Intro
Troubleshoot VBA errors with ease! Discover 5 effective ways to fix the ByRef Argument Type Mismatch error in Visual Basic for Applications. Learn how to identify and correct data type mismatches, incorrect variable declarations, and more. Master VBA debugging techniques and resolve errors quickly, ensuring seamless macro execution and efficient coding.
In the realm of Visual Basic for Applications (VBA), one of the most frustrating errors that developers can encounter is the "ByRef Argument Type Mismatch" error. This error occurs when the data type of the argument passed to a procedure does not match the data type expected by the procedure. If you're struggling with this issue, don't worry, you're not alone. In this article, we'll explore the causes of this error and provide 5 practical ways to fix it.
Understanding the ByRef Argument Type Mismatch Error
The "ByRef Argument Type Mismatch" error is a common issue in VBA programming that can be challenging to resolve, especially for beginners. To understand this error, let's break down what "ByRef" means. In VBA, "ByRef" is a keyword used to pass arguments to a procedure by reference. When an argument is passed by reference, the procedure receives a reference to the original variable, allowing it to modify its value.
The error occurs when the data type of the argument passed to a procedure does not match the data type expected by the procedure. For example, if a procedure expects an integer argument but receives a string argument instead, VBA will throw a "ByRef Argument Type Mismatch" error.
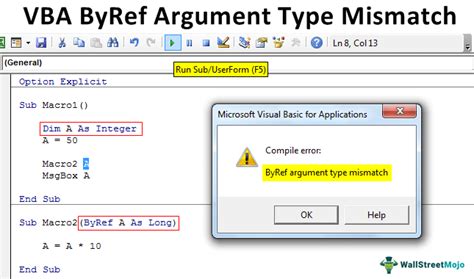
5 Ways to Fix the ByRef Argument Type Mismatch Error
Fortunately, there are several ways to fix the "ByRef Argument Type Mismatch" error in VBA. Here are 5 practical solutions to help you resolve this issue:
1. Check the Data Type of the Argument
The first step in fixing the "ByRef Argument Type Mismatch" error is to check the data type of the argument passed to the procedure. Ensure that the data type of the argument matches the data type expected by the procedure. You can use the TypeName
function to check the data type of a variable.
Sub CheckDataType()
Dim myVariable As Integer
myVariable = 10
' Check the data type of myVariable
MsgBox TypeName(myVariable)
End Sub
2. Use the Correct Data Type
Once you've identified the data type of the argument, ensure that you're using the correct data type in your procedure. For example, if a procedure expects an integer argument, make sure you're passing an integer value.
Sub UseCorrectDataType()
Dim myInteger As Integer
myInteger = 10
' Pass the integer value to the procedure
MyProcedure myInteger
End Sub
Sub MyProcedure(ByRef myArgument As Integer)
' Procedure code here
End Sub
3. Use Variants to Handle Multiple Data Types
If you need to pass different data types to a procedure, you can use variants to handle multiple data types. A variant is a data type that can hold any type of data.
Sub UseVariants()
Dim myVariant As Variant
myVariant = 10
' Pass the variant value to the procedure
MyProcedure myVariant
End Sub
Sub MyProcedure(ByRef myArgument As Variant)
' Procedure code here
End Sub
4. Use ByVal to Pass Arguments by Value
Another way to fix the "ByRef Argument Type Mismatch" error is to use the ByVal
keyword to pass arguments by value. When you pass an argument by value, a copy of the original value is passed to the procedure.
Sub UseByVal()
Dim myInteger As Integer
myInteger = 10
' Pass the integer value to the procedure using ByVal
MyProcedure ByVal myInteger
End Sub
Sub MyProcedure(myArgument As Integer)
' Procedure code here
End Sub
5. Check for Null or Empty Values
Finally, make sure to check for null or empty values before passing them to a procedure. You can use the IsNull
or IsEmpty
function to check for null or empty values.
Sub CheckForNullOrEmptyValues()
Dim myVariable As Variant
myVariable = Null
' Check if myVariable is null or empty
If IsNull(myVariable) Or IsEmpty(myVariable) Then
MsgBox "myVariable is null or empty"
Else
MyProcedure myVariable
End If
End Sub
Sub MyProcedure(ByRef myArgument As Variant)
' Procedure code here
End Sub
Gallery of VBA Error Handling
VBA Error Handling Image Gallery
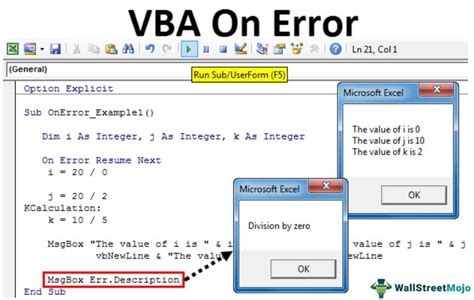
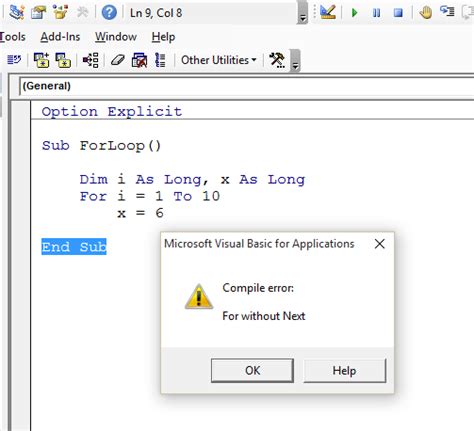
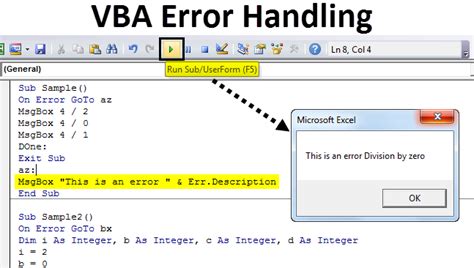
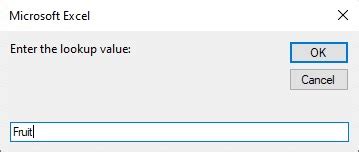
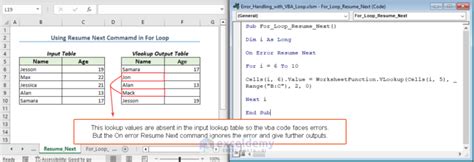
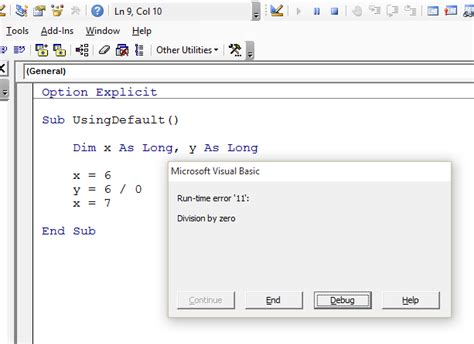
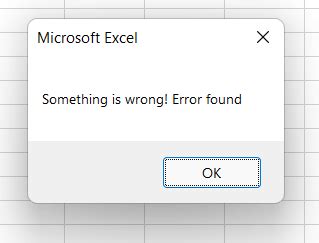
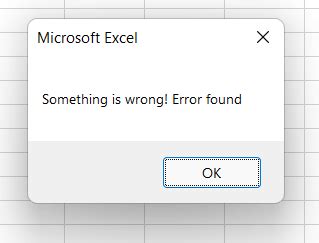
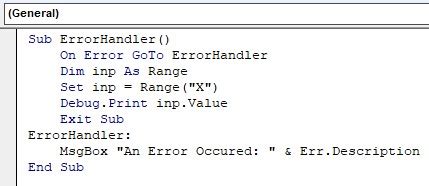
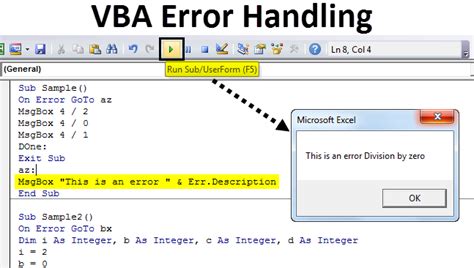
Conclusion
The "ByRef Argument Type Mismatch" error can be frustrating, but with the right techniques, you can resolve this issue quickly. By checking the data type of the argument, using the correct data type, variants, ByVal, and checking for null or empty values, you can fix this error and improve your VBA programming skills.
We hope this article has been helpful in resolving the "ByRef Argument Type Mismatch" error. If you have any questions or need further assistance, please don't hesitate to ask. Share your experiences and tips for handling VBA errors in the comments section below.