Unlock the Power of PivotTables: 5 Ways to Update Pivot Table in VBA
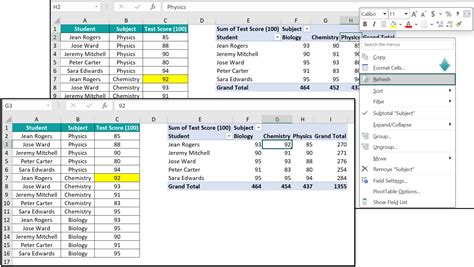
When working with large datasets in Excel, PivotTables are an essential tool for summarizing and analyzing data. However, when you need to update your PivotTable, you may find yourself performing tedious manual updates. Fortunately, VBA (Visual Basic for Applications) provides a powerful way to automate these updates. In this article, we will explore five ways to update a PivotTable in VBA.
1. Refreshing a PivotTable
One of the most basic ways to update a PivotTable in VBA is to refresh it. This is particularly useful when your data source has changed, and you want to reflect those changes in your PivotTable. You can use the following code to refresh a PivotTable:
Sub RefreshPivotTable()
Dim pt As PivotTable
Set pt = ActiveSheet.PivotTables("PivotTableName")
pt.RefreshTable
End Sub
Replace "PivotTableName"
with the name of your PivotTable.
Updating PivotTable Data Source
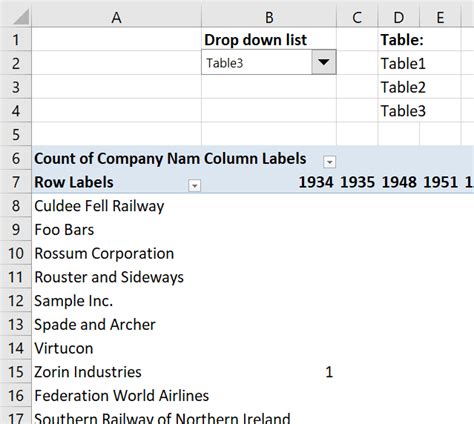
2. Changing the PivotTable Data Source
Sometimes, you may need to update the data source of your PivotTable. This can be done by changing the range of cells that the PivotTable is based on. You can use the following code to update the data source:
Sub UpdatePivotTableDataSource()
Dim pt As PivotTable
Set pt = ActiveSheet.PivotTables("PivotTableName")
pt.ChangePivotCache ActiveWorkbook.PivotCaches.Create( _
SourceType:=xlDatabase, _
SourceData:="NewDataSourceRange")
End Sub
Replace "PivotTableName"
with the name of your PivotTable and "NewDataSourceRange"
with the new range of cells that you want to use as the data source.
Adding and Removing PivotTable Fields
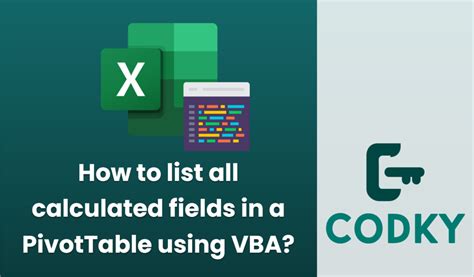
3. Adding and Removing PivotTable Fields
You can also update your PivotTable by adding or removing fields. This can be done using the following code:
Sub AddPivotTableField()
Dim pt As PivotTable
Set pt = ActiveSheet.PivotTables("PivotTableName")
pt.PivotFields("FieldName").Orientation = xlDataField
End Sub
Sub RemovePivotTableField()
Dim pt As PivotTable
Set pt = ActiveSheet.PivotTables("PivotTableName")
pt.PivotFields("FieldName").Orientation = xlHidden
End Sub
Replace "PivotTableName"
with the name of your PivotTable and "FieldName"
with the name of the field that you want to add or remove.
Updating PivotTable Layout
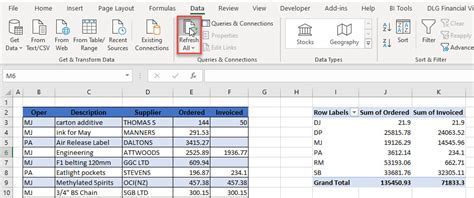
4. Updating PivotTable Layout
You can also update the layout of your PivotTable by changing the way that fields are displayed. This can be done using the following code:
Sub UpdatePivotTableLayout()
Dim pt As PivotTable
Set pt = ActiveSheet.PivotTables("PivotTableName")
pt.CompactLayoutRowHeader = False
pt RepeatAllLabels xlRepeatLabels
End Sub
Replace "PivotTableName"
with the name of your PivotTable.
Automating PivotTable Updates
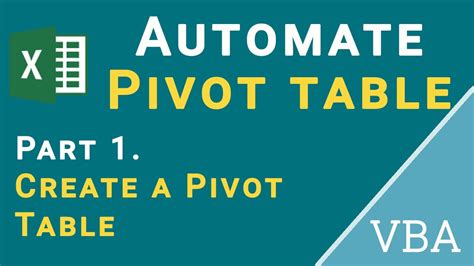
5. Automating PivotTable Updates
Finally, you can automate PivotTable updates by using VBA to schedule updates at regular intervals. This can be done by using the OnTime
method to schedule a macro to run at a specific time. You can use the following code to schedule a PivotTable update:
Sub SchedulePivotTableUpdate()
Application.OnTime TimeValue("14:00:00"), "UpdatePivotTable"
End Sub
Sub UpdatePivotTable()
' Code to update PivotTable goes here
End Sub
Replace the time in the OnTime
method with the time that you want to schedule the update.
PivotTable Update Gallery
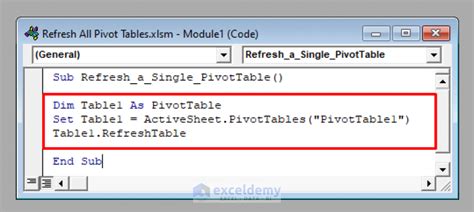
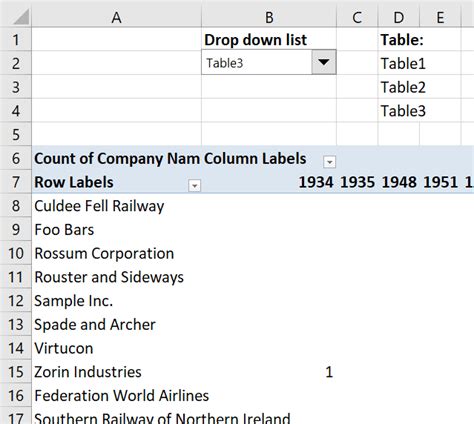
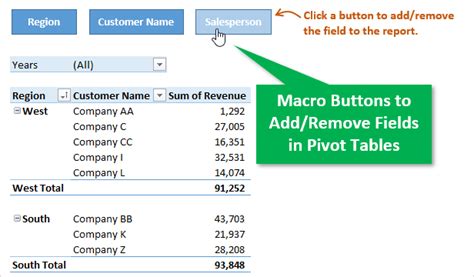
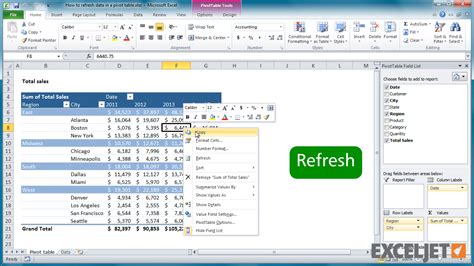
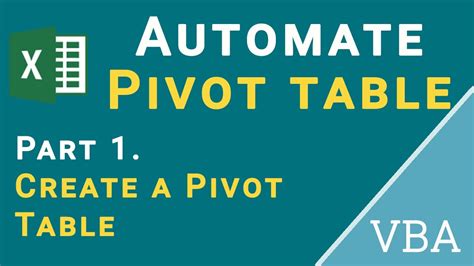
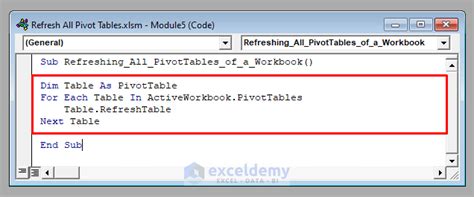
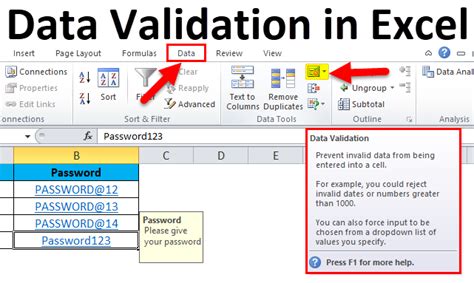
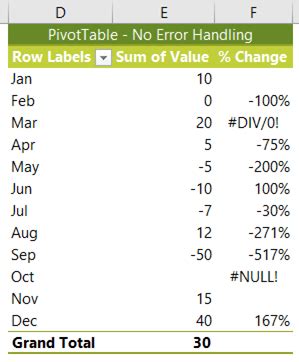
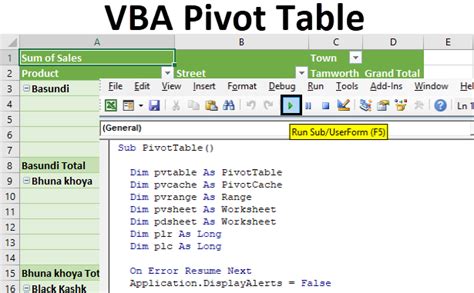
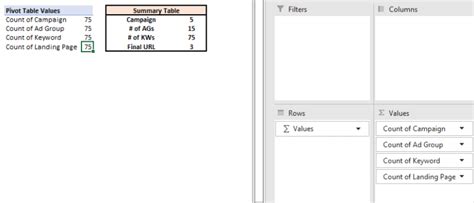
We hope this article has helped you learn how to update PivotTables in VBA. Whether you need to refresh your PivotTable, update its data source, or automate updates, VBA provides a powerful way to get the job done. Do you have any questions or topics you'd like us to cover in future articles? Let us know in the comments below!