Working with data in Excel can be a daunting task, especially when dealing with large datasets. One of the most powerful features in Excel is the ability to filter data, allowing you to quickly and easily narrow down your data to specific criteria. In this article, we will explore how to add a filter to a range using VBA, making it easier to manage and analyze your data.
Why Use VBA to Add a Filter to a Range?
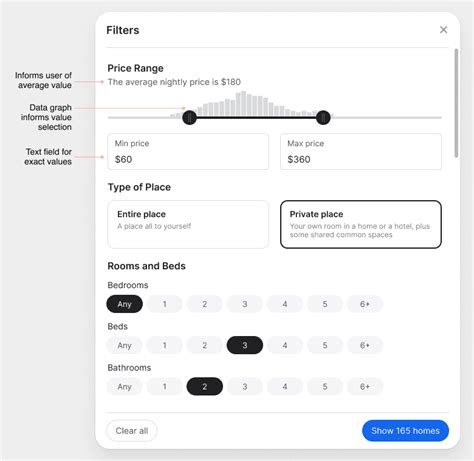
While Excel's built-in filtering feature is robust, there are times when you need more control over the filtering process. VBA (Visual Basic for Applications) provides a powerful way to automate tasks, including adding filters to ranges. By using VBA, you can create custom filters that meet your specific needs, making it easier to work with your data.
Understanding the Basics of VBA Filtering
Before we dive into the code, it's essential to understand the basics of VBA filtering. In VBA, you can use the AutoFilter
method to add a filter to a range. This method allows you to specify the range, field, and criteria for the filter.
VBA Filtering Syntax
The basic syntax for VBA filtering is as follows:
Range.AutoFilter Field:=column_number, Criteria1:=criteria
Range
is the range of cells that you want to filter.Field
is the column number that you want to filter on.Criteria1
is the criteria that you want to apply to the filter.
Adding a Filter to a Range using VBA
Now that we have covered the basics, let's take a look at an example of how to add a filter to a range using VBA.
Sub AddFilterToRange()
Dim rng As Range
Set rng = Range("A1:E10") ' Range to filter
' Add filter to column 2 (B)
rng.AutoFilter Field:=2, Criteria1:="USA"
' Add filter to column 3 (C)
rng.AutoFilter Field:=3, Criteria1:=">100"
End Sub
In this example, we define a range rng
that we want to filter. We then use the AutoFilter
method to add a filter to column 2 (B) with the criteria "USA". We also add a filter to column 3 (C) with the criteria ">100".
Clearing a Filter from a Range using VBA
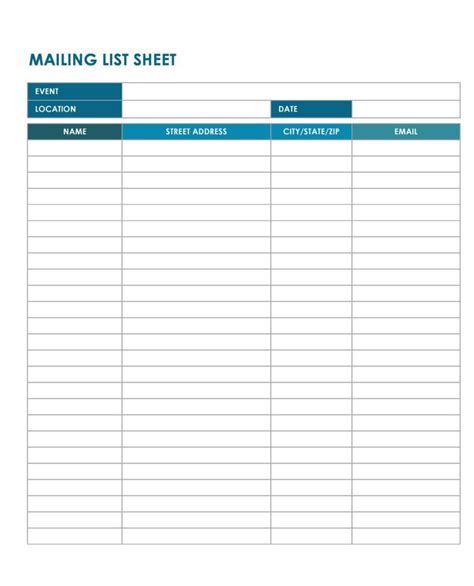
Once you have added a filter to a range, you may want to clear it. You can use the AutoFilter
method with the ShowAllData
argument set to True
to clear the filter.
Sub ClearFilterFromRange()
Dim rng As Range
Set rng = Range("A1:E10") ' Range to clear filter
' Clear filter from range
rng.AutoFilter.ShowAllData
End Sub
In this example, we define a range rng
and use the AutoFilter
method with the ShowAllData
argument set to True
to clear the filter.
Using VBA to Add Multiple Filters to a Range
You can use VBA to add multiple filters to a range by using the AutoFilter
method multiple times.
Sub AddMultipleFiltersToRange()
Dim rng As Range
Set rng = Range("A1:E10") ' Range to filter
' Add filter to column 2 (B)
rng.AutoFilter Field:=2, Criteria1:="USA"
' Add filter to column 3 (C)
rng.AutoFilter Field:=3, Criteria1:=">100"
' Add filter to column 4 (D)
rng.AutoFilter Field:=4, Criteria1:=">500"
End Sub
In this example, we define a range rng
and use the AutoFilter
method multiple times to add filters to columns 2, 3, and 4.
Gallery of Excel Filtering Images
Excel Filtering Images
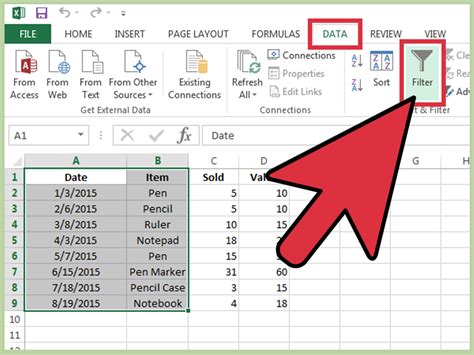
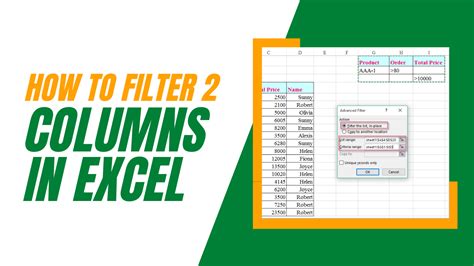
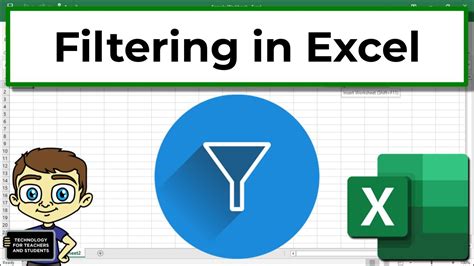
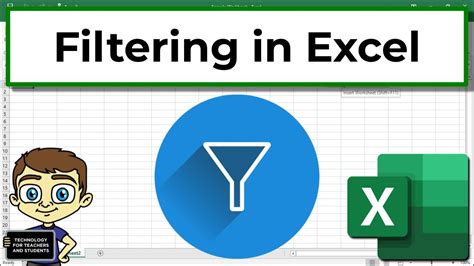
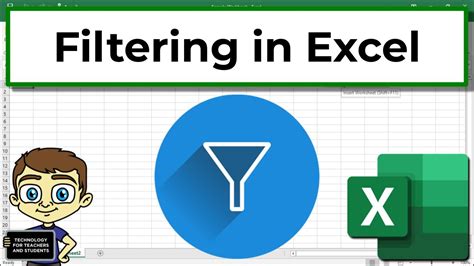
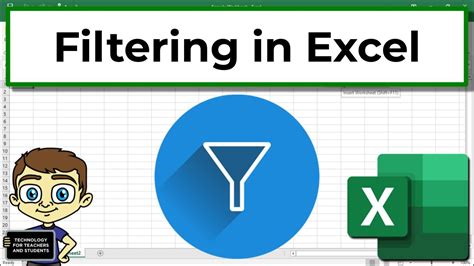
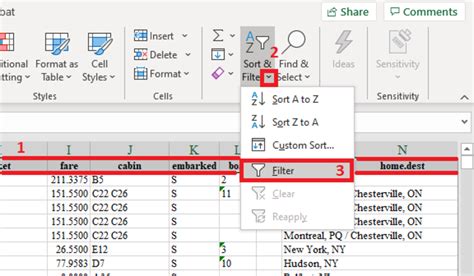
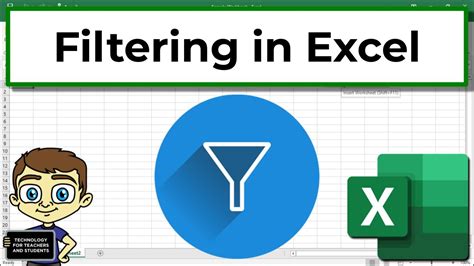
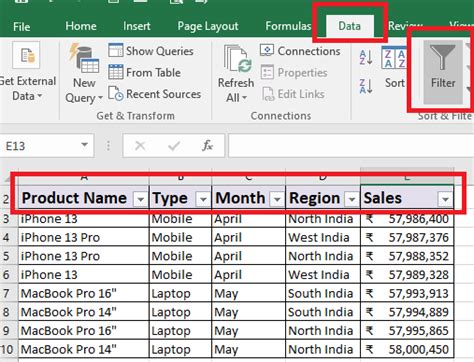
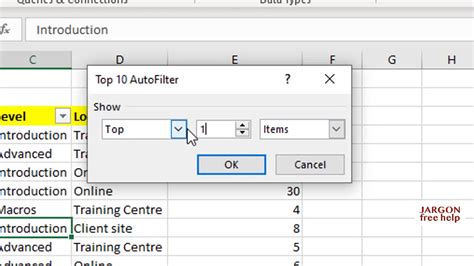
We hope this article has helped you understand how to add a filter to a range using VBA. By following the examples and code provided, you should be able to create custom filters that meet your specific needs. Remember to experiment with different criteria and filters to get the most out of your data.