Intro
Discover how to effortlessly modify column widths in VBA with our expert guide. Learn 5 ways to adjust column width in VBA, including using Range objects, Cells property, and more. Master VBA column manipulation with our step-by-step tutorials and examples. Optimize your Excel VBA code with these practical tips and tricks.
In Microsoft Excel, manipulating column widths is a crucial aspect of data presentation and usability. When working with Visual Basic for Applications (VBA), you can programmatically adjust column widths to enhance the readability and organization of your worksheets. Here are five ways to change column width in VBA, each serving different purposes and offering flexibility in managing your Excel worksheets.
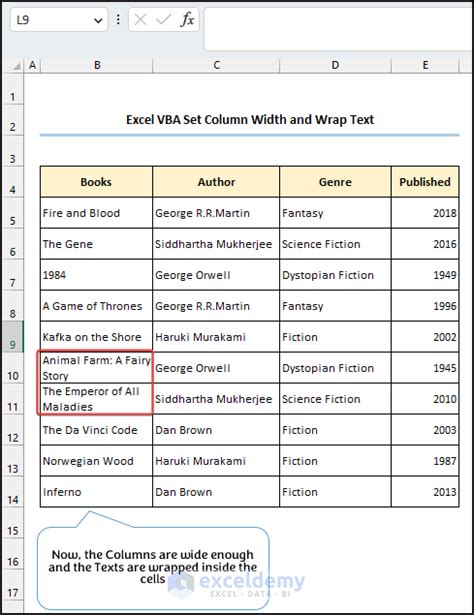
1. Adjusting Column Width to Fit Content
One of the most common reasons to adjust column widths in VBA is to ensure that all content is visible without having to manually resize columns. You can achieve this by using the AutoFit
method.
Sub AutoFitColumns()
Dim ws As Worksheet
Set ws = ActiveSheet
ws.Range("A1").EntireColumn.AutoFit
End Sub
This code snippet will automatically adjust the width of column A to fit the contents of the cells within that column.
2. Setting a Specific Column Width
Sometimes, you might want to set a specific width for one or more columns, especially when you're working with data that has a consistent character length. You can do this by directly setting the ColumnWidth
property.
Sub SetColumnWidth()
Dim ws As Worksheet
Set ws = ActiveSheet
' Setting the width of column A to 20 characters
ws.Range("A1").EntireColumn.ColumnWidth = 20
End Sub
3. Adjusting Multiple Columns at Once
If you need to adjust the widths of multiple columns simultaneously, you can select a range of columns and apply the adjustment.
Sub AdjustMultipleColumns()
Dim ws As Worksheet
Set ws = ActiveSheet
' Adjusting columns A to E to fit content
ws.Range("A:E").AutoFit
End Sub
4. Looping Through Columns to Adjust Widths Dynamically
In scenarios where you need to dynamically adjust column widths based on certain conditions (e.g., the length of the longest string in each column), you can use a loop to go through each column and adjust its width accordingly.
Sub DynamicColumnWidths()
Dim ws As Worksheet
Dim lastColumn As Long
Dim col As Long
Set ws = ActiveSheet
lastColumn = ws.Cells(1, ws.Columns.Count).End(xlToLeft).Column
For col = 1 To lastColumn
ws.Columns(col).AutoFit
Next col
End Sub
5. Adjusting All Columns in a Worksheet
For a more comprehensive approach, you might want to adjust all columns in a worksheet to fit their contents. This can be particularly useful for worksheets with a large number of columns.
Sub AutoFitAllColumns()
Dim ws As Worksheet
Set ws = ActiveSheet
ws.Cells.EntireColumn.AutoFit
End Sub
Gallery of VBA Column Width Adjustments
VBA Column Width Adjustments Gallery
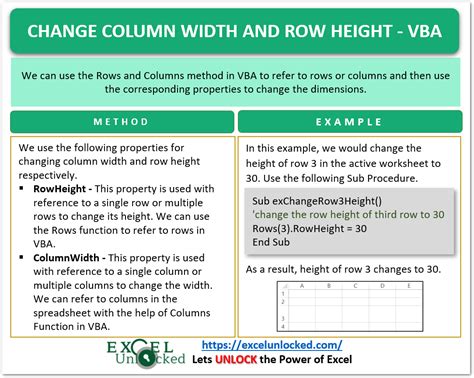
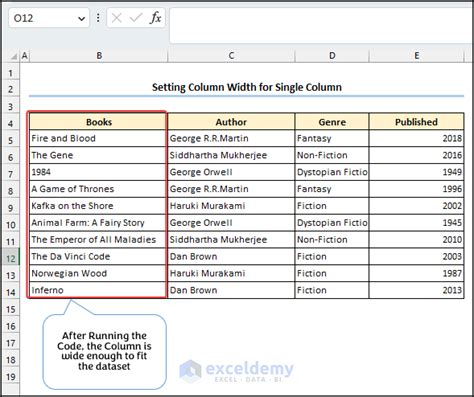
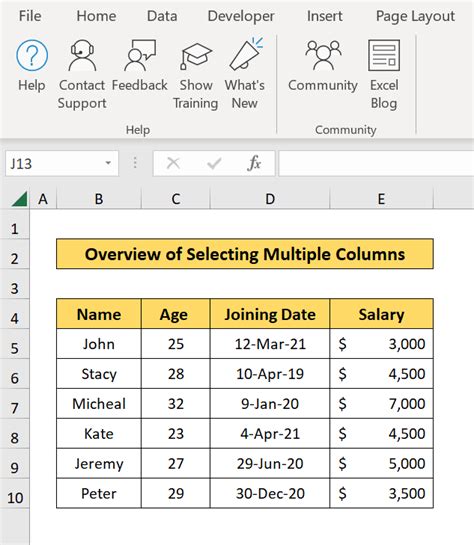
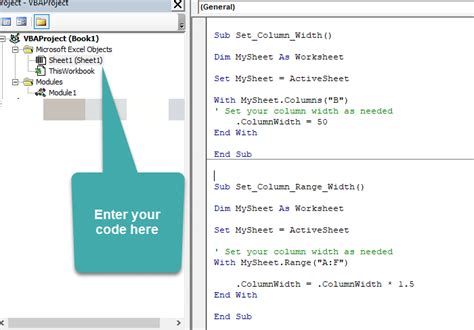
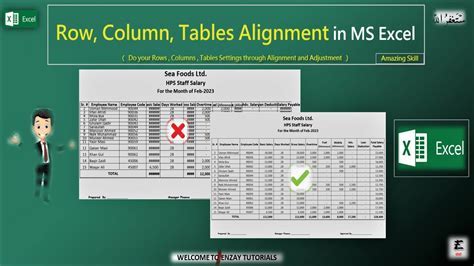
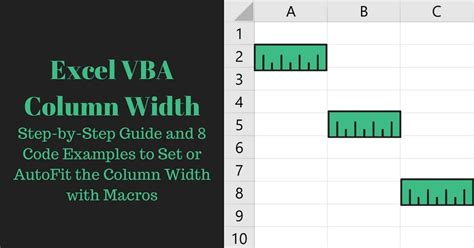
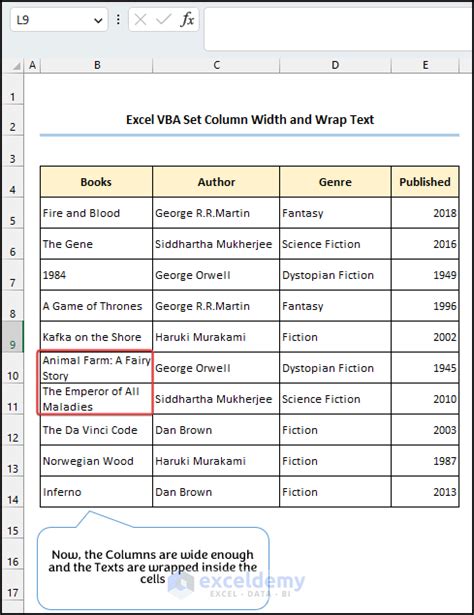
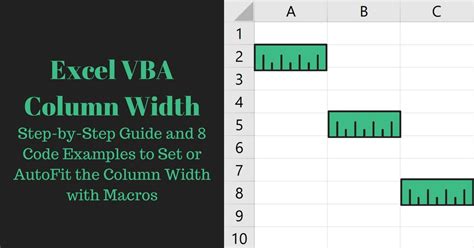
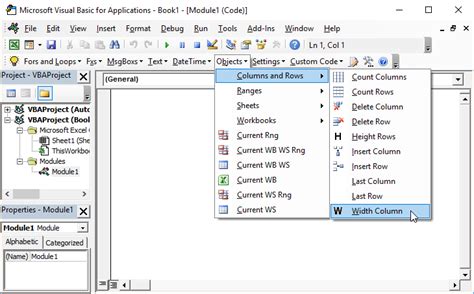
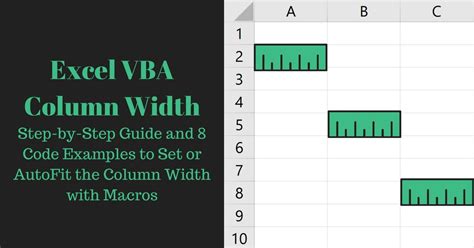
Conclusion
Manipulating column widths in Excel VBA is a versatile skill that can significantly enhance the organization and readability of your worksheets. By mastering the different methods of adjusting column widths, you can tailor your worksheets to perfectly suit your data presentation needs, whether it's for personal projects or professional applications. Feel free to experiment with these approaches and adapt them to fit your specific requirements.