Adding a new sheet in Excel using VBA is a straightforward process that can be achieved with just a few lines of code. In this article, we'll walk you through the simple steps to add a new sheet in Excel using VBA.
Why Use VBA to Add a New Sheet?
Before we dive into the code, let's quickly discuss why you might want to use VBA to add a new sheet in Excel. Here are a few scenarios:
- You need to automate the process of adding new sheets for reporting or data analysis purposes.
- You want to create a template that automatically adds new sheets based on user input.
- You're working on a complex project that requires adding multiple sheets with specific settings.
The Basics of VBA
If you're new to VBA, don't worry! VBA (Visual Basic for Applications) is a programming language developed by Microsoft that allows you to create and automate tasks in Microsoft Office applications, including Excel.
To access the VBA editor in Excel, press Alt + F11
or navigate to Developer
> Visual Basic
in the ribbon.
Adding a New Sheet with VBA
Now, let's get started with the code! To add a new sheet with VBA, you can use the following code:
Sub AddNewSheet()
' Declare a variable to hold the new sheet
Dim newSheet As Worksheet
' Add a new sheet and assign it to the variable
Set newSheet = ThisWorkbook.Worksheets.Add
' Name the new sheet
newSheet.Name = "My New Sheet"
End Sub
Let's break down what this code does:
Sub AddNewSheet()
: This line declares a new subroutine calledAddNewSheet
.Dim newSheet As Worksheet
: This line declares a variable callednewSheet
of typeWorksheet
.Set newSheet = ThisWorkbook.Worksheets.Add
: This line adds a new sheet to the active workbook and assigns it to thenewSheet
variable.newSheet.Name = "My New Sheet"
: This line names the new sheet "My New Sheet".
Tips and Variations
Here are a few tips and variations to keep in mind:
- To add a new sheet with a specific index (e.g., add a new sheet as the first sheet), use the
Worksheets.Add
method with theBefore
orAfter
parameter. - To add a new sheet with a specific template, use the
Worksheets.Add
method with theTemplate
parameter. - To add multiple new sheets at once, use a loop to repeat the
Worksheets.Add
method.
Here's an example of how to add multiple new sheets:
Sub AddMultipleSheets()
' Declare a variable to hold the number of sheets to add
Dim numSheets As Integer
' Set the number of sheets to add
numSheets = 5
' Loop through the number of sheets to add
For i = 1 To numSheets
' Add a new sheet
ThisWorkbook.Worksheets.Add
Next i
End Sub
Troubleshooting Common Errors
Here are some common errors you might encounter when adding new sheets with VBA:
- Error 1004: Method 'Add' of object 'Sheets' failed: This error occurs when the workbook is protected or when there is an issue with the worksheet template.
- Error 424: Object required: This error occurs when the
Worksheets.Add
method is not properly referenced.
To resolve these errors, check the following:
- Ensure the workbook is not protected.
- Verify that the worksheet template is correctly set up.
- Check the code for any typos or syntax errors.
Image:
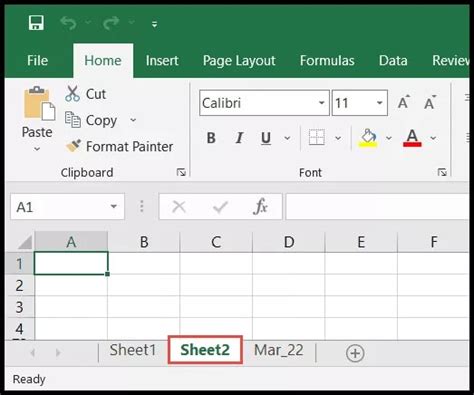
Gallery of VBA Examples
VBA Examples
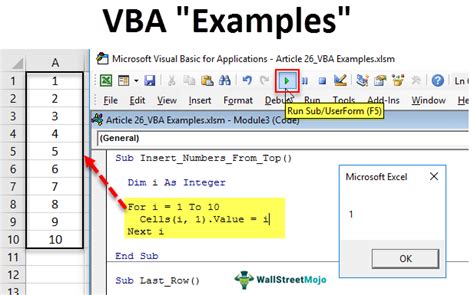
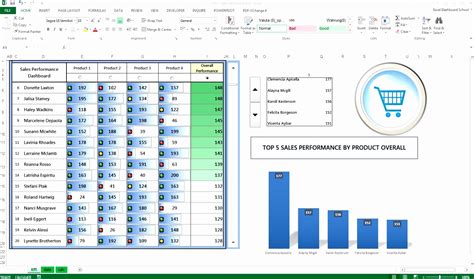
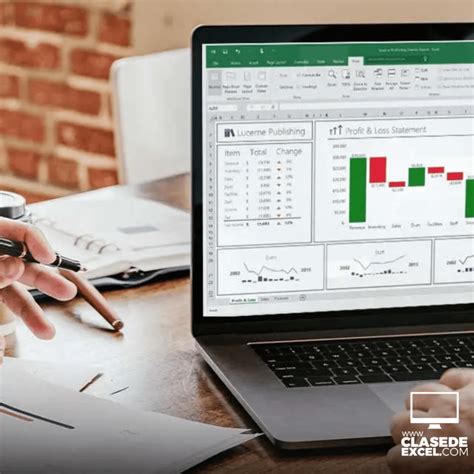
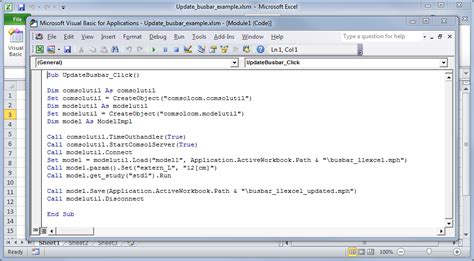
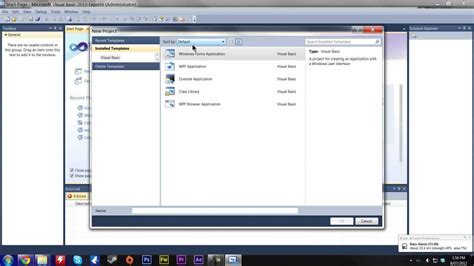
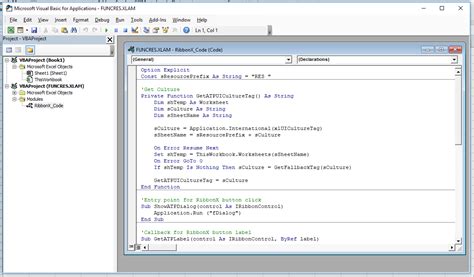
Conclusion
In this article, we've covered the simple steps to add a new sheet in Excel using VBA. We've also discussed some common errors and troubleshooting tips. Whether you're a beginner or an experienced VBA developer, we hope this article has been helpful in your automation journey.
What's Next?
Do you have any questions or comments about this article? Share them with us in the comments section below! If you'd like to learn more about VBA, check out our other tutorials and articles on the subject. Happy coding!