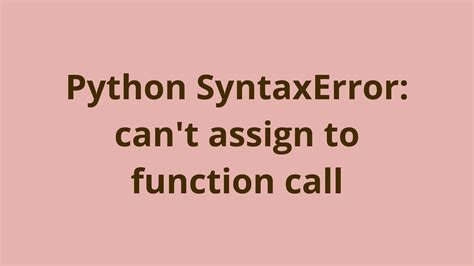
Common Use Cases for Function Calls
Function calls are a fundamental concept in programming, and they have many use cases. Here are some common use cases for function calls:
- Reusable Code: Function calls allow you to write reusable code that can be called multiple times from different parts of your program.
- Modular Code: Function calls enable you to write modular code that is easier to maintain and debug.
- Abstraction: Function calls provide a way to abstract away complex logic and provide a simple interface to users.
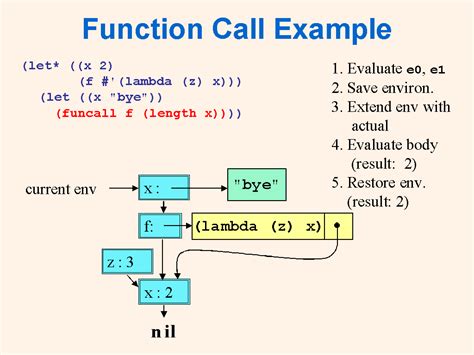
Best Practices for Using Function Calls
Here are some best practices for using function calls:
- Use Descriptive Names: Use descriptive names for your functions to make it clear what they do.
- Keep Functions Short: Keep your functions short and focused on a single task.
- Use Docstrings: Use docstrings to document your functions and make it clear what they do.
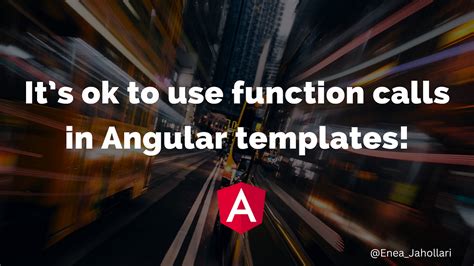
Gallery of SyntaxError: Cannot Assign To Function Call
SyntaxError: Cannot Assign To Function Call Image Gallery
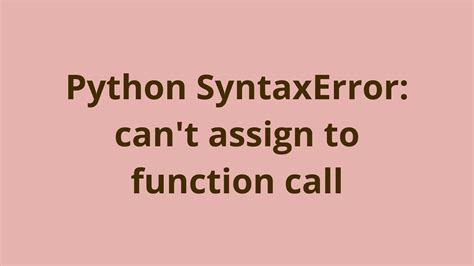
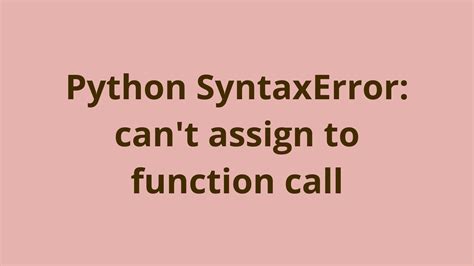
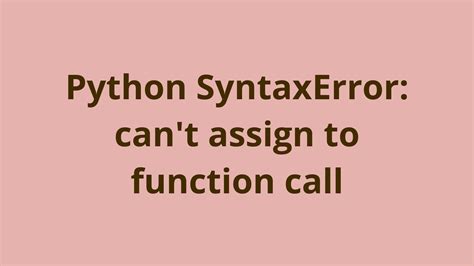
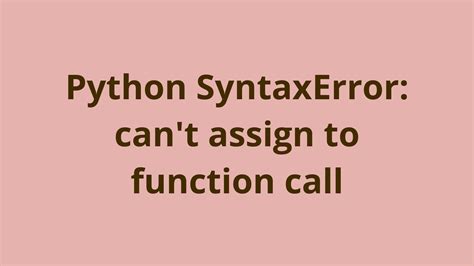
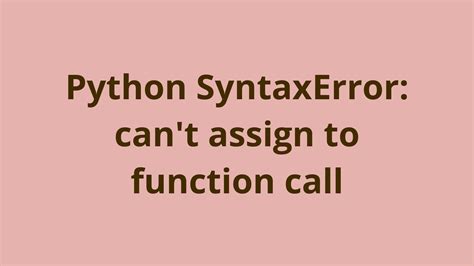
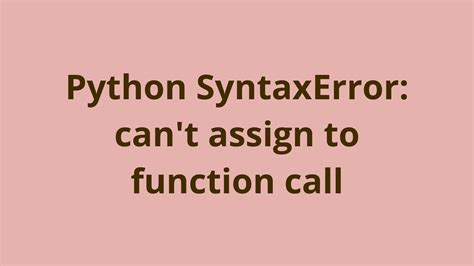
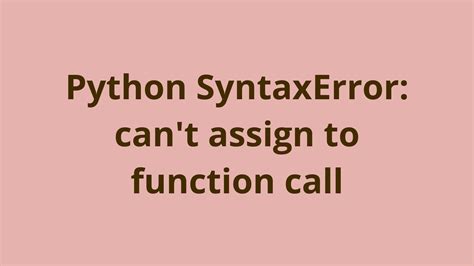
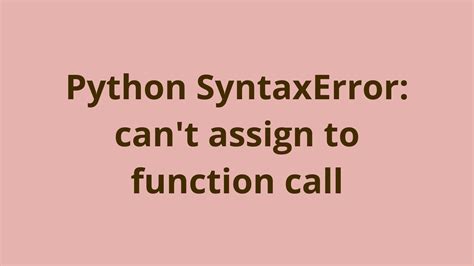
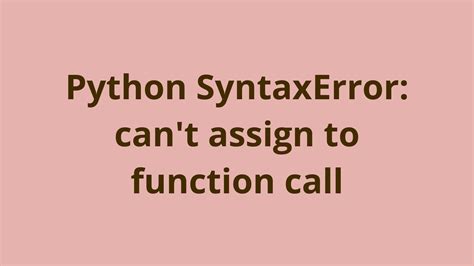
We hope this article has helped you understand and fix the "SyntaxError: cannot assign to function call" error in Python. If you have any questions or comments, please feel free to leave them in the section below.