Intro
Master UVM macros to enhance your testbench functionality. Learn 4 effective ways to disable a single field with UVM macros, including using uvmfieldint and uvmfieldaa macros. Discover how to implement field disabling with UVM sequences and sequences libraries. Boost your SystemVerilog skills with expert tips on field control and testbench optimization.
Disabling a single field with UVM (Universal Verification Methodology) macros is a common requirement in the development and testing of complex digital systems. UVM is a standard for verification of digital designs, providing a set of APIs and methodologies to create reusable, modular, and scalable testbenches. When working with UVM, being able to control the behavior of specific fields within a design can be crucial for targeted testing. Here's how you can disable a single field using UVM macros, exploring four different approaches.
Understanding UVM Macros
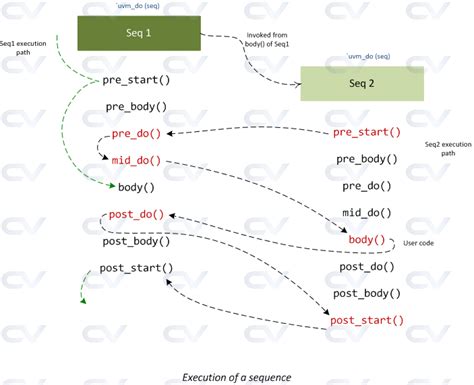
Before diving into the methods, it's essential to have a basic understanding of what UVM macros are and how they are used. UVM macros are pre-defined shortcuts that simplify writing UVM code. They help in reducing the amount of code you need to write, making your testbench more readable and maintainable.
1. Using `uvm_field_int
One of the simplest ways to disable a single field is by using the `uvm_field_int` macro. This macro is used to specify an integer field within a class. By setting the value of this field to a specific value that indicates it's disabled, you can effectively disable the field's functionality within your design.class my_transaction extends uvm_sequence_item;
rand bit [31:0] data;
`uvm_object_utils_begin(my_transaction)
`uvm_field_int(data, UVM_ALL_ON)
`uvm_object_utils_end
function void post_randomize();
if (/* some condition */) begin
data = '0; // Disabling data field
end
endfunction
endclass
Controlling Field Behavior with `uvm_field_int` Macro
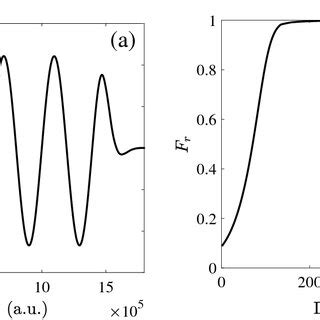
2. Utilizing `uvm_field_aa
For more complex scenarios involving arrays or associative arrays, you can use the `uvm_field_aa` macro. This macro allows you to specify how fields within arrays or associative arrays are handled during the registration process.class my_transaction extends uvm_sequence_item;
rand bit [31:0] data[];
//...
`uvm_object_utils_begin(my_transaction)
`uvm_field_aa_int(data, UVM_ALL_ON)
`uvm_object_utils_end
function void post_randomize();
if (/* some condition */) begin
// Disable data array
foreach (data[i]) begin
data[i] = '0;
end
end
endfunction
endclass
Disabling Array Fields with `uvm_field_aa` Macro
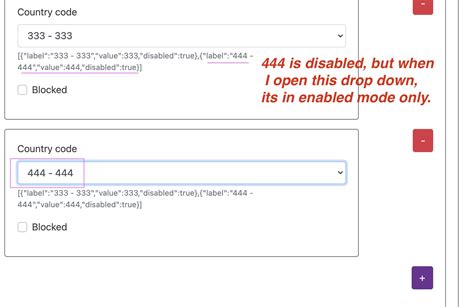
3. Using `uvm_field_saa
For scenarios involving associative arrays of strings, the `uvm_field_saa` macro is your tool of choice. This macro allows you to specify how string associative arrays are handled.class my_transaction extends uvm_sequence_item;
rand string data[string];
//...
`uvm_object_utils_begin(my_transaction)
`uvm_field_saa_string(data, UVM_ALL_ON)
`uvm_object_utils_end
function void post_randomize();
if (/* some condition */) begin
// Disable data associative array
foreach (data[key]) begin
data.delete(key);
end
end
endfunction
endclass
Handling Associative Arrays with `uvm_field_saa` Macro
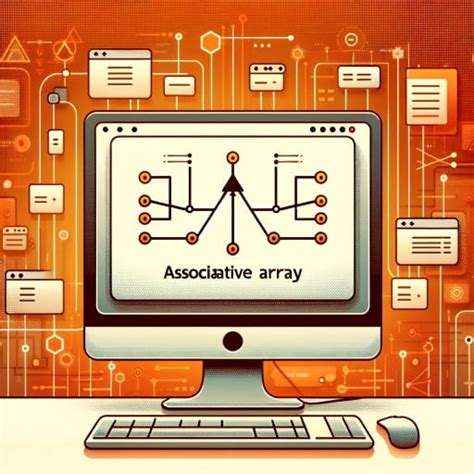
4. Employing `uvm_field_object
When dealing with complex objects, the `uvm_field_object` macro can be used. This macro is versatile and allows you to control how entire objects are handled within your testbench.class my_object extends uvm_object;
//...
class my_transaction extends uvm_sequence_item;
rand my_object obj;
//...
`uvm_object_utils_begin(my_transaction)
`uvm_field_object(obj, UVM_ALL_ON)
`uvm_object_utils_end
function void post_randomize();
if (/* some condition */) begin
// Disable obj object
obj = null;
end
endfunction
endclass
Working with Complex Objects using `uvm_field_object` Macro
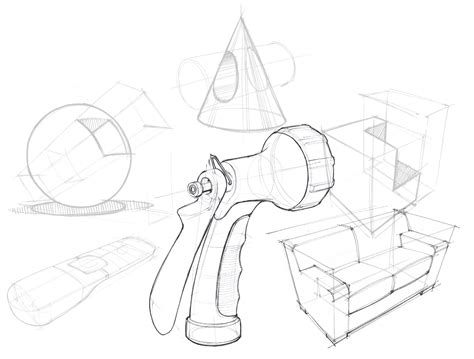
In conclusion, UVM macros offer a powerful way to control and manipulate fields within digital design verification. By understanding and leveraging the different types of UVM macros available, verification engineers can write more efficient, targeted, and effective testbenches.
Disabling Single Fields with UVM Macros: Key Takeaways
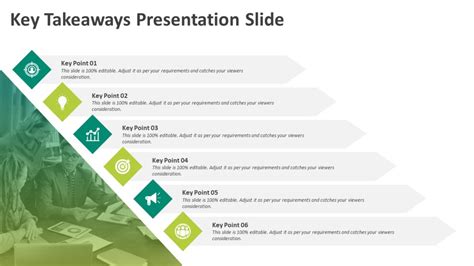
If you're looking to dive deeper into UVM macros and their applications, consider exploring the official UVM documentation and related resources.
We'd love to hear about your experiences with UVM macros and disabling single fields in your verification projects. Share your insights and challenges in the comments below!
Share Your Thoughts
UVM Macros and Digital Design Verification
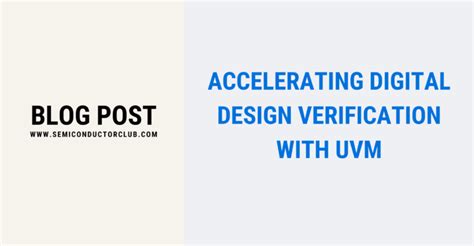
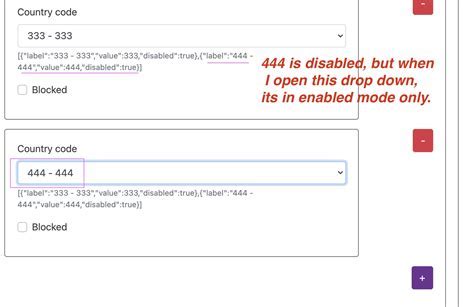
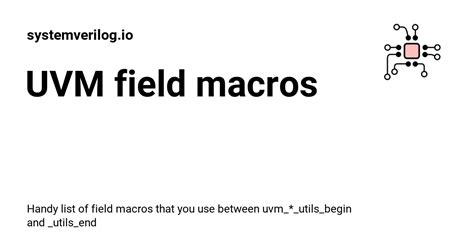
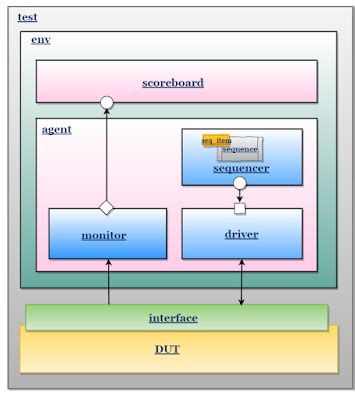
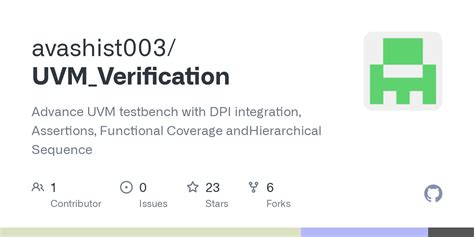
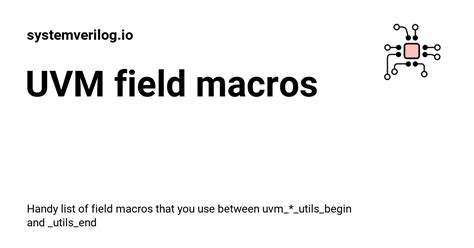
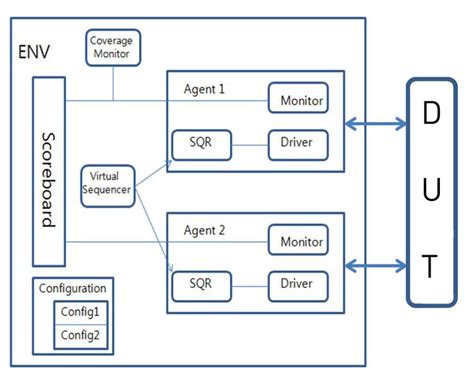
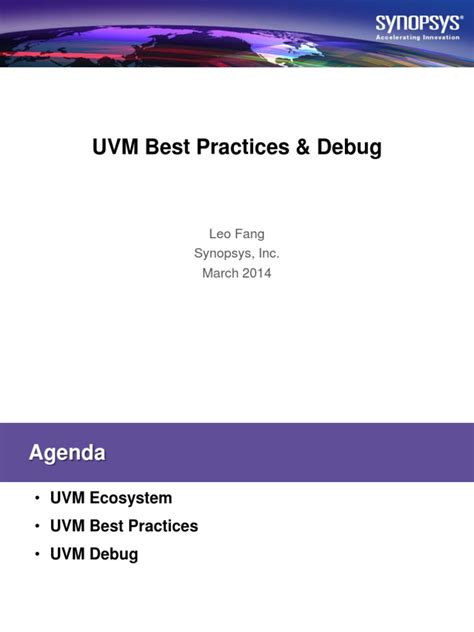
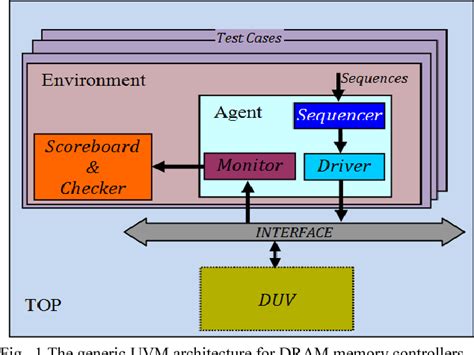
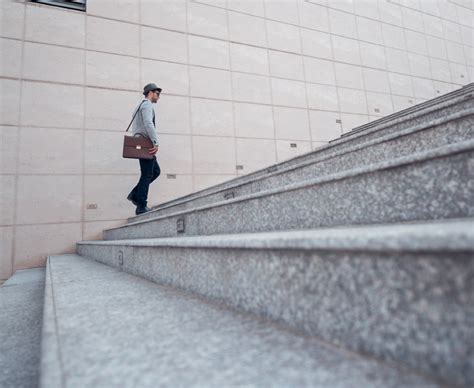