Intro
Unlock the full potential of Excel VBA programming with our comprehensive guide to mastering UsedRange. Learn how to efficiently manipulate and analyze data within worksheets using VBA UsedRange properties and methods, including referencing, resizing, and looping. Discover expert tips and tricks to boost your VBA skills and automate tasks with ease.
The used range in VBA is a crucial concept that every Excel VBA developer should master. It refers to the range of cells in a worksheet that contains data, formulas, or formatting. Understanding how to work with the used range can help you optimize your code, reduce errors, and improve performance.
What is the Used Range in VBA?
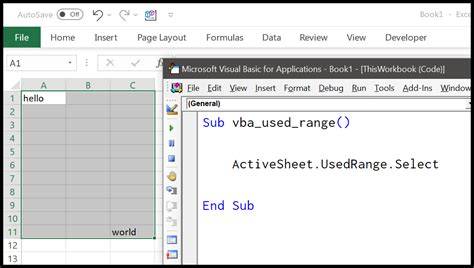
The used range is the range of cells that Excel considers to be in use. It's the range of cells that Excel will iterate over when performing operations such as formatting, calculations, or data manipulation. The used range can be affected by various factors, including data entry, formatting, and formulas.
Why is the Used Range Important in VBA?
The used range is essential in VBA because it allows developers to:
- Optimize code performance by avoiding unnecessary iterations
- Reduce errors by ensuring that data is processed correctly
- Improve data manipulation and analysis by accurately identifying the range of cells that contain data
How to Determine the Used Range in VBA
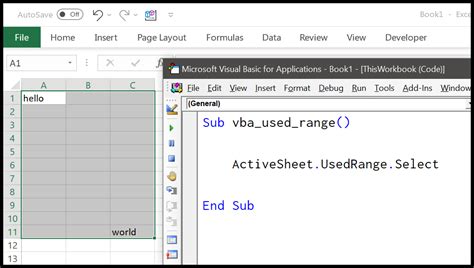
There are several ways to determine the used range in VBA:
- Using the
UsedRange
property: This property returns the used range of the active worksheet. - Using the
Cells
property: This property returns a range of cells that can be used to determine the used range. - Using the
SpecialCells
method: This method returns a range of cells that meet specific criteria, such as cells that contain formulas or values.
Example Code: Determine the Used Range
Sub DetermineUsedRange()
Dim ws As Worksheet
Set ws = ActiveSheet
' Determine the used range using the UsedRange property
Dim usedRange As Range
Set usedRange = ws.UsedRange
' Determine the used range using the Cells property
Dim cellsRange As Range
Set cellsRange = ws.Cells
' Determine the used range using the SpecialCells method
Dim specialCellsRange As Range
Set specialCellsRange = ws.SpecialCells(xlCellTypeConstants)
MsgBox "Used Range: " & usedRange.Address
MsgBox "Cells Range: " & cellsRange.Address
MsgBox "Special Cells Range: " & specialCellsRange.Address
End Sub
How to Reset the Used Range in VBA
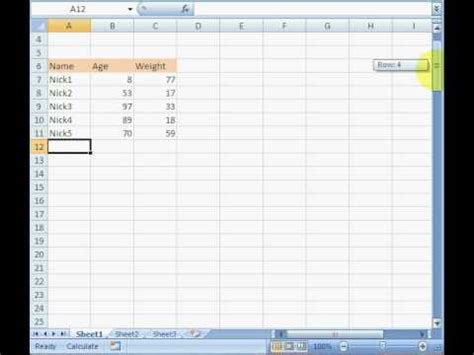
Sometimes, the used range can become corrupted or incorrect, leading to errors or performance issues. To reset the used range, you can use the following methods:
- Using the
UsedRange
property: Set theUsedRange
property toNothing
to reset the used range. - Using the
Cells
property: Clear the contents of the cells to reset the used range. - Using the
SpecialCells
method: Clear the contents of the special cells to reset the used range.
Example Code: Reset the Used Range
Sub ResetUsedRange()
Dim ws As Worksheet
Set ws = ActiveSheet
' Reset the used range using the UsedRange property
ws.UsedRange = Nothing
' Reset the used range using the Cells property
ws.Cells.ClearContents
' Reset the used range using the SpecialCells method
ws.SpecialCells(xlCellTypeConstants).ClearContents
MsgBox "Used Range Reset"
End Sub
Best Practices for Working with the Used Range in VBA
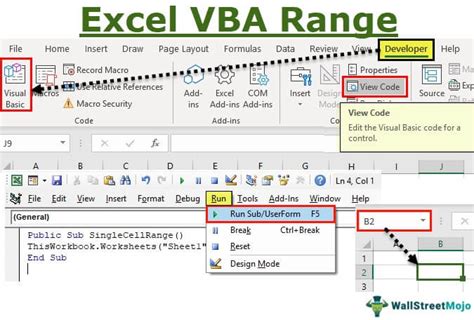
Here are some best practices for working with the used range in VBA:
- Always determine the used range before performing operations on the worksheet.
- Use the
UsedRange
property to get the used range of the active worksheet. - Avoid using the
Cells
property to get the used range, as it can be slow and inefficient. - Use the
SpecialCells
method to get the used range of cells that meet specific criteria. - Reset the used range when necessary to avoid errors and performance issues.
Conclusion
Mastering the used range in VBA is essential for any Excel VBA developer. By understanding how to determine and reset the used range, you can optimize your code, reduce errors, and improve performance. Remember to follow best practices for working with the used range, and always determine the used range before performing operations on the worksheet.
Gallery of VBA Used Range:
VBA Used Range Image Gallery
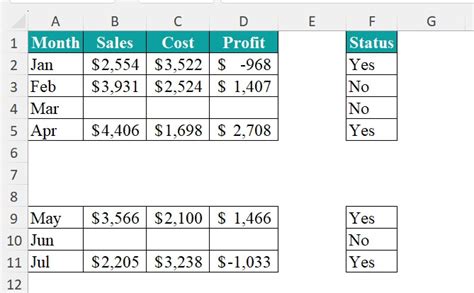
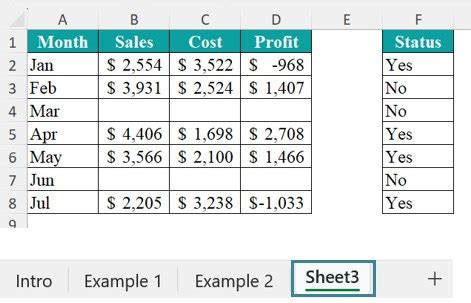
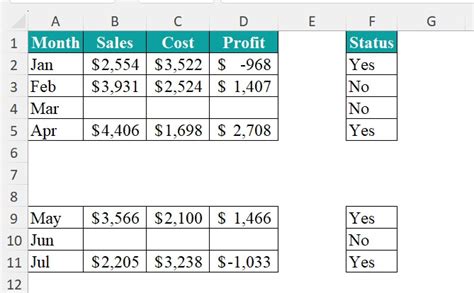
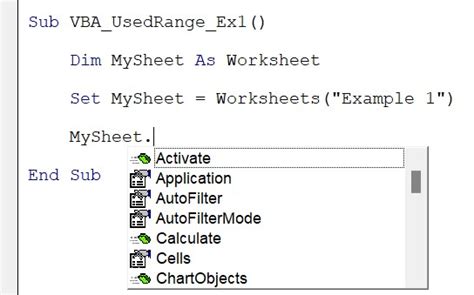
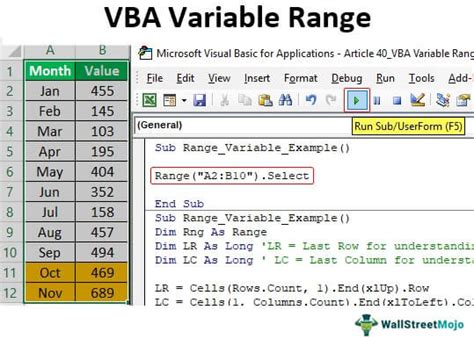
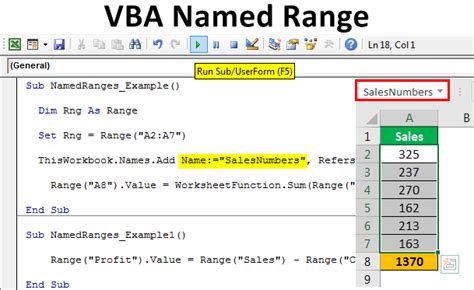
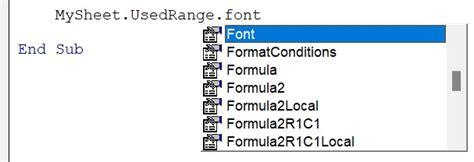
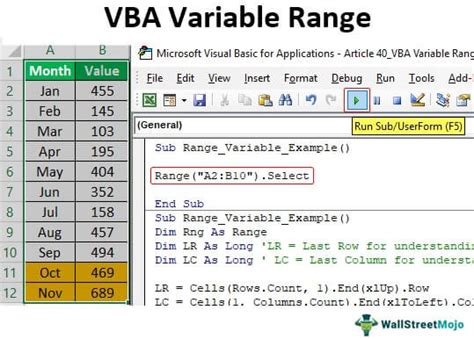
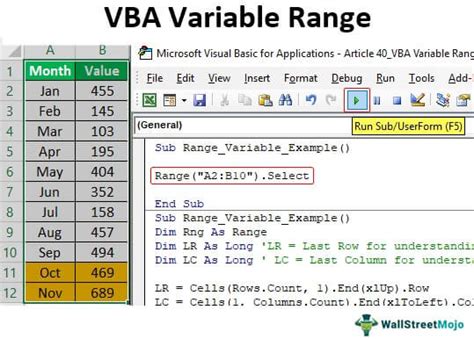
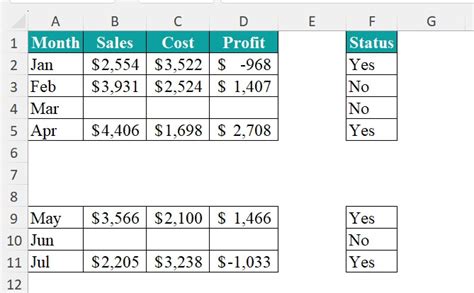